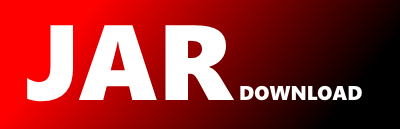
io.tarantool.driver.mappers.factories.ResultMapperFactoryFactory Maven / Gradle / Ivy
Show all versions of cartridge-driver Show documentation
package io.tarantool.driver.mappers.factories;
import io.tarantool.driver.api.MultiValueCallResult;
import io.tarantool.driver.api.SingleValueCallResult;
import io.tarantool.driver.api.TarantoolResult;
import io.tarantool.driver.api.tuple.TarantoolTuple;
import io.tarantool.driver.mappers.TarantoolTupleResultMapperFactory;
import java.util.List;
/**
* Provides different factories for creating result mappers
*
* @author Alexey Kuzin
* @author Artyom Dubinin
*/
public interface ResultMapperFactoryFactory {
/**
* Default factory for call result with a list of tuples.
* Use this factory for handling containers with {@link TarantoolTuple} inside.
* The IProto method results by default contain lists of tuples.
* This factory can be used at the inner level by other mapper factories that are handling higher-level containers.
*
* input: array of tuples with MessagePack values inside ([t1, t2, ...])
*
* mapper result: {@code TarantoolResult}
*
* Mapper result and its inner depends on the parameters or converters you passed.
*
* @return default factory for list of tuples results
*/
ArrayValueToTarantoolTupleResultMapperFactory arrayTupleResultMapperFactory();
/**
* Default factory for call result with different structures.
* Use this factory for handling containers with {@link TarantoolTuple} inside.
* The IProto method results by default contain lists of tuples.
* The crud results by default contain map with list of tuple inside.
* This factory can be used at the inner level by other mapper factories that are handling higher-level containers.
*
* input: structure with array of tuples with MessagePack values inside ([t1, t2, ...])
*
* mapper result: {@code TarantoolResult}
*
*
* @return default factory for list of tuples results
*/
TarantoolTupleResultMapperFactory getTarantoolTupleResultMapperFactory();
/**
* Default factory for call result with a list of tuples in structure with metadata.
* Use this factory for handling containers with {@link TarantoolTuple} inside.
* The IProto method results by default contain lists of tuples.
* This factory can be used at the inner level by other mapper factories that are handling higher-level containers.
*
* input: map with metadata and array of tuples with MessagePack values inside ([t1, t2, ...])
*
* mapper result: {@code TarantoolResult}
*
* Mapper result and its inner depends on the parameters or converters you passed.
*
* @return default factory for list of tuples results
*/
RowsMetadataToTarantoolTupleResultMapperFactory rowsMetadataTupleResultMapperFactory();
/**
* Default factory for single the stored Lua function call result in the form return result, err
* with a list of tuples as a result.
* For example, this form of the result is used for some tarantool/crud library responses.
*
* input: [x, y, ...], MessagePack array from a Lua function multi-return response
*
* where x
is a data structure with an array of tuples inside ([t1, t2, ...]) and y
* can be interpreted as an error structure if it is not empty and there are no more arguments after
* y
`
*
* mapper result: converted value of x
to {@code TarantoolResult}
*
* Mapper result and the inner contents depend on the parameters or the passed converters.
*
* @return default factory for single value call result with a list of tuples
*/
SingleValueWithTarantoolTupleResultMapperFactory singleValueTupleResultMapperFactory();
/**
* Create a factory for mapping stored function call result to {@link SingleValueCallResult} containing a list
* of tuples mapped to {@link TarantoolResult}
*
* input: [x, y, z], MessagePack array from a Lua function multi return response
*
* where x
is a data structure with an array of tuples inside ([t1, t2, ...]) and y
* can be interpreted as an error structure if it is not empty and there are no more arguments after y
.
* A tuple can be represented by any custom object or value.
*
* mapper result: converted value of x
to {@code TarantoolResult}
*
* Mapper result and its inner contents depend on the parameters or the passed converters.
*
* @param target inner content type
* @return new or existing factory instance
*/
SingleValueWithTarantoolResultMapperFactory singleValueTarantoolResultMapperFactory();
/**
* Create a factory for mapping stored Lua function call results to {@link SingleValueCallResult}
*
* input: [x, y, ...], MessagePack array from a Lua function multi-return response
*
* where x, y
are some MessagePack values. x
is interpreted as a stored Lua function
* result and y
may be interpreted as a stored Lua function error if it is not empty and there are
* no more arguments after y
.
*
* mapper result: some Java type converted from the value of x
according to the mapper, passed as a
* parameter to this one for parsing the result contents.
*
* Mapper result and its inner contents depend on the parameters or the passed converters.
*
* @param target result type
* @return new or existing factory instance
*/
SingleValueResultMapperFactory singleValueResultMapperFactory();
/**
* Default factory for the stored Lua function call result, interpreted in a way that each returned item is a tuple.
* Use this factory for handling proxy function call results which return tuples as a multi-return result.
*
* input: [x, y, z, ...], MessagePack array from a Lua function multi-return response
*
* where x, y, z
are some MessagePack values, each one representing a Tarantool tuple
*
* mapper result: {@code TarantoolResult} converted from [x, y, z, ...]
*
* Mapper result and its inner contents depend on the parameters or the passed converters.
*
* @return new or existing factory instance
*/
MultiValueWithTarantoolTupleResultMapperFactory multiValueTupleResultMapperFactory();
/**
* Default factory for the mapping stored Lua function call multi-return result to {@link TarantoolResult}, where
* each value is interpreted as a tuple of custom type.
*
* input: [x, y, z, ...], MessagePack array from a Lua function multi-return response
*
* where x, y, z
are some MessagePack values, each one representing a custom value
*
* mapper result: {@code TarantoolResult} converted from [x, y, z, ...]
*
* Mapper result and its inner contents depend on the parameters or the passed converters.
* Mapper checks errors and that result is not empty and uses
* {@link ArrayValueToTarantoolResultMapperFactory} for further parsing
*
* @param target inner content type
* @return new or existing factory instance
*/
MultiValueWithTarantoolResultMapperFactory multiValueTarantoolResultMapperFactory();
/**
* Create a factory for mapping stored Lua function multi-return call result to {@link MultiValueCallResult} with
* the custom result and tuple types.
*
* input: [x, y, z, ...], MessagePack array from a Lua function multi-return response that is converted to the
* target result type
*
* where x, y, z
are some MessagePack values, each one representing a custom value
*
* mapper result: a custom Java type inheriting {@code List} that is converted from [x, y, z, ...]
*
* Mapper result and its inner contents depend on the parameters or the passed converters.
*
* @param target result type
* @param target result inner contents item type
* @return new or existing factory instance
*/
> MultiValueResultMapperFactory multiValueResultMapperFactory();
/**
* Create a factory for mapping stored function call result to {@link MultiValueCallResult} containing a list
* of tuples mapped to {@link TarantoolResult}
*
* input: storing structure(e.g. [x, y, z] messagepack array)
*
* where x, y, z are some MessagePack Values
*
* mapper result: converted {@code TarantoolResult} from [x, y, z]
*
* Mapper result and its inner contents depend on the parameters or the passed converters.
* For example, you can parse any MessagePack array to TarantoolResult even if it's an empty array.
*
* @param target inner result type
* @return new or existing factory instance
*/
ArrayValueToTarantoolResultMapperFactory rowsMetadataStructureResultMapperFactory();
}