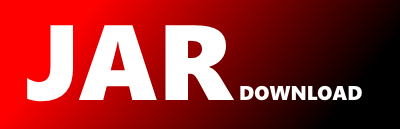
io.temporal.api.command.v1.CommandOrBuilder Maven / Gradle / Ivy
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: temporal/api/command/v1/message.proto
package io.temporal.api.command.v1;
@javax.annotation.Generated(value="protoc", comments="annotations:CommandOrBuilder.java.pb.meta")
public interface CommandOrBuilder extends
// @@protoc_insertion_point(interface_extends:temporal.api.command.v1.Command)
com.google.protobuf.MessageOrBuilder {
/**
* .temporal.api.enums.v1.CommandType command_type = 1;
* @return The enum numeric value on the wire for commandType.
*/
int getCommandTypeValue();
/**
* .temporal.api.enums.v1.CommandType command_type = 1;
* @return The commandType.
*/
io.temporal.api.enums.v1.CommandType getCommandType();
/**
* .temporal.api.command.v1.ScheduleActivityTaskCommandAttributes schedule_activity_task_command_attributes = 2;
* @return Whether the scheduleActivityTaskCommandAttributes field is set.
*/
boolean hasScheduleActivityTaskCommandAttributes();
/**
* .temporal.api.command.v1.ScheduleActivityTaskCommandAttributes schedule_activity_task_command_attributes = 2;
* @return The scheduleActivityTaskCommandAttributes.
*/
io.temporal.api.command.v1.ScheduleActivityTaskCommandAttributes getScheduleActivityTaskCommandAttributes();
/**
* .temporal.api.command.v1.ScheduleActivityTaskCommandAttributes schedule_activity_task_command_attributes = 2;
*/
io.temporal.api.command.v1.ScheduleActivityTaskCommandAttributesOrBuilder getScheduleActivityTaskCommandAttributesOrBuilder();
/**
* .temporal.api.command.v1.StartTimerCommandAttributes start_timer_command_attributes = 3;
* @return Whether the startTimerCommandAttributes field is set.
*/
boolean hasStartTimerCommandAttributes();
/**
* .temporal.api.command.v1.StartTimerCommandAttributes start_timer_command_attributes = 3;
* @return The startTimerCommandAttributes.
*/
io.temporal.api.command.v1.StartTimerCommandAttributes getStartTimerCommandAttributes();
/**
* .temporal.api.command.v1.StartTimerCommandAttributes start_timer_command_attributes = 3;
*/
io.temporal.api.command.v1.StartTimerCommandAttributesOrBuilder getStartTimerCommandAttributesOrBuilder();
/**
* .temporal.api.command.v1.CompleteWorkflowExecutionCommandAttributes complete_workflow_execution_command_attributes = 4;
* @return Whether the completeWorkflowExecutionCommandAttributes field is set.
*/
boolean hasCompleteWorkflowExecutionCommandAttributes();
/**
* .temporal.api.command.v1.CompleteWorkflowExecutionCommandAttributes complete_workflow_execution_command_attributes = 4;
* @return The completeWorkflowExecutionCommandAttributes.
*/
io.temporal.api.command.v1.CompleteWorkflowExecutionCommandAttributes getCompleteWorkflowExecutionCommandAttributes();
/**
* .temporal.api.command.v1.CompleteWorkflowExecutionCommandAttributes complete_workflow_execution_command_attributes = 4;
*/
io.temporal.api.command.v1.CompleteWorkflowExecutionCommandAttributesOrBuilder getCompleteWorkflowExecutionCommandAttributesOrBuilder();
/**
* .temporal.api.command.v1.FailWorkflowExecutionCommandAttributes fail_workflow_execution_command_attributes = 5;
* @return Whether the failWorkflowExecutionCommandAttributes field is set.
*/
boolean hasFailWorkflowExecutionCommandAttributes();
/**
* .temporal.api.command.v1.FailWorkflowExecutionCommandAttributes fail_workflow_execution_command_attributes = 5;
* @return The failWorkflowExecutionCommandAttributes.
*/
io.temporal.api.command.v1.FailWorkflowExecutionCommandAttributes getFailWorkflowExecutionCommandAttributes();
/**
* .temporal.api.command.v1.FailWorkflowExecutionCommandAttributes fail_workflow_execution_command_attributes = 5;
*/
io.temporal.api.command.v1.FailWorkflowExecutionCommandAttributesOrBuilder getFailWorkflowExecutionCommandAttributesOrBuilder();
/**
* .temporal.api.command.v1.RequestCancelActivityTaskCommandAttributes request_cancel_activity_task_command_attributes = 6;
* @return Whether the requestCancelActivityTaskCommandAttributes field is set.
*/
boolean hasRequestCancelActivityTaskCommandAttributes();
/**
* .temporal.api.command.v1.RequestCancelActivityTaskCommandAttributes request_cancel_activity_task_command_attributes = 6;
* @return The requestCancelActivityTaskCommandAttributes.
*/
io.temporal.api.command.v1.RequestCancelActivityTaskCommandAttributes getRequestCancelActivityTaskCommandAttributes();
/**
* .temporal.api.command.v1.RequestCancelActivityTaskCommandAttributes request_cancel_activity_task_command_attributes = 6;
*/
io.temporal.api.command.v1.RequestCancelActivityTaskCommandAttributesOrBuilder getRequestCancelActivityTaskCommandAttributesOrBuilder();
/**
* .temporal.api.command.v1.CancelTimerCommandAttributes cancel_timer_command_attributes = 7;
* @return Whether the cancelTimerCommandAttributes field is set.
*/
boolean hasCancelTimerCommandAttributes();
/**
* .temporal.api.command.v1.CancelTimerCommandAttributes cancel_timer_command_attributes = 7;
* @return The cancelTimerCommandAttributes.
*/
io.temporal.api.command.v1.CancelTimerCommandAttributes getCancelTimerCommandAttributes();
/**
* .temporal.api.command.v1.CancelTimerCommandAttributes cancel_timer_command_attributes = 7;
*/
io.temporal.api.command.v1.CancelTimerCommandAttributesOrBuilder getCancelTimerCommandAttributesOrBuilder();
/**
* .temporal.api.command.v1.CancelWorkflowExecutionCommandAttributes cancel_workflow_execution_command_attributes = 8;
* @return Whether the cancelWorkflowExecutionCommandAttributes field is set.
*/
boolean hasCancelWorkflowExecutionCommandAttributes();
/**
* .temporal.api.command.v1.CancelWorkflowExecutionCommandAttributes cancel_workflow_execution_command_attributes = 8;
* @return The cancelWorkflowExecutionCommandAttributes.
*/
io.temporal.api.command.v1.CancelWorkflowExecutionCommandAttributes getCancelWorkflowExecutionCommandAttributes();
/**
* .temporal.api.command.v1.CancelWorkflowExecutionCommandAttributes cancel_workflow_execution_command_attributes = 8;
*/
io.temporal.api.command.v1.CancelWorkflowExecutionCommandAttributesOrBuilder getCancelWorkflowExecutionCommandAttributesOrBuilder();
/**
* .temporal.api.command.v1.RequestCancelExternalWorkflowExecutionCommandAttributes request_cancel_external_workflow_execution_command_attributes = 9;
* @return Whether the requestCancelExternalWorkflowExecutionCommandAttributes field is set.
*/
boolean hasRequestCancelExternalWorkflowExecutionCommandAttributes();
/**
* .temporal.api.command.v1.RequestCancelExternalWorkflowExecutionCommandAttributes request_cancel_external_workflow_execution_command_attributes = 9;
* @return The requestCancelExternalWorkflowExecutionCommandAttributes.
*/
io.temporal.api.command.v1.RequestCancelExternalWorkflowExecutionCommandAttributes getRequestCancelExternalWorkflowExecutionCommandAttributes();
/**
* .temporal.api.command.v1.RequestCancelExternalWorkflowExecutionCommandAttributes request_cancel_external_workflow_execution_command_attributes = 9;
*/
io.temporal.api.command.v1.RequestCancelExternalWorkflowExecutionCommandAttributesOrBuilder getRequestCancelExternalWorkflowExecutionCommandAttributesOrBuilder();
/**
* .temporal.api.command.v1.RecordMarkerCommandAttributes record_marker_command_attributes = 10;
* @return Whether the recordMarkerCommandAttributes field is set.
*/
boolean hasRecordMarkerCommandAttributes();
/**
* .temporal.api.command.v1.RecordMarkerCommandAttributes record_marker_command_attributes = 10;
* @return The recordMarkerCommandAttributes.
*/
io.temporal.api.command.v1.RecordMarkerCommandAttributes getRecordMarkerCommandAttributes();
/**
* .temporal.api.command.v1.RecordMarkerCommandAttributes record_marker_command_attributes = 10;
*/
io.temporal.api.command.v1.RecordMarkerCommandAttributesOrBuilder getRecordMarkerCommandAttributesOrBuilder();
/**
* .temporal.api.command.v1.ContinueAsNewWorkflowExecutionCommandAttributes continue_as_new_workflow_execution_command_attributes = 11;
* @return Whether the continueAsNewWorkflowExecutionCommandAttributes field is set.
*/
boolean hasContinueAsNewWorkflowExecutionCommandAttributes();
/**
* .temporal.api.command.v1.ContinueAsNewWorkflowExecutionCommandAttributes continue_as_new_workflow_execution_command_attributes = 11;
* @return The continueAsNewWorkflowExecutionCommandAttributes.
*/
io.temporal.api.command.v1.ContinueAsNewWorkflowExecutionCommandAttributes getContinueAsNewWorkflowExecutionCommandAttributes();
/**
* .temporal.api.command.v1.ContinueAsNewWorkflowExecutionCommandAttributes continue_as_new_workflow_execution_command_attributes = 11;
*/
io.temporal.api.command.v1.ContinueAsNewWorkflowExecutionCommandAttributesOrBuilder getContinueAsNewWorkflowExecutionCommandAttributesOrBuilder();
/**
* .temporal.api.command.v1.StartChildWorkflowExecutionCommandAttributes start_child_workflow_execution_command_attributes = 12;
* @return Whether the startChildWorkflowExecutionCommandAttributes field is set.
*/
boolean hasStartChildWorkflowExecutionCommandAttributes();
/**
* .temporal.api.command.v1.StartChildWorkflowExecutionCommandAttributes start_child_workflow_execution_command_attributes = 12;
* @return The startChildWorkflowExecutionCommandAttributes.
*/
io.temporal.api.command.v1.StartChildWorkflowExecutionCommandAttributes getStartChildWorkflowExecutionCommandAttributes();
/**
* .temporal.api.command.v1.StartChildWorkflowExecutionCommandAttributes start_child_workflow_execution_command_attributes = 12;
*/
io.temporal.api.command.v1.StartChildWorkflowExecutionCommandAttributesOrBuilder getStartChildWorkflowExecutionCommandAttributesOrBuilder();
/**
* .temporal.api.command.v1.SignalExternalWorkflowExecutionCommandAttributes signal_external_workflow_execution_command_attributes = 13;
* @return Whether the signalExternalWorkflowExecutionCommandAttributes field is set.
*/
boolean hasSignalExternalWorkflowExecutionCommandAttributes();
/**
* .temporal.api.command.v1.SignalExternalWorkflowExecutionCommandAttributes signal_external_workflow_execution_command_attributes = 13;
* @return The signalExternalWorkflowExecutionCommandAttributes.
*/
io.temporal.api.command.v1.SignalExternalWorkflowExecutionCommandAttributes getSignalExternalWorkflowExecutionCommandAttributes();
/**
* .temporal.api.command.v1.SignalExternalWorkflowExecutionCommandAttributes signal_external_workflow_execution_command_attributes = 13;
*/
io.temporal.api.command.v1.SignalExternalWorkflowExecutionCommandAttributesOrBuilder getSignalExternalWorkflowExecutionCommandAttributesOrBuilder();
/**
* .temporal.api.command.v1.UpsertWorkflowSearchAttributesCommandAttributes upsert_workflow_search_attributes_command_attributes = 14;
* @return Whether the upsertWorkflowSearchAttributesCommandAttributes field is set.
*/
boolean hasUpsertWorkflowSearchAttributesCommandAttributes();
/**
* .temporal.api.command.v1.UpsertWorkflowSearchAttributesCommandAttributes upsert_workflow_search_attributes_command_attributes = 14;
* @return The upsertWorkflowSearchAttributesCommandAttributes.
*/
io.temporal.api.command.v1.UpsertWorkflowSearchAttributesCommandAttributes getUpsertWorkflowSearchAttributesCommandAttributes();
/**
* .temporal.api.command.v1.UpsertWorkflowSearchAttributesCommandAttributes upsert_workflow_search_attributes_command_attributes = 14;
*/
io.temporal.api.command.v1.UpsertWorkflowSearchAttributesCommandAttributesOrBuilder getUpsertWorkflowSearchAttributesCommandAttributesOrBuilder();
/**
* .temporal.api.command.v1.ProtocolMessageCommandAttributes protocol_message_command_attributes = 15;
* @return Whether the protocolMessageCommandAttributes field is set.
*/
boolean hasProtocolMessageCommandAttributes();
/**
* .temporal.api.command.v1.ProtocolMessageCommandAttributes protocol_message_command_attributes = 15;
* @return The protocolMessageCommandAttributes.
*/
io.temporal.api.command.v1.ProtocolMessageCommandAttributes getProtocolMessageCommandAttributes();
/**
* .temporal.api.command.v1.ProtocolMessageCommandAttributes protocol_message_command_attributes = 15;
*/
io.temporal.api.command.v1.ProtocolMessageCommandAttributesOrBuilder getProtocolMessageCommandAttributesOrBuilder();
/**
*
* 16 is available for use - it was used as part of a prototype that never made it into a release
*
*
* .temporal.api.command.v1.ModifyWorkflowPropertiesCommandAttributes modify_workflow_properties_command_attributes = 17;
* @return Whether the modifyWorkflowPropertiesCommandAttributes field is set.
*/
boolean hasModifyWorkflowPropertiesCommandAttributes();
/**
*
* 16 is available for use - it was used as part of a prototype that never made it into a release
*
*
* .temporal.api.command.v1.ModifyWorkflowPropertiesCommandAttributes modify_workflow_properties_command_attributes = 17;
* @return The modifyWorkflowPropertiesCommandAttributes.
*/
io.temporal.api.command.v1.ModifyWorkflowPropertiesCommandAttributes getModifyWorkflowPropertiesCommandAttributes();
/**
*
* 16 is available for use - it was used as part of a prototype that never made it into a release
*
*
* .temporal.api.command.v1.ModifyWorkflowPropertiesCommandAttributes modify_workflow_properties_command_attributes = 17;
*/
io.temporal.api.command.v1.ModifyWorkflowPropertiesCommandAttributesOrBuilder getModifyWorkflowPropertiesCommandAttributesOrBuilder();
public io.temporal.api.command.v1.Command.AttributesCase getAttributesCase();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy