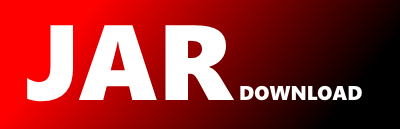
io.tekniq.jdbc.TqSingleConnectionDataSource.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tekniq-jdbc Show documentation
Show all versions of tekniq-jdbc Show documentation
A framework designed around Kotlin
The newest version!
package io.tekniq.jdbc
import java.io.PrintWriter
import java.sql.Connection
import java.sql.DriverManager
import java.sql.SQLException
import java.util.logging.Logger
import javax.sql.DataSource
/**
* Used mostly for testing purposes. Cannot imagine a real use case for a single connection data source otherwise.
* True datasource libraries like hikari or vibur-dbcp should be used instead.
* @author Sejal Patel
*/
@Suppress("unused")
open class TqSingleConnectionDataSource(
url: String,
username: String? = null,
password: String? = null,
autoCommit: Boolean = true
) : DataSource {
private val connection: Connection = DriverManager.getConnection(url, username, password)
.also { it.autoCommit = autoCommit }
fun close() {
connection.close()
}
override fun setLogWriter(out: PrintWriter?) {
throw UnsupportedOperationException("setLogWriter(PrintWriter)")
}
override fun setLoginTimeout(seconds: Int) {
throw UnsupportedOperationException("setLoginTimeout(Int)")
}
override fun getParentLogger(): Logger {
throw UnsupportedOperationException("getParentLogger()")
}
override fun getLogWriter(): PrintWriter {
throw UnsupportedOperationException("getLogWriter()")
}
override fun getLoginTimeout(): Int = 0
override fun isWrapperFor(iface: Class<*>?): Boolean = iface!!.isInstance(this)
override fun unwrap(iface: Class?): T {
if (iface!!.isInstance(this)) {
@Suppress("UNCHECKED_CAST")
return this as T
}
throw SQLException("Connection cannot be unwrapped to ${iface.name}")
}
override fun getConnection(): Connection = UncloseableConnection(connection)
override fun getConnection(username: String?, password: String?): Connection = UncloseableConnection(connection)
private class UncloseableConnection(connection: Connection) : Connection by connection {
override fun close() {
// intentional noop
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy