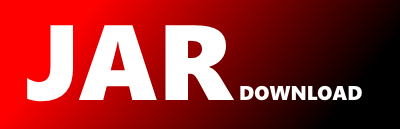
nancyfx.localDateConverter.mustache Maven / Gradle / Ivy
using Nancy.Bootstrapper;
using Nancy.Json;
using NodaTime;
using NodaTime.Text;
using System;
using System.Collections.Generic;
namespace {{packageName}}.{{packageContext}}.Utils
{
///
/// (De)serializes a to a string using
/// the RFC3339
/// full-date
format.
///
public class LocalDateConverter : JavaScriptPrimitiveConverter, IApplicationStartup
{
public override IEnumerable SupportedTypes
{
get
{
yield return typeof(LocalDate);
yield return typeof(LocalDate?);
}
}
public void Initialize(IPipelines pipelines)
{
JsonSettings.PrimitiveConverters.Add(new LocalDateConverter());
}
public override object Serialize(object obj, JavaScriptSerializer serializer)
{
if (obj is LocalDate)
{
LocalDate localDate = (LocalDate)obj;
return LocalDatePattern.IsoPattern.Format(localDate);
}
return null;
}
public override object Deserialize(object primitiveValue, Type type, JavaScriptSerializer serializer)
{
if ((type == typeof(LocalDate) || type == typeof(LocalDate?)) && primitiveValue is string)
{
try
{
return LocalDatePattern.IsoPattern.Parse(primitiveValue as string).GetValueOrThrow();
}
catch { }
}
return null;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy