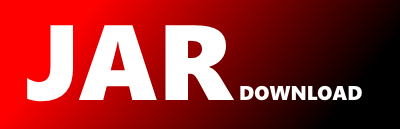
io.takari.builder.internal.model.SimpleParameter Maven / Gradle / Ivy
package io.takari.builder.internal.model;
import java.net.MalformedURLException;
import java.net.URI;
import java.net.URISyntaxException;
import java.net.URL;
import java.util.Collections;
import java.util.LinkedHashMap;
import java.util.Map;
import java.util.function.Function;
import io.takari.builder.Parameter;
public class SimpleParameter extends AbstractParameter {
private static final String[] EMPTY = {};
private final Parameter annotation;
SimpleParameter(MemberAdapter element, TypeAdapter type) {
super(element, type);
this.annotation = element.getAnnotation(Parameter.class);
}
@Override
public Parameter annotation() {
return annotation;
}
@Override
public boolean required() {
return annotation != null ? annotation.required() : false;
}
public String[] value() {
return annotation != null ? annotation.value() : EMPTY;
}
public String[] defaultValue() {
return annotation != null ? annotation.defaultValue() : EMPTY;
}
@Override
public void accept(BuilderMetadataVisitor visitor) {
visitor.visitSimple(this);
}
//
//
//
static final Map> converters;
static {
Map> _converters = new LinkedHashMap<>();
_converters.put(String.class.getCanonicalName(), s -> s);
_converters.put(Boolean.class.getCanonicalName(), Boolean::valueOf);
_converters.put(boolean.class.getCanonicalName(), Boolean::parseBoolean);
_converters.put(int.class.getCanonicalName(), Integer::parseInt);
_converters.put(Integer.class.getCanonicalName(), Integer::parseInt);
_converters.put(long.class.getCanonicalName(), Long::parseLong);
_converters.put(Long.class.getCanonicalName(), Long::parseLong);
_converters.put(URL.class.getCanonicalName(), s -> {
try {
return new URL(s);
} catch (MalformedURLException e) {
throw new IllegalArgumentException(e);
}
});
_converters.put(URI.class.getCanonicalName(), s -> {
try {
return new URI(s);
} catch (URISyntaxException e) {
throw new IllegalArgumentException(e);
}
});
converters = Collections.unmodifiableMap(_converters);
}
public static boolean isSimpleType(TypeAdapter type) {
return converters.containsKey(type.qualifiedName());
}
public static Function getConverter(TypeAdapter type) {
Function converter = converters.get(type.qualifiedName());
assert converter != null;
return converter;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy