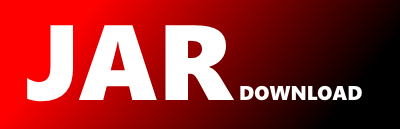
io.tarantool.driver.core.proxy.CRUDAbstractOperationOptions Maven / Gradle / Ivy
Show all versions of cartridge-driver Show documentation
package io.tarantool.driver.core.proxy;
import java.util.HashMap;
import java.util.Map;
import java.util.Optional;
/**
* This class is not part of the public API.
*
* An abstract class necessary for implementing CRT (curiously recurring template)
* pattern for the cluster proxy operation options builders.
*
* @author Alexey Kuzin
*/
abstract class CRUDAbstractOperationOptions {
private final Map resultMap = new HashMap<>();
/**
* Inheritable Builder for cluster proxy operation options.
*
* This abstract class is necessary for implementing fluent builder inheritance.
* The solution with {@code self()} method allows to avoid weird java
* compiler errors when you cannot call the inherited methods from derived
* concrete {@code Builder} classes because their type erasure doesn't
* correspond to the {@code AbstractBuilder} type.
*
* The {@code self()} method must be implemented only in the derived concrete
* classes. These concrete classes are used to work with the operation
* options in the calling code. They doesn't require to specify the generic
* types and therefore are more convenient. Also they ensure that the right
* combination of generic types will be used to avoid potential inheritance
* flaws.
*/
protected abstract static
class AbstractBuilder> {
abstract B self();
public abstract O build();
}
protected void addOption(String option, Optional> value) {
if (value.isPresent()) {
resultMap.put(option, value.get());
}
}
/**
* Return serializable options representation.
*
* @return a map
*/
public Map asMap() {
return resultMap;
}
}