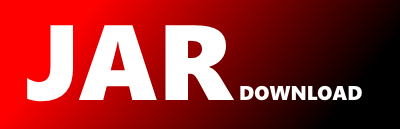
io.tarantool.driver.mappers.MessagePackValueMapper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cartridge-driver Show documentation
Show all versions of cartridge-driver Show documentation
Tarantool Cartridge driver for Tarantool versions 1.10+ based on Netty framework
package io.tarantool.driver.mappers;
import io.tarantool.driver.mappers.converters.ValueConverter;
import org.msgpack.value.Value;
import org.msgpack.value.ValueType;
import java.util.Optional;
/**
* Basic interface for collection of generic converters between MessagePack entities and Java objects.
* Value converters must be added using the {@link #registerValueConverter(ValueType, Class, ValueConverter)} method
*
* @author Alexey Kuzin
* @author Artyom Dubinin
*/
public interface MessagePackValueMapper {
/**
* Create Java object out of its MessagePack representation.
*
* @param v MessagePack entity
* @param source MessagePack entity type
* @param target object type
* @return Java object
* @throws MessagePackValueMapperException if the corresponding conversion cannot be performed
*/
O fromValue(V v) throws MessagePackValueMapperException;
/**
* Create Java object out of its MessagePack representation. Converters will be checked to match the target
* object type.
*
* @param v MessagePack entity
* @param targetClass Java object class
* @param source MessagePack entity type
* @param target object type
* @return Java object
* @throws MessagePackValueMapperException if the corresponding conversion cannot be performed
*/
O fromValue(V v, Class targetClass) throws MessagePackValueMapperException;
/**
* Adds a MessagePack entity converter to this mappers instance.
*
* @param valueType MessagePack source type
* @param objectClass target object class
* @param converter object-to-entity converter
* @param MessagePack's entity type that the converter accepts and/or returns
* @param java object's type that the converter accepts and/or returns
* @see ValueConverter
*/
void registerValueConverter(
ValueType valueType, Class extends O> objectClass,
ValueConverter converter);
void registerValueConverterWithoutTargetClass(
ValueType valueType, ValueConverter converter);
/**
* Get a converter capable of converting from the source entity class to the target class
*
* @param valueType MessagePack source type
* @param objectClass the target conversion class
* @param MessagePack's entity type that the converter accepts and/or returns
* @param java object's type that the converter accepts and/or returns
* @return a nullable converter instance wrapped in {@code Optional}
*/
Optional> getValueConverter(ValueType valueType, Class objectClass);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy