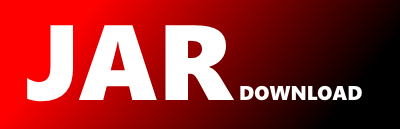
io.syndesis.project.converter.visitor.ImmutableStepVisitorContext Maven / Gradle / Ivy
/*
* Copyright (C) 2016 Red Hat, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.syndesis.project.converter.visitor;
import com.fasterxml.jackson.annotation.JsonProperty;
import edu.umd.cs.findbugs.annotations.SuppressFBWarnings;
import io.syndesis.model.integration.Step;
import java.util.Objects;
import java.util.Queue;
import java.util.Set;
import javax.annotation.Generated;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import javax.annotation.concurrent.NotThreadSafe;
import javax.validation.ConstraintViolation;
import javax.validation.ConstraintViolationException;
import javax.validation.Validation;
import javax.validation.Validator;
/**
* Immutable implementation of {@link StepVisitorContext}.
*
* Use the builder to create immutable instances:
* {@code new StepVisitorContext.Builder()}.
* Use the static factory method to create immutable instances:
* {@code ImmutableStepVisitorContext.of()}.
*/
@SuppressWarnings({"all"})
@SuppressFBWarnings
@ParametersAreNonnullByDefault
@Generated({"Immutables.generator", "StepVisitorContext"})
@Immutable
final class ImmutableStepVisitorContext
implements StepVisitorContext {
private final GeneratorContext generatorContext;
private final int index;
private final Step step;
private final Queue remaining;
private ImmutableStepVisitorContext(
GeneratorContext generatorContext,
int index,
Step step,
Queue remaining) {
this.generatorContext = generatorContext;
this.index = index;
this.step = step;
this.remaining = remaining;
}
/**
* @return The value of the {@code generatorContext} attribute
*/
@JsonProperty("generatorContext")
@Override
public GeneratorContext getGeneratorContext() {
return generatorContext;
}
/**
* @return The value of the {@code index} attribute
*/
@JsonProperty("index")
@Override
public int getIndex() {
return index;
}
/**
* @return The value of the {@code step} attribute
*/
@JsonProperty("step")
@Override
public Step getStep() {
return step;
}
/**
* @return The value of the {@code remaining} attribute
*/
@JsonProperty("remaining")
@Override
public Queue getRemaining() {
return remaining;
}
/**
* Copy the current immutable object by setting a value for the {@link StepVisitorContext#getGeneratorContext() generatorContext} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for generatorContext (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableStepVisitorContext withGeneratorContext(GeneratorContext value) {
if (this.generatorContext == value) return this;
return validate(new ImmutableStepVisitorContext(value, this.index, this.step, this.remaining));
}
/**
* Copy the current immutable object by setting a value for the {@link StepVisitorContext#getIndex() index} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for index
* @return A modified copy of the {@code this} object
*/
public final ImmutableStepVisitorContext withIndex(int value) {
if (this.index == value) return this;
return validate(new ImmutableStepVisitorContext(this.generatorContext, value, this.step, this.remaining));
}
/**
* Copy the current immutable object by setting a value for the {@link StepVisitorContext#getStep() step} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for step (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableStepVisitorContext withStep(Step value) {
if (this.step == value) return this;
return validate(new ImmutableStepVisitorContext(this.generatorContext, this.index, value, this.remaining));
}
/**
* Copy the current immutable object by setting a value for the {@link StepVisitorContext#getRemaining() remaining} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for remaining (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableStepVisitorContext withRemaining(Queue value) {
if (this.remaining == value) return this;
return validate(new ImmutableStepVisitorContext(this.generatorContext, this.index, this.step, value));
}
/**
* This instance is equal to all instances of {@code ImmutableStepVisitorContext} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof ImmutableStepVisitorContext
&& equalTo((ImmutableStepVisitorContext) another);
}
private boolean equalTo(ImmutableStepVisitorContext another) {
return Objects.equals(generatorContext, another.generatorContext)
&& index == another.index
&& Objects.equals(step, another.step)
&& Objects.equals(remaining, another.remaining);
}
/**
* Computes a hash code from attributes: {@code generatorContext}, {@code index}, {@code step}, {@code remaining}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 5381;
h += (h << 5) + Objects.hashCode(generatorContext);
h += (h << 5) + index;
h += (h << 5) + Objects.hashCode(step);
h += (h << 5) + Objects.hashCode(remaining);
return h;
}
/**
* Prints the immutable value {@code StepVisitorContext} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return "StepVisitorContext{"
+ "generatorContext=" + generatorContext
+ ", index=" + index
+ ", step=" + step
+ ", remaining=" + remaining
+ "}";
}
/**
* Construct a new immutable {@code StepVisitorContext} instance.
* @param generatorContext The value for the {@code generatorContext} attribute
* @param index The value for the {@code index} attribute
* @param step The value for the {@code step} attribute
* @param remaining The value for the {@code remaining} attribute
* @return An immutable StepVisitorContext instance
*/
public static StepVisitorContext of(GeneratorContext generatorContext, int index, Step step, Queue remaining) {
return validate(new ImmutableStepVisitorContext(generatorContext, index, step, remaining));
}
private static final Validator validator = Validation.buildDefaultValidatorFactory().getValidator();
private static ImmutableStepVisitorContext validate(ImmutableStepVisitorContext instance) {
Set> constraintViolations = validator.validate(instance);
if (!constraintViolations.isEmpty()) {
throw new ConstraintViolationException(constraintViolations);
}
return instance;
}
/**
* Creates an immutable copy of a {@link StepVisitorContext} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable StepVisitorContext instance
*/
public static StepVisitorContext copyOf(StepVisitorContext instance) {
if (instance instanceof ImmutableStepVisitorContext) {
return (ImmutableStepVisitorContext) instance;
}
return new StepVisitorContext.Builder()
.createFrom(instance)
.build();
}
/**
* Builds instances of type {@link StepVisitorContext StepVisitorContext}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@NotThreadSafe
public static class Builder {
private @Nullable GeneratorContext generatorContext;
private int index;
private @Nullable Step step;
private @Nullable Queue remaining;
/**
* Creates a builder for {@link StepVisitorContext StepVisitorContext} instances.
*/
public Builder() {
if (!(this instanceof StepVisitorContext.Builder)) {
throw new UnsupportedOperationException("Use: new StepVisitorContext.Builder()");
}
}
/**
* Fill a builder with attribute values from the provided {@code StepVisitorContext} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final StepVisitorContext.Builder createFrom(StepVisitorContext instance) {
Objects.requireNonNull(instance, "instance");
GeneratorContext generatorContextValue = instance.getGeneratorContext();
if (generatorContextValue != null) {
generatorContext(generatorContextValue);
}
index(instance.getIndex());
Step stepValue = instance.getStep();
if (stepValue != null) {
step(stepValue);
}
Queue remainingValue = instance.getRemaining();
if (remainingValue != null) {
remaining(remainingValue);
}
return (StepVisitorContext.Builder) this;
}
/**
* Initializes the value for the {@link StepVisitorContext#getGeneratorContext() generatorContext} attribute.
* @param generatorContext The value for generatorContext (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("generatorContext")
public final StepVisitorContext.Builder generatorContext(GeneratorContext generatorContext) {
this.generatorContext = generatorContext;
return (StepVisitorContext.Builder) this;
}
/**
* Initializes the value for the {@link StepVisitorContext#getIndex() index} attribute.
* @param index The value for index
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("index")
public final StepVisitorContext.Builder index(int index) {
this.index = index;
return (StepVisitorContext.Builder) this;
}
/**
* Initializes the value for the {@link StepVisitorContext#getStep() step} attribute.
* @param step The value for step (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("step")
public final StepVisitorContext.Builder step(Step step) {
this.step = step;
return (StepVisitorContext.Builder) this;
}
/**
* Initializes the value for the {@link StepVisitorContext#getRemaining() remaining} attribute.
* @param remaining The value for remaining (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("remaining")
public final StepVisitorContext.Builder remaining(Queue remaining) {
this.remaining = remaining;
return (StepVisitorContext.Builder) this;
}
/**
* Builds a new {@link StepVisitorContext StepVisitorContext}.
* @return An immutable instance of StepVisitorContext
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public StepVisitorContext build() {
return ImmutableStepVisitorContext.validate(new ImmutableStepVisitorContext(generatorContext, index, step, remaining));
}
}
}