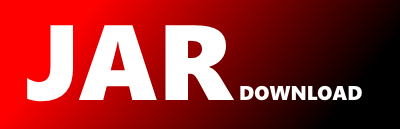
io.temporal.api.command.v1.ScheduleActivityTaskCommandAttributesOrBuilder Maven / Gradle / Ivy
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: temporal/api/command/v1/message.proto
package io.temporal.api.command.v1;
@javax.annotation.Generated(value="protoc", comments="annotations:ScheduleActivityTaskCommandAttributesOrBuilder.java.pb.meta")
public interface ScheduleActivityTaskCommandAttributesOrBuilder extends
// @@protoc_insertion_point(interface_extends:temporal.api.command.v1.ScheduleActivityTaskCommandAttributes)
com.google.protobuf.MessageOrBuilder {
/**
* string activity_id = 1;
* @return The activityId.
*/
java.lang.String getActivityId();
/**
* string activity_id = 1;
* @return The bytes for activityId.
*/
com.google.protobuf.ByteString
getActivityIdBytes();
/**
* .temporal.api.common.v1.ActivityType activity_type = 2;
* @return Whether the activityType field is set.
*/
boolean hasActivityType();
/**
* .temporal.api.common.v1.ActivityType activity_type = 2;
* @return The activityType.
*/
io.temporal.api.common.v1.ActivityType getActivityType();
/**
* .temporal.api.common.v1.ActivityType activity_type = 2;
*/
io.temporal.api.common.v1.ActivityTypeOrBuilder getActivityTypeOrBuilder();
/**
* .temporal.api.taskqueue.v1.TaskQueue task_queue = 4;
* @return Whether the taskQueue field is set.
*/
boolean hasTaskQueue();
/**
* .temporal.api.taskqueue.v1.TaskQueue task_queue = 4;
* @return The taskQueue.
*/
io.temporal.api.taskqueue.v1.TaskQueue getTaskQueue();
/**
* .temporal.api.taskqueue.v1.TaskQueue task_queue = 4;
*/
io.temporal.api.taskqueue.v1.TaskQueueOrBuilder getTaskQueueOrBuilder();
/**
* .temporal.api.common.v1.Header header = 5;
* @return Whether the header field is set.
*/
boolean hasHeader();
/**
* .temporal.api.common.v1.Header header = 5;
* @return The header.
*/
io.temporal.api.common.v1.Header getHeader();
/**
* .temporal.api.common.v1.Header header = 5;
*/
io.temporal.api.common.v1.HeaderOrBuilder getHeaderOrBuilder();
/**
* .temporal.api.common.v1.Payloads input = 6;
* @return Whether the input field is set.
*/
boolean hasInput();
/**
* .temporal.api.common.v1.Payloads input = 6;
* @return The input.
*/
io.temporal.api.common.v1.Payloads getInput();
/**
* .temporal.api.common.v1.Payloads input = 6;
*/
io.temporal.api.common.v1.PayloadsOrBuilder getInputOrBuilder();
/**
*
* Indicates how long the caller is willing to wait for activity completion. The "schedule" time
* is when the activity is initially scheduled, not when the most recent retry is scheduled.
* Limits how long retries will be attempted. Either this or `start_to_close_timeout` must be
* specified. When not specified, defaults to the workflow execution timeout.
* (-- api-linter: core::0140::prepositions=disabled
* aip.dev/not-precedent: "to" is used to indicate interval. --)
*
*
* .google.protobuf.Duration schedule_to_close_timeout = 7 [(.gogoproto.stdduration) = true];
* @return Whether the scheduleToCloseTimeout field is set.
*/
boolean hasScheduleToCloseTimeout();
/**
*
* Indicates how long the caller is willing to wait for activity completion. The "schedule" time
* is when the activity is initially scheduled, not when the most recent retry is scheduled.
* Limits how long retries will be attempted. Either this or `start_to_close_timeout` must be
* specified. When not specified, defaults to the workflow execution timeout.
* (-- api-linter: core::0140::prepositions=disabled
* aip.dev/not-precedent: "to" is used to indicate interval. --)
*
*
* .google.protobuf.Duration schedule_to_close_timeout = 7 [(.gogoproto.stdduration) = true];
* @return The scheduleToCloseTimeout.
*/
com.google.protobuf.Duration getScheduleToCloseTimeout();
/**
*
* Indicates how long the caller is willing to wait for activity completion. The "schedule" time
* is when the activity is initially scheduled, not when the most recent retry is scheduled.
* Limits how long retries will be attempted. Either this or `start_to_close_timeout` must be
* specified. When not specified, defaults to the workflow execution timeout.
* (-- api-linter: core::0140::prepositions=disabled
* aip.dev/not-precedent: "to" is used to indicate interval. --)
*
*
* .google.protobuf.Duration schedule_to_close_timeout = 7 [(.gogoproto.stdduration) = true];
*/
com.google.protobuf.DurationOrBuilder getScheduleToCloseTimeoutOrBuilder();
/**
*
* Limits the time an activity task can stay in a task queue before a worker picks it up. The
* "schedule" time is when the most recent retry is scheduled. This timeout should usually not
* be set: it's useful in specific scenarios like worker-specific task queues. This timeout is
* always non retryable, as all a retry would achieve is to put it back into the same queue.
* Defaults to `schedule_to_close_timeout` or workflow execution timeout if that is not
* specified. More info:
* https://docs.temporal.io/docs/content/what-is-a-schedule-to-start-timeout/
* (-- api-linter: core::0140::prepositions=disabled
* aip.dev/not-precedent: "to" is used to indicate interval. --)
*
*
* .google.protobuf.Duration schedule_to_start_timeout = 8 [(.gogoproto.stdduration) = true];
* @return Whether the scheduleToStartTimeout field is set.
*/
boolean hasScheduleToStartTimeout();
/**
*
* Limits the time an activity task can stay in a task queue before a worker picks it up. The
* "schedule" time is when the most recent retry is scheduled. This timeout should usually not
* be set: it's useful in specific scenarios like worker-specific task queues. This timeout is
* always non retryable, as all a retry would achieve is to put it back into the same queue.
* Defaults to `schedule_to_close_timeout` or workflow execution timeout if that is not
* specified. More info:
* https://docs.temporal.io/docs/content/what-is-a-schedule-to-start-timeout/
* (-- api-linter: core::0140::prepositions=disabled
* aip.dev/not-precedent: "to" is used to indicate interval. --)
*
*
* .google.protobuf.Duration schedule_to_start_timeout = 8 [(.gogoproto.stdduration) = true];
* @return The scheduleToStartTimeout.
*/
com.google.protobuf.Duration getScheduleToStartTimeout();
/**
*
* Limits the time an activity task can stay in a task queue before a worker picks it up. The
* "schedule" time is when the most recent retry is scheduled. This timeout should usually not
* be set: it's useful in specific scenarios like worker-specific task queues. This timeout is
* always non retryable, as all a retry would achieve is to put it back into the same queue.
* Defaults to `schedule_to_close_timeout` or workflow execution timeout if that is not
* specified. More info:
* https://docs.temporal.io/docs/content/what-is-a-schedule-to-start-timeout/
* (-- api-linter: core::0140::prepositions=disabled
* aip.dev/not-precedent: "to" is used to indicate interval. --)
*
*
* .google.protobuf.Duration schedule_to_start_timeout = 8 [(.gogoproto.stdduration) = true];
*/
com.google.protobuf.DurationOrBuilder getScheduleToStartTimeoutOrBuilder();
/**
*
* Maximum time an activity is allowed to execute after being picked up by a worker. This
* timeout is always retryable. Either this or `schedule_to_close_timeout` must be specified.
* (-- api-linter: core::0140::prepositions=disabled
* aip.dev/not-precedent: "to" is used to indicate interval. --)
*
*
* .google.protobuf.Duration start_to_close_timeout = 9 [(.gogoproto.stdduration) = true];
* @return Whether the startToCloseTimeout field is set.
*/
boolean hasStartToCloseTimeout();
/**
*
* Maximum time an activity is allowed to execute after being picked up by a worker. This
* timeout is always retryable. Either this or `schedule_to_close_timeout` must be specified.
* (-- api-linter: core::0140::prepositions=disabled
* aip.dev/not-precedent: "to" is used to indicate interval. --)
*
*
* .google.protobuf.Duration start_to_close_timeout = 9 [(.gogoproto.stdduration) = true];
* @return The startToCloseTimeout.
*/
com.google.protobuf.Duration getStartToCloseTimeout();
/**
*
* Maximum time an activity is allowed to execute after being picked up by a worker. This
* timeout is always retryable. Either this or `schedule_to_close_timeout` must be specified.
* (-- api-linter: core::0140::prepositions=disabled
* aip.dev/not-precedent: "to" is used to indicate interval. --)
*
*
* .google.protobuf.Duration start_to_close_timeout = 9 [(.gogoproto.stdduration) = true];
*/
com.google.protobuf.DurationOrBuilder getStartToCloseTimeoutOrBuilder();
/**
*
* Maximum permitted time between successful worker heartbeats.
*
*
* .google.protobuf.Duration heartbeat_timeout = 10 [(.gogoproto.stdduration) = true];
* @return Whether the heartbeatTimeout field is set.
*/
boolean hasHeartbeatTimeout();
/**
*
* Maximum permitted time between successful worker heartbeats.
*
*
* .google.protobuf.Duration heartbeat_timeout = 10 [(.gogoproto.stdduration) = true];
* @return The heartbeatTimeout.
*/
com.google.protobuf.Duration getHeartbeatTimeout();
/**
*
* Maximum permitted time between successful worker heartbeats.
*
*
* .google.protobuf.Duration heartbeat_timeout = 10 [(.gogoproto.stdduration) = true];
*/
com.google.protobuf.DurationOrBuilder getHeartbeatTimeoutOrBuilder();
/**
*
* Activities are provided by a default retry policy which is controlled through the service's
* dynamic configuration. Retries will be attempted until `schedule_to_close_timeout` has
* elapsed. To disable retries set retry_policy.maximum_attempts to 1.
*
*
* .temporal.api.common.v1.RetryPolicy retry_policy = 11;
* @return Whether the retryPolicy field is set.
*/
boolean hasRetryPolicy();
/**
*
* Activities are provided by a default retry policy which is controlled through the service's
* dynamic configuration. Retries will be attempted until `schedule_to_close_timeout` has
* elapsed. To disable retries set retry_policy.maximum_attempts to 1.
*
*
* .temporal.api.common.v1.RetryPolicy retry_policy = 11;
* @return The retryPolicy.
*/
io.temporal.api.common.v1.RetryPolicy getRetryPolicy();
/**
*
* Activities are provided by a default retry policy which is controlled through the service's
* dynamic configuration. Retries will be attempted until `schedule_to_close_timeout` has
* elapsed. To disable retries set retry_policy.maximum_attempts to 1.
*
*
* .temporal.api.common.v1.RetryPolicy retry_policy = 11;
*/
io.temporal.api.common.v1.RetryPolicyOrBuilder getRetryPolicyOrBuilder();
/**
*
* Request to start the activity directly bypassing matching service and worker polling
* The slot for executing the activity should be reserved when setting this field to true.
*
*
* bool request_eager_execution = 12;
* @return The requestEagerExecution.
*/
boolean getRequestEagerExecution();
/**
*
* If this is set, the workflow executing this command wishes to start the activity using
* a version compatible with the version that this workflow most recently ran on, if such
* behavior is possible.
*
*
* bool use_compatible_version = 13;
* @return The useCompatibleVersion.
*/
boolean getUseCompatibleVersion();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy