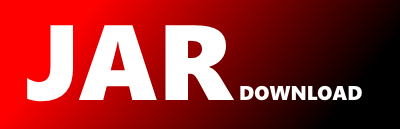
io.syndesis.common.model.integration.ImmutableIntegrationOverview Maven / Gradle / Ivy
/*
* Copyright (C) 2016 Red Hat, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.syndesis.common.model.integration;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.google.errorprone.annotations.CanIgnoreReturnValue;
import com.google.errorprone.annotations.Var;
import edu.umd.cs.findbugs.annotations.SuppressFBWarnings;
import io.syndesis.common.model.ResourceIdentifier;
import io.syndesis.common.model.WithConfigurationProperties;
import io.syndesis.common.model.WithConfiguredProperties;
import io.syndesis.common.model.WithModificationTimestamps;
import io.syndesis.common.model.WithName;
import io.syndesis.common.model.WithResourceId;
import io.syndesis.common.model.WithResources;
import io.syndesis.common.model.WithTags;
import io.syndesis.common.model.WithVersion;
import io.syndesis.common.model.bulletin.IntegrationBulletinBoard;
import io.syndesis.common.model.connection.ConfigurationProperty;
import io.syndesis.common.model.connection.Connection;
import io.syndesis.common.util.json.OptionalStringTrimmingConverter;
import io.syndesis.common.util.json.StringTrimmingConverter;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import java.util.NavigableSet;
import java.util.Objects;
import java.util.Optional;
import java.util.Set;
import java.util.SortedSet;
import java.util.TreeSet;
import javax.annotation.CheckReturnValue;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import javax.annotation.concurrent.NotThreadSafe;
import javax.validation.ConstraintViolation;
import javax.validation.ConstraintViolationException;
import javax.validation.Validation;
import javax.validation.Validator;
import org.immutables.value.Generated;
/**
* Immutable implementation of {@link IntegrationOverview}.
*
* Use the builder to create immutable instances:
* {@code new IntegrationOverview.Builder()}.
* Use the static factory method to create immutable instances:
* {@code ImmutableIntegrationOverview.of()}.
*/
@Generated(from = "IntegrationOverview", generator = "Immutables")
@SuppressWarnings({"all", "deprecation"})
@SuppressFBWarnings
@ParametersAreNonnullByDefault
@javax.annotation.Generated("org.immutables.processor.ProxyProcessor")
@Immutable
@CheckReturnValue
final class ImmutableIntegrationOverview
implements IntegrationOverview {
private final @Nullable String id;
private final Map properties;
private final Map configuredProperties;
private final int version;
private final long createdAt;
private final long updatedAt;
private final SortedSet tags;
private final String name;
private final List resources;
private final boolean isDeleted;
private final List steps;
private final List flows;
private final List connections;
private final @Nullable String description;
private final Map continuousDeliveryState;
private final boolean isDraft;
private final IntegrationDeploymentState currentState;
private final IntegrationDeploymentState targetState;
private final @Nullable Integer deploymentVersion;
private final List deployments;
private final IntegrationBulletinBoard board;
private final @Nullable String url;
private ImmutableIntegrationOverview(
Optional id,
Map properties,
Map configuredProperties,
int version,
long createdAt,
long updatedAt,
Iterable tags,
String name,
Iterable extends ResourceIdentifier> resources,
boolean isDeleted,
Iterable extends Step> steps,
Iterable extends Flow> flows,
Iterable extends Connection> connections,
Optional description,
Map continuousDeliveryState,
boolean isDraft,
IntegrationDeploymentState currentState,
IntegrationDeploymentState targetState,
Optional deploymentVersion,
Iterable extends IntegrationDeploymentOverview> deployments,
IntegrationBulletinBoard board,
Optional url) {
this.id = id.orElse(null);
this.properties = createUnmodifiableMap(true, false, properties);
this.configuredProperties = createUnmodifiableMap(true, false, configuredProperties);
this.version = version;
this.createdAt = createdAt;
this.updatedAt = updatedAt;
this.tags = createUnmodifiableSortedSet(false, createSafeList(tags, false, true));
this.name = name;
this.resources = createUnmodifiableList(false, createSafeList(resources, true, false));
this.isDeleted = isDeleted;
this.steps = createUnmodifiableList(false, createSafeList(steps, true, false));
this.flows = createUnmodifiableList(false, createSafeList(flows, true, false));
this.connections = createUnmodifiableList(false, createSafeList(connections, true, false));
this.description = description.orElse(null);
this.continuousDeliveryState = createUnmodifiableMap(true, false, continuousDeliveryState);
this.isDraft = isDraft;
this.currentState = Objects.requireNonNull(currentState, "currentState");
this.targetState = Objects.requireNonNull(targetState, "targetState");
this.deploymentVersion = deploymentVersion.orElse(null);
this.deployments = createUnmodifiableList(false, createSafeList(deployments, true, false));
this.board = Objects.requireNonNull(board, "board");
this.url = url.orElse(null);
this.initShim = null;
}
private ImmutableIntegrationOverview(ImmutableIntegrationOverview.Builder builder) {
this.id = builder.id;
this.properties = createUnmodifiableMap(false, false, builder.properties);
this.tags = createUnmodifiableSortedSet(false, createSafeList(builder.tags, false, false));
this.name = builder.name;
this.description = builder.description;
this.isDraft = builder.isDraft;
this.deploymentVersion = builder.deploymentVersion;
this.url = builder.url;
if (builder.configuredPropertiesIsSet()) {
initShim.configuredProperties(createUnmodifiableMap(false, false, builder.configuredProperties));
}
if (builder.versionIsSet()) {
initShim.version(builder.version);
}
if (builder.createdAtIsSet()) {
initShim.createdAt(builder.createdAt);
}
if (builder.updatedAtIsSet()) {
initShim.updatedAt(builder.updatedAt);
}
if (builder.resourcesIsSet()) {
initShim.resources(createUnmodifiableList(true, builder.resources));
}
if (builder.isDeletedIsSet()) {
initShim.isDeleted(builder.isDeleted);
}
if (builder.stepsIsSet()) {
initShim.steps(createUnmodifiableList(true, builder.steps));
}
if (builder.flowsIsSet()) {
initShim.flows(createUnmodifiableList(true, builder.flows));
}
if (builder.connectionsIsSet()) {
initShim.connections(createUnmodifiableList(true, builder.connections));
}
if (builder.continuousDeliveryStateIsSet()) {
initShim.continuousDeliveryState(createUnmodifiableMap(false, false, builder.continuousDeliveryState));
}
if (builder.currentState != null) {
initShim.currentState(builder.currentState);
}
if (builder.targetState != null) {
initShim.targetState(builder.targetState);
}
if (builder.deploymentsIsSet()) {
initShim.deployments(createUnmodifiableList(true, builder.deployments));
}
if (builder.board != null) {
initShim.board(builder.board);
}
this.configuredProperties = initShim.getConfiguredProperties();
this.version = initShim.getVersion();
this.createdAt = initShim.getCreatedAt();
this.updatedAt = initShim.getUpdatedAt();
this.resources = initShim.getResources();
this.isDeleted = initShim.isDeleted();
this.steps = initShim.getSteps();
this.flows = initShim.getFlows();
this.connections = initShim.getConnections();
this.continuousDeliveryState = initShim.getContinuousDeliveryState();
this.currentState = initShim.getCurrentState();
this.targetState = initShim.getTargetState();
this.deployments = initShim.getDeployments();
this.board = initShim.getBoard();
this.initShim = null;
}
private ImmutableIntegrationOverview(
ImmutableIntegrationOverview original,
@Nullable String id,
Map properties,
Map configuredProperties,
int version,
long createdAt,
long updatedAt,
SortedSet tags,
String name,
List resources,
boolean isDeleted,
List steps,
List flows,
List connections,
@Nullable String description,
Map continuousDeliveryState,
boolean isDraft,
IntegrationDeploymentState currentState,
IntegrationDeploymentState targetState,
@Nullable Integer deploymentVersion,
List deployments,
IntegrationBulletinBoard board,
@Nullable String url) {
this.id = id;
this.properties = properties;
this.configuredProperties = configuredProperties;
this.version = version;
this.createdAt = createdAt;
this.updatedAt = updatedAt;
this.tags = tags;
this.name = name;
this.resources = resources;
this.isDeleted = isDeleted;
this.steps = steps;
this.flows = flows;
this.connections = connections;
this.description = description;
this.continuousDeliveryState = continuousDeliveryState;
this.isDraft = isDraft;
this.currentState = currentState;
this.targetState = targetState;
this.deploymentVersion = deploymentVersion;
this.deployments = deployments;
this.board = board;
this.url = url;
this.initShim = null;
}
private static final byte STAGE_INITIALIZING = -1;
private static final byte STAGE_UNINITIALIZED = 0;
private static final byte STAGE_INITIALIZED = 1;
@SuppressWarnings("Immutable")
private transient volatile InitShim initShim = new InitShim();
@Generated(from = "IntegrationOverview", generator = "Immutables")
private final class InitShim {
private byte configuredPropertiesBuildStage = STAGE_UNINITIALIZED;
private Map configuredProperties;
Map getConfiguredProperties() {
if (configuredPropertiesBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (configuredPropertiesBuildStage == STAGE_UNINITIALIZED) {
configuredPropertiesBuildStage = STAGE_INITIALIZING;
this.configuredProperties = createUnmodifiableMap(true, false, getConfiguredPropertiesInitialize());
configuredPropertiesBuildStage = STAGE_INITIALIZED;
}
return this.configuredProperties;
}
void configuredProperties(Map configuredProperties) {
this.configuredProperties = configuredProperties;
configuredPropertiesBuildStage = STAGE_INITIALIZED;
}
private byte versionBuildStage = STAGE_UNINITIALIZED;
private int version;
int getVersion() {
if (versionBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (versionBuildStage == STAGE_UNINITIALIZED) {
versionBuildStage = STAGE_INITIALIZING;
this.version = getVersionInitialize();
versionBuildStage = STAGE_INITIALIZED;
}
return this.version;
}
void version(int version) {
this.version = version;
versionBuildStage = STAGE_INITIALIZED;
}
private byte createdAtBuildStage = STAGE_UNINITIALIZED;
private long createdAt;
long getCreatedAt() {
if (createdAtBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (createdAtBuildStage == STAGE_UNINITIALIZED) {
createdAtBuildStage = STAGE_INITIALIZING;
this.createdAt = getCreatedAtInitialize();
createdAtBuildStage = STAGE_INITIALIZED;
}
return this.createdAt;
}
void createdAt(long createdAt) {
this.createdAt = createdAt;
createdAtBuildStage = STAGE_INITIALIZED;
}
private byte updatedAtBuildStage = STAGE_UNINITIALIZED;
private long updatedAt;
long getUpdatedAt() {
if (updatedAtBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (updatedAtBuildStage == STAGE_UNINITIALIZED) {
updatedAtBuildStage = STAGE_INITIALIZING;
this.updatedAt = getUpdatedAtInitialize();
updatedAtBuildStage = STAGE_INITIALIZED;
}
return this.updatedAt;
}
void updatedAt(long updatedAt) {
this.updatedAt = updatedAt;
updatedAtBuildStage = STAGE_INITIALIZED;
}
private byte resourcesBuildStage = STAGE_UNINITIALIZED;
private List resources;
List getResources() {
if (resourcesBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (resourcesBuildStage == STAGE_UNINITIALIZED) {
resourcesBuildStage = STAGE_INITIALIZING;
this.resources = createUnmodifiableList(false, createSafeList(getResourcesInitialize(), true, false));
resourcesBuildStage = STAGE_INITIALIZED;
}
return this.resources;
}
void resources(List resources) {
this.resources = resources;
resourcesBuildStage = STAGE_INITIALIZED;
}
private byte isDeletedBuildStage = STAGE_UNINITIALIZED;
private boolean isDeleted;
boolean isDeleted() {
if (isDeletedBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (isDeletedBuildStage == STAGE_UNINITIALIZED) {
isDeletedBuildStage = STAGE_INITIALIZING;
this.isDeleted = isDeletedInitialize();
isDeletedBuildStage = STAGE_INITIALIZED;
}
return this.isDeleted;
}
void isDeleted(boolean isDeleted) {
this.isDeleted = isDeleted;
isDeletedBuildStage = STAGE_INITIALIZED;
}
private byte stepsBuildStage = STAGE_UNINITIALIZED;
private List steps;
List getSteps() {
if (stepsBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (stepsBuildStage == STAGE_UNINITIALIZED) {
stepsBuildStage = STAGE_INITIALIZING;
this.steps = createUnmodifiableList(false, createSafeList(getStepsInitialize(), true, false));
stepsBuildStage = STAGE_INITIALIZED;
}
return this.steps;
}
void steps(List steps) {
this.steps = steps;
stepsBuildStage = STAGE_INITIALIZED;
}
private byte flowsBuildStage = STAGE_UNINITIALIZED;
private List flows;
List getFlows() {
if (flowsBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (flowsBuildStage == STAGE_UNINITIALIZED) {
flowsBuildStage = STAGE_INITIALIZING;
this.flows = createUnmodifiableList(false, createSafeList(getFlowsInitialize(), true, false));
flowsBuildStage = STAGE_INITIALIZED;
}
return this.flows;
}
void flows(List flows) {
this.flows = flows;
flowsBuildStage = STAGE_INITIALIZED;
}
private byte connectionsBuildStage = STAGE_UNINITIALIZED;
private List connections;
List getConnections() {
if (connectionsBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (connectionsBuildStage == STAGE_UNINITIALIZED) {
connectionsBuildStage = STAGE_INITIALIZING;
this.connections = createUnmodifiableList(false, createSafeList(getConnectionsInitialize(), true, false));
connectionsBuildStage = STAGE_INITIALIZED;
}
return this.connections;
}
void connections(List connections) {
this.connections = connections;
connectionsBuildStage = STAGE_INITIALIZED;
}
private byte continuousDeliveryStateBuildStage = STAGE_UNINITIALIZED;
private Map continuousDeliveryState;
Map getContinuousDeliveryState() {
if (continuousDeliveryStateBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (continuousDeliveryStateBuildStage == STAGE_UNINITIALIZED) {
continuousDeliveryStateBuildStage = STAGE_INITIALIZING;
this.continuousDeliveryState = createUnmodifiableMap(true, false, getContinuousDeliveryStateInitialize());
continuousDeliveryStateBuildStage = STAGE_INITIALIZED;
}
return this.continuousDeliveryState;
}
void continuousDeliveryState(Map continuousDeliveryState) {
this.continuousDeliveryState = continuousDeliveryState;
continuousDeliveryStateBuildStage = STAGE_INITIALIZED;
}
private byte currentStateBuildStage = STAGE_UNINITIALIZED;
private IntegrationDeploymentState currentState;
IntegrationDeploymentState getCurrentState() {
if (currentStateBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (currentStateBuildStage == STAGE_UNINITIALIZED) {
currentStateBuildStage = STAGE_INITIALIZING;
this.currentState = Objects.requireNonNull(getCurrentStateInitialize(), "currentState");
currentStateBuildStage = STAGE_INITIALIZED;
}
return this.currentState;
}
void currentState(IntegrationDeploymentState currentState) {
this.currentState = currentState;
currentStateBuildStage = STAGE_INITIALIZED;
}
private byte targetStateBuildStage = STAGE_UNINITIALIZED;
private IntegrationDeploymentState targetState;
IntegrationDeploymentState getTargetState() {
if (targetStateBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (targetStateBuildStage == STAGE_UNINITIALIZED) {
targetStateBuildStage = STAGE_INITIALIZING;
this.targetState = Objects.requireNonNull(getTargetStateInitialize(), "targetState");
targetStateBuildStage = STAGE_INITIALIZED;
}
return this.targetState;
}
void targetState(IntegrationDeploymentState targetState) {
this.targetState = targetState;
targetStateBuildStage = STAGE_INITIALIZED;
}
private byte deploymentsBuildStage = STAGE_UNINITIALIZED;
private List deployments;
List getDeployments() {
if (deploymentsBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (deploymentsBuildStage == STAGE_UNINITIALIZED) {
deploymentsBuildStage = STAGE_INITIALIZING;
this.deployments = createUnmodifiableList(false, createSafeList(getDeploymentsInitialize(), true, false));
deploymentsBuildStage = STAGE_INITIALIZED;
}
return this.deployments;
}
void deployments(List deployments) {
this.deployments = deployments;
deploymentsBuildStage = STAGE_INITIALIZED;
}
private byte boardBuildStage = STAGE_UNINITIALIZED;
private IntegrationBulletinBoard board;
IntegrationBulletinBoard getBoard() {
if (boardBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (boardBuildStage == STAGE_UNINITIALIZED) {
boardBuildStage = STAGE_INITIALIZING;
this.board = Objects.requireNonNull(getBoardInitialize(), "board");
boardBuildStage = STAGE_INITIALIZED;
}
return this.board;
}
void board(IntegrationBulletinBoard board) {
this.board = board;
boardBuildStage = STAGE_INITIALIZED;
}
private String formatInitCycleMessage() {
List attributes = new ArrayList<>();
if (configuredPropertiesBuildStage == STAGE_INITIALIZING) attributes.add("configuredProperties");
if (versionBuildStage == STAGE_INITIALIZING) attributes.add("version");
if (createdAtBuildStage == STAGE_INITIALIZING) attributes.add("createdAt");
if (updatedAtBuildStage == STAGE_INITIALIZING) attributes.add("updatedAt");
if (resourcesBuildStage == STAGE_INITIALIZING) attributes.add("resources");
if (isDeletedBuildStage == STAGE_INITIALIZING) attributes.add("isDeleted");
if (stepsBuildStage == STAGE_INITIALIZING) attributes.add("steps");
if (flowsBuildStage == STAGE_INITIALIZING) attributes.add("flows");
if (connectionsBuildStage == STAGE_INITIALIZING) attributes.add("connections");
if (continuousDeliveryStateBuildStage == STAGE_INITIALIZING) attributes.add("continuousDeliveryState");
if (currentStateBuildStage == STAGE_INITIALIZING) attributes.add("currentState");
if (targetStateBuildStage == STAGE_INITIALIZING) attributes.add("targetState");
if (deploymentsBuildStage == STAGE_INITIALIZING) attributes.add("deployments");
if (boardBuildStage == STAGE_INITIALIZING) attributes.add("board");
return "Cannot build IntegrationOverview, attribute initializers form cycle " + attributes;
}
}
private Map getConfiguredPropertiesInitialize() {
return IntegrationOverview.super.getConfiguredProperties();
}
private int getVersionInitialize() {
return IntegrationOverview.super.getVersion();
}
private long getCreatedAtInitialize() {
return IntegrationOverview.super.getCreatedAt();
}
private long getUpdatedAtInitialize() {
return IntegrationOverview.super.getUpdatedAt();
}
private List getResourcesInitialize() {
return IntegrationOverview.super.getResources();
}
private boolean isDeletedInitialize() {
return IntegrationOverview.super.isDeleted();
}
private List getStepsInitialize() {
return IntegrationOverview.super.getSteps();
}
private List getFlowsInitialize() {
return IntegrationOverview.super.getFlows();
}
private List getConnectionsInitialize() {
return IntegrationOverview.super.getConnections();
}
private Map getContinuousDeliveryStateInitialize() {
return IntegrationOverview.super.getContinuousDeliveryState();
}
private IntegrationDeploymentState getCurrentStateInitialize() {
return IntegrationOverview.super.getCurrentState();
}
private IntegrationDeploymentState getTargetStateInitialize() {
return IntegrationOverview.super.getTargetState();
}
private List getDeploymentsInitialize() {
return IntegrationOverview.super.getDeployments();
}
private IntegrationBulletinBoard getBoardInitialize() {
return IntegrationOverview.super.getBoard();
}
/**
* @return The value of the {@code id} attribute
*/
@JsonProperty("id")
@Override
public Optional getId() {
return Optional.ofNullable(id);
}
/**
* @return The value of the {@code properties} attribute
*/
@JsonProperty("properties")
@Override
public Map getProperties() {
return properties;
}
/**
* @return The value of the {@code configuredProperties} attribute
*/
@JsonProperty("configuredProperties")
@Override
public Map getConfiguredProperties() {
InitShim shim = this.initShim;
return shim != null
? shim.getConfiguredProperties()
: this.configuredProperties;
}
/**
* @return The value of the {@code version} attribute
*/
@JsonProperty("version")
@Override
public int getVersion() {
InitShim shim = this.initShim;
return shim != null
? shim.getVersion()
: this.version;
}
/**
* @return The value of the {@code createdAt} attribute
*/
@JsonProperty("createdAt")
@Override
public long getCreatedAt() {
InitShim shim = this.initShim;
return shim != null
? shim.getCreatedAt()
: this.createdAt;
}
/**
* @return The value of the {@code updatedAt} attribute
*/
@JsonProperty("updatedAt")
@Override
public long getUpdatedAt() {
InitShim shim = this.initShim;
return shim != null
? shim.getUpdatedAt()
: this.updatedAt;
}
/**
* @return The value of the {@code tags} attribute
*/
@JsonProperty("tags")
@JsonDeserialize(contentConverter = StringTrimmingConverter.class)
@Override
public SortedSet getTags() {
return tags;
}
/**
* @return The value of the {@code name} attribute
*/
@JsonProperty("name")
@JsonDeserialize(converter = StringTrimmingConverter.class)
@Override
public String getName() {
return name;
}
/**
* @return The value of the {@code resources} attribute
*/
@JsonProperty("resources")
@Override
public List getResources() {
InitShim shim = this.initShim;
return shim != null
? shim.getResources()
: this.resources;
}
/**
* @return The value of the {@code isDeleted} attribute
*/
@JsonProperty("isDeleted")
@JsonInclude(JsonInclude.Include.NON_DEFAULT)
@Override
public boolean isDeleted() {
InitShim shim = this.initShim;
return shim != null
? shim.isDeleted()
: this.isDeleted;
}
/**
* @return The value of the {@code steps} attribute
*/
@JsonProperty("steps")
@Deprecated
@Override
public List getSteps() {
InitShim shim = this.initShim;
return shim != null
? shim.getSteps()
: this.steps;
}
/**
* @return The value of the {@code flows} attribute
*/
@JsonProperty("flows")
@Override
public List getFlows() {
InitShim shim = this.initShim;
return shim != null
? shim.getFlows()
: this.flows;
}
/**
* @return The value of the {@code connections} attribute
*/
@JsonProperty("connections")
@Override
public List getConnections() {
InitShim shim = this.initShim;
return shim != null
? shim.getConnections()
: this.connections;
}
/**
* @return The value of the {@code description} attribute
*/
@JsonProperty("description")
@JsonDeserialize(converter = OptionalStringTrimmingConverter.class)
@Override
public Optional getDescription() {
return Optional.ofNullable(description);
}
/**
* @return The value of the {@code continuousDeliveryState} attribute
*/
@JsonProperty("continuousDeliveryState")
@Override
public Map getContinuousDeliveryState() {
InitShim shim = this.initShim;
return shim != null
? shim.getContinuousDeliveryState()
: this.continuousDeliveryState;
}
/**
* @return The value of the {@code isDraft} attribute
*/
@JsonProperty("isDraft")
@Override
public boolean isDraft() {
return isDraft;
}
/**
* @return The value of the {@code currentState} attribute
*/
@JsonProperty("currentState")
@Override
public IntegrationDeploymentState getCurrentState() {
InitShim shim = this.initShim;
return shim != null
? shim.getCurrentState()
: this.currentState;
}
/**
* @return The value of the {@code targetState} attribute
*/
@JsonProperty("targetState")
@Override
public IntegrationDeploymentState getTargetState() {
InitShim shim = this.initShim;
return shim != null
? shim.getTargetState()
: this.targetState;
}
/**
* @return The value of the {@code deploymentVersion} attribute
*/
@JsonProperty("deploymentVersion")
@Override
public Optional getDeploymentVersion() {
return Optional.ofNullable(deploymentVersion);
}
/**
* @return The value of the {@code deployments} attribute
*/
@JsonProperty("deployments")
@Override
public List getDeployments() {
InitShim shim = this.initShim;
return shim != null
? shim.getDeployments()
: this.deployments;
}
/**
* @return The value of the {@code board} attribute
*/
@JsonProperty("board")
@Override
public IntegrationBulletinBoard getBoard() {
InitShim shim = this.initShim;
return shim != null
? shim.getBoard()
: this.board;
}
/**
* @return The value of the {@code url} attribute
*/
@JsonProperty("url")
@Override
public Optional getUrl() {
return Optional.ofNullable(url);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link IntegrationOverview#getId() id} attribute.
* @param value The value for id
* @return A modified copy of {@code this} object
*/
public final ImmutableIntegrationOverview withId(String value) {
@Nullable String newValue = Objects.requireNonNull(value, "id");
if (Objects.equals(this.id, newValue)) return this;
return validate(new ImmutableIntegrationOverview(
this,
newValue,
this.properties,
this.configuredProperties,
this.version,
this.createdAt,
this.updatedAt,
this.tags,
this.name,
this.resources,
this.isDeleted,
this.steps,
this.flows,
this.connections,
this.description,
this.continuousDeliveryState,
this.isDraft,
this.currentState,
this.targetState,
this.deploymentVersion,
this.deployments,
this.board,
this.url));
}
/**
* Copy the current immutable object by setting an optional value for the {@link IntegrationOverview#getId() id} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for id
* @return A modified copy of {@code this} object
*/
public final ImmutableIntegrationOverview withId(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.id, value)) return this;
return validate(new ImmutableIntegrationOverview(
this,
value,
this.properties,
this.configuredProperties,
this.version,
this.createdAt,
this.updatedAt,
this.tags,
this.name,
this.resources,
this.isDeleted,
this.steps,
this.flows,
this.connections,
this.description,
this.continuousDeliveryState,
this.isDraft,
this.currentState,
this.targetState,
this.deploymentVersion,
this.deployments,
this.board,
this.url));
}
/**
* Copy the current immutable object by replacing the {@link IntegrationOverview#getProperties() properties} map with the specified map.
* Nulls are not permitted as keys or values.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param entries The entries to be added to the properties map
* @return A modified copy of {@code this} object
*/
public final ImmutableIntegrationOverview withProperties(Map entries) {
if (this.properties == entries) return this;
Map newValue = createUnmodifiableMap(true, false, entries);
return validate(new ImmutableIntegrationOverview(
this,
this.id,
newValue,
this.configuredProperties,
this.version,
this.createdAt,
this.updatedAt,
this.tags,
this.name,
this.resources,
this.isDeleted,
this.steps,
this.flows,
this.connections,
this.description,
this.continuousDeliveryState,
this.isDraft,
this.currentState,
this.targetState,
this.deploymentVersion,
this.deployments,
this.board,
this.url));
}
/**
* Copy the current immutable object by replacing the {@link IntegrationOverview#getConfiguredProperties() configuredProperties} map with the specified map.
* Nulls are not permitted as keys or values.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param entries The entries to be added to the configuredProperties map
* @return A modified copy of {@code this} object
*/
public final ImmutableIntegrationOverview withConfiguredProperties(Map entries) {
if (this.configuredProperties == entries) return this;
Map newValue = createUnmodifiableMap(true, false, entries);
return validate(new ImmutableIntegrationOverview(
this,
this.id,
this.properties,
newValue,
this.version,
this.createdAt,
this.updatedAt,
this.tags,
this.name,
this.resources,
this.isDeleted,
this.steps,
this.flows,
this.connections,
this.description,
this.continuousDeliveryState,
this.isDraft,
this.currentState,
this.targetState,
this.deploymentVersion,
this.deployments,
this.board,
this.url));
}
/**
* Copy the current immutable object by setting a value for the {@link IntegrationOverview#getVersion() version} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for version
* @return A modified copy of the {@code this} object
*/
public final ImmutableIntegrationOverview withVersion(int value) {
if (this.version == value) return this;
return validate(new ImmutableIntegrationOverview(
this,
this.id,
this.properties,
this.configuredProperties,
value,
this.createdAt,
this.updatedAt,
this.tags,
this.name,
this.resources,
this.isDeleted,
this.steps,
this.flows,
this.connections,
this.description,
this.continuousDeliveryState,
this.isDraft,
this.currentState,
this.targetState,
this.deploymentVersion,
this.deployments,
this.board,
this.url));
}
/**
* Copy the current immutable object by setting a value for the {@link IntegrationOverview#getCreatedAt() createdAt} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for createdAt
* @return A modified copy of the {@code this} object
*/
public final ImmutableIntegrationOverview withCreatedAt(long value) {
if (this.createdAt == value) return this;
return validate(new ImmutableIntegrationOverview(
this,
this.id,
this.properties,
this.configuredProperties,
this.version,
value,
this.updatedAt,
this.tags,
this.name,
this.resources,
this.isDeleted,
this.steps,
this.flows,
this.connections,
this.description,
this.continuousDeliveryState,
this.isDraft,
this.currentState,
this.targetState,
this.deploymentVersion,
this.deployments,
this.board,
this.url));
}
/**
* Copy the current immutable object by setting a value for the {@link IntegrationOverview#getUpdatedAt() updatedAt} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for updatedAt
* @return A modified copy of the {@code this} object
*/
public final ImmutableIntegrationOverview withUpdatedAt(long value) {
if (this.updatedAt == value) return this;
return validate(new ImmutableIntegrationOverview(
this,
this.id,
this.properties,
this.configuredProperties,
this.version,
this.createdAt,
value,
this.tags,
this.name,
this.resources,
this.isDeleted,
this.steps,
this.flows,
this.connections,
this.description,
this.continuousDeliveryState,
this.isDraft,
this.currentState,
this.targetState,
this.deploymentVersion,
this.deployments,
this.board,
this.url));
}
/**
* Copy the current immutable object with elements that replace the content of {@link IntegrationOverview#getTags() tags}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableIntegrationOverview withTags(String... elements) {
SortedSet newValue = createUnmodifiableSortedSet(false, createSafeList(Arrays.asList(elements), false, true));
return validate(new ImmutableIntegrationOverview(
this,
this.id,
this.properties,
this.configuredProperties,
this.version,
this.createdAt,
this.updatedAt,
newValue,
this.name,
this.resources,
this.isDeleted,
this.steps,
this.flows,
this.connections,
this.description,
this.continuousDeliveryState,
this.isDraft,
this.currentState,
this.targetState,
this.deploymentVersion,
this.deployments,
this.board,
this.url));
}
/**
* Copy the current immutable object with elements that replace the content of {@link IntegrationOverview#getTags() tags}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of tags elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableIntegrationOverview withTags(Iterable elements) {
if (this.tags == elements) return this;
SortedSet newValue = createUnmodifiableSortedSet(false, createSafeList(elements, false, true));
return validate(new ImmutableIntegrationOverview(
this,
this.id,
this.properties,
this.configuredProperties,
this.version,
this.createdAt,
this.updatedAt,
newValue,
this.name,
this.resources,
this.isDeleted,
this.steps,
this.flows,
this.connections,
this.description,
this.continuousDeliveryState,
this.isDraft,
this.currentState,
this.targetState,
this.deploymentVersion,
this.deployments,
this.board,
this.url));
}
/**
* Copy the current immutable object by setting a value for the {@link IntegrationOverview#getName() name} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for name (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableIntegrationOverview withName(String value) {
if (Objects.equals(this.name, value)) return this;
return validate(new ImmutableIntegrationOverview(
this,
this.id,
this.properties,
this.configuredProperties,
this.version,
this.createdAt,
this.updatedAt,
this.tags,
value,
this.resources,
this.isDeleted,
this.steps,
this.flows,
this.connections,
this.description,
this.continuousDeliveryState,
this.isDraft,
this.currentState,
this.targetState,
this.deploymentVersion,
this.deployments,
this.board,
this.url));
}
/**
* Copy the current immutable object with elements that replace the content of {@link IntegrationOverview#getResources() resources}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableIntegrationOverview withResources(ResourceIdentifier... elements) {
List newValue = createUnmodifiableList(false, createSafeList(Arrays.asList(elements), true, false));
return validate(new ImmutableIntegrationOverview(
this,
this.id,
this.properties,
this.configuredProperties,
this.version,
this.createdAt,
this.updatedAt,
this.tags,
this.name,
newValue,
this.isDeleted,
this.steps,
this.flows,
this.connections,
this.description,
this.continuousDeliveryState,
this.isDraft,
this.currentState,
this.targetState,
this.deploymentVersion,
this.deployments,
this.board,
this.url));
}
/**
* Copy the current immutable object with elements that replace the content of {@link IntegrationOverview#getResources() resources}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of resources elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableIntegrationOverview withResources(Iterable extends ResourceIdentifier> elements) {
if (this.resources == elements) return this;
List newValue = createUnmodifiableList(false, createSafeList(elements, true, false));
return validate(new ImmutableIntegrationOverview(
this,
this.id,
this.properties,
this.configuredProperties,
this.version,
this.createdAt,
this.updatedAt,
this.tags,
this.name,
newValue,
this.isDeleted,
this.steps,
this.flows,
this.connections,
this.description,
this.continuousDeliveryState,
this.isDraft,
this.currentState,
this.targetState,
this.deploymentVersion,
this.deployments,
this.board,
this.url));
}
/**
* Copy the current immutable object by setting a value for the {@link IntegrationOverview#isDeleted() isDeleted} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for isDeleted
* @return A modified copy of the {@code this} object
*/
public final ImmutableIntegrationOverview withIsDeleted(boolean value) {
if (this.isDeleted == value) return this;
return validate(new ImmutableIntegrationOverview(
this,
this.id,
this.properties,
this.configuredProperties,
this.version,
this.createdAt,
this.updatedAt,
this.tags,
this.name,
this.resources,
value,
this.steps,
this.flows,
this.connections,
this.description,
this.continuousDeliveryState,
this.isDraft,
this.currentState,
this.targetState,
this.deploymentVersion,
this.deployments,
this.board,
this.url));
}
/**
* Copy the current immutable object with elements that replace the content of {@link IntegrationOverview#getSteps() steps}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
@Deprecated
public final ImmutableIntegrationOverview withSteps(Step... elements) {
List newValue = createUnmodifiableList(false, createSafeList(Arrays.asList(elements), true, false));
return validate(new ImmutableIntegrationOverview(
this,
this.id,
this.properties,
this.configuredProperties,
this.version,
this.createdAt,
this.updatedAt,
this.tags,
this.name,
this.resources,
this.isDeleted,
newValue,
this.flows,
this.connections,
this.description,
this.continuousDeliveryState,
this.isDraft,
this.currentState,
this.targetState,
this.deploymentVersion,
this.deployments,
this.board,
this.url));
}
/**
* Copy the current immutable object with elements that replace the content of {@link IntegrationOverview#getSteps() steps}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of steps elements to set
* @return A modified copy of {@code this} object
*/
@Deprecated
public final ImmutableIntegrationOverview withSteps(Iterable extends Step> elements) {
if (this.steps == elements) return this;
List newValue = createUnmodifiableList(false, createSafeList(elements, true, false));
return validate(new ImmutableIntegrationOverview(
this,
this.id,
this.properties,
this.configuredProperties,
this.version,
this.createdAt,
this.updatedAt,
this.tags,
this.name,
this.resources,
this.isDeleted,
newValue,
this.flows,
this.connections,
this.description,
this.continuousDeliveryState,
this.isDraft,
this.currentState,
this.targetState,
this.deploymentVersion,
this.deployments,
this.board,
this.url));
}
/**
* Copy the current immutable object with elements that replace the content of {@link IntegrationOverview#getFlows() flows}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableIntegrationOverview withFlows(Flow... elements) {
List newValue = createUnmodifiableList(false, createSafeList(Arrays.asList(elements), true, false));
return validate(new ImmutableIntegrationOverview(
this,
this.id,
this.properties,
this.configuredProperties,
this.version,
this.createdAt,
this.updatedAt,
this.tags,
this.name,
this.resources,
this.isDeleted,
this.steps,
newValue,
this.connections,
this.description,
this.continuousDeliveryState,
this.isDraft,
this.currentState,
this.targetState,
this.deploymentVersion,
this.deployments,
this.board,
this.url));
}
/**
* Copy the current immutable object with elements that replace the content of {@link IntegrationOverview#getFlows() flows}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of flows elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableIntegrationOverview withFlows(Iterable extends Flow> elements) {
if (this.flows == elements) return this;
List newValue = createUnmodifiableList(false, createSafeList(elements, true, false));
return validate(new ImmutableIntegrationOverview(
this,
this.id,
this.properties,
this.configuredProperties,
this.version,
this.createdAt,
this.updatedAt,
this.tags,
this.name,
this.resources,
this.isDeleted,
this.steps,
newValue,
this.connections,
this.description,
this.continuousDeliveryState,
this.isDraft,
this.currentState,
this.targetState,
this.deploymentVersion,
this.deployments,
this.board,
this.url));
}
/**
* Copy the current immutable object with elements that replace the content of {@link IntegrationOverview#getConnections() connections}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableIntegrationOverview withConnections(Connection... elements) {
List newValue = createUnmodifiableList(false, createSafeList(Arrays.asList(elements), true, false));
return validate(new ImmutableIntegrationOverview(
this,
this.id,
this.properties,
this.configuredProperties,
this.version,
this.createdAt,
this.updatedAt,
this.tags,
this.name,
this.resources,
this.isDeleted,
this.steps,
this.flows,
newValue,
this.description,
this.continuousDeliveryState,
this.isDraft,
this.currentState,
this.targetState,
this.deploymentVersion,
this.deployments,
this.board,
this.url));
}
/**
* Copy the current immutable object with elements that replace the content of {@link IntegrationOverview#getConnections() connections}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of connections elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableIntegrationOverview withConnections(Iterable extends Connection> elements) {
if (this.connections == elements) return this;
List newValue = createUnmodifiableList(false, createSafeList(elements, true, false));
return validate(new ImmutableIntegrationOverview(
this,
this.id,
this.properties,
this.configuredProperties,
this.version,
this.createdAt,
this.updatedAt,
this.tags,
this.name,
this.resources,
this.isDeleted,
this.steps,
this.flows,
newValue,
this.description,
this.continuousDeliveryState,
this.isDraft,
this.currentState,
this.targetState,
this.deploymentVersion,
this.deployments,
this.board,
this.url));
}
/**
* Copy the current immutable object by setting a present value for the optional {@link IntegrationOverview#getDescription() description} attribute.
* @param value The value for description
* @return A modified copy of {@code this} object
*/
public final ImmutableIntegrationOverview withDescription(String value) {
@Nullable String newValue = Objects.requireNonNull(value, "description");
if (Objects.equals(this.description, newValue)) return this;
return validate(new ImmutableIntegrationOverview(
this,
this.id,
this.properties,
this.configuredProperties,
this.version,
this.createdAt,
this.updatedAt,
this.tags,
this.name,
this.resources,
this.isDeleted,
this.steps,
this.flows,
this.connections,
newValue,
this.continuousDeliveryState,
this.isDraft,
this.currentState,
this.targetState,
this.deploymentVersion,
this.deployments,
this.board,
this.url));
}
/**
* Copy the current immutable object by setting an optional value for the {@link IntegrationOverview#getDescription() description} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for description
* @return A modified copy of {@code this} object
*/
public final ImmutableIntegrationOverview withDescription(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.description, value)) return this;
return validate(new ImmutableIntegrationOverview(
this,
this.id,
this.properties,
this.configuredProperties,
this.version,
this.createdAt,
this.updatedAt,
this.tags,
this.name,
this.resources,
this.isDeleted,
this.steps,
this.flows,
this.connections,
value,
this.continuousDeliveryState,
this.isDraft,
this.currentState,
this.targetState,
this.deploymentVersion,
this.deployments,
this.board,
this.url));
}
/**
* Copy the current immutable object by replacing the {@link IntegrationOverview#getContinuousDeliveryState() continuousDeliveryState} map with the specified map.
* Nulls are not permitted as keys or values.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param entries The entries to be added to the continuousDeliveryState map
* @return A modified copy of {@code this} object
*/
public final ImmutableIntegrationOverview withContinuousDeliveryState(Map entries) {
if (this.continuousDeliveryState == entries) return this;
Map newValue = createUnmodifiableMap(true, false, entries);
return validate(new ImmutableIntegrationOverview(
this,
this.id,
this.properties,
this.configuredProperties,
this.version,
this.createdAt,
this.updatedAt,
this.tags,
this.name,
this.resources,
this.isDeleted,
this.steps,
this.flows,
this.connections,
this.description,
newValue,
this.isDraft,
this.currentState,
this.targetState,
this.deploymentVersion,
this.deployments,
this.board,
this.url));
}
/**
* Copy the current immutable object by setting a value for the {@link IntegrationOverview#isDraft() isDraft} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for isDraft
* @return A modified copy of the {@code this} object
*/
public final ImmutableIntegrationOverview withIsDraft(boolean value) {
if (this.isDraft == value) return this;
return validate(new ImmutableIntegrationOverview(
this,
this.id,
this.properties,
this.configuredProperties,
this.version,
this.createdAt,
this.updatedAt,
this.tags,
this.name,
this.resources,
this.isDeleted,
this.steps,
this.flows,
this.connections,
this.description,
this.continuousDeliveryState,
value,
this.currentState,
this.targetState,
this.deploymentVersion,
this.deployments,
this.board,
this.url));
}
/**
* Copy the current immutable object by setting a value for the {@link IntegrationOverview#getCurrentState() currentState} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for currentState
* @return A modified copy of the {@code this} object
*/
public final ImmutableIntegrationOverview withCurrentState(IntegrationDeploymentState value) {
if (this.currentState == value) return this;
IntegrationDeploymentState newValue = Objects.requireNonNull(value, "currentState");
if (this.currentState.equals(newValue)) return this;
return validate(new ImmutableIntegrationOverview(
this,
this.id,
this.properties,
this.configuredProperties,
this.version,
this.createdAt,
this.updatedAt,
this.tags,
this.name,
this.resources,
this.isDeleted,
this.steps,
this.flows,
this.connections,
this.description,
this.continuousDeliveryState,
this.isDraft,
newValue,
this.targetState,
this.deploymentVersion,
this.deployments,
this.board,
this.url));
}
/**
* Copy the current immutable object by setting a value for the {@link IntegrationOverview#getTargetState() targetState} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for targetState
* @return A modified copy of the {@code this} object
*/
public final ImmutableIntegrationOverview withTargetState(IntegrationDeploymentState value) {
if (this.targetState == value) return this;
IntegrationDeploymentState newValue = Objects.requireNonNull(value, "targetState");
if (this.targetState.equals(newValue)) return this;
return validate(new ImmutableIntegrationOverview(
this,
this.id,
this.properties,
this.configuredProperties,
this.version,
this.createdAt,
this.updatedAt,
this.tags,
this.name,
this.resources,
this.isDeleted,
this.steps,
this.flows,
this.connections,
this.description,
this.continuousDeliveryState,
this.isDraft,
this.currentState,
newValue,
this.deploymentVersion,
this.deployments,
this.board,
this.url));
}
/**
* Copy the current immutable object by setting a present value for the optional {@link IntegrationOverview#getDeploymentVersion() deploymentVersion} attribute.
* @param value The value for deploymentVersion
* @return A modified copy of {@code this} object
*/
public final ImmutableIntegrationOverview withDeploymentVersion(int value) {
@Nullable Integer newValue = value;
if (Objects.equals(this.deploymentVersion, newValue)) return this;
return validate(new ImmutableIntegrationOverview(
this,
this.id,
this.properties,
this.configuredProperties,
this.version,
this.createdAt,
this.updatedAt,
this.tags,
this.name,
this.resources,
this.isDeleted,
this.steps,
this.flows,
this.connections,
this.description,
this.continuousDeliveryState,
this.isDraft,
this.currentState,
this.targetState,
newValue,
this.deployments,
this.board,
this.url));
}
/**
* Copy the current immutable object by setting an optional value for the {@link IntegrationOverview#getDeploymentVersion() deploymentVersion} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for deploymentVersion
* @return A modified copy of {@code this} object
*/
public final ImmutableIntegrationOverview withDeploymentVersion(Optional optional) {
@Nullable Integer value = optional.orElse(null);
if (Objects.equals(this.deploymentVersion, value)) return this;
return validate(new ImmutableIntegrationOverview(
this,
this.id,
this.properties,
this.configuredProperties,
this.version,
this.createdAt,
this.updatedAt,
this.tags,
this.name,
this.resources,
this.isDeleted,
this.steps,
this.flows,
this.connections,
this.description,
this.continuousDeliveryState,
this.isDraft,
this.currentState,
this.targetState,
value,
this.deployments,
this.board,
this.url));
}
/**
* Copy the current immutable object with elements that replace the content of {@link IntegrationOverview#getDeployments() deployments}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableIntegrationOverview withDeployments(IntegrationDeploymentOverview... elements) {
List newValue = createUnmodifiableList(false, createSafeList(Arrays.asList(elements), true, false));
return validate(new ImmutableIntegrationOverview(
this,
this.id,
this.properties,
this.configuredProperties,
this.version,
this.createdAt,
this.updatedAt,
this.tags,
this.name,
this.resources,
this.isDeleted,
this.steps,
this.flows,
this.connections,
this.description,
this.continuousDeliveryState,
this.isDraft,
this.currentState,
this.targetState,
this.deploymentVersion,
newValue,
this.board,
this.url));
}
/**
* Copy the current immutable object with elements that replace the content of {@link IntegrationOverview#getDeployments() deployments}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of deployments elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableIntegrationOverview withDeployments(Iterable extends IntegrationDeploymentOverview> elements) {
if (this.deployments == elements) return this;
List newValue = createUnmodifiableList(false, createSafeList(elements, true, false));
return validate(new ImmutableIntegrationOverview(
this,
this.id,
this.properties,
this.configuredProperties,
this.version,
this.createdAt,
this.updatedAt,
this.tags,
this.name,
this.resources,
this.isDeleted,
this.steps,
this.flows,
this.connections,
this.description,
this.continuousDeliveryState,
this.isDraft,
this.currentState,
this.targetState,
this.deploymentVersion,
newValue,
this.board,
this.url));
}
/**
* Copy the current immutable object by setting a value for the {@link IntegrationOverview#getBoard() board} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for board
* @return A modified copy of the {@code this} object
*/
public final ImmutableIntegrationOverview withBoard(IntegrationBulletinBoard value) {
if (this.board == value) return this;
IntegrationBulletinBoard newValue = Objects.requireNonNull(value, "board");
return validate(new ImmutableIntegrationOverview(
this,
this.id,
this.properties,
this.configuredProperties,
this.version,
this.createdAt,
this.updatedAt,
this.tags,
this.name,
this.resources,
this.isDeleted,
this.steps,
this.flows,
this.connections,
this.description,
this.continuousDeliveryState,
this.isDraft,
this.currentState,
this.targetState,
this.deploymentVersion,
this.deployments,
newValue,
this.url));
}
/**
* Copy the current immutable object by setting a present value for the optional {@link IntegrationOverview#getUrl() url} attribute.
* @param value The value for url
* @return A modified copy of {@code this} object
*/
public final ImmutableIntegrationOverview withUrl(String value) {
@Nullable String newValue = Objects.requireNonNull(value, "url");
if (Objects.equals(this.url, newValue)) return this;
return validate(new ImmutableIntegrationOverview(
this,
this.id,
this.properties,
this.configuredProperties,
this.version,
this.createdAt,
this.updatedAt,
this.tags,
this.name,
this.resources,
this.isDeleted,
this.steps,
this.flows,
this.connections,
this.description,
this.continuousDeliveryState,
this.isDraft,
this.currentState,
this.targetState,
this.deploymentVersion,
this.deployments,
this.board,
newValue));
}
/**
* Copy the current immutable object by setting an optional value for the {@link IntegrationOverview#getUrl() url} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for url
* @return A modified copy of {@code this} object
*/
public final ImmutableIntegrationOverview withUrl(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.url, value)) return this;
return validate(new ImmutableIntegrationOverview(
this,
this.id,
this.properties,
this.configuredProperties,
this.version,
this.createdAt,
this.updatedAt,
this.tags,
this.name,
this.resources,
this.isDeleted,
this.steps,
this.flows,
this.connections,
this.description,
this.continuousDeliveryState,
this.isDraft,
this.currentState,
this.targetState,
this.deploymentVersion,
this.deployments,
this.board,
value));
}
/**
* This instance is equal to all instances of {@code ImmutableIntegrationOverview} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof ImmutableIntegrationOverview
&& equalTo((ImmutableIntegrationOverview) another);
}
private boolean equalTo(ImmutableIntegrationOverview another) {
return Objects.equals(id, another.id)
&& properties.equals(another.properties)
&& configuredProperties.equals(another.configuredProperties)
&& version == another.version
&& createdAt == another.createdAt
&& updatedAt == another.updatedAt
&& tags.equals(another.tags)
&& Objects.equals(name, another.name)
&& resources.equals(another.resources)
&& isDeleted == another.isDeleted
&& steps.equals(another.steps)
&& flows.equals(another.flows)
&& connections.equals(another.connections)
&& Objects.equals(description, another.description)
&& continuousDeliveryState.equals(another.continuousDeliveryState)
&& isDraft == another.isDraft
&& currentState.equals(another.currentState)
&& targetState.equals(another.targetState)
&& Objects.equals(deploymentVersion, another.deploymentVersion)
&& deployments.equals(another.deployments)
&& board.equals(another.board)
&& Objects.equals(url, another.url);
}
/**
* Computes a hash code from attributes: {@code id}, {@code properties}, {@code configuredProperties}, {@code version}, {@code createdAt}, {@code updatedAt}, {@code tags}, {@code name}, {@code resources}, {@code isDeleted}, {@code steps}, {@code flows}, {@code connections}, {@code description}, {@code continuousDeliveryState}, {@code isDraft}, {@code currentState}, {@code targetState}, {@code deploymentVersion}, {@code deployments}, {@code board}, {@code url}.
* @return hashCode value
*/
@Override
public int hashCode() {
@Var int h = 5381;
h += (h << 5) + Objects.hashCode(id);
h += (h << 5) + properties.hashCode();
h += (h << 5) + configuredProperties.hashCode();
h += (h << 5) + version;
h += (h << 5) + Long.hashCode(createdAt);
h += (h << 5) + Long.hashCode(updatedAt);
h += (h << 5) + tags.hashCode();
h += (h << 5) + Objects.hashCode(name);
h += (h << 5) + resources.hashCode();
h += (h << 5) + Boolean.hashCode(isDeleted);
h += (h << 5) + steps.hashCode();
h += (h << 5) + flows.hashCode();
h += (h << 5) + connections.hashCode();
h += (h << 5) + Objects.hashCode(description);
h += (h << 5) + continuousDeliveryState.hashCode();
h += (h << 5) + Boolean.hashCode(isDraft);
h += (h << 5) + currentState.hashCode();
h += (h << 5) + targetState.hashCode();
h += (h << 5) + Objects.hashCode(deploymentVersion);
h += (h << 5) + deployments.hashCode();
h += (h << 5) + board.hashCode();
h += (h << 5) + Objects.hashCode(url);
return h;
}
/**
* Prints the immutable value {@code IntegrationOverview} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
StringBuilder builder = new StringBuilder("IntegrationOverview{");
if (id != null) {
builder.append("id=").append(id);
}
if (builder.length() > 20) builder.append(", ");
builder.append("properties=").append(properties);
builder.append(", ");
builder.append("configuredProperties=").append(configuredProperties);
builder.append(", ");
builder.append("version=").append(version);
builder.append(", ");
builder.append("createdAt=").append(createdAt);
builder.append(", ");
builder.append("updatedAt=").append(updatedAt);
builder.append(", ");
builder.append("tags=").append(tags);
if (name != null) {
builder.append(", ");
builder.append("name=").append(name);
}
builder.append(", ");
builder.append("resources=").append(resources);
builder.append(", ");
builder.append("isDeleted=").append(isDeleted);
builder.append(", ");
builder.append("steps=").append(steps);
builder.append(", ");
builder.append("flows=").append(flows);
builder.append(", ");
builder.append("connections=").append(connections);
if (description != null) {
builder.append(", ");
builder.append("description=").append(description);
}
builder.append(", ");
builder.append("continuousDeliveryState=").append(continuousDeliveryState);
builder.append(", ");
builder.append("isDraft=").append(isDraft);
builder.append(", ");
builder.append("currentState=").append(currentState);
builder.append(", ");
builder.append("targetState=").append(targetState);
if (deploymentVersion != null) {
builder.append(", ");
builder.append("deploymentVersion=").append(deploymentVersion);
}
builder.append(", ");
builder.append("deployments=").append(deployments);
builder.append(", ");
builder.append("board=").append(board);
if (url != null) {
builder.append(", ");
builder.append("url=").append(url);
}
return builder.append("}").toString();
}
/**
* Construct a new immutable {@code IntegrationOverview} instance.
* @param id The value for the {@code id} attribute
* @param properties The value for the {@code properties} attribute
* @param configuredProperties The value for the {@code configuredProperties} attribute
* @param version The value for the {@code version} attribute
* @param createdAt The value for the {@code createdAt} attribute
* @param updatedAt The value for the {@code updatedAt} attribute
* @param tags The value for the {@code tags} attribute
* @param name The value for the {@code name} attribute
* @param resources The value for the {@code resources} attribute
* @param isDeleted The value for the {@code isDeleted} attribute
* @param steps The value for the {@code steps} attribute
* @param flows The value for the {@code flows} attribute
* @param connections The value for the {@code connections} attribute
* @param description The value for the {@code description} attribute
* @param continuousDeliveryState The value for the {@code continuousDeliveryState} attribute
* @param isDraft The value for the {@code isDraft} attribute
* @param currentState The value for the {@code currentState} attribute
* @param targetState The value for the {@code targetState} attribute
* @param deploymentVersion The value for the {@code deploymentVersion} attribute
* @param deployments The value for the {@code deployments} attribute
* @param board The value for the {@code board} attribute
* @param url The value for the {@code url} attribute
* @return An immutable IntegrationOverview instance
*/
public static IntegrationOverview of(Optional id, Map properties, Map configuredProperties, int version, long createdAt, long updatedAt, SortedSet tags, String name, List resources, boolean isDeleted, List steps, List flows, List connections, Optional description, Map continuousDeliveryState, boolean isDraft, IntegrationDeploymentState currentState, IntegrationDeploymentState targetState, Optional deploymentVersion, List deployments, IntegrationBulletinBoard board, Optional url) {
return of(id, properties, configuredProperties, version, createdAt, updatedAt, (Iterable) tags, name, (Iterable extends ResourceIdentifier>) resources, isDeleted, (Iterable extends Step>) steps, (Iterable extends Flow>) flows, (Iterable extends Connection>) connections, description, continuousDeliveryState, isDraft, currentState, targetState, deploymentVersion, (Iterable extends IntegrationDeploymentOverview>) deployments, board, url);
}
/**
* Construct a new immutable {@code IntegrationOverview} instance.
* @param id The value for the {@code id} attribute
* @param properties The value for the {@code properties} attribute
* @param configuredProperties The value for the {@code configuredProperties} attribute
* @param version The value for the {@code version} attribute
* @param createdAt The value for the {@code createdAt} attribute
* @param updatedAt The value for the {@code updatedAt} attribute
* @param tags The value for the {@code tags} attribute
* @param name The value for the {@code name} attribute
* @param resources The value for the {@code resources} attribute
* @param isDeleted The value for the {@code isDeleted} attribute
* @param steps The value for the {@code steps} attribute
* @param flows The value for the {@code flows} attribute
* @param connections The value for the {@code connections} attribute
* @param description The value for the {@code description} attribute
* @param continuousDeliveryState The value for the {@code continuousDeliveryState} attribute
* @param isDraft The value for the {@code isDraft} attribute
* @param currentState The value for the {@code currentState} attribute
* @param targetState The value for the {@code targetState} attribute
* @param deploymentVersion The value for the {@code deploymentVersion} attribute
* @param deployments The value for the {@code deployments} attribute
* @param board The value for the {@code board} attribute
* @param url The value for the {@code url} attribute
* @return An immutable IntegrationOverview instance
*/
public static IntegrationOverview of(Optional id, Map properties, Map configuredProperties, int version, long createdAt, long updatedAt, Iterable tags, String name, Iterable extends ResourceIdentifier> resources, boolean isDeleted, Iterable extends Step> steps, Iterable extends Flow> flows, Iterable extends Connection> connections, Optional description, Map continuousDeliveryState, boolean isDraft, IntegrationDeploymentState currentState, IntegrationDeploymentState targetState, Optional deploymentVersion, Iterable extends IntegrationDeploymentOverview> deployments, IntegrationBulletinBoard board, Optional url) {
return validate(new ImmutableIntegrationOverview(id, properties, configuredProperties, version, createdAt, updatedAt, tags, name, resources, isDeleted, steps, flows, connections, description, continuousDeliveryState, isDraft, currentState, targetState, deploymentVersion, deployments, board, url));
}
private static final Validator validator = Validation.buildDefaultValidatorFactory().getValidator();
private static ImmutableIntegrationOverview validate(ImmutableIntegrationOverview instance) {
Set> constraintViolations = validator.validate(instance);
if (!constraintViolations.isEmpty()) {
throw new ConstraintViolationException(constraintViolations);
}
return instance;
}
/**
* Creates an immutable copy of a {@link IntegrationOverview} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable IntegrationOverview instance
*/
public static IntegrationOverview copyOf(IntegrationOverview instance) {
if (instance instanceof ImmutableIntegrationOverview) {
return (ImmutableIntegrationOverview) instance;
}
return new IntegrationOverview.Builder()
.createFrom(instance)
.build();
}
/**
* Builds instances of type {@link IntegrationOverview IntegrationOverview}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@Generated(from = "IntegrationOverview", generator = "Immutables")
@NotThreadSafe
public static class Builder {
private static final long OPT_BIT_CONFIGURED_PROPERTIES = 0x1L;
private static final long OPT_BIT_VERSION = 0x2L;
private static final long OPT_BIT_CREATED_AT = 0x4L;
private static final long OPT_BIT_UPDATED_AT = 0x8L;
private static final long OPT_BIT_RESOURCES = 0x10L;
private static final long OPT_BIT_IS_DELETED = 0x20L;
private static final long OPT_BIT_STEPS = 0x40L;
private static final long OPT_BIT_FLOWS = 0x80L;
private static final long OPT_BIT_CONNECTIONS = 0x100L;
private static final long OPT_BIT_CONTINUOUS_DELIVERY_STATE = 0x200L;
private static final long OPT_BIT_DEPLOYMENTS = 0x400L;
private long optBits;
private @Nullable String id;
private Map properties = new LinkedHashMap();
private Map configuredProperties = new LinkedHashMap();
private int version;
private long createdAt;
private long updatedAt;
private List tags = new ArrayList();
private @Nullable String name;
private List resources = new ArrayList();
private boolean isDeleted;
private List steps = new ArrayList();
private List flows = new ArrayList();
private List connections = new ArrayList();
private @Nullable String description;
private Map continuousDeliveryState = new LinkedHashMap();
private boolean isDraft;
private @Nullable IntegrationDeploymentState currentState;
private @Nullable IntegrationDeploymentState targetState;
private @Nullable Integer deploymentVersion;
private List deployments = new ArrayList();
private @Nullable IntegrationBulletinBoard board;
private @Nullable String url;
/**
* Creates a builder for {@link IntegrationOverview IntegrationOverview} instances.
*
* new IntegrationOverview.Builder()
* .id(String) // optional {@link IntegrationOverview#getId() id}
* .putProperty|putAllProperties(String => io.syndesis.common.model.connection.ConfigurationProperty) // {@link IntegrationOverview#getProperties() properties} mappings
* .putConfiguredProperty|putAllConfiguredProperties(String => String) // {@link IntegrationOverview#getConfiguredProperties() configuredProperties} mappings
* .version(int) // optional {@link IntegrationOverview#getVersion() version}
* .createdAt(long) // optional {@link IntegrationOverview#getCreatedAt() createdAt}
* .updatedAt(long) // optional {@link IntegrationOverview#getUpdatedAt() updatedAt}
* .addTag|addAllTags(String) // {@link IntegrationOverview#getTags() tags} elements
* .name(String | null) // nullable {@link IntegrationOverview#getName() name}
* .addResource|addAllResources(io.syndesis.common.model.ResourceIdentifier) // {@link IntegrationOverview#getResources() resources} elements
* .isDeleted(boolean) // optional {@link IntegrationOverview#isDeleted() isDeleted}
* .addStep|addAllSteps(io.syndesis.common.model.integration.Step) // {@link IntegrationOverview#getSteps() steps} elements
* .addFlow|addAllFlows(io.syndesis.common.model.integration.Flow) // {@link IntegrationOverview#getFlows() flows} elements
* .addConnection|addAllConnections(io.syndesis.common.model.connection.Connection) // {@link IntegrationOverview#getConnections() connections} elements
* .description(String) // optional {@link IntegrationOverview#getDescription() description}
* .putContinuousDeliveryState|putAllContinuousDeliveryState(String => io.syndesis.common.model.integration.ContinuousDeliveryEnvironment) // {@link IntegrationOverview#getContinuousDeliveryState() continuousDeliveryState} mappings
* .isDraft(boolean) // optional {@link IntegrationOverview#isDraft() isDraft}
* .currentState(io.syndesis.common.model.integration.IntegrationDeploymentState) // optional {@link IntegrationOverview#getCurrentState() currentState}
* .targetState(io.syndesis.common.model.integration.IntegrationDeploymentState) // optional {@link IntegrationOverview#getTargetState() targetState}
* .deploymentVersion(Integer) // optional {@link IntegrationOverview#getDeploymentVersion() deploymentVersion}
* .addDeployment|addAllDeployments(io.syndesis.common.model.integration.IntegrationDeploymentOverview) // {@link IntegrationOverview#getDeployments() deployments} elements
* .board(io.syndesis.common.model.bulletin.IntegrationBulletinBoard) // optional {@link IntegrationOverview#getBoard() board}
* .url(String) // optional {@link IntegrationOverview#getUrl() url}
* .build();
*
*/
public Builder() {
if (!(this instanceof IntegrationOverview.Builder)) {
throw new UnsupportedOperationException("Use: new IntegrationOverview.Builder()");
}
}
/**
* Fill a builder with attribute values from the provided {@code io.syndesis.common.model.WithName} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final IntegrationOverview.Builder createFrom(WithName instance) {
Objects.requireNonNull(instance, "instance");
from((Object) instance);
return (IntegrationOverview.Builder) this;
}
/**
* Fill a builder with attribute values from the provided {@code io.syndesis.common.model.integration.IntegrationBase} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final IntegrationOverview.Builder createFrom(IntegrationBase instance) {
Objects.requireNonNull(instance, "instance");
from((Object) instance);
return (IntegrationOverview.Builder) this;
}
/**
* Fill a builder with attribute values from the provided {@code io.syndesis.common.model.WithConfiguredProperties} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final IntegrationOverview.Builder createFrom(WithConfiguredProperties instance) {
Objects.requireNonNull(instance, "instance");
from((Object) instance);
return (IntegrationOverview.Builder) this;
}
/**
* Fill a builder with attribute values from the provided {@code io.syndesis.common.model.integration.WithSteps} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final IntegrationOverview.Builder createFrom(WithSteps instance) {
Objects.requireNonNull(instance, "instance");
from((Object) instance);
return (IntegrationOverview.Builder) this;
}
/**
* Fill a builder with attribute values from the provided {@code io.syndesis.common.model.WithResources} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final IntegrationOverview.Builder createFrom(WithResources instance) {
Objects.requireNonNull(instance, "instance");
from((Object) instance);
return (IntegrationOverview.Builder) this;
}
/**
* Fill a builder with attribute values from the provided {@code io.syndesis.common.model.WithVersion} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final IntegrationOverview.Builder createFrom(WithVersion instance) {
Objects.requireNonNull(instance, "instance");
from((Object) instance);
return (IntegrationOverview.Builder) this;
}
/**
* Fill a builder with attribute values from the provided {@code io.syndesis.common.model.integration.IntegrationOverview} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final IntegrationOverview.Builder createFrom(IntegrationOverview instance) {
Objects.requireNonNull(instance, "instance");
from((Object) instance);
return (IntegrationOverview.Builder) this;
}
/**
* Fill a builder with attribute values from the provided {@code io.syndesis.common.model.WithTags} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final IntegrationOverview.Builder createFrom(WithTags instance) {
Objects.requireNonNull(instance, "instance");
from((Object) instance);
return (IntegrationOverview.Builder) this;
}
/**
* Fill a builder with attribute values from the provided {@code io.syndesis.common.model.WithResourceId} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final IntegrationOverview.Builder createFrom(WithResourceId instance) {
Objects.requireNonNull(instance, "instance");
from((Object) instance);
return (IntegrationOverview.Builder) this;
}
/**
* Fill a builder with attribute values from the provided {@code io.syndesis.common.model.WithModificationTimestamps} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final IntegrationOverview.Builder createFrom(WithModificationTimestamps instance) {
Objects.requireNonNull(instance, "instance");
from((Object) instance);
return (IntegrationOverview.Builder) this;
}
/**
* Fill a builder with attribute values from the provided {@code io.syndesis.common.model.WithConfigurationProperties} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final IntegrationOverview.Builder createFrom(WithConfigurationProperties instance) {
Objects.requireNonNull(instance, "instance");
from((Object) instance);
return (IntegrationOverview.Builder) this;
}
private void from(Object object) {
@Var long bits = 0;
if (object instanceof WithName) {
WithName instance = (WithName) object;
String nameValue = instance.getName();
if (nameValue != null) {
name(nameValue);
}
}
if (object instanceof IntegrationBase) {
IntegrationBase instance = (IntegrationBase) object;
putAllContinuousDeliveryState(instance.getContinuousDeliveryState());
Optional descriptionOptional = instance.getDescription();
if (descriptionOptional.isPresent()) {
description(descriptionOptional);
}
isDeleted(instance.isDeleted());
if ((bits & 0x1L) == 0) {
addAllSteps(instance.getSteps());
bits |= 0x1L;
}
addAllFlows(instance.getFlows());
addAllConnections(instance.getConnections());
}
if (object instanceof WithConfiguredProperties) {
WithConfiguredProperties instance = (WithConfiguredProperties) object;
putAllConfiguredProperties(instance.getConfiguredProperties());
}
if (object instanceof WithSteps) {
WithSteps instance = (WithSteps) object;
if ((bits & 0x1L) == 0) {
addAllSteps(instance.getSteps());
bits |= 0x1L;
}
}
if (object instanceof WithResources) {
WithResources instance = (WithResources) object;
addAllResources(instance.getResources());
}
if (object instanceof WithVersion) {
WithVersion instance = (WithVersion) object;
version(instance.getVersion());
}
if (object instanceof IntegrationOverview) {
IntegrationOverview instance = (IntegrationOverview) object;
addAllDeployments(instance.getDeployments());
Optional deploymentVersionOptional = instance.getDeploymentVersion();
if (deploymentVersionOptional.isPresent()) {
deploymentVersion(deploymentVersionOptional);
}
targetState(instance.getTargetState());
isDraft(instance.isDraft());
currentState(instance.getCurrentState());
Optional urlOptional = instance.getUrl();
if (urlOptional.isPresent()) {
url(urlOptional);
}
board(instance.getBoard());
}
if (object instanceof WithTags) {
WithTags instance = (WithTags) object;
addAllTags(instance.getTags());
}
if (object instanceof WithResourceId) {
WithResourceId instance = (WithResourceId) object;
Optional idOptional = instance.getId();
if (idOptional.isPresent()) {
id(idOptional);
}
}
if (object instanceof WithModificationTimestamps) {
WithModificationTimestamps instance = (WithModificationTimestamps) object;
createdAt(instance.getCreatedAt());
updatedAt(instance.getUpdatedAt());
}
if (object instanceof WithConfigurationProperties) {
WithConfigurationProperties instance = (WithConfigurationProperties) object;
putAllProperties(instance.getProperties());
}
}
/**
* Initializes the optional value {@link IntegrationOverview#getId() id} to id.
* @param id The value for id
* @return {@code this} builder for chained invocation
*/
@CanIgnoreReturnValue
public final IntegrationOverview.Builder id(String id) {
this.id = Objects.requireNonNull(id, "id");
return (IntegrationOverview.Builder) this;
}
/**
* Initializes the optional value {@link IntegrationOverview#getId() id} to id.
* @param id The value for id
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("id")
public final IntegrationOverview.Builder id(Optional id) {
this.id = id.orElse(null);
return (IntegrationOverview.Builder) this;
}
/**
* Put one entry to the {@link IntegrationOverview#getProperties() properties} map.
* @param key The key in the properties map
* @param value The associated value in the properties map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final IntegrationOverview.Builder putProperty(String key, ConfigurationProperty value) {
this.properties.put(
Objects.requireNonNull(key, "properties key"),
Objects.requireNonNull(value, "properties value"));
return (IntegrationOverview.Builder) this;
}
/**
* Put one entry to the {@link IntegrationOverview#getProperties() properties} map. Nulls are not permitted
* @param entry The key and value entry
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final IntegrationOverview.Builder putProperty(Map.Entry entry) {
String k = entry.getKey();
ConfigurationProperty v = entry.getValue();
this.properties.put(
Objects.requireNonNull(k, "properties key"),
Objects.requireNonNull(v, "properties value"));
return (IntegrationOverview.Builder) this;
}
/**
* Sets or replaces all mappings from the specified map as entries for the {@link IntegrationOverview#getProperties() properties} map. Nulls are not permitted
* @param entries The entries that will be added to the properties map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("properties")
public final IntegrationOverview.Builder properties(Map entries) {
this.properties.clear();
return putAllProperties(entries);
}
/**
* Put all mappings from the specified map as entries to {@link IntegrationOverview#getProperties() properties} map. Nulls are not permitted
* @param entries The entries that will be added to the properties map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final IntegrationOverview.Builder putAllProperties(Map entries) {
for (Map.Entry e : entries.entrySet()) {
String k = e.getKey();
ConfigurationProperty v = e.getValue();
this.properties.put(
Objects.requireNonNull(k, "properties key"),
Objects.requireNonNull(v, "properties value"));
}
return (IntegrationOverview.Builder) this;
}
/**
* Put one entry to the {@link IntegrationOverview#getConfiguredProperties() configuredProperties} map.
* @param key The key in the configuredProperties map
* @param value The associated value in the configuredProperties map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final IntegrationOverview.Builder putConfiguredProperty(String key, String value) {
this.configuredProperties.put(
Objects.requireNonNull(key, "configuredProperties key"),
Objects.requireNonNull(value, "configuredProperties value"));
optBits |= OPT_BIT_CONFIGURED_PROPERTIES;
return (IntegrationOverview.Builder) this;
}
/**
* Put one entry to the {@link IntegrationOverview#getConfiguredProperties() configuredProperties} map. Nulls are not permitted
* @param entry The key and value entry
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final IntegrationOverview.Builder putConfiguredProperty(Map.Entry entry) {
String k = entry.getKey();
String v = entry.getValue();
this.configuredProperties.put(
Objects.requireNonNull(k, "configuredProperties key"),
Objects.requireNonNull(v, "configuredProperties value"));
optBits |= OPT_BIT_CONFIGURED_PROPERTIES;
return (IntegrationOverview.Builder) this;
}
/**
* Sets or replaces all mappings from the specified map as entries for the {@link IntegrationOverview#getConfiguredProperties() configuredProperties} map. Nulls are not permitted
* @param entries The entries that will be added to the configuredProperties map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("configuredProperties")
public final IntegrationOverview.Builder configuredProperties(Map entries) {
this.configuredProperties.clear();
optBits |= OPT_BIT_CONFIGURED_PROPERTIES;
return putAllConfiguredProperties(entries);
}
/**
* Put all mappings from the specified map as entries to {@link IntegrationOverview#getConfiguredProperties() configuredProperties} map. Nulls are not permitted
* @param entries The entries that will be added to the configuredProperties map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final IntegrationOverview.Builder putAllConfiguredProperties(Map entries) {
for (Map.Entry e : entries.entrySet()) {
String k = e.getKey();
String v = e.getValue();
this.configuredProperties.put(
Objects.requireNonNull(k, "configuredProperties key"),
Objects.requireNonNull(v, "configuredProperties value"));
}
optBits |= OPT_BIT_CONFIGURED_PROPERTIES;
return (IntegrationOverview.Builder) this;
}
/**
* Initializes the value for the {@link IntegrationOverview#getVersion() version} attribute.
* If not set, this attribute will have a default value as returned by the initializer of {@link IntegrationOverview#getVersion() version}.
* @param version The value for version
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("version")
public final IntegrationOverview.Builder version(int version) {
this.version = version;
optBits |= OPT_BIT_VERSION;
return (IntegrationOverview.Builder) this;
}
/**
* Initializes the value for the {@link IntegrationOverview#getCreatedAt() createdAt} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link IntegrationOverview#getCreatedAt() createdAt}.
* @param createdAt The value for createdAt
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("createdAt")
public final IntegrationOverview.Builder createdAt(long createdAt) {
this.createdAt = createdAt;
optBits |= OPT_BIT_CREATED_AT;
return (IntegrationOverview.Builder) this;
}
/**
* Initializes the value for the {@link IntegrationOverview#getUpdatedAt() updatedAt} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link IntegrationOverview#getUpdatedAt() updatedAt}.
* @param updatedAt The value for updatedAt
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("updatedAt")
public final IntegrationOverview.Builder updatedAt(long updatedAt) {
this.updatedAt = updatedAt;
optBits |= OPT_BIT_UPDATED_AT;
return (IntegrationOverview.Builder) this;
}
/**
* Adds one element to {@link IntegrationOverview#getTags() tags} sortedSet.
* @param element A tags element
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final IntegrationOverview.Builder addTag(@Nullable String element) {
if (element != null) this.tags.add(element);
return (IntegrationOverview.Builder) this;
}
/**
* Adds elements to {@link IntegrationOverview#getTags() tags} sortedSet.
* @param elements An array of tags elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final IntegrationOverview.Builder addTags(String... elements) {
for (String element : elements) {
if (element != null) this.tags.add(element);
}
return (IntegrationOverview.Builder) this;
}
/**
* Sets or replaces all elements for {@link IntegrationOverview#getTags() tags} sortedSet.
* @param elements An iterable of tags elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("tags")
@JsonDeserialize(contentConverter = StringTrimmingConverter.class)
public final IntegrationOverview.Builder tags(Iterable elements) {
this.tags.clear();
return addAllTags(elements);
}
/**
* Adds elements to {@link IntegrationOverview#getTags() tags} sortedSet.
* @param elements An iterable of tags elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final IntegrationOverview.Builder addAllTags(Iterable elements) {
for (String element : elements) {
if (element != null) this.tags.add(element);
}
return (IntegrationOverview.Builder) this;
}
/**
* Initializes the value for the {@link IntegrationOverview#getName() name} attribute.
* @param name The value for name (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("name")
@JsonDeserialize(converter = StringTrimmingConverter.class)
public final IntegrationOverview.Builder name(String name) {
this.name = name;
return (IntegrationOverview.Builder) this;
}
/**
* Adds one element to {@link IntegrationOverview#getResources() resources} list.
* @param element A resources element
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final IntegrationOverview.Builder addResource(ResourceIdentifier element) {
this.resources.add(Objects.requireNonNull(element, "resources element"));
optBits |= OPT_BIT_RESOURCES;
return (IntegrationOverview.Builder) this;
}
/**
* Adds elements to {@link IntegrationOverview#getResources() resources} list.
* @param elements An array of resources elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final IntegrationOverview.Builder addResources(ResourceIdentifier... elements) {
for (ResourceIdentifier element : elements) {
this.resources.add(Objects.requireNonNull(element, "resources element"));
}
optBits |= OPT_BIT_RESOURCES;
return (IntegrationOverview.Builder) this;
}
/**
* Sets or replaces all elements for {@link IntegrationOverview#getResources() resources} list.
* @param elements An iterable of resources elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("resources")
public final IntegrationOverview.Builder resources(Iterable extends ResourceIdentifier> elements) {
this.resources.clear();
return addAllResources(elements);
}
/**
* Adds elements to {@link IntegrationOverview#getResources() resources} list.
* @param elements An iterable of resources elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final IntegrationOverview.Builder addAllResources(Iterable extends ResourceIdentifier> elements) {
for (ResourceIdentifier element : elements) {
this.resources.add(Objects.requireNonNull(element, "resources element"));
}
optBits |= OPT_BIT_RESOURCES;
return (IntegrationOverview.Builder) this;
}
/**
* Initializes the value for the {@link IntegrationOverview#isDeleted() isDeleted} attribute.
* If not set, this attribute will have a default value as returned by the initializer of {@link IntegrationOverview#isDeleted() isDeleted}.
* @param isDeleted The value for isDeleted
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("isDeleted")
@JsonInclude(JsonInclude.Include.NON_DEFAULT)
public final IntegrationOverview.Builder isDeleted(boolean isDeleted) {
this.isDeleted = isDeleted;
optBits |= OPT_BIT_IS_DELETED;
return (IntegrationOverview.Builder) this;
}
/**
* Adds one element to {@link IntegrationOverview#getSteps() steps} list.
* @param element A steps element
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@Deprecated
public final IntegrationOverview.Builder addStep(Step element) {
this.steps.add(Objects.requireNonNull(element, "steps element"));
optBits |= OPT_BIT_STEPS;
return (IntegrationOverview.Builder) this;
}
/**
* Adds elements to {@link IntegrationOverview#getSteps() steps} list.
* @param elements An array of steps elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@Deprecated
public final IntegrationOverview.Builder addSteps(Step... elements) {
for (Step element : elements) {
this.steps.add(Objects.requireNonNull(element, "steps element"));
}
optBits |= OPT_BIT_STEPS;
return (IntegrationOverview.Builder) this;
}
/**
* Sets or replaces all elements for {@link IntegrationOverview#getSteps() steps} list.
* @param elements An iterable of steps elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("steps")
@Deprecated
public final IntegrationOverview.Builder steps(Iterable extends Step> elements) {
this.steps.clear();
return addAllSteps(elements);
}
/**
* Adds elements to {@link IntegrationOverview#getSteps() steps} list.
* @param elements An iterable of steps elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@Deprecated
public final IntegrationOverview.Builder addAllSteps(Iterable extends Step> elements) {
for (Step element : elements) {
this.steps.add(Objects.requireNonNull(element, "steps element"));
}
optBits |= OPT_BIT_STEPS;
return (IntegrationOverview.Builder) this;
}
/**
* Adds one element to {@link IntegrationOverview#getFlows() flows} list.
* @param element A flows element
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final IntegrationOverview.Builder addFlow(Flow element) {
this.flows.add(Objects.requireNonNull(element, "flows element"));
optBits |= OPT_BIT_FLOWS;
return (IntegrationOverview.Builder) this;
}
/**
* Adds elements to {@link IntegrationOverview#getFlows() flows} list.
* @param elements An array of flows elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final IntegrationOverview.Builder addFlows(Flow... elements) {
for (Flow element : elements) {
this.flows.add(Objects.requireNonNull(element, "flows element"));
}
optBits |= OPT_BIT_FLOWS;
return (IntegrationOverview.Builder) this;
}
/**
* Sets or replaces all elements for {@link IntegrationOverview#getFlows() flows} list.
* @param elements An iterable of flows elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("flows")
public final IntegrationOverview.Builder flows(Iterable extends Flow> elements) {
this.flows.clear();
return addAllFlows(elements);
}
/**
* Adds elements to {@link IntegrationOverview#getFlows() flows} list.
* @param elements An iterable of flows elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final IntegrationOverview.Builder addAllFlows(Iterable extends Flow> elements) {
for (Flow element : elements) {
this.flows.add(Objects.requireNonNull(element, "flows element"));
}
optBits |= OPT_BIT_FLOWS;
return (IntegrationOverview.Builder) this;
}
/**
* Adds one element to {@link IntegrationOverview#getConnections() connections} list.
* @param element A connections element
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final IntegrationOverview.Builder addConnection(Connection element) {
this.connections.add(Objects.requireNonNull(element, "connections element"));
optBits |= OPT_BIT_CONNECTIONS;
return (IntegrationOverview.Builder) this;
}
/**
* Adds elements to {@link IntegrationOverview#getConnections() connections} list.
* @param elements An array of connections elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final IntegrationOverview.Builder addConnections(Connection... elements) {
for (Connection element : elements) {
this.connections.add(Objects.requireNonNull(element, "connections element"));
}
optBits |= OPT_BIT_CONNECTIONS;
return (IntegrationOverview.Builder) this;
}
/**
* Sets or replaces all elements for {@link IntegrationOverview#getConnections() connections} list.
* @param elements An iterable of connections elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("connections")
public final IntegrationOverview.Builder connections(Iterable extends Connection> elements) {
this.connections.clear();
return addAllConnections(elements);
}
/**
* Adds elements to {@link IntegrationOverview#getConnections() connections} list.
* @param elements An iterable of connections elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final IntegrationOverview.Builder addAllConnections(Iterable extends Connection> elements) {
for (Connection element : elements) {
this.connections.add(Objects.requireNonNull(element, "connections element"));
}
optBits |= OPT_BIT_CONNECTIONS;
return (IntegrationOverview.Builder) this;
}
/**
* Initializes the optional value {@link IntegrationOverview#getDescription() description} to description.
* @param description The value for description
* @return {@code this} builder for chained invocation
*/
@CanIgnoreReturnValue
public final IntegrationOverview.Builder description(String description) {
this.description = Objects.requireNonNull(description, "description");
return (IntegrationOverview.Builder) this;
}
/**
* Initializes the optional value {@link IntegrationOverview#getDescription() description} to description.
* @param description The value for description
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("description")
@JsonDeserialize(converter = OptionalStringTrimmingConverter.class)
public final IntegrationOverview.Builder description(Optional description) {
this.description = description.orElse(null);
return (IntegrationOverview.Builder) this;
}
/**
* Put one entry to the {@link IntegrationOverview#getContinuousDeliveryState() continuousDeliveryState} map.
* @param key The key in the continuousDeliveryState map
* @param value The associated value in the continuousDeliveryState map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final IntegrationOverview.Builder putContinuousDeliveryState(String key, ContinuousDeliveryEnvironment value) {
this.continuousDeliveryState.put(
Objects.requireNonNull(key, "continuousDeliveryState key"),
Objects.requireNonNull(value, "continuousDeliveryState value"));
optBits |= OPT_BIT_CONTINUOUS_DELIVERY_STATE;
return (IntegrationOverview.Builder) this;
}
/**
* Put one entry to the {@link IntegrationOverview#getContinuousDeliveryState() continuousDeliveryState} map. Nulls are not permitted
* @param entry The key and value entry
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final IntegrationOverview.Builder putContinuousDeliveryState(Map.Entry entry) {
String k = entry.getKey();
ContinuousDeliveryEnvironment v = entry.getValue();
this.continuousDeliveryState.put(
Objects.requireNonNull(k, "continuousDeliveryState key"),
Objects.requireNonNull(v, "continuousDeliveryState value"));
optBits |= OPT_BIT_CONTINUOUS_DELIVERY_STATE;
return (IntegrationOverview.Builder) this;
}
/**
* Sets or replaces all mappings from the specified map as entries for the {@link IntegrationOverview#getContinuousDeliveryState() continuousDeliveryState} map. Nulls are not permitted
* @param entries The entries that will be added to the continuousDeliveryState map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("continuousDeliveryState")
public final IntegrationOverview.Builder continuousDeliveryState(Map entries) {
this.continuousDeliveryState.clear();
optBits |= OPT_BIT_CONTINUOUS_DELIVERY_STATE;
return putAllContinuousDeliveryState(entries);
}
/**
* Put all mappings from the specified map as entries to {@link IntegrationOverview#getContinuousDeliveryState() continuousDeliveryState} map. Nulls are not permitted
* @param entries The entries that will be added to the continuousDeliveryState map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final IntegrationOverview.Builder putAllContinuousDeliveryState(Map entries) {
for (Map.Entry e : entries.entrySet()) {
String k = e.getKey();
ContinuousDeliveryEnvironment v = e.getValue();
this.continuousDeliveryState.put(
Objects.requireNonNull(k, "continuousDeliveryState key"),
Objects.requireNonNull(v, "continuousDeliveryState value"));
}
optBits |= OPT_BIT_CONTINUOUS_DELIVERY_STATE;
return (IntegrationOverview.Builder) this;
}
/**
* Initializes the value for the {@link IntegrationOverview#isDraft() isDraft} attribute.
* @param isDraft The value for isDraft
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("isDraft")
public final IntegrationOverview.Builder isDraft(boolean isDraft) {
this.isDraft = isDraft;
return (IntegrationOverview.Builder) this;
}
/**
* Initializes the value for the {@link IntegrationOverview#getCurrentState() currentState} attribute.
* If not set, this attribute will have a default value as returned by the initializer of {@link IntegrationOverview#getCurrentState() currentState}.
* @param currentState The value for currentState
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("currentState")
public final IntegrationOverview.Builder currentState(IntegrationDeploymentState currentState) {
this.currentState = Objects.requireNonNull(currentState, "currentState");
return (IntegrationOverview.Builder) this;
}
/**
* Initializes the value for the {@link IntegrationOverview#getTargetState() targetState} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link IntegrationOverview#getTargetState() targetState}.
* @param targetState The value for targetState
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("targetState")
public final IntegrationOverview.Builder targetState(IntegrationDeploymentState targetState) {
this.targetState = Objects.requireNonNull(targetState, "targetState");
return (IntegrationOverview.Builder) this;
}
/**
* Initializes the optional value {@link IntegrationOverview#getDeploymentVersion() deploymentVersion} to deploymentVersion.
* @param deploymentVersion The value for deploymentVersion
* @return {@code this} builder for chained invocation
*/
@CanIgnoreReturnValue
public final IntegrationOverview.Builder deploymentVersion(int deploymentVersion) {
this.deploymentVersion = deploymentVersion;
return (IntegrationOverview.Builder) this;
}
/**
* Initializes the optional value {@link IntegrationOverview#getDeploymentVersion() deploymentVersion} to deploymentVersion.
* @param deploymentVersion The value for deploymentVersion
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("deploymentVersion")
public final IntegrationOverview.Builder deploymentVersion(Optional deploymentVersion) {
this.deploymentVersion = deploymentVersion.orElse(null);
return (IntegrationOverview.Builder) this;
}
/**
* Adds one element to {@link IntegrationOverview#getDeployments() deployments} list.
* @param element A deployments element
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final IntegrationOverview.Builder addDeployment(IntegrationDeploymentOverview element) {
this.deployments.add(Objects.requireNonNull(element, "deployments element"));
optBits |= OPT_BIT_DEPLOYMENTS;
return (IntegrationOverview.Builder) this;
}
/**
* Adds elements to {@link IntegrationOverview#getDeployments() deployments} list.
* @param elements An array of deployments elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final IntegrationOverview.Builder addDeployments(IntegrationDeploymentOverview... elements) {
for (IntegrationDeploymentOverview element : elements) {
this.deployments.add(Objects.requireNonNull(element, "deployments element"));
}
optBits |= OPT_BIT_DEPLOYMENTS;
return (IntegrationOverview.Builder) this;
}
/**
* Sets or replaces all elements for {@link IntegrationOverview#getDeployments() deployments} list.
* @param elements An iterable of deployments elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("deployments")
public final IntegrationOverview.Builder deployments(Iterable extends IntegrationDeploymentOverview> elements) {
this.deployments.clear();
return addAllDeployments(elements);
}
/**
* Adds elements to {@link IntegrationOverview#getDeployments() deployments} list.
* @param elements An iterable of deployments elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final IntegrationOverview.Builder addAllDeployments(Iterable extends IntegrationDeploymentOverview> elements) {
for (IntegrationDeploymentOverview element : elements) {
this.deployments.add(Objects.requireNonNull(element, "deployments element"));
}
optBits |= OPT_BIT_DEPLOYMENTS;
return (IntegrationOverview.Builder) this;
}
/**
* Initializes the value for the {@link IntegrationOverview#getBoard() board} attribute.
* If not set, this attribute will have a default value as returned by the initializer of {@link IntegrationOverview#getBoard() board}.
* @param board The value for board
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("board")
public final IntegrationOverview.Builder board(IntegrationBulletinBoard board) {
this.board = Objects.requireNonNull(board, "board");
return (IntegrationOverview.Builder) this;
}
/**
* Initializes the optional value {@link IntegrationOverview#getUrl() url} to url.
* @param url The value for url
* @return {@code this} builder for chained invocation
*/
@CanIgnoreReturnValue
public final IntegrationOverview.Builder url(String url) {
this.url = Objects.requireNonNull(url, "url");
return (IntegrationOverview.Builder) this;
}
/**
* Initializes the optional value {@link IntegrationOverview#getUrl() url} to url.
* @param url The value for url
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("url")
public final IntegrationOverview.Builder url(Optional url) {
this.url = url.orElse(null);
return (IntegrationOverview.Builder) this;
}
/**
* Builds a new {@link IntegrationOverview IntegrationOverview}.
* @return An immutable instance of IntegrationOverview
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public IntegrationOverview build() {
return ImmutableIntegrationOverview.validate(new ImmutableIntegrationOverview(this));
}
private boolean configuredPropertiesIsSet() {
return (optBits & OPT_BIT_CONFIGURED_PROPERTIES) != 0;
}
private boolean versionIsSet() {
return (optBits & OPT_BIT_VERSION) != 0;
}
private boolean createdAtIsSet() {
return (optBits & OPT_BIT_CREATED_AT) != 0;
}
private boolean updatedAtIsSet() {
return (optBits & OPT_BIT_UPDATED_AT) != 0;
}
private boolean resourcesIsSet() {
return (optBits & OPT_BIT_RESOURCES) != 0;
}
private boolean isDeletedIsSet() {
return (optBits & OPT_BIT_IS_DELETED) != 0;
}
private boolean stepsIsSet() {
return (optBits & OPT_BIT_STEPS) != 0;
}
private boolean flowsIsSet() {
return (optBits & OPT_BIT_FLOWS) != 0;
}
private boolean connectionsIsSet() {
return (optBits & OPT_BIT_CONNECTIONS) != 0;
}
private boolean continuousDeliveryStateIsSet() {
return (optBits & OPT_BIT_CONTINUOUS_DELIVERY_STATE) != 0;
}
private boolean deploymentsIsSet() {
return (optBits & OPT_BIT_DEPLOYMENTS) != 0;
}
}
private static List createSafeList(Iterable extends T> iterable, boolean checkNulls, boolean skipNulls) {
ArrayList list;
if (iterable instanceof Collection>) {
int size = ((Collection>) iterable).size();
if (size == 0) return Collections.emptyList();
list = new ArrayList<>();
} else {
list = new ArrayList<>();
}
for (T element : iterable) {
if (skipNulls && element == null) continue;
if (checkNulls) Objects.requireNonNull(element, "element");
list.add(element);
}
return list;
}
private static List createUnmodifiableList(boolean clone, List list) {
switch(list.size()) {
case 0: return Collections.emptyList();
case 1: return Collections.singletonList(list.get(0));
default:
if (clone) {
return Collections.unmodifiableList(new ArrayList<>(list));
} else {
if (list instanceof ArrayList>) {
((ArrayList>) list).trimToSize();
}
return Collections.unmodifiableList(list);
}
}
}
private static > NavigableSet createUnmodifiableSortedSet(boolean reverse, List list) {
TreeSet set = reverse
? new TreeSet<>(Collections.reverseOrder())
: new TreeSet<>();
set.addAll(list);
return Collections.unmodifiableNavigableSet(set);
}
private static Map createUnmodifiableMap(boolean checkNulls, boolean skipNulls, Map extends K, ? extends V> map) {
switch (map.size()) {
case 0: return Collections.emptyMap();
case 1: {
Map.Entry extends K, ? extends V> e = map.entrySet().iterator().next();
K k = e.getKey();
V v = e.getValue();
if (checkNulls) {
Objects.requireNonNull(k, "key");
Objects.requireNonNull(v, "value");
}
if (skipNulls && (k == null || v == null)) {
return Collections.emptyMap();
}
return Collections.singletonMap(k, v);
}
default: {
Map linkedMap = new LinkedHashMap<>(map.size());
if (skipNulls || checkNulls) {
for (Map.Entry extends K, ? extends V> e : map.entrySet()) {
K k = e.getKey();
V v = e.getValue();
if (skipNulls) {
if (k == null || v == null) continue;
} else if (checkNulls) {
Objects.requireNonNull(k, "key");
Objects.requireNonNull(v, "value");
}
linkedMap.put(k, v);
}
} else {
linkedMap.putAll(map);
}
return Collections.unmodifiableMap(linkedMap);
}
}
}
}