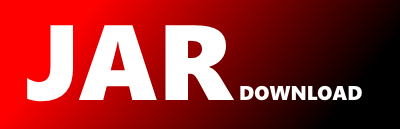
io.syndesis.connector.odata.component.ODataComponent Maven / Gradle / Ivy
/*
* Copyright (C) 2016 Red Hat, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.syndesis.connector.odata.component;
import java.util.HashMap;
import java.util.Map;
import java.util.Optional;
import org.apache.camel.Component;
import org.apache.camel.Endpoint;
import org.apache.camel.component.olingo4.Olingo4AppEndpointConfiguration;
import org.apache.camel.component.olingo4.Olingo4Component;
import org.apache.camel.util.ObjectHelper;
import org.apache.http.impl.client.HttpClientBuilder;
import org.apache.http.impl.nio.client.HttpAsyncClientBuilder;
import io.syndesis.connector.odata.ODataConstants;
import io.syndesis.connector.odata.ODataUtil;
import io.syndesis.connector.support.util.ConnectorOptions;
import io.syndesis.connector.support.util.PropertyBuilder;
import io.syndesis.integration.component.proxy.ComponentDefinition;
import io.syndesis.integration.component.proxy.ComponentProxyComponent;
public final class ODataComponent extends ComponentProxyComponent implements ODataConstants {
/**
* These fields are populated using reflection by the HandlerCustomizer class.
*
* The values are resolved then the appropriate setter called, while the original
* key/value pairs are removed from the options map.
*
* Note 1:
* Should a property be secret then its raw value is the property placeholder and
* the resolving process converts it accordingly hence the importance of doing it
* this way rather than using the options map directly.
*
* Note 2:
* methodName not included as not required here but required later in the options map.
*/
private String serviceUri;
private String basicUserName;
private String basicPassword;
private String serverCertificate;
private String keyPredicate;
private String queryParams;
private boolean filterAlreadySeen;
private String connectorDirection = "from";
// Consumer properties
private long delay = -1;
private long initialDelay = -1;
private int backoffIdleThreshold = -1;
private int backoffMultiplier = -1;
private boolean splitResult;
ODataComponent(String componentId, String componentScheme) {
super(componentId, componentScheme);
}
public String getServiceUri() {
return serviceUri;
}
public void setServiceUri(String serviceUri) {
this.serviceUri = ODataUtil.removeEndSlashes(serviceUri);
}
public String getBasicUserName() {
return basicUserName;
}
public void setBasicUserName(String basicUserName) {
this.basicUserName = basicUserName;
}
public String getBasicPassword() {
return basicPassword;
}
public void setBasicPassword(String basicPassword) {
this.basicPassword = basicPassword;
}
public String getServerCertificate() {
return serverCertificate;
}
public void setServerCertificate(String serverCertificate) {
this.serverCertificate = serverCertificate;
}
public String getKeyPredicate() {
return keyPredicate;
}
public void setKeyPredicate(String keyPredicate) {
this.keyPredicate = keyPredicate;
}
public String getQueryParams() {
return queryParams;
}
public void setQueryParams(String queryParams) {
this.queryParams = queryParams;
}
@SuppressWarnings("PMD")
public boolean isFilterAlreadySeen() {
return filterAlreadySeen ;
}
public void setFilterAlreadySeen(boolean filterAlreadySeen) {
this.filterAlreadySeen = filterAlreadySeen;
}
public long getDelay() {
return delay;
}
public void setDelay(long delay) {
this.delay = delay;
}
public long getInitialDelay() {
return initialDelay;
}
public void setInitialDelay(long initialDelay) {
this.initialDelay = initialDelay;
}
public int getBackoffIdleThreshold() {
return backoffIdleThreshold;
}
public void setBackoffIdleThreshold(int backoffIdleThreshold) {
this.backoffIdleThreshold = backoffIdleThreshold;
}
public int getBackoffMultiplier() {
return backoffMultiplier;
}
public void setBackoffMultiplier(int backoffMultiplier) {
this.backoffMultiplier = backoffMultiplier;
}
public boolean isSplitResult() {
return splitResult;
}
public void setSplitResult(boolean splitResult) {
this.splitResult = splitResult;
}
public String getConnectorDirection() {
return connectorDirection;
}
public void setConnectorDirection(String connectorDirection) {
this.connectorDirection = connectorDirection;
}
private Map bundleOptions(Map options) {
PropertyBuilder
© 2015 - 2024 Weber Informatics LLC | Privacy Policy