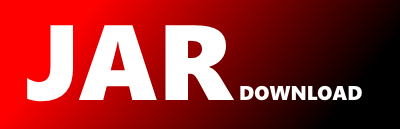
io.takari.maven.plugin.testing.ProjectDump Maven / Gradle / Ivy
The newest version!
package io.takari.maven.plugin.testing;
/*
* Copyright 2001-2005 The Apache Software Foundation.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.OutputStreamWriter;
import java.io.Writer;
import java.util.List;
import org.apache.maven.artifact.DependencyResolutionRequiredException;
import org.apache.maven.execution.MavenSession;
import org.apache.maven.plugin.AbstractMojo;
import org.apache.maven.plugin.MojoExecutionException;
import org.apache.maven.plugins.annotations.LifecyclePhase;
import org.apache.maven.plugins.annotations.Mojo;
import org.apache.maven.plugins.annotations.Parameter;
import org.apache.maven.plugins.annotations.ResolutionScope;
import org.apache.maven.project.MavenProject;
import com.google.common.collect.Lists;
import com.google.common.io.Closer;
@Mojo(name = "project-dump", defaultPhase = LifecyclePhase.COMPILE, requiresDependencyResolution = ResolutionScope.COMPILE, requiresProject = true)
public class ProjectDump extends AbstractMojo {
@Parameter(defaultValue = "${project.build.directory}/project.json")
private File projectJson;
@Parameter(defaultValue = "${project}")
private MavenProject project;
@Parameter(defaultValue = "${session}")
private MavenSession session;
@Override
public void execute() throws MojoExecutionException {
try {
dumpProject(session);
} catch (IOException e) {
throw new MojoExecutionException("Error dumping Maven project", e);
} catch (DependencyResolutionRequiredException e) {
throw new MojoExecutionException("Error dumping Maven project", e);
}
}
public void dumpProject(MavenSession session) throws IOException, DependencyResolutionRequiredException {
if (!projectJson.getParentFile().exists()) {
projectJson.getParentFile().mkdirs();
}
Closer closer = Closer.create();
try {
BlackboxMavenProjectSerializer serializer = new BlackboxMavenProjectSerializer();
Writer writer = closer.register(new OutputStreamWriter(new FileOutputStream(projectJson), "UTF-8"));
serializer.serialize(blackboxMavenProject(project, session), writer);
} finally {
closer.close();
}
}
private BlackboxMavenProject blackboxMavenProject(MavenProject project, MavenSession session) throws DependencyResolutionRequiredException {
List buildspaceProjects = Lists.newArrayList();
for(MavenProject p : session.getProjects()) {
buildspaceProjects.add(p.getArtifactId());
}
List workspaceProjects = Lists.newArrayList();
/*
for(MavenProject p : session.getWorkspaceProjects()) {
workspaceProjects.add(p.getArtifactId());
}
*/
List compileClasspathElements = Lists.newArrayList();
for (String classpathElement : project.getCompileClasspathElements()) {
String basePath = project.getBasedir().getParentFile().getAbsolutePath();
if(classpathElement.startsWith(basePath)) {
String relativePath = classpathElement.substring(basePath.length()+1);
compileClasspathElements.add(0, relativePath);
} else {
compileClasspathElements.add(classpathElement);
}
}
return new BlackboxMavenProject(project.getArtifactId(), buildspaceProjects, workspaceProjects, compileClasspathElements);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy