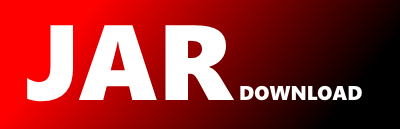
io.tarantool.driver.core.proxy.TruncateProxyOperation Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cartridge-driver Show documentation
Show all versions of cartridge-driver Show documentation
Tarantool Cartridge driver for Tarantool versions 1.10+ based on Netty framework
package io.tarantool.driver.core.proxy;
import io.tarantool.driver.api.TarantoolCallOperations;
import io.tarantool.driver.api.TarantoolVoidResult;
import io.tarantool.driver.api.space.options.OperationWithTimeoutOptions;
import java.util.Arrays;
import java.util.List;
import java.util.concurrent.CompletableFuture;
/**
* Proxy operation for truncate
*
* @author Ivan Dneprov
* @author Artyom Dubinin
*/
public final class TruncateProxyOperation implements ProxyOperation {
private final TarantoolCallOperations client;
private final String functionName;
private final List> arguments;
private TruncateProxyOperation(
TarantoolCallOperations client,
String functionName,
List> arguments) {
this.client = client;
this.arguments = arguments;
this.functionName = functionName;
}
public TarantoolCallOperations getClient() {
return client;
}
public String getFunctionName() {
return functionName;
}
public List> getArguments() {
return arguments;
}
@Override
public CompletableFuture execute() {
return client.callForSingleResult(functionName, arguments, Boolean.class)
.thenApply(v -> TarantoolVoidResult.INSTANCE.value());
}
/**
* Create a builder instance.
*
* @return a builder
*/
public static Builder builder() {
return new Builder();
}
public static final class Builder
extends AbstractProxyOperation.GenericOperationsBuilder {
public Builder() {
}
@Override
Builder self() {
return this;
}
/**
* Prepare request of truncate operation to Tarantool server
*
* @return TruncateProxyOperation instance
*/
public TruncateProxyOperation build() {
CRUDBaseOptions requestOptions = new CRUDBaseOptions.Builder()
.withTimeout(options.getTimeout())
.build();
List> arguments = Arrays.asList(spaceName, requestOptions.asMap());
return new TruncateProxyOperation(this.client, this.functionName, arguments);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy