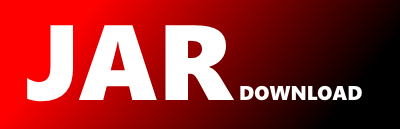
io.syndesis.common.model.action.ImmutableStepAction Maven / Gradle / Ivy
/*
* Copyright (C) 2016 Red Hat, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.syndesis.common.model.action;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.google.errorprone.annotations.CanIgnoreReturnValue;
import com.google.errorprone.annotations.Var;
import edu.umd.cs.findbugs.annotations.SuppressFBWarnings;
import io.syndesis.common.model.WithMetadata;
import io.syndesis.common.model.WithName;
import io.syndesis.common.model.WithResourceId;
import io.syndesis.common.model.WithTags;
import io.syndesis.common.util.json.StringTrimmingConverter;
import java.io.ObjectStreamException;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import java.util.NavigableSet;
import java.util.Objects;
import java.util.Optional;
import java.util.Set;
import java.util.SortedSet;
import java.util.TreeSet;
import javax.annotation.CheckReturnValue;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import javax.annotation.concurrent.NotThreadSafe;
import javax.validation.ConstraintViolation;
import javax.validation.ConstraintViolationException;
import javax.validation.Validation;
import javax.validation.Validator;
import org.immutables.value.Generated;
/**
* Immutable implementation of {@link StepAction}.
*
* Use the builder to create immutable instances:
* {@code new StepAction.Builder()}.
* Use the static factory method to create immutable instances:
* {@code ImmutableStepAction.of()}.
*/
@Generated(from = "StepAction", generator = "Immutables")
@SuppressWarnings({"all"})
@SuppressFBWarnings
@ParametersAreNonnullByDefault
@javax.annotation.Generated("org.immutables.processor.ProxyProcessor")
@Immutable
@CheckReturnValue
final class ImmutableStepAction implements StepAction {
private final @Nullable String id;
private final String name;
private final SortedSet tags;
private final Map metadata;
private final String description;
private final Action.Pattern pattern;
private final String actionType;
private final StepDescriptor descriptor;
private ImmutableStepAction(
Optional id,
String name,
Iterable tags,
Map metadata,
String description,
Action.Pattern pattern,
String actionType,
StepDescriptor descriptor) {
this.id = id.orElse(null);
this.name = name;
this.tags = createUnmodifiableSortedSet(false, createSafeList(tags, false, true));
this.metadata = createUnmodifiableMap(true, false, metadata);
this.description = description;
this.pattern = pattern;
this.actionType = Objects.requireNonNull(actionType, "actionType");
this.descriptor = descriptor;
this.initShim = null;
}
private ImmutableStepAction(ImmutableStepAction.Builder builder) {
this.id = builder.id;
this.name = builder.name;
this.tags = createUnmodifiableSortedSet(false, createSafeList(builder.tags, false, false));
this.description = builder.description;
this.pattern = builder.pattern;
this.descriptor = builder.descriptor;
if (builder.metadataIsSet()) {
initShim.metadata(createUnmodifiableMap(false, false, builder.metadata));
}
if (builder.actionType != null) {
initShim.actionType(builder.actionType);
}
this.metadata = initShim.getMetadata();
this.actionType = initShim.getActionType();
this.initShim = null;
}
private ImmutableStepAction(
ImmutableStepAction original,
@Nullable String id,
String name,
SortedSet tags,
Map metadata,
String description,
Action.Pattern pattern,
String actionType,
StepDescriptor descriptor) {
this.id = id;
this.name = name;
this.tags = tags;
this.metadata = metadata;
this.description = description;
this.pattern = pattern;
this.actionType = actionType;
this.descriptor = descriptor;
this.initShim = null;
}
private static final byte STAGE_INITIALIZING = -1;
private static final byte STAGE_UNINITIALIZED = 0;
private static final byte STAGE_INITIALIZED = 1;
@SuppressWarnings("Immutable")
private transient volatile InitShim initShim = new InitShim();
@Generated(from = "StepAction", generator = "Immutables")
private final class InitShim {
private byte metadataBuildStage = STAGE_UNINITIALIZED;
private Map metadata;
Map getMetadata() {
if (metadataBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (metadataBuildStage == STAGE_UNINITIALIZED) {
metadataBuildStage = STAGE_INITIALIZING;
this.metadata = createUnmodifiableMap(true, false, getMetadataInitialize());
metadataBuildStage = STAGE_INITIALIZED;
}
return this.metadata;
}
void metadata(Map metadata) {
this.metadata = metadata;
metadataBuildStage = STAGE_INITIALIZED;
}
private byte actionTypeBuildStage = STAGE_UNINITIALIZED;
private String actionType;
String getActionType() {
if (actionTypeBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (actionTypeBuildStage == STAGE_UNINITIALIZED) {
actionTypeBuildStage = STAGE_INITIALIZING;
this.actionType = Objects.requireNonNull(getActionTypeInitialize(), "actionType");
actionTypeBuildStage = STAGE_INITIALIZED;
}
return this.actionType;
}
void actionType(String actionType) {
this.actionType = actionType;
actionTypeBuildStage = STAGE_INITIALIZED;
}
private String formatInitCycleMessage() {
List attributes = new ArrayList<>();
if (metadataBuildStage == STAGE_INITIALIZING) attributes.add("metadata");
if (actionTypeBuildStage == STAGE_INITIALIZING) attributes.add("actionType");
return "Cannot build StepAction, attribute initializers form cycle " + attributes;
}
}
private Map getMetadataInitialize() {
return StepAction.super.getMetadata();
}
private String getActionTypeInitialize() {
return StepAction.super.getActionType();
}
/**
* @return The value of the {@code id} attribute
*/
@JsonProperty("id")
@Override
public Optional getId() {
return Optional.ofNullable(id);
}
/**
* @return The value of the {@code name} attribute
*/
@JsonProperty("name")
@JsonDeserialize(converter = StringTrimmingConverter.class)
@Override
public String getName() {
return name;
}
/**
* @return The value of the {@code tags} attribute
*/
@JsonProperty("tags")
@JsonDeserialize(contentConverter = StringTrimmingConverter.class)
@Override
public SortedSet getTags() {
return tags;
}
/**
* @return The value of the {@code metadata} attribute
*/
@JsonProperty("metadata")
@Override
public Map getMetadata() {
InitShim shim = this.initShim;
return shim != null
? shim.getMetadata()
: this.metadata;
}
/**
* @return The value of the {@code description} attribute
*/
@JsonProperty("description")
@Override
public String getDescription() {
return description;
}
/**
* @return The value of the {@code pattern} attribute
*/
@JsonProperty("pattern")
@Override
public Action.Pattern getPattern() {
return pattern;
}
/**
* @return The value of the {@code actionType} attribute
*/
@JsonProperty("actionType")
@Override
public String getActionType() {
InitShim shim = this.initShim;
return shim != null
? shim.getActionType()
: this.actionType;
}
/**
* @return The value of the {@code descriptor} attribute
*/
@JsonProperty("descriptor")
@Override
public StepDescriptor getDescriptor() {
return descriptor;
}
/**
* Copy the current immutable object by setting a present value for the optional {@link StepAction#getId() id} attribute.
* @param value The value for id
* @return A modified copy of {@code this} object
*/
public final ImmutableStepAction withId(String value) {
@Nullable String newValue = Objects.requireNonNull(value, "id");
if (Objects.equals(this.id, newValue)) return this;
return validate(new ImmutableStepAction(
this,
newValue,
this.name,
this.tags,
this.metadata,
this.description,
this.pattern,
this.actionType,
this.descriptor));
}
/**
* Copy the current immutable object by setting an optional value for the {@link StepAction#getId() id} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for id
* @return A modified copy of {@code this} object
*/
public final ImmutableStepAction withId(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.id, value)) return this;
return validate(new ImmutableStepAction(
this,
value,
this.name,
this.tags,
this.metadata,
this.description,
this.pattern,
this.actionType,
this.descriptor));
}
/**
* Copy the current immutable object by setting a value for the {@link StepAction#getName() name} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for name (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableStepAction withName(String value) {
if (Objects.equals(this.name, value)) return this;
return validate(new ImmutableStepAction(
this,
this.id,
value,
this.tags,
this.metadata,
this.description,
this.pattern,
this.actionType,
this.descriptor));
}
/**
* Copy the current immutable object with elements that replace the content of {@link StepAction#getTags() tags}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableStepAction withTags(String... elements) {
SortedSet newValue = createUnmodifiableSortedSet(false, createSafeList(Arrays.asList(elements), false, true));
return validate(new ImmutableStepAction(
this,
this.id,
this.name,
newValue,
this.metadata,
this.description,
this.pattern,
this.actionType,
this.descriptor));
}
/**
* Copy the current immutable object with elements that replace the content of {@link StepAction#getTags() tags}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of tags elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableStepAction withTags(Iterable elements) {
if (this.tags == elements) return this;
SortedSet newValue = createUnmodifiableSortedSet(false, createSafeList(elements, false, true));
return validate(new ImmutableStepAction(
this,
this.id,
this.name,
newValue,
this.metadata,
this.description,
this.pattern,
this.actionType,
this.descriptor));
}
/**
* Copy the current immutable object by replacing the {@link StepAction#getMetadata() metadata} map with the specified map.
* Nulls are not permitted as keys or values.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param entries The entries to be added to the metadata map
* @return A modified copy of {@code this} object
*/
public final ImmutableStepAction withMetadata(Map entries) {
if (this.metadata == entries) return this;
Map newValue = createUnmodifiableMap(true, false, entries);
return validate(new ImmutableStepAction(
this,
this.id,
this.name,
this.tags,
newValue,
this.description,
this.pattern,
this.actionType,
this.descriptor));
}
/**
* Copy the current immutable object by setting a value for the {@link StepAction#getDescription() description} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for description (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableStepAction withDescription(String value) {
if (Objects.equals(this.description, value)) return this;
return validate(new ImmutableStepAction(
this,
this.id,
this.name,
this.tags,
this.metadata,
value,
this.pattern,
this.actionType,
this.descriptor));
}
/**
* Copy the current immutable object by setting a value for the {@link StepAction#getPattern() pattern} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for pattern (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableStepAction withPattern(Action.Pattern value) {
if (this.pattern == value) return this;
if (Objects.equals(this.pattern, value)) return this;
return validate(new ImmutableStepAction(
this,
this.id,
this.name,
this.tags,
this.metadata,
this.description,
value,
this.actionType,
this.descriptor));
}
/**
* Copy the current immutable object by setting a value for the {@link StepAction#getActionType() actionType} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for actionType
* @return A modified copy of the {@code this} object
*/
public final ImmutableStepAction withActionType(String value) {
String newValue = Objects.requireNonNull(value, "actionType");
if (this.actionType.equals(newValue)) return this;
return validate(new ImmutableStepAction(
this,
this.id,
this.name,
this.tags,
this.metadata,
this.description,
this.pattern,
newValue,
this.descriptor));
}
/**
* Copy the current immutable object by setting a value for the {@link StepAction#getDescriptor() descriptor} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for descriptor (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableStepAction withDescriptor(StepDescriptor value) {
if (this.descriptor == value) return this;
return validate(new ImmutableStepAction(
this,
this.id,
this.name,
this.tags,
this.metadata,
this.description,
this.pattern,
this.actionType,
value));
}
/**
* This instance is equal to all instances of {@code ImmutableStepAction} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof ImmutableStepAction
&& equalTo((ImmutableStepAction) another);
}
private boolean equalTo(ImmutableStepAction another) {
return Objects.equals(id, another.id)
&& Objects.equals(name, another.name)
&& tags.equals(another.tags)
&& metadata.equals(another.metadata)
&& Objects.equals(description, another.description)
&& Objects.equals(pattern, another.pattern)
&& actionType.equals(another.actionType)
&& Objects.equals(descriptor, another.descriptor);
}
/**
* Computes a hash code from attributes: {@code id}, {@code name}, {@code tags}, {@code metadata}, {@code description}, {@code pattern}, {@code actionType}, {@code descriptor}.
* @return hashCode value
*/
@Override
public int hashCode() {
@Var int h = 5381;
h += (h << 5) + Objects.hashCode(id);
h += (h << 5) + Objects.hashCode(name);
h += (h << 5) + tags.hashCode();
h += (h << 5) + metadata.hashCode();
h += (h << 5) + Objects.hashCode(description);
h += (h << 5) + Objects.hashCode(pattern);
h += (h << 5) + actionType.hashCode();
h += (h << 5) + Objects.hashCode(descriptor);
return h;
}
/**
* Prints the immutable value {@code StepAction} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
StringBuilder builder = new StringBuilder("StepAction{");
if (id != null) {
builder.append("id=").append(id);
}
if (name != null) {
if (builder.length() > 11) builder.append(", ");
builder.append("name=").append(name);
}
if (builder.length() > 11) builder.append(", ");
builder.append("tags=").append(tags);
builder.append(", ");
builder.append("metadata=").append(metadata);
if (description != null) {
builder.append(", ");
builder.append("description=").append(description);
}
if (pattern != null) {
builder.append(", ");
builder.append("pattern=").append(pattern);
}
builder.append(", ");
builder.append("actionType=").append(actionType);
if (descriptor != null) {
builder.append(", ");
builder.append("descriptor=").append(descriptor);
}
return builder.append("}").toString();
}
/**
* Construct a new immutable {@code StepAction} instance.
* @param id The value for the {@code id} attribute
* @param name The value for the {@code name} attribute
* @param tags The value for the {@code tags} attribute
* @param metadata The value for the {@code metadata} attribute
* @param description The value for the {@code description} attribute
* @param pattern The value for the {@code pattern} attribute
* @param actionType The value for the {@code actionType} attribute
* @param descriptor The value for the {@code descriptor} attribute
* @return An immutable StepAction instance
*/
public static StepAction of(Optional id, String name, SortedSet tags, Map metadata, String description, Action.Pattern pattern, String actionType, StepDescriptor descriptor) {
return of(id, name, (Iterable) tags, metadata, description, pattern, actionType, descriptor);
}
/**
* Construct a new immutable {@code StepAction} instance.
* @param id The value for the {@code id} attribute
* @param name The value for the {@code name} attribute
* @param tags The value for the {@code tags} attribute
* @param metadata The value for the {@code metadata} attribute
* @param description The value for the {@code description} attribute
* @param pattern The value for the {@code pattern} attribute
* @param actionType The value for the {@code actionType} attribute
* @param descriptor The value for the {@code descriptor} attribute
* @return An immutable StepAction instance
*/
public static StepAction of(Optional id, String name, Iterable tags, Map metadata, String description, Action.Pattern pattern, String actionType, StepDescriptor descriptor) {
return validate(new ImmutableStepAction(id, name, tags, metadata, description, pattern, actionType, descriptor));
}
private static final Validator validator = Validation.buildDefaultValidatorFactory().getValidator();
private static ImmutableStepAction validate(ImmutableStepAction instance) {
Set> constraintViolations = validator.validate(instance);
if (!constraintViolations.isEmpty()) {
throw new ConstraintViolationException(constraintViolations);
}
return instance;
}
/**
* Creates an immutable copy of a {@link StepAction} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable StepAction instance
*/
public static StepAction copyOf(StepAction instance) {
if (instance instanceof ImmutableStepAction) {
return (ImmutableStepAction) instance;
}
return new StepAction.Builder()
.createFrom(instance)
.build();
}
private Object readResolve() throws ObjectStreamException {
return validate(this);
}
/**
* Builds instances of type {@link StepAction StepAction}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@Generated(from = "StepAction", generator = "Immutables")
@NotThreadSafe
public static class Builder {
private static final long OPT_BIT_METADATA = 0x1L;
private long optBits;
private @Nullable String id;
private @Nullable String name;
private List tags = new ArrayList();
private Map metadata = new LinkedHashMap();
private @Nullable String description;
private @Nullable Action.Pattern pattern;
private @Nullable String actionType;
private @Nullable StepDescriptor descriptor;
/**
* Creates a builder for {@link StepAction StepAction} instances.
*
* new StepAction.Builder()
* .id(String) // optional {@link StepAction#getId() id}
* .name(String | null) // nullable {@link StepAction#getName() name}
* .addTag|addAllTags(String) // {@link StepAction#getTags() tags} elements
* .putMetadata|putAllMetadata(String => String) // {@link StepAction#getMetadata() metadata} mappings
* .description(String | null) // nullable {@link StepAction#getDescription() description}
* .pattern(io.syndesis.common.model.action.Action.Pattern | null) // nullable {@link StepAction#getPattern() pattern}
* .actionType(String) // optional {@link StepAction#getActionType() actionType}
* .descriptor(io.syndesis.common.model.action.StepDescriptor | null) // nullable {@link StepAction#getDescriptor() descriptor}
* .build();
*
*/
public Builder() {
if (!(this instanceof StepAction.Builder)) {
throw new UnsupportedOperationException("Use: new StepAction.Builder()");
}
}
/**
* Fill a builder with attribute values from the provided {@code io.syndesis.common.model.WithName} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final StepAction.Builder createFrom(WithName instance) {
Objects.requireNonNull(instance, "instance");
from((Object) instance);
return (StepAction.Builder) this;
}
/**
* Fill a builder with attribute values from the provided {@code io.syndesis.common.model.action.Action} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final StepAction.Builder createFrom(Action instance) {
Objects.requireNonNull(instance, "instance");
from((Object) instance);
return (StepAction.Builder) this;
}
/**
* Fill a builder with attribute values from the provided {@code io.syndesis.common.model.action.StepAction} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final StepAction.Builder createFrom(StepAction instance) {
Objects.requireNonNull(instance, "instance");
from((Object) instance);
return (StepAction.Builder) this;
}
/**
* Fill a builder with attribute values from the provided {@code io.syndesis.common.model.WithMetadata} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final StepAction.Builder createFrom(WithMetadata instance) {
Objects.requireNonNull(instance, "instance");
from((Object) instance);
return (StepAction.Builder) this;
}
/**
* Fill a builder with attribute values from the provided {@code io.syndesis.common.model.WithResourceId} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final StepAction.Builder createFrom(WithResourceId instance) {
Objects.requireNonNull(instance, "instance");
from((Object) instance);
return (StepAction.Builder) this;
}
/**
* Fill a builder with attribute values from the provided {@code io.syndesis.common.model.WithTags} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final StepAction.Builder createFrom(WithTags instance) {
Objects.requireNonNull(instance, "instance");
from((Object) instance);
return (StepAction.Builder) this;
}
private void from(Object object) {
@Var long bits = 0;
if (object instanceof WithName) {
WithName instance = (WithName) object;
String nameValue = instance.getName();
if (nameValue != null) {
name(nameValue);
}
}
if (object instanceof Action) {
Action instance = (Action) object;
Action.Pattern patternValue = instance.getPattern();
if (patternValue != null) {
pattern(patternValue);
}
if ((bits & 0x1L) == 0) {
actionType(instance.getActionType());
bits |= 0x1L;
}
String descriptionValue = instance.getDescription();
if (descriptionValue != null) {
description(descriptionValue);
}
}
if (object instanceof StepAction) {
StepAction instance = (StepAction) object;
if ((bits & 0x1L) == 0) {
actionType(instance.getActionType());
bits |= 0x1L;
}
StepDescriptor descriptorValue = instance.getDescriptor();
if (descriptorValue != null) {
descriptor(descriptorValue);
}
}
if (object instanceof WithMetadata) {
WithMetadata instance = (WithMetadata) object;
putAllMetadata(instance.getMetadata());
}
if (object instanceof WithResourceId) {
WithResourceId instance = (WithResourceId) object;
Optional idOptional = instance.getId();
if (idOptional.isPresent()) {
id(idOptional);
}
}
if (object instanceof WithTags) {
WithTags instance = (WithTags) object;
addAllTags(instance.getTags());
}
}
/**
* Initializes the optional value {@link StepAction#getId() id} to id.
* @param id The value for id
* @return {@code this} builder for chained invocation
*/
@CanIgnoreReturnValue
public final StepAction.Builder id(String id) {
this.id = Objects.requireNonNull(id, "id");
return (StepAction.Builder) this;
}
/**
* Initializes the optional value {@link StepAction#getId() id} to id.
* @param id The value for id
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("id")
public final StepAction.Builder id(Optional id) {
this.id = id.orElse(null);
return (StepAction.Builder) this;
}
/**
* Initializes the value for the {@link StepAction#getName() name} attribute.
* @param name The value for name (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("name")
@JsonDeserialize(converter = StringTrimmingConverter.class)
public final StepAction.Builder name(String name) {
this.name = name;
return (StepAction.Builder) this;
}
/**
* Adds one element to {@link StepAction#getTags() tags} sortedSet.
* @param element A tags element
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final StepAction.Builder addTag(@Nullable String element) {
if (element != null) this.tags.add(element);
return (StepAction.Builder) this;
}
/**
* Adds elements to {@link StepAction#getTags() tags} sortedSet.
* @param elements An array of tags elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final StepAction.Builder addTags(String... elements) {
for (String element : elements) {
if (element != null) this.tags.add(element);
}
return (StepAction.Builder) this;
}
/**
* Sets or replaces all elements for {@link StepAction#getTags() tags} sortedSet.
* @param elements An iterable of tags elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("tags")
@JsonDeserialize(contentConverter = StringTrimmingConverter.class)
public final StepAction.Builder tags(Iterable elements) {
this.tags.clear();
return addAllTags(elements);
}
/**
* Adds elements to {@link StepAction#getTags() tags} sortedSet.
* @param elements An iterable of tags elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final StepAction.Builder addAllTags(Iterable elements) {
for (String element : elements) {
if (element != null) this.tags.add(element);
}
return (StepAction.Builder) this;
}
/**
* Put one entry to the {@link StepAction#getMetadata() metadata} map.
* @param key The key in the metadata map
* @param value The associated value in the metadata map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final StepAction.Builder putMetadata(String key, String value) {
this.metadata.put(
Objects.requireNonNull(key, "metadata key"),
Objects.requireNonNull(value, "metadata value"));
optBits |= OPT_BIT_METADATA;
return (StepAction.Builder) this;
}
/**
* Put one entry to the {@link StepAction#getMetadata() metadata} map. Nulls are not permitted
* @param entry The key and value entry
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final StepAction.Builder putMetadata(Map.Entry entry) {
String k = entry.getKey();
String v = entry.getValue();
this.metadata.put(
Objects.requireNonNull(k, "metadata key"),
Objects.requireNonNull(v, "metadata value"));
optBits |= OPT_BIT_METADATA;
return (StepAction.Builder) this;
}
/**
* Sets or replaces all mappings from the specified map as entries for the {@link StepAction#getMetadata() metadata} map. Nulls are not permitted
* @param entries The entries that will be added to the metadata map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("metadata")
public final StepAction.Builder metadata(Map entries) {
this.metadata.clear();
optBits |= OPT_BIT_METADATA;
return putAllMetadata(entries);
}
/**
* Put all mappings from the specified map as entries to {@link StepAction#getMetadata() metadata} map. Nulls are not permitted
* @param entries The entries that will be added to the metadata map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final StepAction.Builder putAllMetadata(Map entries) {
for (Map.Entry e : entries.entrySet()) {
String k = e.getKey();
String v = e.getValue();
this.metadata.put(
Objects.requireNonNull(k, "metadata key"),
Objects.requireNonNull(v, "metadata value"));
}
optBits |= OPT_BIT_METADATA;
return (StepAction.Builder) this;
}
/**
* Initializes the value for the {@link StepAction#getDescription() description} attribute.
* @param description The value for description (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("description")
public final StepAction.Builder description(String description) {
this.description = description;
return (StepAction.Builder) this;
}
/**
* Initializes the value for the {@link StepAction#getPattern() pattern} attribute.
* @param pattern The value for pattern (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("pattern")
public final StepAction.Builder pattern(Action.Pattern pattern) {
this.pattern = pattern;
return (StepAction.Builder) this;
}
/**
* Initializes the value for the {@link StepAction#getActionType() actionType} attribute.
* If not set, this attribute will have a default value as returned by the initializer of {@link StepAction#getActionType() actionType}.
* @param actionType The value for actionType
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("actionType")
public final StepAction.Builder actionType(String actionType) {
this.actionType = Objects.requireNonNull(actionType, "actionType");
return (StepAction.Builder) this;
}
/**
* Initializes the value for the {@link StepAction#getDescriptor() descriptor} attribute.
* @param descriptor The value for descriptor (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("descriptor")
public final StepAction.Builder descriptor(StepDescriptor descriptor) {
this.descriptor = descriptor;
return (StepAction.Builder) this;
}
/**
* Builds a new {@link StepAction StepAction}.
* @return An immutable instance of StepAction
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public StepAction build() {
return ImmutableStepAction.validate(new ImmutableStepAction(this));
}
private boolean metadataIsSet() {
return (optBits & OPT_BIT_METADATA) != 0;
}
}
private static List createSafeList(Iterable extends T> iterable, boolean checkNulls, boolean skipNulls) {
ArrayList list;
if (iterable instanceof Collection>) {
int size = ((Collection>) iterable).size();
if (size == 0) return Collections.emptyList();
list = new ArrayList<>();
} else {
list = new ArrayList<>();
}
for (T element : iterable) {
if (skipNulls && element == null) continue;
if (checkNulls) Objects.requireNonNull(element, "element");
list.add(element);
}
return list;
}
private static > NavigableSet createUnmodifiableSortedSet(boolean reverse, List list) {
TreeSet set = reverse
? new TreeSet<>(Collections.reverseOrder())
: new TreeSet<>();
set.addAll(list);
return Collections.unmodifiableNavigableSet(set);
}
private static Map createUnmodifiableMap(boolean checkNulls, boolean skipNulls, Map extends K, ? extends V> map) {
switch (map.size()) {
case 0: return Collections.emptyMap();
case 1: {
Map.Entry extends K, ? extends V> e = map.entrySet().iterator().next();
K k = e.getKey();
V v = e.getValue();
if (checkNulls) {
Objects.requireNonNull(k, "key");
Objects.requireNonNull(v, "value");
}
if (skipNulls && (k == null || v == null)) {
return Collections.emptyMap();
}
return Collections.singletonMap(k, v);
}
default: {
Map linkedMap = new LinkedHashMap<>(map.size());
if (skipNulls || checkNulls) {
for (Map.Entry extends K, ? extends V> e : map.entrySet()) {
K k = e.getKey();
V v = e.getValue();
if (skipNulls) {
if (k == null || v == null) continue;
} else if (checkNulls) {
Objects.requireNonNull(k, "key");
Objects.requireNonNull(v, "value");
}
linkedMap.put(k, v);
}
} else {
linkedMap.putAll(map);
}
return Collections.unmodifiableMap(linkedMap);
}
}
}
}