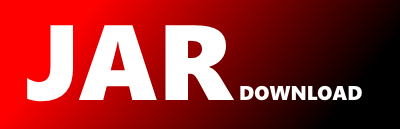
io.tarantool.driver.api.MessagePackMapperBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cartridge-driver Show documentation
Show all versions of cartridge-driver Show documentation
Tarantool Cartridge driver for Tarantool versions 1.10+ based on Netty framework
package io.tarantool.driver.api;
import io.tarantool.driver.mappers.DefaultMessagePackMapper;
import io.tarantool.driver.mappers.MessagePackMapper;
import io.tarantool.driver.mappers.converters.ObjectConverter;
import io.tarantool.driver.mappers.converters.ValueConverter;
import org.msgpack.value.Value;
import org.msgpack.value.ValueType;
import java.util.List;
import java.util.Map;
/**
* Builder for {@link MessagePackMapper}
*/
public interface MessagePackMapperBuilder {
/**
* Configure the mapper with default {@code MP_MAP} entity to {@link Map} converter
*
* @return builder
*/
MessagePackMapperBuilder withDefaultMapValueConverter();
/**
* Configure the mapper with default {@link Map} to {@code MP_MAP} entity converter
*
* @return builder
*/
MessagePackMapperBuilder withDefaultMapObjectConverter();
/**
* Configure the mapper with default {@code MP_ARRAY} entity to {@link List} converter
*
* @return builder
*/
MessagePackMapperBuilder withDefaultArrayValueConverter();
/**
* Configure the mapper with default {@link List} to {@code MP_ARRAY} entity converter
*
* @return builder
*/
MessagePackMapperBuilder withDefaultListObjectConverter();
/**
* Configure the mapper with a specified MessagePack entity-to-object converter
*
* @param valueType MessagePack source type
* @param converter MessagePack entity-to-object and object-to-entity converter
* @param MessagePack's entity type that the converter accepts and/or returns
* @param java object's type that the converter accepts and/or returns
* @return builder
* @see io.tarantool.driver.mappers.DefaultMessagePackMapper#registerValueConverter(ValueType, ValueConverter)
*/
MessagePackMapperBuilder
withValueConverter(ValueType valueType, ValueConverter converter);
/**
* Configure the mapper with a specified MessagePack entity-to-object converter
*
* @param valueType MessagePack source type
* @param objectClass target object class
* @param converter MessagePack entity-to-object and object-to-entity converter
* @param MessagePack's entity type that the converter accepts and/or returns
* @param java object's type that the converter accepts and/or returns
* @return builder
* @see DefaultMessagePackMapper#registerValueConverter(ValueType, Class, ValueConverter)
*/
MessagePackMapperBuilder withValueConverter(
ValueType valueType,
Class objectClass,
ValueConverter converter);
/**
* Configure the mapper with a specified MessagePack object-to-entity converter
*
* @param converter MessagePack entity-to-object and object-to-entity converter
* @param MessagePack's entity type that the converter accepts and/or returns
* @param java object's type that the converter accepts and/or returns
* @return builder
*/
MessagePackMapperBuilder withObjectConverter(ObjectConverter converter);
/**
* Configure the mapper with a specified MessagePack object-to-entity converter
*
* @param objectClass source object class
* @param converter MessagePack entity-to-object and object-to-entity converter
* @param MessagePack's entity type that the converter accepts and/or returns
* @param java object's type that the converter accepts and/or returns
* @return builder
*/
MessagePackMapperBuilder withObjectConverter(
Class objectClass,
ObjectConverter converter);
/**
* Configure the mapper with a specified MessagePack object-to-entity converter
*
* @param objectClass source object class
* @param valueClass target object class
* @param converter MessagePack entity-to-object and object-to-entity converter
* @param MessagePack entity type
* @param object type
* @return builder
*/
MessagePackMapperBuilder withObjectConverter(
Class objectClass, Class valueClass,
ObjectConverter converter);
/**
* Build the mapper instance
*
* @return a new mapper instance
*/
MessagePackMapper build();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy