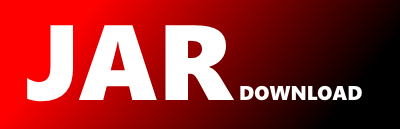
io.tarantool.driver.api.retry.RequestTimeoutOperation Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cartridge-driver Show documentation
Show all versions of cartridge-driver Show documentation
Tarantool Cartridge driver for Tarantool versions 1.10+ based on Netty framework
package io.tarantool.driver.api.retry;
import java.util.concurrent.CompletableFuture;
import java.util.concurrent.ScheduledFuture;
import java.util.concurrent.TimeUnit;
import java.util.concurrent.TimeoutException;
import java.util.function.Supplier;
/**
* Supplier of scheduled request timeout tasks.
* These tasks are never completed successfully, but only with a timeout exception.
*
* @author Artyom Dubinin
*/
public class RequestTimeoutOperation implements Supplier> {
private final CompletableFuture resultFuture;
private final long requestTimeout;
public RequestTimeoutOperation(CompletableFuture resultFuture, long requestTimeout) {
this.resultFuture = resultFuture;
this.requestTimeout = requestTimeout;
}
@Override
public CompletableFuture get() {
final CompletableFuture future = new CompletableFuture<>();
ScheduledFuture scheduledFuture = TarantoolRequestRetryPolicies.getTimeoutScheduler().schedule(() -> {
final TimeoutException ex = new TimeoutException("Request timeout after " + requestTimeout + " ms");
return future.completeExceptionally(ex);
}, requestTimeout, TimeUnit.MILLISECONDS);
// optimization: stop timeout future if resultFuture is already completed externally
resultFuture.whenComplete((res, ex) -> scheduledFuture.cancel(false));
return future;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy