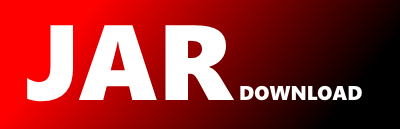
io.swagger.parser.processors.PathsProcessor Maven / Gradle / Ivy
package io.swagger.parser.processors;
import io.swagger.models.HttpMethod;
import io.swagger.models.Operation;
import io.swagger.models.Path;
import io.swagger.models.RefPath;
import io.swagger.models.Swagger;
import io.swagger.models.parameters.Parameter;
import io.swagger.parser.ResolverCache;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
public class PathsProcessor {
private final Swagger swagger;
private final ResolverCache cache;
private final ParameterProcessor parameterProcessor;
private final OperationProcessor operationProcessor;
public PathsProcessor(ResolverCache cache, Swagger swagger) {
this.swagger = swagger;
this.cache = cache;
parameterProcessor = new ParameterProcessor(cache, swagger);
operationProcessor = new OperationProcessor(cache, swagger);
}
public void processPaths() {
final Map pathMap = swagger.getPaths();
if (pathMap == null) {
return;
}
for (String pathStr : pathMap.keySet()) {
Path path = pathMap.get(pathStr);
List parameters = path.getParameters();
if(parameters != null) {
// add parameters to each operation
List operations = path.getOperations();
if(operations != null) {
for(Operation operation : operations) {
List parametersToAdd = new ArrayList();
boolean matched = false;
List existingParameters = operation.getParameters();
for(Parameter parameterToAdd : parameters) {
for(Parameter existingParameter : existingParameters) {
if(parameterToAdd.getIn() != null && parameterToAdd.getIn().equals(existingParameter.getIn()) &&
parameterToAdd.getName().equals(existingParameter.getName())) {
matched = true;
}
}
if(!matched) {
parametersToAdd.add(parameterToAdd);
}
}
if(parametersToAdd.size() > 0) {
operation.getParameters().addAll(0, parametersToAdd);
}
}
}
}
// remove the shared parameters
path.setParameters(null);
if (path instanceof RefPath) {
RefPath refPath = (RefPath) path;
Path resolvedPath = cache.loadRef(refPath.get$ref(), refPath.getRefFormat(), Path.class);
if (resolvedPath != null) {
//we need to put the resolved path into swagger object
swagger.path(pathStr, resolvedPath);
path = resolvedPath;
}
}
//at this point we can process this path
final List processedPathParameters = parameterProcessor.processParameters(path.getParameters());
path.setParameters(processedPathParameters);
final Map operationMap = path.getOperationMap();
for (HttpMethod httpMethod : operationMap.keySet()) {
Operation operation = operationMap.get(httpMethod);
operationProcessor.processOperation(operation);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy