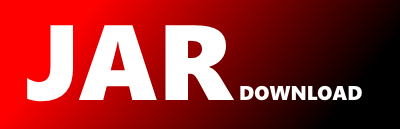
aspnetcore.Startup.mustache Maven / Gradle / Ivy
{{>partial_header}}
using System;
using System.IO;
using Microsoft.AspNetCore.Builder;
using Microsoft.AspNetCore.Hosting;
using Microsoft.Extensions.Configuration;
using Microsoft.Extensions.DependencyInjection;
using Microsoft.Extensions.Logging;
using Newtonsoft.Json.Converters;
using Newtonsoft.Json.Serialization;
using Swashbuckle.AspNetCore.Swagger;
using Swashbuckle.AspNetCore.SwaggerGen;
using {{packageName}}.Filters;
using {{packageName}}.Security;
using Microsoft.AspNetCore.Authentication;
namespace {{packageName}}
{
///
/// Startup
///
public class Startup
{
private readonly IHostingEnvironment _hostingEnv;
private IConfiguration Configuration { get; }
///
/// Constructor
///
///
///
public Startup(IHostingEnvironment env, IConfiguration configuration)
{
_hostingEnv = env;
Configuration = configuration;
}
///
/// This method gets called by the runtime. Use this method to add services to the container.
///
///
public void ConfigureServices(IServiceCollection services)
{
// Add framework services.
services
.AddMvc()
.AddJsonOptions(opts =>
{
opts.SerializerSettings.ContractResolver = new CamelCasePropertyNamesContractResolver();
opts.SerializerSettings.Converters.Add(new StringEnumConverter {
CamelCaseText = true
});
})
.AddXmlSerializerFormatters();
{{#authMethods}}
{{#isBasic}}
services.AddAuthentication(BasicAuthenticationHandler.SchemeName)
.AddScheme(BasicAuthenticationHandler.SchemeName, null);
{{/isBasic}}
{{#isBearer}}
services.AddAuthentication(BearerAuthenticationHandler.SchemeName)
.AddScheme(BearerAuthenticationHandler.SchemeName, null);
{{/isBearer}}
{{#isApiKey}}
services.AddAuthentication(ApiKeyAuthenticationHandler.SchemeName)
.AddScheme(ApiKeyAuthenticationHandler.SchemeName, null);
{{/isApiKey}}
{{/authMethods}}
services
.AddSwaggerGen(c =>
{
c.SwaggerDoc("{{#version}}{{{version}}}{{/version}}{{^version}}v1{{/version}}", new Info
{
Version = "{{#version}}{{{version}}}{{/version}}{{^version}}v1{{/version}}",
Title = "{{#appName}}{{{appName}}}{{/appName}}{{^appName}}{{packageName}}{{/appName}}",
Description = "{{#appName}}{{{appName}}}{{/appName}}{{^appName}}{{packageName}}{{/appName}} (ASP.NET Core 2.0)",
Contact = new Contact()
{
Name = "{{#infoName}}{{{infoName}}}{{/infoName}}{{^infoName}}Swagger Codegen Contributors{{/infoName}}",
Url = "{{#infoUrl}}{{{infoUrl}}}{{/infoUrl}}{{^infoUrl}}https://github.com/swagger-api/swagger-codegen{{/infoUrl}}",
Email = "{{#infoEmail}}{{{infoEmail}}}{{/infoEmail}}"
},
TermsOfService = "{{#termsOfService}}{{{termsOfService}}}{{/termsOfService}}"
});
c.CustomSchemaIds(type => type.FriendlyId(true));
c.DescribeAllEnumsAsStrings();
c.IncludeXmlComments($"{AppContext.BaseDirectory}{Path.DirectorySeparatorChar}{_hostingEnv.ApplicationName}.xml");
{{#basePathWithoutHost}}
// Sets the basePath property in the Swagger document generated
c.DocumentFilter("{{{basePathWithoutHost}}}");
{{/basePathWithoutHost}}
// Include DataAnnotation attributes on Controller Action parameters as Swagger validation rules (e.g required, pattern, ..)
// Use [ValidateModelState] on Actions to actually validate it in C# as well!
c.OperationFilter();
});
}
///
/// This method gets called by the runtime. Use this method to configure the HTTP request pipeline.
///
///
///
///
public void Configure(IApplicationBuilder app, IHostingEnvironment env, ILoggerFactory loggerFactory)
{
app
.UseMvc()
.UseDefaultFiles()
.UseStaticFiles()
.UseSwagger()
.UseSwaggerUI(c =>
{
//TODO: Either use the SwaggerGen generated Swagger contract (generated from C# classes)
c.SwaggerEndpoint("/swagger/{{#version}}{{{version}}}{{/version}}{{^version}}v1{{/version}}/swagger.json", "{{#appName}}{{{appName}}}{{/appName}}{{^appName}}{{packageName}}{{/appName}}");
//TODO: Or alternatively use the original Swagger contract that's included in the static files
// c.SwaggerEndpoint("/swagger-original.json", "{{#appName}}{{{appName}}}{{/appName}}{{^appName}}{{packageName}}{{/appName}} Original");
});
if (env.IsDevelopment())
{
app.UseDeveloperExceptionPage();
}
else
{
//TODO: Enable production exception handling (https://docs.microsoft.com/en-us/aspnet/core/fundamentals/error-handling)
// app.UseExceptionHandler("/Home/Error");
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy