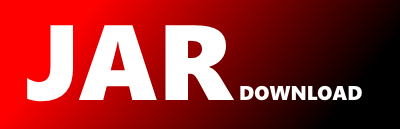
io.temporal.api.schedule.v1.ScheduleSpec Maven / Gradle / Ivy
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: temporal/api/schedule/v1/message.proto
package io.temporal.api.schedule.v1;
/**
*
* ScheduleSpec is a complete description of a set of absolute timestamps
* (possibly infinite) that an action should occur at. The meaning of a
* ScheduleSpec depends only on its contents and never changes, except that the
* definition of a time zone can change over time (most commonly, when daylight
* saving time policy changes for an area). To create a totally self-contained
* ScheduleSpec, use UTC or include timezone_data.
* For input, you can provide zero or more of: structured_calendar, calendar,
* cron_string, interval, and exclude_structured_calendar, and all of them will
* be used (the schedule will take action at the union of all of their times,
* minus the ones that match exclude_structured_calendar).
* On input, calendar and cron_string fields will be compiled into
* structured_calendar (and maybe interval and timezone_name), so if you
* Describe a schedule, you'll see only structured_calendar, interval, etc.
*
*
* Protobuf type {@code temporal.api.schedule.v1.ScheduleSpec}
*/
@javax.annotation.Generated(value="protoc", comments="annotations:ScheduleSpec.java.pb.meta")
public final class ScheduleSpec extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:temporal.api.schedule.v1.ScheduleSpec)
ScheduleSpecOrBuilder {
private static final long serialVersionUID = 0L;
// Use ScheduleSpec.newBuilder() to construct.
private ScheduleSpec(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ScheduleSpec() {
structuredCalendar_ = java.util.Collections.emptyList();
cronString_ = com.google.protobuf.LazyStringArrayList.EMPTY;
calendar_ = java.util.Collections.emptyList();
interval_ = java.util.Collections.emptyList();
excludeCalendar_ = java.util.Collections.emptyList();
excludeStructuredCalendar_ = java.util.Collections.emptyList();
timezoneName_ = "";
timezoneData_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new ScheduleSpec();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ScheduleSpec(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
if (!((mutable_bitField0_ & 0x00000004) != 0)) {
calendar_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000004;
}
calendar_.add(
input.readMessage(io.temporal.api.schedule.v1.CalendarSpec.parser(), extensionRegistry));
break;
}
case 18: {
if (!((mutable_bitField0_ & 0x00000008) != 0)) {
interval_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000008;
}
interval_.add(
input.readMessage(io.temporal.api.schedule.v1.IntervalSpec.parser(), extensionRegistry));
break;
}
case 26: {
if (!((mutable_bitField0_ & 0x00000010) != 0)) {
excludeCalendar_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000010;
}
excludeCalendar_.add(
input.readMessage(io.temporal.api.schedule.v1.CalendarSpec.parser(), extensionRegistry));
break;
}
case 34: {
com.google.protobuf.Timestamp.Builder subBuilder = null;
if (startTime_ != null) {
subBuilder = startTime_.toBuilder();
}
startTime_ = input.readMessage(com.google.protobuf.Timestamp.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(startTime_);
startTime_ = subBuilder.buildPartial();
}
break;
}
case 42: {
com.google.protobuf.Timestamp.Builder subBuilder = null;
if (endTime_ != null) {
subBuilder = endTime_.toBuilder();
}
endTime_ = input.readMessage(com.google.protobuf.Timestamp.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(endTime_);
endTime_ = subBuilder.buildPartial();
}
break;
}
case 50: {
com.google.protobuf.Duration.Builder subBuilder = null;
if (jitter_ != null) {
subBuilder = jitter_.toBuilder();
}
jitter_ = input.readMessage(com.google.protobuf.Duration.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(jitter_);
jitter_ = subBuilder.buildPartial();
}
break;
}
case 58: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
structuredCalendar_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
structuredCalendar_.add(
input.readMessage(io.temporal.api.schedule.v1.StructuredCalendarSpec.parser(), extensionRegistry));
break;
}
case 66: {
java.lang.String s = input.readStringRequireUtf8();
if (!((mutable_bitField0_ & 0x00000002) != 0)) {
cronString_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000002;
}
cronString_.add(s);
break;
}
case 74: {
if (!((mutable_bitField0_ & 0x00000020) != 0)) {
excludeStructuredCalendar_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000020;
}
excludeStructuredCalendar_.add(
input.readMessage(io.temporal.api.schedule.v1.StructuredCalendarSpec.parser(), extensionRegistry));
break;
}
case 82: {
java.lang.String s = input.readStringRequireUtf8();
timezoneName_ = s;
break;
}
case 90: {
timezoneData_ = input.readBytes();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000004) != 0)) {
calendar_ = java.util.Collections.unmodifiableList(calendar_);
}
if (((mutable_bitField0_ & 0x00000008) != 0)) {
interval_ = java.util.Collections.unmodifiableList(interval_);
}
if (((mutable_bitField0_ & 0x00000010) != 0)) {
excludeCalendar_ = java.util.Collections.unmodifiableList(excludeCalendar_);
}
if (((mutable_bitField0_ & 0x00000001) != 0)) {
structuredCalendar_ = java.util.Collections.unmodifiableList(structuredCalendar_);
}
if (((mutable_bitField0_ & 0x00000002) != 0)) {
cronString_ = cronString_.getUnmodifiableView();
}
if (((mutable_bitField0_ & 0x00000020) != 0)) {
excludeStructuredCalendar_ = java.util.Collections.unmodifiableList(excludeStructuredCalendar_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.temporal.api.schedule.v1.MessageProto.internal_static_temporal_api_schedule_v1_ScheduleSpec_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.temporal.api.schedule.v1.MessageProto.internal_static_temporal_api_schedule_v1_ScheduleSpec_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.temporal.api.schedule.v1.ScheduleSpec.class, io.temporal.api.schedule.v1.ScheduleSpec.Builder.class);
}
public static final int STRUCTURED_CALENDAR_FIELD_NUMBER = 7;
private java.util.List structuredCalendar_;
/**
*
* Calendar-based specifications of times.
*
*
* repeated .temporal.api.schedule.v1.StructuredCalendarSpec structured_calendar = 7;
*/
public java.util.List getStructuredCalendarList() {
return structuredCalendar_;
}
/**
*
* Calendar-based specifications of times.
*
*
* repeated .temporal.api.schedule.v1.StructuredCalendarSpec structured_calendar = 7;
*/
public java.util.List extends io.temporal.api.schedule.v1.StructuredCalendarSpecOrBuilder>
getStructuredCalendarOrBuilderList() {
return structuredCalendar_;
}
/**
*
* Calendar-based specifications of times.
*
*
* repeated .temporal.api.schedule.v1.StructuredCalendarSpec structured_calendar = 7;
*/
public int getStructuredCalendarCount() {
return structuredCalendar_.size();
}
/**
*
* Calendar-based specifications of times.
*
*
* repeated .temporal.api.schedule.v1.StructuredCalendarSpec structured_calendar = 7;
*/
public io.temporal.api.schedule.v1.StructuredCalendarSpec getStructuredCalendar(int index) {
return structuredCalendar_.get(index);
}
/**
*
* Calendar-based specifications of times.
*
*
* repeated .temporal.api.schedule.v1.StructuredCalendarSpec structured_calendar = 7;
*/
public io.temporal.api.schedule.v1.StructuredCalendarSpecOrBuilder getStructuredCalendarOrBuilder(
int index) {
return structuredCalendar_.get(index);
}
public static final int CRON_STRING_FIELD_NUMBER = 8;
private com.google.protobuf.LazyStringList cronString_;
/**
*
* cron_string holds a traditional cron specification as a string. It
* accepts 5, 6, or 7 fields, separated by spaces, and interprets them the
* same way as CalendarSpec.
* 5 fields: minute, hour, day_of_month, month, day_of_week
* 6 fields: minute, hour, day_of_month, month, day_of_week, year
* 7 fields: second, minute, hour, day_of_month, month, day_of_week, year
* If year is not given, it defaults to *. If second is not given, it
* defaults to 0.
* Shorthands @yearly, @monthly, @weekly, @daily, and @hourly are also
* accepted instead of the 5-7 time fields.
* Optionally, the string can be preceded by CRON_TZ=<timezone name> or
* TZ=<timezone name>, which will get copied to timezone_name. (There must
* not also be a timezone_name present.)
* Optionally "#" followed by a comment can appear at the end of the string.
* Note that the special case that some cron implementations have for
* treating day_of_month and day_of_week as "or" instead of "and" when both
* are set is not implemented.
* @every <interval>[/<phase>] is accepted and gets compiled into an
* IntervalSpec instead. <interval> and <phase> should be a decimal integer
* with a unit suffix s, m, h, or d.
*
*
* repeated string cron_string = 8;
* @return A list containing the cronString.
*/
public com.google.protobuf.ProtocolStringList
getCronStringList() {
return cronString_;
}
/**
*
* cron_string holds a traditional cron specification as a string. It
* accepts 5, 6, or 7 fields, separated by spaces, and interprets them the
* same way as CalendarSpec.
* 5 fields: minute, hour, day_of_month, month, day_of_week
* 6 fields: minute, hour, day_of_month, month, day_of_week, year
* 7 fields: second, minute, hour, day_of_month, month, day_of_week, year
* If year is not given, it defaults to *. If second is not given, it
* defaults to 0.
* Shorthands @yearly, @monthly, @weekly, @daily, and @hourly are also
* accepted instead of the 5-7 time fields.
* Optionally, the string can be preceded by CRON_TZ=<timezone name> or
* TZ=<timezone name>, which will get copied to timezone_name. (There must
* not also be a timezone_name present.)
* Optionally "#" followed by a comment can appear at the end of the string.
* Note that the special case that some cron implementations have for
* treating day_of_month and day_of_week as "or" instead of "and" when both
* are set is not implemented.
* @every <interval>[/<phase>] is accepted and gets compiled into an
* IntervalSpec instead. <interval> and <phase> should be a decimal integer
* with a unit suffix s, m, h, or d.
*
*
* repeated string cron_string = 8;
* @return The count of cronString.
*/
public int getCronStringCount() {
return cronString_.size();
}
/**
*
* cron_string holds a traditional cron specification as a string. It
* accepts 5, 6, or 7 fields, separated by spaces, and interprets them the
* same way as CalendarSpec.
* 5 fields: minute, hour, day_of_month, month, day_of_week
* 6 fields: minute, hour, day_of_month, month, day_of_week, year
* 7 fields: second, minute, hour, day_of_month, month, day_of_week, year
* If year is not given, it defaults to *. If second is not given, it
* defaults to 0.
* Shorthands @yearly, @monthly, @weekly, @daily, and @hourly are also
* accepted instead of the 5-7 time fields.
* Optionally, the string can be preceded by CRON_TZ=<timezone name> or
* TZ=<timezone name>, which will get copied to timezone_name. (There must
* not also be a timezone_name present.)
* Optionally "#" followed by a comment can appear at the end of the string.
* Note that the special case that some cron implementations have for
* treating day_of_month and day_of_week as "or" instead of "and" when both
* are set is not implemented.
* @every <interval>[/<phase>] is accepted and gets compiled into an
* IntervalSpec instead. <interval> and <phase> should be a decimal integer
* with a unit suffix s, m, h, or d.
*
*
* repeated string cron_string = 8;
* @param index The index of the element to return.
* @return The cronString at the given index.
*/
public java.lang.String getCronString(int index) {
return cronString_.get(index);
}
/**
*
* cron_string holds a traditional cron specification as a string. It
* accepts 5, 6, or 7 fields, separated by spaces, and interprets them the
* same way as CalendarSpec.
* 5 fields: minute, hour, day_of_month, month, day_of_week
* 6 fields: minute, hour, day_of_month, month, day_of_week, year
* 7 fields: second, minute, hour, day_of_month, month, day_of_week, year
* If year is not given, it defaults to *. If second is not given, it
* defaults to 0.
* Shorthands @yearly, @monthly, @weekly, @daily, and @hourly are also
* accepted instead of the 5-7 time fields.
* Optionally, the string can be preceded by CRON_TZ=<timezone name> or
* TZ=<timezone name>, which will get copied to timezone_name. (There must
* not also be a timezone_name present.)
* Optionally "#" followed by a comment can appear at the end of the string.
* Note that the special case that some cron implementations have for
* treating day_of_month and day_of_week as "or" instead of "and" when both
* are set is not implemented.
* @every <interval>[/<phase>] is accepted and gets compiled into an
* IntervalSpec instead. <interval> and <phase> should be a decimal integer
* with a unit suffix s, m, h, or d.
*
*
* repeated string cron_string = 8;
* @param index The index of the value to return.
* @return The bytes of the cronString at the given index.
*/
public com.google.protobuf.ByteString
getCronStringBytes(int index) {
return cronString_.getByteString(index);
}
public static final int CALENDAR_FIELD_NUMBER = 1;
private java.util.List calendar_;
/**
*
* Calendar-based specifications of times.
*
*
* repeated .temporal.api.schedule.v1.CalendarSpec calendar = 1;
*/
public java.util.List getCalendarList() {
return calendar_;
}
/**
*
* Calendar-based specifications of times.
*
*
* repeated .temporal.api.schedule.v1.CalendarSpec calendar = 1;
*/
public java.util.List extends io.temporal.api.schedule.v1.CalendarSpecOrBuilder>
getCalendarOrBuilderList() {
return calendar_;
}
/**
*
* Calendar-based specifications of times.
*
*
* repeated .temporal.api.schedule.v1.CalendarSpec calendar = 1;
*/
public int getCalendarCount() {
return calendar_.size();
}
/**
*
* Calendar-based specifications of times.
*
*
* repeated .temporal.api.schedule.v1.CalendarSpec calendar = 1;
*/
public io.temporal.api.schedule.v1.CalendarSpec getCalendar(int index) {
return calendar_.get(index);
}
/**
*
* Calendar-based specifications of times.
*
*
* repeated .temporal.api.schedule.v1.CalendarSpec calendar = 1;
*/
public io.temporal.api.schedule.v1.CalendarSpecOrBuilder getCalendarOrBuilder(
int index) {
return calendar_.get(index);
}
public static final int INTERVAL_FIELD_NUMBER = 2;
private java.util.List interval_;
/**
*
* Interval-based specifications of times.
*
*
* repeated .temporal.api.schedule.v1.IntervalSpec interval = 2;
*/
public java.util.List getIntervalList() {
return interval_;
}
/**
*
* Interval-based specifications of times.
*
*
* repeated .temporal.api.schedule.v1.IntervalSpec interval = 2;
*/
public java.util.List extends io.temporal.api.schedule.v1.IntervalSpecOrBuilder>
getIntervalOrBuilderList() {
return interval_;
}
/**
*
* Interval-based specifications of times.
*
*
* repeated .temporal.api.schedule.v1.IntervalSpec interval = 2;
*/
public int getIntervalCount() {
return interval_.size();
}
/**
*
* Interval-based specifications of times.
*
*
* repeated .temporal.api.schedule.v1.IntervalSpec interval = 2;
*/
public io.temporal.api.schedule.v1.IntervalSpec getInterval(int index) {
return interval_.get(index);
}
/**
*
* Interval-based specifications of times.
*
*
* repeated .temporal.api.schedule.v1.IntervalSpec interval = 2;
*/
public io.temporal.api.schedule.v1.IntervalSpecOrBuilder getIntervalOrBuilder(
int index) {
return interval_.get(index);
}
public static final int EXCLUDE_CALENDAR_FIELD_NUMBER = 3;
private java.util.List excludeCalendar_;
/**
*
* Any timestamps matching any of exclude_* will be skipped.
*
*
* repeated .temporal.api.schedule.v1.CalendarSpec exclude_calendar = 3 [deprecated = true];
*/
@java.lang.Deprecated public java.util.List getExcludeCalendarList() {
return excludeCalendar_;
}
/**
*
* Any timestamps matching any of exclude_* will be skipped.
*
*
* repeated .temporal.api.schedule.v1.CalendarSpec exclude_calendar = 3 [deprecated = true];
*/
@java.lang.Deprecated public java.util.List extends io.temporal.api.schedule.v1.CalendarSpecOrBuilder>
getExcludeCalendarOrBuilderList() {
return excludeCalendar_;
}
/**
*
* Any timestamps matching any of exclude_* will be skipped.
*
*
* repeated .temporal.api.schedule.v1.CalendarSpec exclude_calendar = 3 [deprecated = true];
*/
@java.lang.Deprecated public int getExcludeCalendarCount() {
return excludeCalendar_.size();
}
/**
*
* Any timestamps matching any of exclude_* will be skipped.
*
*
* repeated .temporal.api.schedule.v1.CalendarSpec exclude_calendar = 3 [deprecated = true];
*/
@java.lang.Deprecated public io.temporal.api.schedule.v1.CalendarSpec getExcludeCalendar(int index) {
return excludeCalendar_.get(index);
}
/**
*
* Any timestamps matching any of exclude_* will be skipped.
*
*
* repeated .temporal.api.schedule.v1.CalendarSpec exclude_calendar = 3 [deprecated = true];
*/
@java.lang.Deprecated public io.temporal.api.schedule.v1.CalendarSpecOrBuilder getExcludeCalendarOrBuilder(
int index) {
return excludeCalendar_.get(index);
}
public static final int EXCLUDE_STRUCTURED_CALENDAR_FIELD_NUMBER = 9;
private java.util.List excludeStructuredCalendar_;
/**
* repeated .temporal.api.schedule.v1.StructuredCalendarSpec exclude_structured_calendar = 9;
*/
public java.util.List getExcludeStructuredCalendarList() {
return excludeStructuredCalendar_;
}
/**
* repeated .temporal.api.schedule.v1.StructuredCalendarSpec exclude_structured_calendar = 9;
*/
public java.util.List extends io.temporal.api.schedule.v1.StructuredCalendarSpecOrBuilder>
getExcludeStructuredCalendarOrBuilderList() {
return excludeStructuredCalendar_;
}
/**
* repeated .temporal.api.schedule.v1.StructuredCalendarSpec exclude_structured_calendar = 9;
*/
public int getExcludeStructuredCalendarCount() {
return excludeStructuredCalendar_.size();
}
/**
* repeated .temporal.api.schedule.v1.StructuredCalendarSpec exclude_structured_calendar = 9;
*/
public io.temporal.api.schedule.v1.StructuredCalendarSpec getExcludeStructuredCalendar(int index) {
return excludeStructuredCalendar_.get(index);
}
/**
* repeated .temporal.api.schedule.v1.StructuredCalendarSpec exclude_structured_calendar = 9;
*/
public io.temporal.api.schedule.v1.StructuredCalendarSpecOrBuilder getExcludeStructuredCalendarOrBuilder(
int index) {
return excludeStructuredCalendar_.get(index);
}
public static final int START_TIME_FIELD_NUMBER = 4;
private com.google.protobuf.Timestamp startTime_;
/**
*
* If start_time is set, any timestamps before start_time will be skipped.
* (Together, start_time and end_time make an inclusive interval.)
*
*
* .google.protobuf.Timestamp start_time = 4 [(.gogoproto.stdtime) = true];
* @return Whether the startTime field is set.
*/
public boolean hasStartTime() {
return startTime_ != null;
}
/**
*
* If start_time is set, any timestamps before start_time will be skipped.
* (Together, start_time and end_time make an inclusive interval.)
*
*
* .google.protobuf.Timestamp start_time = 4 [(.gogoproto.stdtime) = true];
* @return The startTime.
*/
public com.google.protobuf.Timestamp getStartTime() {
return startTime_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : startTime_;
}
/**
*
* If start_time is set, any timestamps before start_time will be skipped.
* (Together, start_time and end_time make an inclusive interval.)
*
*
* .google.protobuf.Timestamp start_time = 4 [(.gogoproto.stdtime) = true];
*/
public com.google.protobuf.TimestampOrBuilder getStartTimeOrBuilder() {
return getStartTime();
}
public static final int END_TIME_FIELD_NUMBER = 5;
private com.google.protobuf.Timestamp endTime_;
/**
*
* If end_time is set, any timestamps after end_time will be skipped.
*
*
* .google.protobuf.Timestamp end_time = 5 [(.gogoproto.stdtime) = true];
* @return Whether the endTime field is set.
*/
public boolean hasEndTime() {
return endTime_ != null;
}
/**
*
* If end_time is set, any timestamps after end_time will be skipped.
*
*
* .google.protobuf.Timestamp end_time = 5 [(.gogoproto.stdtime) = true];
* @return The endTime.
*/
public com.google.protobuf.Timestamp getEndTime() {
return endTime_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : endTime_;
}
/**
*
* If end_time is set, any timestamps after end_time will be skipped.
*
*
* .google.protobuf.Timestamp end_time = 5 [(.gogoproto.stdtime) = true];
*/
public com.google.protobuf.TimestampOrBuilder getEndTimeOrBuilder() {
return getEndTime();
}
public static final int JITTER_FIELD_NUMBER = 6;
private com.google.protobuf.Duration jitter_;
/**
*
* All timestamps will be incremented by a random value from 0 to this
* amount of jitter. Default: 0
*
*
* .google.protobuf.Duration jitter = 6 [(.gogoproto.stdduration) = true];
* @return Whether the jitter field is set.
*/
public boolean hasJitter() {
return jitter_ != null;
}
/**
*
* All timestamps will be incremented by a random value from 0 to this
* amount of jitter. Default: 0
*
*
* .google.protobuf.Duration jitter = 6 [(.gogoproto.stdduration) = true];
* @return The jitter.
*/
public com.google.protobuf.Duration getJitter() {
return jitter_ == null ? com.google.protobuf.Duration.getDefaultInstance() : jitter_;
}
/**
*
* All timestamps will be incremented by a random value from 0 to this
* amount of jitter. Default: 0
*
*
* .google.protobuf.Duration jitter = 6 [(.gogoproto.stdduration) = true];
*/
public com.google.protobuf.DurationOrBuilder getJitterOrBuilder() {
return getJitter();
}
public static final int TIMEZONE_NAME_FIELD_NUMBER = 10;
private volatile java.lang.Object timezoneName_;
/**
*
* Time zone to interpret all calendar-based specs in.
* If unset, defaults to UTC. We recommend using UTC for your application if
* at all possible, to avoid various surprising properties of time zones.
* Time zones may be provided by name, corresponding to names in the IANA
* time zone database (see https://www.iana.org/time-zones). The definition
* will be loaded by the Temporal server from the environment it runs in.
* If your application requires more control over the time zone definition
* used, it may pass in a complete definition in the form of a TZif file
* from the time zone database. If present, this will be used instead of
* loading anything from the environment. You are then responsible for
* updating timezone_data when the definition changes.
* Calendar spec matching is based on literal matching of the clock time
* with no special handling of DST: if you write a calendar spec that fires
* at 2:30am and specify a time zone that follows DST, that action will not
* be triggered on the day that has no 2:30am. Similarly, an action that
* fires at 1:30am will be triggered twice on the day that has two 1:30s.
* Also note that no actions are taken on leap-seconds (e.g. 23:59:60 UTC).
*
*
* string timezone_name = 10;
* @return The timezoneName.
*/
public java.lang.String getTimezoneName() {
java.lang.Object ref = timezoneName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
timezoneName_ = s;
return s;
}
}
/**
*
* Time zone to interpret all calendar-based specs in.
* If unset, defaults to UTC. We recommend using UTC for your application if
* at all possible, to avoid various surprising properties of time zones.
* Time zones may be provided by name, corresponding to names in the IANA
* time zone database (see https://www.iana.org/time-zones). The definition
* will be loaded by the Temporal server from the environment it runs in.
* If your application requires more control over the time zone definition
* used, it may pass in a complete definition in the form of a TZif file
* from the time zone database. If present, this will be used instead of
* loading anything from the environment. You are then responsible for
* updating timezone_data when the definition changes.
* Calendar spec matching is based on literal matching of the clock time
* with no special handling of DST: if you write a calendar spec that fires
* at 2:30am and specify a time zone that follows DST, that action will not
* be triggered on the day that has no 2:30am. Similarly, an action that
* fires at 1:30am will be triggered twice on the day that has two 1:30s.
* Also note that no actions are taken on leap-seconds (e.g. 23:59:60 UTC).
*
*
* string timezone_name = 10;
* @return The bytes for timezoneName.
*/
public com.google.protobuf.ByteString
getTimezoneNameBytes() {
java.lang.Object ref = timezoneName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
timezoneName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TIMEZONE_DATA_FIELD_NUMBER = 11;
private com.google.protobuf.ByteString timezoneData_;
/**
* bytes timezone_data = 11;
* @return The timezoneData.
*/
public com.google.protobuf.ByteString getTimezoneData() {
return timezoneData_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < calendar_.size(); i++) {
output.writeMessage(1, calendar_.get(i));
}
for (int i = 0; i < interval_.size(); i++) {
output.writeMessage(2, interval_.get(i));
}
for (int i = 0; i < excludeCalendar_.size(); i++) {
output.writeMessage(3, excludeCalendar_.get(i));
}
if (startTime_ != null) {
output.writeMessage(4, getStartTime());
}
if (endTime_ != null) {
output.writeMessage(5, getEndTime());
}
if (jitter_ != null) {
output.writeMessage(6, getJitter());
}
for (int i = 0; i < structuredCalendar_.size(); i++) {
output.writeMessage(7, structuredCalendar_.get(i));
}
for (int i = 0; i < cronString_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 8, cronString_.getRaw(i));
}
for (int i = 0; i < excludeStructuredCalendar_.size(); i++) {
output.writeMessage(9, excludeStructuredCalendar_.get(i));
}
if (!getTimezoneNameBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 10, timezoneName_);
}
if (!timezoneData_.isEmpty()) {
output.writeBytes(11, timezoneData_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < calendar_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, calendar_.get(i));
}
for (int i = 0; i < interval_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, interval_.get(i));
}
for (int i = 0; i < excludeCalendar_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, excludeCalendar_.get(i));
}
if (startTime_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, getStartTime());
}
if (endTime_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, getEndTime());
}
if (jitter_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, getJitter());
}
for (int i = 0; i < structuredCalendar_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(7, structuredCalendar_.get(i));
}
{
int dataSize = 0;
for (int i = 0; i < cronString_.size(); i++) {
dataSize += computeStringSizeNoTag(cronString_.getRaw(i));
}
size += dataSize;
size += 1 * getCronStringList().size();
}
for (int i = 0; i < excludeStructuredCalendar_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(9, excludeStructuredCalendar_.get(i));
}
if (!getTimezoneNameBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(10, timezoneName_);
}
if (!timezoneData_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(11, timezoneData_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.temporal.api.schedule.v1.ScheduleSpec)) {
return super.equals(obj);
}
io.temporal.api.schedule.v1.ScheduleSpec other = (io.temporal.api.schedule.v1.ScheduleSpec) obj;
if (!getStructuredCalendarList()
.equals(other.getStructuredCalendarList())) return false;
if (!getCronStringList()
.equals(other.getCronStringList())) return false;
if (!getCalendarList()
.equals(other.getCalendarList())) return false;
if (!getIntervalList()
.equals(other.getIntervalList())) return false;
if (!getExcludeCalendarList()
.equals(other.getExcludeCalendarList())) return false;
if (!getExcludeStructuredCalendarList()
.equals(other.getExcludeStructuredCalendarList())) return false;
if (hasStartTime() != other.hasStartTime()) return false;
if (hasStartTime()) {
if (!getStartTime()
.equals(other.getStartTime())) return false;
}
if (hasEndTime() != other.hasEndTime()) return false;
if (hasEndTime()) {
if (!getEndTime()
.equals(other.getEndTime())) return false;
}
if (hasJitter() != other.hasJitter()) return false;
if (hasJitter()) {
if (!getJitter()
.equals(other.getJitter())) return false;
}
if (!getTimezoneName()
.equals(other.getTimezoneName())) return false;
if (!getTimezoneData()
.equals(other.getTimezoneData())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getStructuredCalendarCount() > 0) {
hash = (37 * hash) + STRUCTURED_CALENDAR_FIELD_NUMBER;
hash = (53 * hash) + getStructuredCalendarList().hashCode();
}
if (getCronStringCount() > 0) {
hash = (37 * hash) + CRON_STRING_FIELD_NUMBER;
hash = (53 * hash) + getCronStringList().hashCode();
}
if (getCalendarCount() > 0) {
hash = (37 * hash) + CALENDAR_FIELD_NUMBER;
hash = (53 * hash) + getCalendarList().hashCode();
}
if (getIntervalCount() > 0) {
hash = (37 * hash) + INTERVAL_FIELD_NUMBER;
hash = (53 * hash) + getIntervalList().hashCode();
}
if (getExcludeCalendarCount() > 0) {
hash = (37 * hash) + EXCLUDE_CALENDAR_FIELD_NUMBER;
hash = (53 * hash) + getExcludeCalendarList().hashCode();
}
if (getExcludeStructuredCalendarCount() > 0) {
hash = (37 * hash) + EXCLUDE_STRUCTURED_CALENDAR_FIELD_NUMBER;
hash = (53 * hash) + getExcludeStructuredCalendarList().hashCode();
}
if (hasStartTime()) {
hash = (37 * hash) + START_TIME_FIELD_NUMBER;
hash = (53 * hash) + getStartTime().hashCode();
}
if (hasEndTime()) {
hash = (37 * hash) + END_TIME_FIELD_NUMBER;
hash = (53 * hash) + getEndTime().hashCode();
}
if (hasJitter()) {
hash = (37 * hash) + JITTER_FIELD_NUMBER;
hash = (53 * hash) + getJitter().hashCode();
}
hash = (37 * hash) + TIMEZONE_NAME_FIELD_NUMBER;
hash = (53 * hash) + getTimezoneName().hashCode();
hash = (37 * hash) + TIMEZONE_DATA_FIELD_NUMBER;
hash = (53 * hash) + getTimezoneData().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.temporal.api.schedule.v1.ScheduleSpec parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.temporal.api.schedule.v1.ScheduleSpec parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.temporal.api.schedule.v1.ScheduleSpec parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.temporal.api.schedule.v1.ScheduleSpec parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.temporal.api.schedule.v1.ScheduleSpec parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.temporal.api.schedule.v1.ScheduleSpec parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.temporal.api.schedule.v1.ScheduleSpec parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.temporal.api.schedule.v1.ScheduleSpec parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.temporal.api.schedule.v1.ScheduleSpec parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.temporal.api.schedule.v1.ScheduleSpec parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.temporal.api.schedule.v1.ScheduleSpec parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.temporal.api.schedule.v1.ScheduleSpec parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.temporal.api.schedule.v1.ScheduleSpec prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* ScheduleSpec is a complete description of a set of absolute timestamps
* (possibly infinite) that an action should occur at. The meaning of a
* ScheduleSpec depends only on its contents and never changes, except that the
* definition of a time zone can change over time (most commonly, when daylight
* saving time policy changes for an area). To create a totally self-contained
* ScheduleSpec, use UTC or include timezone_data.
* For input, you can provide zero or more of: structured_calendar, calendar,
* cron_string, interval, and exclude_structured_calendar, and all of them will
* be used (the schedule will take action at the union of all of their times,
* minus the ones that match exclude_structured_calendar).
* On input, calendar and cron_string fields will be compiled into
* structured_calendar (and maybe interval and timezone_name), so if you
* Describe a schedule, you'll see only structured_calendar, interval, etc.
*
*
* Protobuf type {@code temporal.api.schedule.v1.ScheduleSpec}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:temporal.api.schedule.v1.ScheduleSpec)
io.temporal.api.schedule.v1.ScheduleSpecOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.temporal.api.schedule.v1.MessageProto.internal_static_temporal_api_schedule_v1_ScheduleSpec_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.temporal.api.schedule.v1.MessageProto.internal_static_temporal_api_schedule_v1_ScheduleSpec_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.temporal.api.schedule.v1.ScheduleSpec.class, io.temporal.api.schedule.v1.ScheduleSpec.Builder.class);
}
// Construct using io.temporal.api.schedule.v1.ScheduleSpec.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getStructuredCalendarFieldBuilder();
getCalendarFieldBuilder();
getIntervalFieldBuilder();
getExcludeCalendarFieldBuilder();
getExcludeStructuredCalendarFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (structuredCalendarBuilder_ == null) {
structuredCalendar_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
structuredCalendarBuilder_.clear();
}
cronString_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000002);
if (calendarBuilder_ == null) {
calendar_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
} else {
calendarBuilder_.clear();
}
if (intervalBuilder_ == null) {
interval_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000008);
} else {
intervalBuilder_.clear();
}
if (excludeCalendarBuilder_ == null) {
excludeCalendar_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000010);
} else {
excludeCalendarBuilder_.clear();
}
if (excludeStructuredCalendarBuilder_ == null) {
excludeStructuredCalendar_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000020);
} else {
excludeStructuredCalendarBuilder_.clear();
}
if (startTimeBuilder_ == null) {
startTime_ = null;
} else {
startTime_ = null;
startTimeBuilder_ = null;
}
if (endTimeBuilder_ == null) {
endTime_ = null;
} else {
endTime_ = null;
endTimeBuilder_ = null;
}
if (jitterBuilder_ == null) {
jitter_ = null;
} else {
jitter_ = null;
jitterBuilder_ = null;
}
timezoneName_ = "";
timezoneData_ = com.google.protobuf.ByteString.EMPTY;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.temporal.api.schedule.v1.MessageProto.internal_static_temporal_api_schedule_v1_ScheduleSpec_descriptor;
}
@java.lang.Override
public io.temporal.api.schedule.v1.ScheduleSpec getDefaultInstanceForType() {
return io.temporal.api.schedule.v1.ScheduleSpec.getDefaultInstance();
}
@java.lang.Override
public io.temporal.api.schedule.v1.ScheduleSpec build() {
io.temporal.api.schedule.v1.ScheduleSpec result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.temporal.api.schedule.v1.ScheduleSpec buildPartial() {
io.temporal.api.schedule.v1.ScheduleSpec result = new io.temporal.api.schedule.v1.ScheduleSpec(this);
int from_bitField0_ = bitField0_;
if (structuredCalendarBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)) {
structuredCalendar_ = java.util.Collections.unmodifiableList(structuredCalendar_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.structuredCalendar_ = structuredCalendar_;
} else {
result.structuredCalendar_ = structuredCalendarBuilder_.build();
}
if (((bitField0_ & 0x00000002) != 0)) {
cronString_ = cronString_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00000002);
}
result.cronString_ = cronString_;
if (calendarBuilder_ == null) {
if (((bitField0_ & 0x00000004) != 0)) {
calendar_ = java.util.Collections.unmodifiableList(calendar_);
bitField0_ = (bitField0_ & ~0x00000004);
}
result.calendar_ = calendar_;
} else {
result.calendar_ = calendarBuilder_.build();
}
if (intervalBuilder_ == null) {
if (((bitField0_ & 0x00000008) != 0)) {
interval_ = java.util.Collections.unmodifiableList(interval_);
bitField0_ = (bitField0_ & ~0x00000008);
}
result.interval_ = interval_;
} else {
result.interval_ = intervalBuilder_.build();
}
if (excludeCalendarBuilder_ == null) {
if (((bitField0_ & 0x00000010) != 0)) {
excludeCalendar_ = java.util.Collections.unmodifiableList(excludeCalendar_);
bitField0_ = (bitField0_ & ~0x00000010);
}
result.excludeCalendar_ = excludeCalendar_;
} else {
result.excludeCalendar_ = excludeCalendarBuilder_.build();
}
if (excludeStructuredCalendarBuilder_ == null) {
if (((bitField0_ & 0x00000020) != 0)) {
excludeStructuredCalendar_ = java.util.Collections.unmodifiableList(excludeStructuredCalendar_);
bitField0_ = (bitField0_ & ~0x00000020);
}
result.excludeStructuredCalendar_ = excludeStructuredCalendar_;
} else {
result.excludeStructuredCalendar_ = excludeStructuredCalendarBuilder_.build();
}
if (startTimeBuilder_ == null) {
result.startTime_ = startTime_;
} else {
result.startTime_ = startTimeBuilder_.build();
}
if (endTimeBuilder_ == null) {
result.endTime_ = endTime_;
} else {
result.endTime_ = endTimeBuilder_.build();
}
if (jitterBuilder_ == null) {
result.jitter_ = jitter_;
} else {
result.jitter_ = jitterBuilder_.build();
}
result.timezoneName_ = timezoneName_;
result.timezoneData_ = timezoneData_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.temporal.api.schedule.v1.ScheduleSpec) {
return mergeFrom((io.temporal.api.schedule.v1.ScheduleSpec)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.temporal.api.schedule.v1.ScheduleSpec other) {
if (other == io.temporal.api.schedule.v1.ScheduleSpec.getDefaultInstance()) return this;
if (structuredCalendarBuilder_ == null) {
if (!other.structuredCalendar_.isEmpty()) {
if (structuredCalendar_.isEmpty()) {
structuredCalendar_ = other.structuredCalendar_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureStructuredCalendarIsMutable();
structuredCalendar_.addAll(other.structuredCalendar_);
}
onChanged();
}
} else {
if (!other.structuredCalendar_.isEmpty()) {
if (structuredCalendarBuilder_.isEmpty()) {
structuredCalendarBuilder_.dispose();
structuredCalendarBuilder_ = null;
structuredCalendar_ = other.structuredCalendar_;
bitField0_ = (bitField0_ & ~0x00000001);
structuredCalendarBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getStructuredCalendarFieldBuilder() : null;
} else {
structuredCalendarBuilder_.addAllMessages(other.structuredCalendar_);
}
}
}
if (!other.cronString_.isEmpty()) {
if (cronString_.isEmpty()) {
cronString_ = other.cronString_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureCronStringIsMutable();
cronString_.addAll(other.cronString_);
}
onChanged();
}
if (calendarBuilder_ == null) {
if (!other.calendar_.isEmpty()) {
if (calendar_.isEmpty()) {
calendar_ = other.calendar_;
bitField0_ = (bitField0_ & ~0x00000004);
} else {
ensureCalendarIsMutable();
calendar_.addAll(other.calendar_);
}
onChanged();
}
} else {
if (!other.calendar_.isEmpty()) {
if (calendarBuilder_.isEmpty()) {
calendarBuilder_.dispose();
calendarBuilder_ = null;
calendar_ = other.calendar_;
bitField0_ = (bitField0_ & ~0x00000004);
calendarBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getCalendarFieldBuilder() : null;
} else {
calendarBuilder_.addAllMessages(other.calendar_);
}
}
}
if (intervalBuilder_ == null) {
if (!other.interval_.isEmpty()) {
if (interval_.isEmpty()) {
interval_ = other.interval_;
bitField0_ = (bitField0_ & ~0x00000008);
} else {
ensureIntervalIsMutable();
interval_.addAll(other.interval_);
}
onChanged();
}
} else {
if (!other.interval_.isEmpty()) {
if (intervalBuilder_.isEmpty()) {
intervalBuilder_.dispose();
intervalBuilder_ = null;
interval_ = other.interval_;
bitField0_ = (bitField0_ & ~0x00000008);
intervalBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getIntervalFieldBuilder() : null;
} else {
intervalBuilder_.addAllMessages(other.interval_);
}
}
}
if (excludeCalendarBuilder_ == null) {
if (!other.excludeCalendar_.isEmpty()) {
if (excludeCalendar_.isEmpty()) {
excludeCalendar_ = other.excludeCalendar_;
bitField0_ = (bitField0_ & ~0x00000010);
} else {
ensureExcludeCalendarIsMutable();
excludeCalendar_.addAll(other.excludeCalendar_);
}
onChanged();
}
} else {
if (!other.excludeCalendar_.isEmpty()) {
if (excludeCalendarBuilder_.isEmpty()) {
excludeCalendarBuilder_.dispose();
excludeCalendarBuilder_ = null;
excludeCalendar_ = other.excludeCalendar_;
bitField0_ = (bitField0_ & ~0x00000010);
excludeCalendarBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getExcludeCalendarFieldBuilder() : null;
} else {
excludeCalendarBuilder_.addAllMessages(other.excludeCalendar_);
}
}
}
if (excludeStructuredCalendarBuilder_ == null) {
if (!other.excludeStructuredCalendar_.isEmpty()) {
if (excludeStructuredCalendar_.isEmpty()) {
excludeStructuredCalendar_ = other.excludeStructuredCalendar_;
bitField0_ = (bitField0_ & ~0x00000020);
} else {
ensureExcludeStructuredCalendarIsMutable();
excludeStructuredCalendar_.addAll(other.excludeStructuredCalendar_);
}
onChanged();
}
} else {
if (!other.excludeStructuredCalendar_.isEmpty()) {
if (excludeStructuredCalendarBuilder_.isEmpty()) {
excludeStructuredCalendarBuilder_.dispose();
excludeStructuredCalendarBuilder_ = null;
excludeStructuredCalendar_ = other.excludeStructuredCalendar_;
bitField0_ = (bitField0_ & ~0x00000020);
excludeStructuredCalendarBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getExcludeStructuredCalendarFieldBuilder() : null;
} else {
excludeStructuredCalendarBuilder_.addAllMessages(other.excludeStructuredCalendar_);
}
}
}
if (other.hasStartTime()) {
mergeStartTime(other.getStartTime());
}
if (other.hasEndTime()) {
mergeEndTime(other.getEndTime());
}
if (other.hasJitter()) {
mergeJitter(other.getJitter());
}
if (!other.getTimezoneName().isEmpty()) {
timezoneName_ = other.timezoneName_;
onChanged();
}
if (other.getTimezoneData() != com.google.protobuf.ByteString.EMPTY) {
setTimezoneData(other.getTimezoneData());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.temporal.api.schedule.v1.ScheduleSpec parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.temporal.api.schedule.v1.ScheduleSpec) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List structuredCalendar_ =
java.util.Collections.emptyList();
private void ensureStructuredCalendarIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
structuredCalendar_ = new java.util.ArrayList(structuredCalendar_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
io.temporal.api.schedule.v1.StructuredCalendarSpec, io.temporal.api.schedule.v1.StructuredCalendarSpec.Builder, io.temporal.api.schedule.v1.StructuredCalendarSpecOrBuilder> structuredCalendarBuilder_;
/**
*
* Calendar-based specifications of times.
*
*
* repeated .temporal.api.schedule.v1.StructuredCalendarSpec structured_calendar = 7;
*/
public java.util.List getStructuredCalendarList() {
if (structuredCalendarBuilder_ == null) {
return java.util.Collections.unmodifiableList(structuredCalendar_);
} else {
return structuredCalendarBuilder_.getMessageList();
}
}
/**
*
* Calendar-based specifications of times.
*
*
* repeated .temporal.api.schedule.v1.StructuredCalendarSpec structured_calendar = 7;
*/
public int getStructuredCalendarCount() {
if (structuredCalendarBuilder_ == null) {
return structuredCalendar_.size();
} else {
return structuredCalendarBuilder_.getCount();
}
}
/**
*
* Calendar-based specifications of times.
*
*
* repeated .temporal.api.schedule.v1.StructuredCalendarSpec structured_calendar = 7;
*/
public io.temporal.api.schedule.v1.StructuredCalendarSpec getStructuredCalendar(int index) {
if (structuredCalendarBuilder_ == null) {
return structuredCalendar_.get(index);
} else {
return structuredCalendarBuilder_.getMessage(index);
}
}
/**
*
* Calendar-based specifications of times.
*
*
* repeated .temporal.api.schedule.v1.StructuredCalendarSpec structured_calendar = 7;
*/
public Builder setStructuredCalendar(
int index, io.temporal.api.schedule.v1.StructuredCalendarSpec value) {
if (structuredCalendarBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureStructuredCalendarIsMutable();
structuredCalendar_.set(index, value);
onChanged();
} else {
structuredCalendarBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* Calendar-based specifications of times.
*
*
* repeated .temporal.api.schedule.v1.StructuredCalendarSpec structured_calendar = 7;
*/
public Builder setStructuredCalendar(
int index, io.temporal.api.schedule.v1.StructuredCalendarSpec.Builder builderForValue) {
if (structuredCalendarBuilder_ == null) {
ensureStructuredCalendarIsMutable();
structuredCalendar_.set(index, builderForValue.build());
onChanged();
} else {
structuredCalendarBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Calendar-based specifications of times.
*
*
* repeated .temporal.api.schedule.v1.StructuredCalendarSpec structured_calendar = 7;
*/
public Builder addStructuredCalendar(io.temporal.api.schedule.v1.StructuredCalendarSpec value) {
if (structuredCalendarBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureStructuredCalendarIsMutable();
structuredCalendar_.add(value);
onChanged();
} else {
structuredCalendarBuilder_.addMessage(value);
}
return this;
}
/**
*
* Calendar-based specifications of times.
*
*
* repeated .temporal.api.schedule.v1.StructuredCalendarSpec structured_calendar = 7;
*/
public Builder addStructuredCalendar(
int index, io.temporal.api.schedule.v1.StructuredCalendarSpec value) {
if (structuredCalendarBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureStructuredCalendarIsMutable();
structuredCalendar_.add(index, value);
onChanged();
} else {
structuredCalendarBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* Calendar-based specifications of times.
*
*
* repeated .temporal.api.schedule.v1.StructuredCalendarSpec structured_calendar = 7;
*/
public Builder addStructuredCalendar(
io.temporal.api.schedule.v1.StructuredCalendarSpec.Builder builderForValue) {
if (structuredCalendarBuilder_ == null) {
ensureStructuredCalendarIsMutable();
structuredCalendar_.add(builderForValue.build());
onChanged();
} else {
structuredCalendarBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* Calendar-based specifications of times.
*
*
* repeated .temporal.api.schedule.v1.StructuredCalendarSpec structured_calendar = 7;
*/
public Builder addStructuredCalendar(
int index, io.temporal.api.schedule.v1.StructuredCalendarSpec.Builder builderForValue) {
if (structuredCalendarBuilder_ == null) {
ensureStructuredCalendarIsMutable();
structuredCalendar_.add(index, builderForValue.build());
onChanged();
} else {
structuredCalendarBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Calendar-based specifications of times.
*
*
* repeated .temporal.api.schedule.v1.StructuredCalendarSpec structured_calendar = 7;
*/
public Builder addAllStructuredCalendar(
java.lang.Iterable extends io.temporal.api.schedule.v1.StructuredCalendarSpec> values) {
if (structuredCalendarBuilder_ == null) {
ensureStructuredCalendarIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, structuredCalendar_);
onChanged();
} else {
structuredCalendarBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* Calendar-based specifications of times.
*
*
* repeated .temporal.api.schedule.v1.StructuredCalendarSpec structured_calendar = 7;
*/
public Builder clearStructuredCalendar() {
if (structuredCalendarBuilder_ == null) {
structuredCalendar_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
structuredCalendarBuilder_.clear();
}
return this;
}
/**
*
* Calendar-based specifications of times.
*
*
* repeated .temporal.api.schedule.v1.StructuredCalendarSpec structured_calendar = 7;
*/
public Builder removeStructuredCalendar(int index) {
if (structuredCalendarBuilder_ == null) {
ensureStructuredCalendarIsMutable();
structuredCalendar_.remove(index);
onChanged();
} else {
structuredCalendarBuilder_.remove(index);
}
return this;
}
/**
*
* Calendar-based specifications of times.
*
*
* repeated .temporal.api.schedule.v1.StructuredCalendarSpec structured_calendar = 7;
*/
public io.temporal.api.schedule.v1.StructuredCalendarSpec.Builder getStructuredCalendarBuilder(
int index) {
return getStructuredCalendarFieldBuilder().getBuilder(index);
}
/**
*
* Calendar-based specifications of times.
*
*
* repeated .temporal.api.schedule.v1.StructuredCalendarSpec structured_calendar = 7;
*/
public io.temporal.api.schedule.v1.StructuredCalendarSpecOrBuilder getStructuredCalendarOrBuilder(
int index) {
if (structuredCalendarBuilder_ == null) {
return structuredCalendar_.get(index); } else {
return structuredCalendarBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* Calendar-based specifications of times.
*
*
* repeated .temporal.api.schedule.v1.StructuredCalendarSpec structured_calendar = 7;
*/
public java.util.List extends io.temporal.api.schedule.v1.StructuredCalendarSpecOrBuilder>
getStructuredCalendarOrBuilderList() {
if (structuredCalendarBuilder_ != null) {
return structuredCalendarBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(structuredCalendar_);
}
}
/**
*
* Calendar-based specifications of times.
*
*
* repeated .temporal.api.schedule.v1.StructuredCalendarSpec structured_calendar = 7;
*/
public io.temporal.api.schedule.v1.StructuredCalendarSpec.Builder addStructuredCalendarBuilder() {
return getStructuredCalendarFieldBuilder().addBuilder(
io.temporal.api.schedule.v1.StructuredCalendarSpec.getDefaultInstance());
}
/**
*
* Calendar-based specifications of times.
*
*
* repeated .temporal.api.schedule.v1.StructuredCalendarSpec structured_calendar = 7;
*/
public io.temporal.api.schedule.v1.StructuredCalendarSpec.Builder addStructuredCalendarBuilder(
int index) {
return getStructuredCalendarFieldBuilder().addBuilder(
index, io.temporal.api.schedule.v1.StructuredCalendarSpec.getDefaultInstance());
}
/**
*
* Calendar-based specifications of times.
*
*
* repeated .temporal.api.schedule.v1.StructuredCalendarSpec structured_calendar = 7;
*/
public java.util.List
getStructuredCalendarBuilderList() {
return getStructuredCalendarFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
io.temporal.api.schedule.v1.StructuredCalendarSpec, io.temporal.api.schedule.v1.StructuredCalendarSpec.Builder, io.temporal.api.schedule.v1.StructuredCalendarSpecOrBuilder>
getStructuredCalendarFieldBuilder() {
if (structuredCalendarBuilder_ == null) {
structuredCalendarBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
io.temporal.api.schedule.v1.StructuredCalendarSpec, io.temporal.api.schedule.v1.StructuredCalendarSpec.Builder, io.temporal.api.schedule.v1.StructuredCalendarSpecOrBuilder>(
structuredCalendar_,
((bitField0_ & 0x00000001) != 0),
getParentForChildren(),
isClean());
structuredCalendar_ = null;
}
return structuredCalendarBuilder_;
}
private com.google.protobuf.LazyStringList cronString_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureCronStringIsMutable() {
if (!((bitField0_ & 0x00000002) != 0)) {
cronString_ = new com.google.protobuf.LazyStringArrayList(cronString_);
bitField0_ |= 0x00000002;
}
}
/**
*
* cron_string holds a traditional cron specification as a string. It
* accepts 5, 6, or 7 fields, separated by spaces, and interprets them the
* same way as CalendarSpec.
* 5 fields: minute, hour, day_of_month, month, day_of_week
* 6 fields: minute, hour, day_of_month, month, day_of_week, year
* 7 fields: second, minute, hour, day_of_month, month, day_of_week, year
* If year is not given, it defaults to *. If second is not given, it
* defaults to 0.
* Shorthands @yearly, @monthly, @weekly, @daily, and @hourly are also
* accepted instead of the 5-7 time fields.
* Optionally, the string can be preceded by CRON_TZ=<timezone name> or
* TZ=<timezone name>, which will get copied to timezone_name. (There must
* not also be a timezone_name present.)
* Optionally "#" followed by a comment can appear at the end of the string.
* Note that the special case that some cron implementations have for
* treating day_of_month and day_of_week as "or" instead of "and" when both
* are set is not implemented.
* @every <interval>[/<phase>] is accepted and gets compiled into an
* IntervalSpec instead. <interval> and <phase> should be a decimal integer
* with a unit suffix s, m, h, or d.
*
*
* repeated string cron_string = 8;
* @return A list containing the cronString.
*/
public com.google.protobuf.ProtocolStringList
getCronStringList() {
return cronString_.getUnmodifiableView();
}
/**
*
* cron_string holds a traditional cron specification as a string. It
* accepts 5, 6, or 7 fields, separated by spaces, and interprets them the
* same way as CalendarSpec.
* 5 fields: minute, hour, day_of_month, month, day_of_week
* 6 fields: minute, hour, day_of_month, month, day_of_week, year
* 7 fields: second, minute, hour, day_of_month, month, day_of_week, year
* If year is not given, it defaults to *. If second is not given, it
* defaults to 0.
* Shorthands @yearly, @monthly, @weekly, @daily, and @hourly are also
* accepted instead of the 5-7 time fields.
* Optionally, the string can be preceded by CRON_TZ=<timezone name> or
* TZ=<timezone name>, which will get copied to timezone_name. (There must
* not also be a timezone_name present.)
* Optionally "#" followed by a comment can appear at the end of the string.
* Note that the special case that some cron implementations have for
* treating day_of_month and day_of_week as "or" instead of "and" when both
* are set is not implemented.
* @every <interval>[/<phase>] is accepted and gets compiled into an
* IntervalSpec instead. <interval> and <phase> should be a decimal integer
* with a unit suffix s, m, h, or d.
*
*
* repeated string cron_string = 8;
* @return The count of cronString.
*/
public int getCronStringCount() {
return cronString_.size();
}
/**
*
* cron_string holds a traditional cron specification as a string. It
* accepts 5, 6, or 7 fields, separated by spaces, and interprets them the
* same way as CalendarSpec.
* 5 fields: minute, hour, day_of_month, month, day_of_week
* 6 fields: minute, hour, day_of_month, month, day_of_week, year
* 7 fields: second, minute, hour, day_of_month, month, day_of_week, year
* If year is not given, it defaults to *. If second is not given, it
* defaults to 0.
* Shorthands @yearly, @monthly, @weekly, @daily, and @hourly are also
* accepted instead of the 5-7 time fields.
* Optionally, the string can be preceded by CRON_TZ=<timezone name> or
* TZ=<timezone name>, which will get copied to timezone_name. (There must
* not also be a timezone_name present.)
* Optionally "#" followed by a comment can appear at the end of the string.
* Note that the special case that some cron implementations have for
* treating day_of_month and day_of_week as "or" instead of "and" when both
* are set is not implemented.
* @every <interval>[/<phase>] is accepted and gets compiled into an
* IntervalSpec instead. <interval> and <phase> should be a decimal integer
* with a unit suffix s, m, h, or d.
*
*
* repeated string cron_string = 8;
* @param index The index of the element to return.
* @return The cronString at the given index.
*/
public java.lang.String getCronString(int index) {
return cronString_.get(index);
}
/**
*
* cron_string holds a traditional cron specification as a string. It
* accepts 5, 6, or 7 fields, separated by spaces, and interprets them the
* same way as CalendarSpec.
* 5 fields: minute, hour, day_of_month, month, day_of_week
* 6 fields: minute, hour, day_of_month, month, day_of_week, year
* 7 fields: second, minute, hour, day_of_month, month, day_of_week, year
* If year is not given, it defaults to *. If second is not given, it
* defaults to 0.
* Shorthands @yearly, @monthly, @weekly, @daily, and @hourly are also
* accepted instead of the 5-7 time fields.
* Optionally, the string can be preceded by CRON_TZ=<timezone name> or
* TZ=<timezone name>, which will get copied to timezone_name. (There must
* not also be a timezone_name present.)
* Optionally "#" followed by a comment can appear at the end of the string.
* Note that the special case that some cron implementations have for
* treating day_of_month and day_of_week as "or" instead of "and" when both
* are set is not implemented.
* @every <interval>[/<phase>] is accepted and gets compiled into an
* IntervalSpec instead. <interval> and <phase> should be a decimal integer
* with a unit suffix s, m, h, or d.
*
*
* repeated string cron_string = 8;
* @param index The index of the value to return.
* @return The bytes of the cronString at the given index.
*/
public com.google.protobuf.ByteString
getCronStringBytes(int index) {
return cronString_.getByteString(index);
}
/**
*
* cron_string holds a traditional cron specification as a string. It
* accepts 5, 6, or 7 fields, separated by spaces, and interprets them the
* same way as CalendarSpec.
* 5 fields: minute, hour, day_of_month, month, day_of_week
* 6 fields: minute, hour, day_of_month, month, day_of_week, year
* 7 fields: second, minute, hour, day_of_month, month, day_of_week, year
* If year is not given, it defaults to *. If second is not given, it
* defaults to 0.
* Shorthands @yearly, @monthly, @weekly, @daily, and @hourly are also
* accepted instead of the 5-7 time fields.
* Optionally, the string can be preceded by CRON_TZ=<timezone name> or
* TZ=<timezone name>, which will get copied to timezone_name. (There must
* not also be a timezone_name present.)
* Optionally "#" followed by a comment can appear at the end of the string.
* Note that the special case that some cron implementations have for
* treating day_of_month and day_of_week as "or" instead of "and" when both
* are set is not implemented.
* @every <interval>[/<phase>] is accepted and gets compiled into an
* IntervalSpec instead. <interval> and <phase> should be a decimal integer
* with a unit suffix s, m, h, or d.
*
*
* repeated string cron_string = 8;
* @param index The index to set the value at.
* @param value The cronString to set.
* @return This builder for chaining.
*/
public Builder setCronString(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureCronStringIsMutable();
cronString_.set(index, value);
onChanged();
return this;
}
/**
*
* cron_string holds a traditional cron specification as a string. It
* accepts 5, 6, or 7 fields, separated by spaces, and interprets them the
* same way as CalendarSpec.
* 5 fields: minute, hour, day_of_month, month, day_of_week
* 6 fields: minute, hour, day_of_month, month, day_of_week, year
* 7 fields: second, minute, hour, day_of_month, month, day_of_week, year
* If year is not given, it defaults to *. If second is not given, it
* defaults to 0.
* Shorthands @yearly, @monthly, @weekly, @daily, and @hourly are also
* accepted instead of the 5-7 time fields.
* Optionally, the string can be preceded by CRON_TZ=<timezone name> or
* TZ=<timezone name>, which will get copied to timezone_name. (There must
* not also be a timezone_name present.)
* Optionally "#" followed by a comment can appear at the end of the string.
* Note that the special case that some cron implementations have for
* treating day_of_month and day_of_week as "or" instead of "and" when both
* are set is not implemented.
* @every <interval>[/<phase>] is accepted and gets compiled into an
* IntervalSpec instead. <interval> and <phase> should be a decimal integer
* with a unit suffix s, m, h, or d.
*
*
* repeated string cron_string = 8;
* @param value The cronString to add.
* @return This builder for chaining.
*/
public Builder addCronString(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureCronStringIsMutable();
cronString_.add(value);
onChanged();
return this;
}
/**
*
* cron_string holds a traditional cron specification as a string. It
* accepts 5, 6, or 7 fields, separated by spaces, and interprets them the
* same way as CalendarSpec.
* 5 fields: minute, hour, day_of_month, month, day_of_week
* 6 fields: minute, hour, day_of_month, month, day_of_week, year
* 7 fields: second, minute, hour, day_of_month, month, day_of_week, year
* If year is not given, it defaults to *. If second is not given, it
* defaults to 0.
* Shorthands @yearly, @monthly, @weekly, @daily, and @hourly are also
* accepted instead of the 5-7 time fields.
* Optionally, the string can be preceded by CRON_TZ=<timezone name> or
* TZ=<timezone name>, which will get copied to timezone_name. (There must
* not also be a timezone_name present.)
* Optionally "#" followed by a comment can appear at the end of the string.
* Note that the special case that some cron implementations have for
* treating day_of_month and day_of_week as "or" instead of "and" when both
* are set is not implemented.
* @every <interval>[/<phase>] is accepted and gets compiled into an
* IntervalSpec instead. <interval> and <phase> should be a decimal integer
* with a unit suffix s, m, h, or d.
*
*
* repeated string cron_string = 8;
* @param values The cronString to add.
* @return This builder for chaining.
*/
public Builder addAllCronString(
java.lang.Iterable values) {
ensureCronStringIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, cronString_);
onChanged();
return this;
}
/**
*
* cron_string holds a traditional cron specification as a string. It
* accepts 5, 6, or 7 fields, separated by spaces, and interprets them the
* same way as CalendarSpec.
* 5 fields: minute, hour, day_of_month, month, day_of_week
* 6 fields: minute, hour, day_of_month, month, day_of_week, year
* 7 fields: second, minute, hour, day_of_month, month, day_of_week, year
* If year is not given, it defaults to *. If second is not given, it
* defaults to 0.
* Shorthands @yearly, @monthly, @weekly, @daily, and @hourly are also
* accepted instead of the 5-7 time fields.
* Optionally, the string can be preceded by CRON_TZ=<timezone name> or
* TZ=<timezone name>, which will get copied to timezone_name. (There must
* not also be a timezone_name present.)
* Optionally "#" followed by a comment can appear at the end of the string.
* Note that the special case that some cron implementations have for
* treating day_of_month and day_of_week as "or" instead of "and" when both
* are set is not implemented.
* @every <interval>[/<phase>] is accepted and gets compiled into an
* IntervalSpec instead. <interval> and <phase> should be a decimal integer
* with a unit suffix s, m, h, or d.
*
*
* repeated string cron_string = 8;
* @return This builder for chaining.
*/
public Builder clearCronString() {
cronString_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
*
* cron_string holds a traditional cron specification as a string. It
* accepts 5, 6, or 7 fields, separated by spaces, and interprets them the
* same way as CalendarSpec.
* 5 fields: minute, hour, day_of_month, month, day_of_week
* 6 fields: minute, hour, day_of_month, month, day_of_week, year
* 7 fields: second, minute, hour, day_of_month, month, day_of_week, year
* If year is not given, it defaults to *. If second is not given, it
* defaults to 0.
* Shorthands @yearly, @monthly, @weekly, @daily, and @hourly are also
* accepted instead of the 5-7 time fields.
* Optionally, the string can be preceded by CRON_TZ=<timezone name> or
* TZ=<timezone name>, which will get copied to timezone_name. (There must
* not also be a timezone_name present.)
* Optionally "#" followed by a comment can appear at the end of the string.
* Note that the special case that some cron implementations have for
* treating day_of_month and day_of_week as "or" instead of "and" when both
* are set is not implemented.
* @every <interval>[/<phase>] is accepted and gets compiled into an
* IntervalSpec instead. <interval> and <phase> should be a decimal integer
* with a unit suffix s, m, h, or d.
*
*
* repeated string cron_string = 8;
* @param value The bytes of the cronString to add.
* @return This builder for chaining.
*/
public Builder addCronStringBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensureCronStringIsMutable();
cronString_.add(value);
onChanged();
return this;
}
private java.util.List calendar_ =
java.util.Collections.emptyList();
private void ensureCalendarIsMutable() {
if (!((bitField0_ & 0x00000004) != 0)) {
calendar_ = new java.util.ArrayList(calendar_);
bitField0_ |= 0x00000004;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
io.temporal.api.schedule.v1.CalendarSpec, io.temporal.api.schedule.v1.CalendarSpec.Builder, io.temporal.api.schedule.v1.CalendarSpecOrBuilder> calendarBuilder_;
/**
*
* Calendar-based specifications of times.
*
*
* repeated .temporal.api.schedule.v1.CalendarSpec calendar = 1;
*/
public java.util.List getCalendarList() {
if (calendarBuilder_ == null) {
return java.util.Collections.unmodifiableList(calendar_);
} else {
return calendarBuilder_.getMessageList();
}
}
/**
*
* Calendar-based specifications of times.
*
*
* repeated .temporal.api.schedule.v1.CalendarSpec calendar = 1;
*/
public int getCalendarCount() {
if (calendarBuilder_ == null) {
return calendar_.size();
} else {
return calendarBuilder_.getCount();
}
}
/**
*
* Calendar-based specifications of times.
*
*
* repeated .temporal.api.schedule.v1.CalendarSpec calendar = 1;
*/
public io.temporal.api.schedule.v1.CalendarSpec getCalendar(int index) {
if (calendarBuilder_ == null) {
return calendar_.get(index);
} else {
return calendarBuilder_.getMessage(index);
}
}
/**
*
* Calendar-based specifications of times.
*
*
* repeated .temporal.api.schedule.v1.CalendarSpec calendar = 1;
*/
public Builder setCalendar(
int index, io.temporal.api.schedule.v1.CalendarSpec value) {
if (calendarBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureCalendarIsMutable();
calendar_.set(index, value);
onChanged();
} else {
calendarBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* Calendar-based specifications of times.
*
*
* repeated .temporal.api.schedule.v1.CalendarSpec calendar = 1;
*/
public Builder setCalendar(
int index, io.temporal.api.schedule.v1.CalendarSpec.Builder builderForValue) {
if (calendarBuilder_ == null) {
ensureCalendarIsMutable();
calendar_.set(index, builderForValue.build());
onChanged();
} else {
calendarBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Calendar-based specifications of times.
*
*
* repeated .temporal.api.schedule.v1.CalendarSpec calendar = 1;
*/
public Builder addCalendar(io.temporal.api.schedule.v1.CalendarSpec value) {
if (calendarBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureCalendarIsMutable();
calendar_.add(value);
onChanged();
} else {
calendarBuilder_.addMessage(value);
}
return this;
}
/**
*
* Calendar-based specifications of times.
*
*
* repeated .temporal.api.schedule.v1.CalendarSpec calendar = 1;
*/
public Builder addCalendar(
int index, io.temporal.api.schedule.v1.CalendarSpec value) {
if (calendarBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureCalendarIsMutable();
calendar_.add(index, value);
onChanged();
} else {
calendarBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* Calendar-based specifications of times.
*
*
* repeated .temporal.api.schedule.v1.CalendarSpec calendar = 1;
*/
public Builder addCalendar(
io.temporal.api.schedule.v1.CalendarSpec.Builder builderForValue) {
if (calendarBuilder_ == null) {
ensureCalendarIsMutable();
calendar_.add(builderForValue.build());
onChanged();
} else {
calendarBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* Calendar-based specifications of times.
*
*
* repeated .temporal.api.schedule.v1.CalendarSpec calendar = 1;
*/
public Builder addCalendar(
int index, io.temporal.api.schedule.v1.CalendarSpec.Builder builderForValue) {
if (calendarBuilder_ == null) {
ensureCalendarIsMutable();
calendar_.add(index, builderForValue.build());
onChanged();
} else {
calendarBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Calendar-based specifications of times.
*
*
* repeated .temporal.api.schedule.v1.CalendarSpec calendar = 1;
*/
public Builder addAllCalendar(
java.lang.Iterable extends io.temporal.api.schedule.v1.CalendarSpec> values) {
if (calendarBuilder_ == null) {
ensureCalendarIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, calendar_);
onChanged();
} else {
calendarBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* Calendar-based specifications of times.
*
*
* repeated .temporal.api.schedule.v1.CalendarSpec calendar = 1;
*/
public Builder clearCalendar() {
if (calendarBuilder_ == null) {
calendar_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
} else {
calendarBuilder_.clear();
}
return this;
}
/**
*
* Calendar-based specifications of times.
*
*
* repeated .temporal.api.schedule.v1.CalendarSpec calendar = 1;
*/
public Builder removeCalendar(int index) {
if (calendarBuilder_ == null) {
ensureCalendarIsMutable();
calendar_.remove(index);
onChanged();
} else {
calendarBuilder_.remove(index);
}
return this;
}
/**
*
* Calendar-based specifications of times.
*
*
* repeated .temporal.api.schedule.v1.CalendarSpec calendar = 1;
*/
public io.temporal.api.schedule.v1.CalendarSpec.Builder getCalendarBuilder(
int index) {
return getCalendarFieldBuilder().getBuilder(index);
}
/**
*
* Calendar-based specifications of times.
*
*
* repeated .temporal.api.schedule.v1.CalendarSpec calendar = 1;
*/
public io.temporal.api.schedule.v1.CalendarSpecOrBuilder getCalendarOrBuilder(
int index) {
if (calendarBuilder_ == null) {
return calendar_.get(index); } else {
return calendarBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* Calendar-based specifications of times.
*
*
* repeated .temporal.api.schedule.v1.CalendarSpec calendar = 1;
*/
public java.util.List extends io.temporal.api.schedule.v1.CalendarSpecOrBuilder>
getCalendarOrBuilderList() {
if (calendarBuilder_ != null) {
return calendarBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(calendar_);
}
}
/**
*
* Calendar-based specifications of times.
*
*
* repeated .temporal.api.schedule.v1.CalendarSpec calendar = 1;
*/
public io.temporal.api.schedule.v1.CalendarSpec.Builder addCalendarBuilder() {
return getCalendarFieldBuilder().addBuilder(
io.temporal.api.schedule.v1.CalendarSpec.getDefaultInstance());
}
/**
*
* Calendar-based specifications of times.
*
*
* repeated .temporal.api.schedule.v1.CalendarSpec calendar = 1;
*/
public io.temporal.api.schedule.v1.CalendarSpec.Builder addCalendarBuilder(
int index) {
return getCalendarFieldBuilder().addBuilder(
index, io.temporal.api.schedule.v1.CalendarSpec.getDefaultInstance());
}
/**
*
* Calendar-based specifications of times.
*
*
* repeated .temporal.api.schedule.v1.CalendarSpec calendar = 1;
*/
public java.util.List
getCalendarBuilderList() {
return getCalendarFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
io.temporal.api.schedule.v1.CalendarSpec, io.temporal.api.schedule.v1.CalendarSpec.Builder, io.temporal.api.schedule.v1.CalendarSpecOrBuilder>
getCalendarFieldBuilder() {
if (calendarBuilder_ == null) {
calendarBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
io.temporal.api.schedule.v1.CalendarSpec, io.temporal.api.schedule.v1.CalendarSpec.Builder, io.temporal.api.schedule.v1.CalendarSpecOrBuilder>(
calendar_,
((bitField0_ & 0x00000004) != 0),
getParentForChildren(),
isClean());
calendar_ = null;
}
return calendarBuilder_;
}
private java.util.List interval_ =
java.util.Collections.emptyList();
private void ensureIntervalIsMutable() {
if (!((bitField0_ & 0x00000008) != 0)) {
interval_ = new java.util.ArrayList(interval_);
bitField0_ |= 0x00000008;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
io.temporal.api.schedule.v1.IntervalSpec, io.temporal.api.schedule.v1.IntervalSpec.Builder, io.temporal.api.schedule.v1.IntervalSpecOrBuilder> intervalBuilder_;
/**
*
* Interval-based specifications of times.
*
*
* repeated .temporal.api.schedule.v1.IntervalSpec interval = 2;
*/
public java.util.List getIntervalList() {
if (intervalBuilder_ == null) {
return java.util.Collections.unmodifiableList(interval_);
} else {
return intervalBuilder_.getMessageList();
}
}
/**
*
* Interval-based specifications of times.
*
*
* repeated .temporal.api.schedule.v1.IntervalSpec interval = 2;
*/
public int getIntervalCount() {
if (intervalBuilder_ == null) {
return interval_.size();
} else {
return intervalBuilder_.getCount();
}
}
/**
*
* Interval-based specifications of times.
*
*
* repeated .temporal.api.schedule.v1.IntervalSpec interval = 2;
*/
public io.temporal.api.schedule.v1.IntervalSpec getInterval(int index) {
if (intervalBuilder_ == null) {
return interval_.get(index);
} else {
return intervalBuilder_.getMessage(index);
}
}
/**
*
* Interval-based specifications of times.
*
*
* repeated .temporal.api.schedule.v1.IntervalSpec interval = 2;
*/
public Builder setInterval(
int index, io.temporal.api.schedule.v1.IntervalSpec value) {
if (intervalBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureIntervalIsMutable();
interval_.set(index, value);
onChanged();
} else {
intervalBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* Interval-based specifications of times.
*
*
* repeated .temporal.api.schedule.v1.IntervalSpec interval = 2;
*/
public Builder setInterval(
int index, io.temporal.api.schedule.v1.IntervalSpec.Builder builderForValue) {
if (intervalBuilder_ == null) {
ensureIntervalIsMutable();
interval_.set(index, builderForValue.build());
onChanged();
} else {
intervalBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Interval-based specifications of times.
*
*
* repeated .temporal.api.schedule.v1.IntervalSpec interval = 2;
*/
public Builder addInterval(io.temporal.api.schedule.v1.IntervalSpec value) {
if (intervalBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureIntervalIsMutable();
interval_.add(value);
onChanged();
} else {
intervalBuilder_.addMessage(value);
}
return this;
}
/**
*
* Interval-based specifications of times.
*
*
* repeated .temporal.api.schedule.v1.IntervalSpec interval = 2;
*/
public Builder addInterval(
int index, io.temporal.api.schedule.v1.IntervalSpec value) {
if (intervalBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureIntervalIsMutable();
interval_.add(index, value);
onChanged();
} else {
intervalBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* Interval-based specifications of times.
*
*
* repeated .temporal.api.schedule.v1.IntervalSpec interval = 2;
*/
public Builder addInterval(
io.temporal.api.schedule.v1.IntervalSpec.Builder builderForValue) {
if (intervalBuilder_ == null) {
ensureIntervalIsMutable();
interval_.add(builderForValue.build());
onChanged();
} else {
intervalBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* Interval-based specifications of times.
*
*
* repeated .temporal.api.schedule.v1.IntervalSpec interval = 2;
*/
public Builder addInterval(
int index, io.temporal.api.schedule.v1.IntervalSpec.Builder builderForValue) {
if (intervalBuilder_ == null) {
ensureIntervalIsMutable();
interval_.add(index, builderForValue.build());
onChanged();
} else {
intervalBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Interval-based specifications of times.
*
*
* repeated .temporal.api.schedule.v1.IntervalSpec interval = 2;
*/
public Builder addAllInterval(
java.lang.Iterable extends io.temporal.api.schedule.v1.IntervalSpec> values) {
if (intervalBuilder_ == null) {
ensureIntervalIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, interval_);
onChanged();
} else {
intervalBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* Interval-based specifications of times.
*
*
* repeated .temporal.api.schedule.v1.IntervalSpec interval = 2;
*/
public Builder clearInterval() {
if (intervalBuilder_ == null) {
interval_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000008);
onChanged();
} else {
intervalBuilder_.clear();
}
return this;
}
/**
*
* Interval-based specifications of times.
*
*
* repeated .temporal.api.schedule.v1.IntervalSpec interval = 2;
*/
public Builder removeInterval(int index) {
if (intervalBuilder_ == null) {
ensureIntervalIsMutable();
interval_.remove(index);
onChanged();
} else {
intervalBuilder_.remove(index);
}
return this;
}
/**
*
* Interval-based specifications of times.
*
*
* repeated .temporal.api.schedule.v1.IntervalSpec interval = 2;
*/
public io.temporal.api.schedule.v1.IntervalSpec.Builder getIntervalBuilder(
int index) {
return getIntervalFieldBuilder().getBuilder(index);
}
/**
*
* Interval-based specifications of times.
*
*
* repeated .temporal.api.schedule.v1.IntervalSpec interval = 2;
*/
public io.temporal.api.schedule.v1.IntervalSpecOrBuilder getIntervalOrBuilder(
int index) {
if (intervalBuilder_ == null) {
return interval_.get(index); } else {
return intervalBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* Interval-based specifications of times.
*
*
* repeated .temporal.api.schedule.v1.IntervalSpec interval = 2;
*/
public java.util.List extends io.temporal.api.schedule.v1.IntervalSpecOrBuilder>
getIntervalOrBuilderList() {
if (intervalBuilder_ != null) {
return intervalBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(interval_);
}
}
/**
*
* Interval-based specifications of times.
*
*
* repeated .temporal.api.schedule.v1.IntervalSpec interval = 2;
*/
public io.temporal.api.schedule.v1.IntervalSpec.Builder addIntervalBuilder() {
return getIntervalFieldBuilder().addBuilder(
io.temporal.api.schedule.v1.IntervalSpec.getDefaultInstance());
}
/**
*
* Interval-based specifications of times.
*
*
* repeated .temporal.api.schedule.v1.IntervalSpec interval = 2;
*/
public io.temporal.api.schedule.v1.IntervalSpec.Builder addIntervalBuilder(
int index) {
return getIntervalFieldBuilder().addBuilder(
index, io.temporal.api.schedule.v1.IntervalSpec.getDefaultInstance());
}
/**
*
* Interval-based specifications of times.
*
*
* repeated .temporal.api.schedule.v1.IntervalSpec interval = 2;
*/
public java.util.List
getIntervalBuilderList() {
return getIntervalFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
io.temporal.api.schedule.v1.IntervalSpec, io.temporal.api.schedule.v1.IntervalSpec.Builder, io.temporal.api.schedule.v1.IntervalSpecOrBuilder>
getIntervalFieldBuilder() {
if (intervalBuilder_ == null) {
intervalBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
io.temporal.api.schedule.v1.IntervalSpec, io.temporal.api.schedule.v1.IntervalSpec.Builder, io.temporal.api.schedule.v1.IntervalSpecOrBuilder>(
interval_,
((bitField0_ & 0x00000008) != 0),
getParentForChildren(),
isClean());
interval_ = null;
}
return intervalBuilder_;
}
private java.util.List excludeCalendar_ =
java.util.Collections.emptyList();
private void ensureExcludeCalendarIsMutable() {
if (!((bitField0_ & 0x00000010) != 0)) {
excludeCalendar_ = new java.util.ArrayList(excludeCalendar_);
bitField0_ |= 0x00000010;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
io.temporal.api.schedule.v1.CalendarSpec, io.temporal.api.schedule.v1.CalendarSpec.Builder, io.temporal.api.schedule.v1.CalendarSpecOrBuilder> excludeCalendarBuilder_;
/**
*
* Any timestamps matching any of exclude_* will be skipped.
*
*
* repeated .temporal.api.schedule.v1.CalendarSpec exclude_calendar = 3 [deprecated = true];
*/
@java.lang.Deprecated public java.util.List getExcludeCalendarList() {
if (excludeCalendarBuilder_ == null) {
return java.util.Collections.unmodifiableList(excludeCalendar_);
} else {
return excludeCalendarBuilder_.getMessageList();
}
}
/**
*
* Any timestamps matching any of exclude_* will be skipped.
*
*
* repeated .temporal.api.schedule.v1.CalendarSpec exclude_calendar = 3 [deprecated = true];
*/
@java.lang.Deprecated public int getExcludeCalendarCount() {
if (excludeCalendarBuilder_ == null) {
return excludeCalendar_.size();
} else {
return excludeCalendarBuilder_.getCount();
}
}
/**
*
* Any timestamps matching any of exclude_* will be skipped.
*
*
* repeated .temporal.api.schedule.v1.CalendarSpec exclude_calendar = 3 [deprecated = true];
*/
@java.lang.Deprecated public io.temporal.api.schedule.v1.CalendarSpec getExcludeCalendar(int index) {
if (excludeCalendarBuilder_ == null) {
return excludeCalendar_.get(index);
} else {
return excludeCalendarBuilder_.getMessage(index);
}
}
/**
*
* Any timestamps matching any of exclude_* will be skipped.
*
*
* repeated .temporal.api.schedule.v1.CalendarSpec exclude_calendar = 3 [deprecated = true];
*/
@java.lang.Deprecated public Builder setExcludeCalendar(
int index, io.temporal.api.schedule.v1.CalendarSpec value) {
if (excludeCalendarBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureExcludeCalendarIsMutable();
excludeCalendar_.set(index, value);
onChanged();
} else {
excludeCalendarBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* Any timestamps matching any of exclude_* will be skipped.
*
*
* repeated .temporal.api.schedule.v1.CalendarSpec exclude_calendar = 3 [deprecated = true];
*/
@java.lang.Deprecated public Builder setExcludeCalendar(
int index, io.temporal.api.schedule.v1.CalendarSpec.Builder builderForValue) {
if (excludeCalendarBuilder_ == null) {
ensureExcludeCalendarIsMutable();
excludeCalendar_.set(index, builderForValue.build());
onChanged();
} else {
excludeCalendarBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Any timestamps matching any of exclude_* will be skipped.
*
*
* repeated .temporal.api.schedule.v1.CalendarSpec exclude_calendar = 3 [deprecated = true];
*/
@java.lang.Deprecated public Builder addExcludeCalendar(io.temporal.api.schedule.v1.CalendarSpec value) {
if (excludeCalendarBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureExcludeCalendarIsMutable();
excludeCalendar_.add(value);
onChanged();
} else {
excludeCalendarBuilder_.addMessage(value);
}
return this;
}
/**
*
* Any timestamps matching any of exclude_* will be skipped.
*
*
* repeated .temporal.api.schedule.v1.CalendarSpec exclude_calendar = 3 [deprecated = true];
*/
@java.lang.Deprecated public Builder addExcludeCalendar(
int index, io.temporal.api.schedule.v1.CalendarSpec value) {
if (excludeCalendarBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureExcludeCalendarIsMutable();
excludeCalendar_.add(index, value);
onChanged();
} else {
excludeCalendarBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* Any timestamps matching any of exclude_* will be skipped.
*
*
* repeated .temporal.api.schedule.v1.CalendarSpec exclude_calendar = 3 [deprecated = true];
*/
@java.lang.Deprecated public Builder addExcludeCalendar(
io.temporal.api.schedule.v1.CalendarSpec.Builder builderForValue) {
if (excludeCalendarBuilder_ == null) {
ensureExcludeCalendarIsMutable();
excludeCalendar_.add(builderForValue.build());
onChanged();
} else {
excludeCalendarBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* Any timestamps matching any of exclude_* will be skipped.
*
*
* repeated .temporal.api.schedule.v1.CalendarSpec exclude_calendar = 3 [deprecated = true];
*/
@java.lang.Deprecated public Builder addExcludeCalendar(
int index, io.temporal.api.schedule.v1.CalendarSpec.Builder builderForValue) {
if (excludeCalendarBuilder_ == null) {
ensureExcludeCalendarIsMutable();
excludeCalendar_.add(index, builderForValue.build());
onChanged();
} else {
excludeCalendarBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Any timestamps matching any of exclude_* will be skipped.
*
*
* repeated .temporal.api.schedule.v1.CalendarSpec exclude_calendar = 3 [deprecated = true];
*/
@java.lang.Deprecated public Builder addAllExcludeCalendar(
java.lang.Iterable extends io.temporal.api.schedule.v1.CalendarSpec> values) {
if (excludeCalendarBuilder_ == null) {
ensureExcludeCalendarIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, excludeCalendar_);
onChanged();
} else {
excludeCalendarBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* Any timestamps matching any of exclude_* will be skipped.
*
*
* repeated .temporal.api.schedule.v1.CalendarSpec exclude_calendar = 3 [deprecated = true];
*/
@java.lang.Deprecated public Builder clearExcludeCalendar() {
if (excludeCalendarBuilder_ == null) {
excludeCalendar_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000010);
onChanged();
} else {
excludeCalendarBuilder_.clear();
}
return this;
}
/**
*
* Any timestamps matching any of exclude_* will be skipped.
*
*
* repeated .temporal.api.schedule.v1.CalendarSpec exclude_calendar = 3 [deprecated = true];
*/
@java.lang.Deprecated public Builder removeExcludeCalendar(int index) {
if (excludeCalendarBuilder_ == null) {
ensureExcludeCalendarIsMutable();
excludeCalendar_.remove(index);
onChanged();
} else {
excludeCalendarBuilder_.remove(index);
}
return this;
}
/**
*
* Any timestamps matching any of exclude_* will be skipped.
*
*
* repeated .temporal.api.schedule.v1.CalendarSpec exclude_calendar = 3 [deprecated = true];
*/
@java.lang.Deprecated public io.temporal.api.schedule.v1.CalendarSpec.Builder getExcludeCalendarBuilder(
int index) {
return getExcludeCalendarFieldBuilder().getBuilder(index);
}
/**
*
* Any timestamps matching any of exclude_* will be skipped.
*
*
* repeated .temporal.api.schedule.v1.CalendarSpec exclude_calendar = 3 [deprecated = true];
*/
@java.lang.Deprecated public io.temporal.api.schedule.v1.CalendarSpecOrBuilder getExcludeCalendarOrBuilder(
int index) {
if (excludeCalendarBuilder_ == null) {
return excludeCalendar_.get(index); } else {
return excludeCalendarBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* Any timestamps matching any of exclude_* will be skipped.
*
*
* repeated .temporal.api.schedule.v1.CalendarSpec exclude_calendar = 3 [deprecated = true];
*/
@java.lang.Deprecated public java.util.List extends io.temporal.api.schedule.v1.CalendarSpecOrBuilder>
getExcludeCalendarOrBuilderList() {
if (excludeCalendarBuilder_ != null) {
return excludeCalendarBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(excludeCalendar_);
}
}
/**
*
* Any timestamps matching any of exclude_* will be skipped.
*
*
* repeated .temporal.api.schedule.v1.CalendarSpec exclude_calendar = 3 [deprecated = true];
*/
@java.lang.Deprecated public io.temporal.api.schedule.v1.CalendarSpec.Builder addExcludeCalendarBuilder() {
return getExcludeCalendarFieldBuilder().addBuilder(
io.temporal.api.schedule.v1.CalendarSpec.getDefaultInstance());
}
/**
*
* Any timestamps matching any of exclude_* will be skipped.
*
*
* repeated .temporal.api.schedule.v1.CalendarSpec exclude_calendar = 3 [deprecated = true];
*/
@java.lang.Deprecated public io.temporal.api.schedule.v1.CalendarSpec.Builder addExcludeCalendarBuilder(
int index) {
return getExcludeCalendarFieldBuilder().addBuilder(
index, io.temporal.api.schedule.v1.CalendarSpec.getDefaultInstance());
}
/**
*
* Any timestamps matching any of exclude_* will be skipped.
*
*
* repeated .temporal.api.schedule.v1.CalendarSpec exclude_calendar = 3 [deprecated = true];
*/
@java.lang.Deprecated public java.util.List
getExcludeCalendarBuilderList() {
return getExcludeCalendarFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
io.temporal.api.schedule.v1.CalendarSpec, io.temporal.api.schedule.v1.CalendarSpec.Builder, io.temporal.api.schedule.v1.CalendarSpecOrBuilder>
getExcludeCalendarFieldBuilder() {
if (excludeCalendarBuilder_ == null) {
excludeCalendarBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
io.temporal.api.schedule.v1.CalendarSpec, io.temporal.api.schedule.v1.CalendarSpec.Builder, io.temporal.api.schedule.v1.CalendarSpecOrBuilder>(
excludeCalendar_,
((bitField0_ & 0x00000010) != 0),
getParentForChildren(),
isClean());
excludeCalendar_ = null;
}
return excludeCalendarBuilder_;
}
private java.util.List excludeStructuredCalendar_ =
java.util.Collections.emptyList();
private void ensureExcludeStructuredCalendarIsMutable() {
if (!((bitField0_ & 0x00000020) != 0)) {
excludeStructuredCalendar_ = new java.util.ArrayList(excludeStructuredCalendar_);
bitField0_ |= 0x00000020;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
io.temporal.api.schedule.v1.StructuredCalendarSpec, io.temporal.api.schedule.v1.StructuredCalendarSpec.Builder, io.temporal.api.schedule.v1.StructuredCalendarSpecOrBuilder> excludeStructuredCalendarBuilder_;
/**
* repeated .temporal.api.schedule.v1.StructuredCalendarSpec exclude_structured_calendar = 9;
*/
public java.util.List getExcludeStructuredCalendarList() {
if (excludeStructuredCalendarBuilder_ == null) {
return java.util.Collections.unmodifiableList(excludeStructuredCalendar_);
} else {
return excludeStructuredCalendarBuilder_.getMessageList();
}
}
/**
* repeated .temporal.api.schedule.v1.StructuredCalendarSpec exclude_structured_calendar = 9;
*/
public int getExcludeStructuredCalendarCount() {
if (excludeStructuredCalendarBuilder_ == null) {
return excludeStructuredCalendar_.size();
} else {
return excludeStructuredCalendarBuilder_.getCount();
}
}
/**
* repeated .temporal.api.schedule.v1.StructuredCalendarSpec exclude_structured_calendar = 9;
*/
public io.temporal.api.schedule.v1.StructuredCalendarSpec getExcludeStructuredCalendar(int index) {
if (excludeStructuredCalendarBuilder_ == null) {
return excludeStructuredCalendar_.get(index);
} else {
return excludeStructuredCalendarBuilder_.getMessage(index);
}
}
/**
* repeated .temporal.api.schedule.v1.StructuredCalendarSpec exclude_structured_calendar = 9;
*/
public Builder setExcludeStructuredCalendar(
int index, io.temporal.api.schedule.v1.StructuredCalendarSpec value) {
if (excludeStructuredCalendarBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureExcludeStructuredCalendarIsMutable();
excludeStructuredCalendar_.set(index, value);
onChanged();
} else {
excludeStructuredCalendarBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .temporal.api.schedule.v1.StructuredCalendarSpec exclude_structured_calendar = 9;
*/
public Builder setExcludeStructuredCalendar(
int index, io.temporal.api.schedule.v1.StructuredCalendarSpec.Builder builderForValue) {
if (excludeStructuredCalendarBuilder_ == null) {
ensureExcludeStructuredCalendarIsMutable();
excludeStructuredCalendar_.set(index, builderForValue.build());
onChanged();
} else {
excludeStructuredCalendarBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .temporal.api.schedule.v1.StructuredCalendarSpec exclude_structured_calendar = 9;
*/
public Builder addExcludeStructuredCalendar(io.temporal.api.schedule.v1.StructuredCalendarSpec value) {
if (excludeStructuredCalendarBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureExcludeStructuredCalendarIsMutable();
excludeStructuredCalendar_.add(value);
onChanged();
} else {
excludeStructuredCalendarBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .temporal.api.schedule.v1.StructuredCalendarSpec exclude_structured_calendar = 9;
*/
public Builder addExcludeStructuredCalendar(
int index, io.temporal.api.schedule.v1.StructuredCalendarSpec value) {
if (excludeStructuredCalendarBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureExcludeStructuredCalendarIsMutable();
excludeStructuredCalendar_.add(index, value);
onChanged();
} else {
excludeStructuredCalendarBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .temporal.api.schedule.v1.StructuredCalendarSpec exclude_structured_calendar = 9;
*/
public Builder addExcludeStructuredCalendar(
io.temporal.api.schedule.v1.StructuredCalendarSpec.Builder builderForValue) {
if (excludeStructuredCalendarBuilder_ == null) {
ensureExcludeStructuredCalendarIsMutable();
excludeStructuredCalendar_.add(builderForValue.build());
onChanged();
} else {
excludeStructuredCalendarBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .temporal.api.schedule.v1.StructuredCalendarSpec exclude_structured_calendar = 9;
*/
public Builder addExcludeStructuredCalendar(
int index, io.temporal.api.schedule.v1.StructuredCalendarSpec.Builder builderForValue) {
if (excludeStructuredCalendarBuilder_ == null) {
ensureExcludeStructuredCalendarIsMutable();
excludeStructuredCalendar_.add(index, builderForValue.build());
onChanged();
} else {
excludeStructuredCalendarBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .temporal.api.schedule.v1.StructuredCalendarSpec exclude_structured_calendar = 9;
*/
public Builder addAllExcludeStructuredCalendar(
java.lang.Iterable extends io.temporal.api.schedule.v1.StructuredCalendarSpec> values) {
if (excludeStructuredCalendarBuilder_ == null) {
ensureExcludeStructuredCalendarIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, excludeStructuredCalendar_);
onChanged();
} else {
excludeStructuredCalendarBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .temporal.api.schedule.v1.StructuredCalendarSpec exclude_structured_calendar = 9;
*/
public Builder clearExcludeStructuredCalendar() {
if (excludeStructuredCalendarBuilder_ == null) {
excludeStructuredCalendar_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000020);
onChanged();
} else {
excludeStructuredCalendarBuilder_.clear();
}
return this;
}
/**
* repeated .temporal.api.schedule.v1.StructuredCalendarSpec exclude_structured_calendar = 9;
*/
public Builder removeExcludeStructuredCalendar(int index) {
if (excludeStructuredCalendarBuilder_ == null) {
ensureExcludeStructuredCalendarIsMutable();
excludeStructuredCalendar_.remove(index);
onChanged();
} else {
excludeStructuredCalendarBuilder_.remove(index);
}
return this;
}
/**
* repeated .temporal.api.schedule.v1.StructuredCalendarSpec exclude_structured_calendar = 9;
*/
public io.temporal.api.schedule.v1.StructuredCalendarSpec.Builder getExcludeStructuredCalendarBuilder(
int index) {
return getExcludeStructuredCalendarFieldBuilder().getBuilder(index);
}
/**
* repeated .temporal.api.schedule.v1.StructuredCalendarSpec exclude_structured_calendar = 9;
*/
public io.temporal.api.schedule.v1.StructuredCalendarSpecOrBuilder getExcludeStructuredCalendarOrBuilder(
int index) {
if (excludeStructuredCalendarBuilder_ == null) {
return excludeStructuredCalendar_.get(index); } else {
return excludeStructuredCalendarBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .temporal.api.schedule.v1.StructuredCalendarSpec exclude_structured_calendar = 9;
*/
public java.util.List extends io.temporal.api.schedule.v1.StructuredCalendarSpecOrBuilder>
getExcludeStructuredCalendarOrBuilderList() {
if (excludeStructuredCalendarBuilder_ != null) {
return excludeStructuredCalendarBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(excludeStructuredCalendar_);
}
}
/**
* repeated .temporal.api.schedule.v1.StructuredCalendarSpec exclude_structured_calendar = 9;
*/
public io.temporal.api.schedule.v1.StructuredCalendarSpec.Builder addExcludeStructuredCalendarBuilder() {
return getExcludeStructuredCalendarFieldBuilder().addBuilder(
io.temporal.api.schedule.v1.StructuredCalendarSpec.getDefaultInstance());
}
/**
* repeated .temporal.api.schedule.v1.StructuredCalendarSpec exclude_structured_calendar = 9;
*/
public io.temporal.api.schedule.v1.StructuredCalendarSpec.Builder addExcludeStructuredCalendarBuilder(
int index) {
return getExcludeStructuredCalendarFieldBuilder().addBuilder(
index, io.temporal.api.schedule.v1.StructuredCalendarSpec.getDefaultInstance());
}
/**
* repeated .temporal.api.schedule.v1.StructuredCalendarSpec exclude_structured_calendar = 9;
*/
public java.util.List
getExcludeStructuredCalendarBuilderList() {
return getExcludeStructuredCalendarFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
io.temporal.api.schedule.v1.StructuredCalendarSpec, io.temporal.api.schedule.v1.StructuredCalendarSpec.Builder, io.temporal.api.schedule.v1.StructuredCalendarSpecOrBuilder>
getExcludeStructuredCalendarFieldBuilder() {
if (excludeStructuredCalendarBuilder_ == null) {
excludeStructuredCalendarBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
io.temporal.api.schedule.v1.StructuredCalendarSpec, io.temporal.api.schedule.v1.StructuredCalendarSpec.Builder, io.temporal.api.schedule.v1.StructuredCalendarSpecOrBuilder>(
excludeStructuredCalendar_,
((bitField0_ & 0x00000020) != 0),
getParentForChildren(),
isClean());
excludeStructuredCalendar_ = null;
}
return excludeStructuredCalendarBuilder_;
}
private com.google.protobuf.Timestamp startTime_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder> startTimeBuilder_;
/**
*
* If start_time is set, any timestamps before start_time will be skipped.
* (Together, start_time and end_time make an inclusive interval.)
*
*
* .google.protobuf.Timestamp start_time = 4 [(.gogoproto.stdtime) = true];
* @return Whether the startTime field is set.
*/
public boolean hasStartTime() {
return startTimeBuilder_ != null || startTime_ != null;
}
/**
*
* If start_time is set, any timestamps before start_time will be skipped.
* (Together, start_time and end_time make an inclusive interval.)
*
*
* .google.protobuf.Timestamp start_time = 4 [(.gogoproto.stdtime) = true];
* @return The startTime.
*/
public com.google.protobuf.Timestamp getStartTime() {
if (startTimeBuilder_ == null) {
return startTime_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : startTime_;
} else {
return startTimeBuilder_.getMessage();
}
}
/**
*
* If start_time is set, any timestamps before start_time will be skipped.
* (Together, start_time and end_time make an inclusive interval.)
*
*
* .google.protobuf.Timestamp start_time = 4 [(.gogoproto.stdtime) = true];
*/
public Builder setStartTime(com.google.protobuf.Timestamp value) {
if (startTimeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
startTime_ = value;
onChanged();
} else {
startTimeBuilder_.setMessage(value);
}
return this;
}
/**
*
* If start_time is set, any timestamps before start_time will be skipped.
* (Together, start_time and end_time make an inclusive interval.)
*
*
* .google.protobuf.Timestamp start_time = 4 [(.gogoproto.stdtime) = true];
*/
public Builder setStartTime(
com.google.protobuf.Timestamp.Builder builderForValue) {
if (startTimeBuilder_ == null) {
startTime_ = builderForValue.build();
onChanged();
} else {
startTimeBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* If start_time is set, any timestamps before start_time will be skipped.
* (Together, start_time and end_time make an inclusive interval.)
*
*
* .google.protobuf.Timestamp start_time = 4 [(.gogoproto.stdtime) = true];
*/
public Builder mergeStartTime(com.google.protobuf.Timestamp value) {
if (startTimeBuilder_ == null) {
if (startTime_ != null) {
startTime_ =
com.google.protobuf.Timestamp.newBuilder(startTime_).mergeFrom(value).buildPartial();
} else {
startTime_ = value;
}
onChanged();
} else {
startTimeBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* If start_time is set, any timestamps before start_time will be skipped.
* (Together, start_time and end_time make an inclusive interval.)
*
*
* .google.protobuf.Timestamp start_time = 4 [(.gogoproto.stdtime) = true];
*/
public Builder clearStartTime() {
if (startTimeBuilder_ == null) {
startTime_ = null;
onChanged();
} else {
startTime_ = null;
startTimeBuilder_ = null;
}
return this;
}
/**
*
* If start_time is set, any timestamps before start_time will be skipped.
* (Together, start_time and end_time make an inclusive interval.)
*
*
* .google.protobuf.Timestamp start_time = 4 [(.gogoproto.stdtime) = true];
*/
public com.google.protobuf.Timestamp.Builder getStartTimeBuilder() {
onChanged();
return getStartTimeFieldBuilder().getBuilder();
}
/**
*
* If start_time is set, any timestamps before start_time will be skipped.
* (Together, start_time and end_time make an inclusive interval.)
*
*
* .google.protobuf.Timestamp start_time = 4 [(.gogoproto.stdtime) = true];
*/
public com.google.protobuf.TimestampOrBuilder getStartTimeOrBuilder() {
if (startTimeBuilder_ != null) {
return startTimeBuilder_.getMessageOrBuilder();
} else {
return startTime_ == null ?
com.google.protobuf.Timestamp.getDefaultInstance() : startTime_;
}
}
/**
*
* If start_time is set, any timestamps before start_time will be skipped.
* (Together, start_time and end_time make an inclusive interval.)
*
*
* .google.protobuf.Timestamp start_time = 4 [(.gogoproto.stdtime) = true];
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>
getStartTimeFieldBuilder() {
if (startTimeBuilder_ == null) {
startTimeBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>(
getStartTime(),
getParentForChildren(),
isClean());
startTime_ = null;
}
return startTimeBuilder_;
}
private com.google.protobuf.Timestamp endTime_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder> endTimeBuilder_;
/**
*
* If end_time is set, any timestamps after end_time will be skipped.
*
*
* .google.protobuf.Timestamp end_time = 5 [(.gogoproto.stdtime) = true];
* @return Whether the endTime field is set.
*/
public boolean hasEndTime() {
return endTimeBuilder_ != null || endTime_ != null;
}
/**
*
* If end_time is set, any timestamps after end_time will be skipped.
*
*
* .google.protobuf.Timestamp end_time = 5 [(.gogoproto.stdtime) = true];
* @return The endTime.
*/
public com.google.protobuf.Timestamp getEndTime() {
if (endTimeBuilder_ == null) {
return endTime_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : endTime_;
} else {
return endTimeBuilder_.getMessage();
}
}
/**
*
* If end_time is set, any timestamps after end_time will be skipped.
*
*
* .google.protobuf.Timestamp end_time = 5 [(.gogoproto.stdtime) = true];
*/
public Builder setEndTime(com.google.protobuf.Timestamp value) {
if (endTimeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
endTime_ = value;
onChanged();
} else {
endTimeBuilder_.setMessage(value);
}
return this;
}
/**
*
* If end_time is set, any timestamps after end_time will be skipped.
*
*
* .google.protobuf.Timestamp end_time = 5 [(.gogoproto.stdtime) = true];
*/
public Builder setEndTime(
com.google.protobuf.Timestamp.Builder builderForValue) {
if (endTimeBuilder_ == null) {
endTime_ = builderForValue.build();
onChanged();
} else {
endTimeBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* If end_time is set, any timestamps after end_time will be skipped.
*
*
* .google.protobuf.Timestamp end_time = 5 [(.gogoproto.stdtime) = true];
*/
public Builder mergeEndTime(com.google.protobuf.Timestamp value) {
if (endTimeBuilder_ == null) {
if (endTime_ != null) {
endTime_ =
com.google.protobuf.Timestamp.newBuilder(endTime_).mergeFrom(value).buildPartial();
} else {
endTime_ = value;
}
onChanged();
} else {
endTimeBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* If end_time is set, any timestamps after end_time will be skipped.
*
*
* .google.protobuf.Timestamp end_time = 5 [(.gogoproto.stdtime) = true];
*/
public Builder clearEndTime() {
if (endTimeBuilder_ == null) {
endTime_ = null;
onChanged();
} else {
endTime_ = null;
endTimeBuilder_ = null;
}
return this;
}
/**
*
* If end_time is set, any timestamps after end_time will be skipped.
*
*
* .google.protobuf.Timestamp end_time = 5 [(.gogoproto.stdtime) = true];
*/
public com.google.protobuf.Timestamp.Builder getEndTimeBuilder() {
onChanged();
return getEndTimeFieldBuilder().getBuilder();
}
/**
*
* If end_time is set, any timestamps after end_time will be skipped.
*
*
* .google.protobuf.Timestamp end_time = 5 [(.gogoproto.stdtime) = true];
*/
public com.google.protobuf.TimestampOrBuilder getEndTimeOrBuilder() {
if (endTimeBuilder_ != null) {
return endTimeBuilder_.getMessageOrBuilder();
} else {
return endTime_ == null ?
com.google.protobuf.Timestamp.getDefaultInstance() : endTime_;
}
}
/**
*
* If end_time is set, any timestamps after end_time will be skipped.
*
*
* .google.protobuf.Timestamp end_time = 5 [(.gogoproto.stdtime) = true];
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>
getEndTimeFieldBuilder() {
if (endTimeBuilder_ == null) {
endTimeBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>(
getEndTime(),
getParentForChildren(),
isClean());
endTime_ = null;
}
return endTimeBuilder_;
}
private com.google.protobuf.Duration jitter_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Duration, com.google.protobuf.Duration.Builder, com.google.protobuf.DurationOrBuilder> jitterBuilder_;
/**
*
* All timestamps will be incremented by a random value from 0 to this
* amount of jitter. Default: 0
*
*
* .google.protobuf.Duration jitter = 6 [(.gogoproto.stdduration) = true];
* @return Whether the jitter field is set.
*/
public boolean hasJitter() {
return jitterBuilder_ != null || jitter_ != null;
}
/**
*
* All timestamps will be incremented by a random value from 0 to this
* amount of jitter. Default: 0
*
*
* .google.protobuf.Duration jitter = 6 [(.gogoproto.stdduration) = true];
* @return The jitter.
*/
public com.google.protobuf.Duration getJitter() {
if (jitterBuilder_ == null) {
return jitter_ == null ? com.google.protobuf.Duration.getDefaultInstance() : jitter_;
} else {
return jitterBuilder_.getMessage();
}
}
/**
*
* All timestamps will be incremented by a random value from 0 to this
* amount of jitter. Default: 0
*
*
* .google.protobuf.Duration jitter = 6 [(.gogoproto.stdduration) = true];
*/
public Builder setJitter(com.google.protobuf.Duration value) {
if (jitterBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
jitter_ = value;
onChanged();
} else {
jitterBuilder_.setMessage(value);
}
return this;
}
/**
*
* All timestamps will be incremented by a random value from 0 to this
* amount of jitter. Default: 0
*
*
* .google.protobuf.Duration jitter = 6 [(.gogoproto.stdduration) = true];
*/
public Builder setJitter(
com.google.protobuf.Duration.Builder builderForValue) {
if (jitterBuilder_ == null) {
jitter_ = builderForValue.build();
onChanged();
} else {
jitterBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* All timestamps will be incremented by a random value from 0 to this
* amount of jitter. Default: 0
*
*
* .google.protobuf.Duration jitter = 6 [(.gogoproto.stdduration) = true];
*/
public Builder mergeJitter(com.google.protobuf.Duration value) {
if (jitterBuilder_ == null) {
if (jitter_ != null) {
jitter_ =
com.google.protobuf.Duration.newBuilder(jitter_).mergeFrom(value).buildPartial();
} else {
jitter_ = value;
}
onChanged();
} else {
jitterBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* All timestamps will be incremented by a random value from 0 to this
* amount of jitter. Default: 0
*
*
* .google.protobuf.Duration jitter = 6 [(.gogoproto.stdduration) = true];
*/
public Builder clearJitter() {
if (jitterBuilder_ == null) {
jitter_ = null;
onChanged();
} else {
jitter_ = null;
jitterBuilder_ = null;
}
return this;
}
/**
*
* All timestamps will be incremented by a random value from 0 to this
* amount of jitter. Default: 0
*
*
* .google.protobuf.Duration jitter = 6 [(.gogoproto.stdduration) = true];
*/
public com.google.protobuf.Duration.Builder getJitterBuilder() {
onChanged();
return getJitterFieldBuilder().getBuilder();
}
/**
*
* All timestamps will be incremented by a random value from 0 to this
* amount of jitter. Default: 0
*
*
* .google.protobuf.Duration jitter = 6 [(.gogoproto.stdduration) = true];
*/
public com.google.protobuf.DurationOrBuilder getJitterOrBuilder() {
if (jitterBuilder_ != null) {
return jitterBuilder_.getMessageOrBuilder();
} else {
return jitter_ == null ?
com.google.protobuf.Duration.getDefaultInstance() : jitter_;
}
}
/**
*
* All timestamps will be incremented by a random value from 0 to this
* amount of jitter. Default: 0
*
*
* .google.protobuf.Duration jitter = 6 [(.gogoproto.stdduration) = true];
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Duration, com.google.protobuf.Duration.Builder, com.google.protobuf.DurationOrBuilder>
getJitterFieldBuilder() {
if (jitterBuilder_ == null) {
jitterBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Duration, com.google.protobuf.Duration.Builder, com.google.protobuf.DurationOrBuilder>(
getJitter(),
getParentForChildren(),
isClean());
jitter_ = null;
}
return jitterBuilder_;
}
private java.lang.Object timezoneName_ = "";
/**
*
* Time zone to interpret all calendar-based specs in.
* If unset, defaults to UTC. We recommend using UTC for your application if
* at all possible, to avoid various surprising properties of time zones.
* Time zones may be provided by name, corresponding to names in the IANA
* time zone database (see https://www.iana.org/time-zones). The definition
* will be loaded by the Temporal server from the environment it runs in.
* If your application requires more control over the time zone definition
* used, it may pass in a complete definition in the form of a TZif file
* from the time zone database. If present, this will be used instead of
* loading anything from the environment. You are then responsible for
* updating timezone_data when the definition changes.
* Calendar spec matching is based on literal matching of the clock time
* with no special handling of DST: if you write a calendar spec that fires
* at 2:30am and specify a time zone that follows DST, that action will not
* be triggered on the day that has no 2:30am. Similarly, an action that
* fires at 1:30am will be triggered twice on the day that has two 1:30s.
* Also note that no actions are taken on leap-seconds (e.g. 23:59:60 UTC).
*
*
* string timezone_name = 10;
* @return The timezoneName.
*/
public java.lang.String getTimezoneName() {
java.lang.Object ref = timezoneName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
timezoneName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Time zone to interpret all calendar-based specs in.
* If unset, defaults to UTC. We recommend using UTC for your application if
* at all possible, to avoid various surprising properties of time zones.
* Time zones may be provided by name, corresponding to names in the IANA
* time zone database (see https://www.iana.org/time-zones). The definition
* will be loaded by the Temporal server from the environment it runs in.
* If your application requires more control over the time zone definition
* used, it may pass in a complete definition in the form of a TZif file
* from the time zone database. If present, this will be used instead of
* loading anything from the environment. You are then responsible for
* updating timezone_data when the definition changes.
* Calendar spec matching is based on literal matching of the clock time
* with no special handling of DST: if you write a calendar spec that fires
* at 2:30am and specify a time zone that follows DST, that action will not
* be triggered on the day that has no 2:30am. Similarly, an action that
* fires at 1:30am will be triggered twice on the day that has two 1:30s.
* Also note that no actions are taken on leap-seconds (e.g. 23:59:60 UTC).
*
*
* string timezone_name = 10;
* @return The bytes for timezoneName.
*/
public com.google.protobuf.ByteString
getTimezoneNameBytes() {
java.lang.Object ref = timezoneName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
timezoneName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Time zone to interpret all calendar-based specs in.
* If unset, defaults to UTC. We recommend using UTC for your application if
* at all possible, to avoid various surprising properties of time zones.
* Time zones may be provided by name, corresponding to names in the IANA
* time zone database (see https://www.iana.org/time-zones). The definition
* will be loaded by the Temporal server from the environment it runs in.
* If your application requires more control over the time zone definition
* used, it may pass in a complete definition in the form of a TZif file
* from the time zone database. If present, this will be used instead of
* loading anything from the environment. You are then responsible for
* updating timezone_data when the definition changes.
* Calendar spec matching is based on literal matching of the clock time
* with no special handling of DST: if you write a calendar spec that fires
* at 2:30am and specify a time zone that follows DST, that action will not
* be triggered on the day that has no 2:30am. Similarly, an action that
* fires at 1:30am will be triggered twice on the day that has two 1:30s.
* Also note that no actions are taken on leap-seconds (e.g. 23:59:60 UTC).
*
*
* string timezone_name = 10;
* @param value The timezoneName to set.
* @return This builder for chaining.
*/
public Builder setTimezoneName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
timezoneName_ = value;
onChanged();
return this;
}
/**
*
* Time zone to interpret all calendar-based specs in.
* If unset, defaults to UTC. We recommend using UTC for your application if
* at all possible, to avoid various surprising properties of time zones.
* Time zones may be provided by name, corresponding to names in the IANA
* time zone database (see https://www.iana.org/time-zones). The definition
* will be loaded by the Temporal server from the environment it runs in.
* If your application requires more control over the time zone definition
* used, it may pass in a complete definition in the form of a TZif file
* from the time zone database. If present, this will be used instead of
* loading anything from the environment. You are then responsible for
* updating timezone_data when the definition changes.
* Calendar spec matching is based on literal matching of the clock time
* with no special handling of DST: if you write a calendar spec that fires
* at 2:30am and specify a time zone that follows DST, that action will not
* be triggered on the day that has no 2:30am. Similarly, an action that
* fires at 1:30am will be triggered twice on the day that has two 1:30s.
* Also note that no actions are taken on leap-seconds (e.g. 23:59:60 UTC).
*
*
* string timezone_name = 10;
* @return This builder for chaining.
*/
public Builder clearTimezoneName() {
timezoneName_ = getDefaultInstance().getTimezoneName();
onChanged();
return this;
}
/**
*
* Time zone to interpret all calendar-based specs in.
* If unset, defaults to UTC. We recommend using UTC for your application if
* at all possible, to avoid various surprising properties of time zones.
* Time zones may be provided by name, corresponding to names in the IANA
* time zone database (see https://www.iana.org/time-zones). The definition
* will be loaded by the Temporal server from the environment it runs in.
* If your application requires more control over the time zone definition
* used, it may pass in a complete definition in the form of a TZif file
* from the time zone database. If present, this will be used instead of
* loading anything from the environment. You are then responsible for
* updating timezone_data when the definition changes.
* Calendar spec matching is based on literal matching of the clock time
* with no special handling of DST: if you write a calendar spec that fires
* at 2:30am and specify a time zone that follows DST, that action will not
* be triggered on the day that has no 2:30am. Similarly, an action that
* fires at 1:30am will be triggered twice on the day that has two 1:30s.
* Also note that no actions are taken on leap-seconds (e.g. 23:59:60 UTC).
*
*
* string timezone_name = 10;
* @param value The bytes for timezoneName to set.
* @return This builder for chaining.
*/
public Builder setTimezoneNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
timezoneName_ = value;
onChanged();
return this;
}
private com.google.protobuf.ByteString timezoneData_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes timezone_data = 11;
* @return The timezoneData.
*/
public com.google.protobuf.ByteString getTimezoneData() {
return timezoneData_;
}
/**
* bytes timezone_data = 11;
* @param value The timezoneData to set.
* @return This builder for chaining.
*/
public Builder setTimezoneData(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
timezoneData_ = value;
onChanged();
return this;
}
/**
* bytes timezone_data = 11;
* @return This builder for chaining.
*/
public Builder clearTimezoneData() {
timezoneData_ = getDefaultInstance().getTimezoneData();
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:temporal.api.schedule.v1.ScheduleSpec)
}
// @@protoc_insertion_point(class_scope:temporal.api.schedule.v1.ScheduleSpec)
private static final io.temporal.api.schedule.v1.ScheduleSpec DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.temporal.api.schedule.v1.ScheduleSpec();
}
public static io.temporal.api.schedule.v1.ScheduleSpec getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ScheduleSpec parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ScheduleSpec(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.temporal.api.schedule.v1.ScheduleSpec getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy