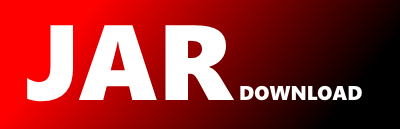
io.syndesis.common.model.api.ImmutableAPISummary Maven / Gradle / Ivy
/*
* Copyright (C) 2016 Red Hat, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.syndesis.common.model.api;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.google.errorprone.annotations.CanIgnoreReturnValue;
import com.google.errorprone.annotations.Var;
import edu.umd.cs.findbugs.annotations.SuppressFBWarnings;
import io.syndesis.common.model.Violation;
import io.syndesis.common.model.WithConfigurationProperties;
import io.syndesis.common.model.WithConfiguredProperties;
import io.syndesis.common.model.WithName;
import io.syndesis.common.model.action.ActionsSummary;
import io.syndesis.common.model.connection.ConfigurationProperty;
import io.syndesis.common.util.json.StringTrimmingConverter;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.Set;
import javax.annotation.CheckReturnValue;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import javax.annotation.concurrent.NotThreadSafe;
import javax.validation.ConstraintViolation;
import javax.validation.ConstraintViolationException;
import javax.validation.Validation;
import javax.validation.Validator;
import org.immutables.value.Generated;
/**
* Immutable implementation of {@link APISummary}.
*
* Use the builder to create immutable instances:
* {@code new APISummary.Builder()}.
* Use the static factory method to create immutable instances:
* {@code ImmutableAPISummary.of()}.
*/
@Generated(from = "APISummary", generator = "Immutables")
@SuppressWarnings({"all"})
@SuppressFBWarnings
@ParametersAreNonnullByDefault
@javax.annotation.Generated("org.immutables.processor.ProxyProcessor")
@Immutable
@CheckReturnValue
final class ImmutableAPISummary implements APISummary {
private final String name;
private final Map properties;
private final Map configuredProperties;
private final ActionsSummary actionsSummary;
private final String description;
private final List errors;
private final @Nullable String icon;
private final List warnings;
private ImmutableAPISummary(
String name,
Map properties,
Map configuredProperties,
ActionsSummary actionsSummary,
String description,
Iterable extends Violation> errors,
Optional icon,
Iterable extends Violation> warnings) {
this.name = name;
this.properties = createUnmodifiableMap(true, false, properties);
this.configuredProperties = createUnmodifiableMap(true, false, configuredProperties);
this.actionsSummary = actionsSummary;
this.description = description;
this.errors = createUnmodifiableList(false, createSafeList(errors, true, false));
this.icon = icon.orElse(null);
this.warnings = createUnmodifiableList(false, createSafeList(warnings, true, false));
}
private ImmutableAPISummary(ImmutableAPISummary.Builder builder) {
this.name = builder.name;
this.properties = createUnmodifiableMap(false, false, builder.properties);
this.actionsSummary = builder.actionsSummary;
this.description = builder.description;
this.errors = createUnmodifiableList(true, builder.errors);
this.icon = builder.icon;
this.warnings = createUnmodifiableList(true, builder.warnings);
this.configuredProperties = builder.configuredPropertiesIsSet()
? createUnmodifiableMap(false, false, builder.configuredProperties)
: createUnmodifiableMap(true, false, APISummary.super.getConfiguredProperties());
}
private ImmutableAPISummary(
ImmutableAPISummary original,
String name,
Map properties,
Map configuredProperties,
ActionsSummary actionsSummary,
String description,
List errors,
@Nullable String icon,
List warnings) {
this.name = name;
this.properties = properties;
this.configuredProperties = configuredProperties;
this.actionsSummary = actionsSummary;
this.description = description;
this.errors = errors;
this.icon = icon;
this.warnings = warnings;
}
/**
* @return The value of the {@code name} attribute
*/
@JsonProperty("name")
@JsonDeserialize(converter = StringTrimmingConverter.class)
@Override
public String getName() {
return name;
}
/**
* @return The value of the {@code properties} attribute
*/
@JsonProperty("properties")
@Override
public Map getProperties() {
return properties;
}
/**
* @return The value of the {@code configuredProperties} attribute
*/
@JsonProperty("configuredProperties")
@Override
public Map getConfiguredProperties() {
return configuredProperties;
}
/**
* @return The value of the {@code actionsSummary} attribute
*/
@JsonProperty("actionsSummary")
@Override
public ActionsSummary getActionsSummary() {
return actionsSummary;
}
/**
* @return The value of the {@code description} attribute
*/
@JsonProperty("description")
@Override
public String getDescription() {
return description;
}
/**
* @return The value of the {@code errors} attribute
*/
@JsonProperty("errors")
@Override
public List getErrors() {
return errors;
}
/**
* @return The value of the {@code icon} attribute
*/
@JsonProperty("icon")
@Override
public Optional getIcon() {
return Optional.ofNullable(icon);
}
/**
* @return The value of the {@code warnings} attribute
*/
@JsonProperty("warnings")
@Override
public List getWarnings() {
return warnings;
}
/**
* Copy the current immutable object by setting a value for the {@link APISummary#getName() name} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for name (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableAPISummary withName(String value) {
if (Objects.equals(this.name, value)) return this;
return validate(new ImmutableAPISummary(
this,
value,
this.properties,
this.configuredProperties,
this.actionsSummary,
this.description,
this.errors,
this.icon,
this.warnings));
}
/**
* Copy the current immutable object by replacing the {@link APISummary#getProperties() properties} map with the specified map.
* Nulls are not permitted as keys or values.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param entries The entries to be added to the properties map
* @return A modified copy of {@code this} object
*/
public final ImmutableAPISummary withProperties(Map entries) {
if (this.properties == entries) return this;
Map newValue = createUnmodifiableMap(true, false, entries);
return validate(new ImmutableAPISummary(
this,
this.name,
newValue,
this.configuredProperties,
this.actionsSummary,
this.description,
this.errors,
this.icon,
this.warnings));
}
/**
* Copy the current immutable object by replacing the {@link APISummary#getConfiguredProperties() configuredProperties} map with the specified map.
* Nulls are not permitted as keys or values.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param entries The entries to be added to the configuredProperties map
* @return A modified copy of {@code this} object
*/
public final ImmutableAPISummary withConfiguredProperties(Map entries) {
if (this.configuredProperties == entries) return this;
Map newValue = createUnmodifiableMap(true, false, entries);
return validate(new ImmutableAPISummary(
this,
this.name,
this.properties,
newValue,
this.actionsSummary,
this.description,
this.errors,
this.icon,
this.warnings));
}
/**
* Copy the current immutable object by setting a value for the {@link APISummary#getActionsSummary() actionsSummary} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for actionsSummary (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableAPISummary withActionsSummary(ActionsSummary value) {
if (this.actionsSummary == value) return this;
return validate(new ImmutableAPISummary(
this,
this.name,
this.properties,
this.configuredProperties,
value,
this.description,
this.errors,
this.icon,
this.warnings));
}
/**
* Copy the current immutable object by setting a value for the {@link APISummary#getDescription() description} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for description (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableAPISummary withDescription(String value) {
if (Objects.equals(this.description, value)) return this;
return validate(new ImmutableAPISummary(
this,
this.name,
this.properties,
this.configuredProperties,
this.actionsSummary,
value,
this.errors,
this.icon,
this.warnings));
}
/**
* Copy the current immutable object with elements that replace the content of {@link APISummary#getErrors() errors}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableAPISummary withErrors(Violation... elements) {
List newValue = createUnmodifiableList(false, createSafeList(Arrays.asList(elements), true, false));
return validate(new ImmutableAPISummary(
this,
this.name,
this.properties,
this.configuredProperties,
this.actionsSummary,
this.description,
newValue,
this.icon,
this.warnings));
}
/**
* Copy the current immutable object with elements that replace the content of {@link APISummary#getErrors() errors}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of errors elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableAPISummary withErrors(Iterable extends Violation> elements) {
if (this.errors == elements) return this;
List newValue = createUnmodifiableList(false, createSafeList(elements, true, false));
return validate(new ImmutableAPISummary(
this,
this.name,
this.properties,
this.configuredProperties,
this.actionsSummary,
this.description,
newValue,
this.icon,
this.warnings));
}
/**
* Copy the current immutable object by setting a present value for the optional {@link APISummary#getIcon() icon} attribute.
* @param value The value for icon
* @return A modified copy of {@code this} object
*/
public final ImmutableAPISummary withIcon(String value) {
@Nullable String newValue = Objects.requireNonNull(value, "icon");
if (Objects.equals(this.icon, newValue)) return this;
return validate(new ImmutableAPISummary(
this,
this.name,
this.properties,
this.configuredProperties,
this.actionsSummary,
this.description,
this.errors,
newValue,
this.warnings));
}
/**
* Copy the current immutable object by setting an optional value for the {@link APISummary#getIcon() icon} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for icon
* @return A modified copy of {@code this} object
*/
public final ImmutableAPISummary withIcon(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.icon, value)) return this;
return validate(new ImmutableAPISummary(
this,
this.name,
this.properties,
this.configuredProperties,
this.actionsSummary,
this.description,
this.errors,
value,
this.warnings));
}
/**
* Copy the current immutable object with elements that replace the content of {@link APISummary#getWarnings() warnings}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableAPISummary withWarnings(Violation... elements) {
List newValue = createUnmodifiableList(false, createSafeList(Arrays.asList(elements), true, false));
return validate(new ImmutableAPISummary(
this,
this.name,
this.properties,
this.configuredProperties,
this.actionsSummary,
this.description,
this.errors,
this.icon,
newValue));
}
/**
* Copy the current immutable object with elements that replace the content of {@link APISummary#getWarnings() warnings}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of warnings elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableAPISummary withWarnings(Iterable extends Violation> elements) {
if (this.warnings == elements) return this;
List newValue = createUnmodifiableList(false, createSafeList(elements, true, false));
return validate(new ImmutableAPISummary(
this,
this.name,
this.properties,
this.configuredProperties,
this.actionsSummary,
this.description,
this.errors,
this.icon,
newValue));
}
/**
* This instance is equal to all instances of {@code ImmutableAPISummary} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof ImmutableAPISummary
&& equalTo((ImmutableAPISummary) another);
}
private boolean equalTo(ImmutableAPISummary another) {
return Objects.equals(name, another.name)
&& properties.equals(another.properties)
&& configuredProperties.equals(another.configuredProperties)
&& Objects.equals(actionsSummary, another.actionsSummary)
&& Objects.equals(description, another.description)
&& errors.equals(another.errors)
&& Objects.equals(icon, another.icon)
&& warnings.equals(another.warnings);
}
/**
* Computes a hash code from attributes: {@code name}, {@code properties}, {@code configuredProperties}, {@code actionsSummary}, {@code description}, {@code errors}, {@code icon}, {@code warnings}.
* @return hashCode value
*/
@Override
public int hashCode() {
@Var int h = 5381;
h += (h << 5) + Objects.hashCode(name);
h += (h << 5) + properties.hashCode();
h += (h << 5) + configuredProperties.hashCode();
h += (h << 5) + Objects.hashCode(actionsSummary);
h += (h << 5) + Objects.hashCode(description);
h += (h << 5) + errors.hashCode();
h += (h << 5) + Objects.hashCode(icon);
h += (h << 5) + warnings.hashCode();
return h;
}
/**
* Prints the immutable value {@code APISummary} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
StringBuilder builder = new StringBuilder("APISummary{");
if (name != null) {
builder.append("name=").append(name);
}
if (builder.length() > 11) builder.append(", ");
builder.append("properties=").append(properties);
builder.append(", ");
builder.append("configuredProperties=").append(configuredProperties);
if (actionsSummary != null) {
builder.append(", ");
builder.append("actionsSummary=").append(actionsSummary);
}
if (description != null) {
builder.append(", ");
builder.append("description=").append(description);
}
builder.append(", ");
builder.append("errors=").append(errors);
if (icon != null) {
builder.append(", ");
builder.append("icon=").append(icon);
}
builder.append(", ");
builder.append("warnings=").append(warnings);
return builder.append("}").toString();
}
/**
* Construct a new immutable {@code APISummary} instance.
* @param name The value for the {@code name} attribute
* @param properties The value for the {@code properties} attribute
* @param configuredProperties The value for the {@code configuredProperties} attribute
* @param actionsSummary The value for the {@code actionsSummary} attribute
* @param description The value for the {@code description} attribute
* @param errors The value for the {@code errors} attribute
* @param icon The value for the {@code icon} attribute
* @param warnings The value for the {@code warnings} attribute
* @return An immutable APISummary instance
*/
public static APISummary of(String name, Map properties, Map configuredProperties, ActionsSummary actionsSummary, String description, List errors, Optional icon, List warnings) {
return of(name, properties, configuredProperties, actionsSummary, description, (Iterable extends Violation>) errors, icon, (Iterable extends Violation>) warnings);
}
/**
* Construct a new immutable {@code APISummary} instance.
* @param name The value for the {@code name} attribute
* @param properties The value for the {@code properties} attribute
* @param configuredProperties The value for the {@code configuredProperties} attribute
* @param actionsSummary The value for the {@code actionsSummary} attribute
* @param description The value for the {@code description} attribute
* @param errors The value for the {@code errors} attribute
* @param icon The value for the {@code icon} attribute
* @param warnings The value for the {@code warnings} attribute
* @return An immutable APISummary instance
*/
public static APISummary of(String name, Map properties, Map configuredProperties, ActionsSummary actionsSummary, String description, Iterable extends Violation> errors, Optional icon, Iterable extends Violation> warnings) {
return validate(new ImmutableAPISummary(name, properties, configuredProperties, actionsSummary, description, errors, icon, warnings));
}
private static final Validator validator = Validation.buildDefaultValidatorFactory().getValidator();
private static ImmutableAPISummary validate(ImmutableAPISummary instance) {
Set> constraintViolations = validator.validate(instance);
if (!constraintViolations.isEmpty()) {
throw new ConstraintViolationException(constraintViolations);
}
return instance;
}
/**
* Creates an immutable copy of a {@link APISummary} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable APISummary instance
*/
public static APISummary copyOf(APISummary instance) {
if (instance instanceof ImmutableAPISummary) {
return (ImmutableAPISummary) instance;
}
return new APISummary.Builder()
.createFrom(instance)
.build();
}
/**
* Builds instances of type {@link APISummary APISummary}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@Generated(from = "APISummary", generator = "Immutables")
@NotThreadSafe
public static class Builder {
private static final long OPT_BIT_CONFIGURED_PROPERTIES = 0x1L;
private long optBits;
private @Nullable String name;
private Map properties = new LinkedHashMap();
private Map configuredProperties = new LinkedHashMap();
private @Nullable ActionsSummary actionsSummary;
private @Nullable String description;
private List errors = new ArrayList();
private @Nullable String icon;
private List warnings = new ArrayList();
/**
* Creates a builder for {@link APISummary APISummary} instances.
*
* new APISummary.Builder()
* .name(String | null) // nullable {@link APISummary#getName() name}
* .putProperty|putAllProperties(String => io.syndesis.common.model.connection.ConfigurationProperty) // {@link APISummary#getProperties() properties} mappings
* .putConfiguredProperty|putAllConfiguredProperties(String => String) // {@link APISummary#getConfiguredProperties() configuredProperties} mappings
* .actionsSummary(io.syndesis.common.model.action.ActionsSummary | null) // nullable {@link APISummary#getActionsSummary() actionsSummary}
* .description(String | null) // nullable {@link APISummary#getDescription() description}
* .addError|addAllErrors(io.syndesis.common.model.Violation) // {@link APISummary#getErrors() errors} elements
* .icon(String) // optional {@link APISummary#getIcon() icon}
* .addWarning|addAllWarnings(io.syndesis.common.model.Violation) // {@link APISummary#getWarnings() warnings} elements
* .build();
*
*/
public Builder() {
if (!(this instanceof APISummary.Builder)) {
throw new UnsupportedOperationException("Use: new APISummary.Builder()");
}
}
/**
* Fill a builder with attribute values from the provided {@code io.syndesis.common.model.api.APISummary} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final APISummary.Builder createFrom(APISummary instance) {
Objects.requireNonNull(instance, "instance");
from((Object) instance);
return (APISummary.Builder) this;
}
/**
* Fill a builder with attribute values from the provided {@code io.syndesis.common.model.WithName} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final APISummary.Builder createFrom(WithName instance) {
Objects.requireNonNull(instance, "instance");
from((Object) instance);
return (APISummary.Builder) this;
}
/**
* Fill a builder with attribute values from the provided {@code io.syndesis.common.model.WithConfiguredProperties} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final APISummary.Builder createFrom(WithConfiguredProperties instance) {
Objects.requireNonNull(instance, "instance");
from((Object) instance);
return (APISummary.Builder) this;
}
/**
* Fill a builder with attribute values from the provided {@code io.syndesis.common.model.WithConfigurationProperties} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final APISummary.Builder createFrom(WithConfigurationProperties instance) {
Objects.requireNonNull(instance, "instance");
from((Object) instance);
return (APISummary.Builder) this;
}
private void from(Object object) {
if (object instanceof APISummary) {
APISummary instance = (APISummary) object;
Optional iconOptional = instance.getIcon();
if (iconOptional.isPresent()) {
icon(iconOptional);
}
String descriptionValue = instance.getDescription();
if (descriptionValue != null) {
description(descriptionValue);
}
ActionsSummary actionsSummaryValue = instance.getActionsSummary();
if (actionsSummaryValue != null) {
actionsSummary(actionsSummaryValue);
}
addAllErrors(instance.getErrors());
addAllWarnings(instance.getWarnings());
}
if (object instanceof WithName) {
WithName instance = (WithName) object;
String nameValue = instance.getName();
if (nameValue != null) {
name(nameValue);
}
}
if (object instanceof WithConfiguredProperties) {
WithConfiguredProperties instance = (WithConfiguredProperties) object;
putAllConfiguredProperties(instance.getConfiguredProperties());
}
if (object instanceof WithConfigurationProperties) {
WithConfigurationProperties instance = (WithConfigurationProperties) object;
putAllProperties(instance.getProperties());
}
}
/**
* Initializes the value for the {@link APISummary#getName() name} attribute.
* @param name The value for name (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("name")
@JsonDeserialize(converter = StringTrimmingConverter.class)
public final APISummary.Builder name(String name) {
this.name = name;
return (APISummary.Builder) this;
}
/**
* Put one entry to the {@link APISummary#getProperties() properties} map.
* @param key The key in the properties map
* @param value The associated value in the properties map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final APISummary.Builder putProperty(String key, ConfigurationProperty value) {
this.properties.put(
Objects.requireNonNull(key, "properties key"),
Objects.requireNonNull(value, "properties value"));
return (APISummary.Builder) this;
}
/**
* Put one entry to the {@link APISummary#getProperties() properties} map. Nulls are not permitted
* @param entry The key and value entry
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final APISummary.Builder putProperty(Map.Entry entry) {
String k = entry.getKey();
ConfigurationProperty v = entry.getValue();
this.properties.put(
Objects.requireNonNull(k, "properties key"),
Objects.requireNonNull(v, "properties value"));
return (APISummary.Builder) this;
}
/**
* Sets or replaces all mappings from the specified map as entries for the {@link APISummary#getProperties() properties} map. Nulls are not permitted
* @param entries The entries that will be added to the properties map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("properties")
public final APISummary.Builder properties(Map entries) {
this.properties.clear();
return putAllProperties(entries);
}
/**
* Put all mappings from the specified map as entries to {@link APISummary#getProperties() properties} map. Nulls are not permitted
* @param entries The entries that will be added to the properties map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final APISummary.Builder putAllProperties(Map entries) {
for (Map.Entry e : entries.entrySet()) {
String k = e.getKey();
ConfigurationProperty v = e.getValue();
this.properties.put(
Objects.requireNonNull(k, "properties key"),
Objects.requireNonNull(v, "properties value"));
}
return (APISummary.Builder) this;
}
/**
* Put one entry to the {@link APISummary#getConfiguredProperties() configuredProperties} map.
* @param key The key in the configuredProperties map
* @param value The associated value in the configuredProperties map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final APISummary.Builder putConfiguredProperty(String key, String value) {
this.configuredProperties.put(
Objects.requireNonNull(key, "configuredProperties key"),
Objects.requireNonNull(value, "configuredProperties value"));
optBits |= OPT_BIT_CONFIGURED_PROPERTIES;
return (APISummary.Builder) this;
}
/**
* Put one entry to the {@link APISummary#getConfiguredProperties() configuredProperties} map. Nulls are not permitted
* @param entry The key and value entry
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final APISummary.Builder putConfiguredProperty(Map.Entry entry) {
String k = entry.getKey();
String v = entry.getValue();
this.configuredProperties.put(
Objects.requireNonNull(k, "configuredProperties key"),
Objects.requireNonNull(v, "configuredProperties value"));
optBits |= OPT_BIT_CONFIGURED_PROPERTIES;
return (APISummary.Builder) this;
}
/**
* Sets or replaces all mappings from the specified map as entries for the {@link APISummary#getConfiguredProperties() configuredProperties} map. Nulls are not permitted
* @param entries The entries that will be added to the configuredProperties map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("configuredProperties")
public final APISummary.Builder configuredProperties(Map entries) {
this.configuredProperties.clear();
optBits |= OPT_BIT_CONFIGURED_PROPERTIES;
return putAllConfiguredProperties(entries);
}
/**
* Put all mappings from the specified map as entries to {@link APISummary#getConfiguredProperties() configuredProperties} map. Nulls are not permitted
* @param entries The entries that will be added to the configuredProperties map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final APISummary.Builder putAllConfiguredProperties(Map entries) {
for (Map.Entry e : entries.entrySet()) {
String k = e.getKey();
String v = e.getValue();
this.configuredProperties.put(
Objects.requireNonNull(k, "configuredProperties key"),
Objects.requireNonNull(v, "configuredProperties value"));
}
optBits |= OPT_BIT_CONFIGURED_PROPERTIES;
return (APISummary.Builder) this;
}
/**
* Initializes the value for the {@link APISummary#getActionsSummary() actionsSummary} attribute.
* @param actionsSummary The value for actionsSummary (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("actionsSummary")
public final APISummary.Builder actionsSummary(ActionsSummary actionsSummary) {
this.actionsSummary = actionsSummary;
return (APISummary.Builder) this;
}
/**
* Initializes the value for the {@link APISummary#getDescription() description} attribute.
* @param description The value for description (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("description")
public final APISummary.Builder description(String description) {
this.description = description;
return (APISummary.Builder) this;
}
/**
* Adds one element to {@link APISummary#getErrors() errors} list.
* @param element A errors element
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final APISummary.Builder addError(Violation element) {
this.errors.add(Objects.requireNonNull(element, "errors element"));
return (APISummary.Builder) this;
}
/**
* Adds elements to {@link APISummary#getErrors() errors} list.
* @param elements An array of errors elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final APISummary.Builder addErrors(Violation... elements) {
for (Violation element : elements) {
this.errors.add(Objects.requireNonNull(element, "errors element"));
}
return (APISummary.Builder) this;
}
/**
* Sets or replaces all elements for {@link APISummary#getErrors() errors} list.
* @param elements An iterable of errors elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("errors")
public final APISummary.Builder errors(Iterable extends Violation> elements) {
this.errors.clear();
return addAllErrors(elements);
}
/**
* Adds elements to {@link APISummary#getErrors() errors} list.
* @param elements An iterable of errors elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final APISummary.Builder addAllErrors(Iterable extends Violation> elements) {
for (Violation element : elements) {
this.errors.add(Objects.requireNonNull(element, "errors element"));
}
return (APISummary.Builder) this;
}
/**
* Initializes the optional value {@link APISummary#getIcon() icon} to icon.
* @param icon The value for icon
* @return {@code this} builder for chained invocation
*/
@CanIgnoreReturnValue
public final APISummary.Builder icon(String icon) {
this.icon = Objects.requireNonNull(icon, "icon");
return (APISummary.Builder) this;
}
/**
* Initializes the optional value {@link APISummary#getIcon() icon} to icon.
* @param icon The value for icon
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("icon")
public final APISummary.Builder icon(Optional icon) {
this.icon = icon.orElse(null);
return (APISummary.Builder) this;
}
/**
* Adds one element to {@link APISummary#getWarnings() warnings} list.
* @param element A warnings element
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final APISummary.Builder addWarning(Violation element) {
this.warnings.add(Objects.requireNonNull(element, "warnings element"));
return (APISummary.Builder) this;
}
/**
* Adds elements to {@link APISummary#getWarnings() warnings} list.
* @param elements An array of warnings elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final APISummary.Builder addWarnings(Violation... elements) {
for (Violation element : elements) {
this.warnings.add(Objects.requireNonNull(element, "warnings element"));
}
return (APISummary.Builder) this;
}
/**
* Sets or replaces all elements for {@link APISummary#getWarnings() warnings} list.
* @param elements An iterable of warnings elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("warnings")
public final APISummary.Builder warnings(Iterable extends Violation> elements) {
this.warnings.clear();
return addAllWarnings(elements);
}
/**
* Adds elements to {@link APISummary#getWarnings() warnings} list.
* @param elements An iterable of warnings elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final APISummary.Builder addAllWarnings(Iterable extends Violation> elements) {
for (Violation element : elements) {
this.warnings.add(Objects.requireNonNull(element, "warnings element"));
}
return (APISummary.Builder) this;
}
/**
* Builds a new {@link APISummary APISummary}.
* @return An immutable instance of APISummary
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public APISummary build() {
return ImmutableAPISummary.validate(new ImmutableAPISummary(this));
}
private boolean configuredPropertiesIsSet() {
return (optBits & OPT_BIT_CONFIGURED_PROPERTIES) != 0;
}
}
private static List createSafeList(Iterable extends T> iterable, boolean checkNulls, boolean skipNulls) {
ArrayList list;
if (iterable instanceof Collection>) {
int size = ((Collection>) iterable).size();
if (size == 0) return Collections.emptyList();
list = new ArrayList<>();
} else {
list = new ArrayList<>();
}
for (T element : iterable) {
if (skipNulls && element == null) continue;
if (checkNulls) Objects.requireNonNull(element, "element");
list.add(element);
}
return list;
}
private static List createUnmodifiableList(boolean clone, List list) {
switch(list.size()) {
case 0: return Collections.emptyList();
case 1: return Collections.singletonList(list.get(0));
default:
if (clone) {
return Collections.unmodifiableList(new ArrayList<>(list));
} else {
if (list instanceof ArrayList>) {
((ArrayList>) list).trimToSize();
}
return Collections.unmodifiableList(list);
}
}
}
private static Map createUnmodifiableMap(boolean checkNulls, boolean skipNulls, Map extends K, ? extends V> map) {
switch (map.size()) {
case 0: return Collections.emptyMap();
case 1: {
Map.Entry extends K, ? extends V> e = map.entrySet().iterator().next();
K k = e.getKey();
V v = e.getValue();
if (checkNulls) {
Objects.requireNonNull(k, "key");
Objects.requireNonNull(v, "value");
}
if (skipNulls && (k == null || v == null)) {
return Collections.emptyMap();
}
return Collections.singletonMap(k, v);
}
default: {
Map linkedMap = new LinkedHashMap<>(map.size());
if (skipNulls || checkNulls) {
for (Map.Entry extends K, ? extends V> e : map.entrySet()) {
K k = e.getKey();
V v = e.getValue();
if (skipNulls) {
if (k == null || v == null) continue;
} else if (checkNulls) {
Objects.requireNonNull(k, "key");
Objects.requireNonNull(v, "value");
}
linkedMap.put(k, v);
}
} else {
linkedMap.putAll(map);
}
return Collections.unmodifiableMap(linkedMap);
}
}
}
}