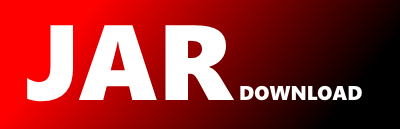
org.apache.maven.plugin.descriptor.model.Parameter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of maven-plugin-api Show documentation
Show all versions of maven-plugin-api Show documentation
The API for plugins - Mojos - development.
// =================== DO NOT EDIT THIS FILE ====================
// Generated by Modello 1.7,
// any modifications will be overwritten.
// ==============================================================
package org.apache.maven.plugin.descriptor.model;
/**
* A phase mapping definition.
*
* @version $Revision$ $Date$
*/
@SuppressWarnings( "all" )
public class Parameter
implements java.io.Serializable
{
//--------------------------/
//- Class/Member Variables -/
//--------------------------/
/**
*
* The name of the parameter, to be used while
* configuring this parameter from the Mojo's declared defaults
* or from the POM.
*
*/
private String name;
/**
*
* Specifies an alias which can be used to
* configure this parameter from the POM.
* This is primarily useful to improve
* user-friendliness, where Mojo field names are not intuitive
* to the
* user or are otherwise not conducive to
* configuration via the POM.
*
*/
private String alias;
/**
*
* The Java type for this parameter. This is used
* to validate the result of any expressions used to calculate
* the value which should be injected into the Mojo
* for this parameter.
*
*/
private String type;
/**
*
* Whether this parameter is required for the Mojo
* to function. This is used to validate the configuration
* for a Mojo before it is injected, and before the
* Mojo is executed from some half-state.
*
*/
private boolean required = false;
/**
*
* Specifies that this parameter can be configured
* directly by the user (as in the case of POM-specified
* configuration). This is useful when you want to
* force the user to use common POM elements rather than
* plugin configurations, as in the case where you
* want to use the artifact's final name as a parameter. In
* this case, you want the user to modify
* <build><finalName/></build>
* rather
* than specifying a value for finalName directly
* in the plugin configuration section. It is also useful to
* ensure that - for example - a List-typed
* parameter which expects items of type Artifact doesn't get a
* List
* full of Strings.
*
*/
private boolean editable = true;
/**
* Field implementation.
*/
private String implementation;
/**
* The description of this parameter's use inside the Mojo.
*/
private String description;
/**
* Specify the version when the parameter was added to the API.
* Similar to Javadoc since.
*/
private String since;
/**
*
* Specify the version when the parameter was
* deprecated to the API. Similar to Javadoc deprecated.
* This will trigger a warning when a user tries to
* configure a parameter marked as deprecated.
*
*/
private String deprecated;
//-----------/
//- Methods -/
//-----------/
/**
* Get specifies an alias which can be used to configure this
* parameter from the POM.
* This is primarily useful to improve
* user-friendliness, where Mojo field names are not intuitive
* to the
* user or are otherwise not conducive to
* configuration via the POM.
*
* @return String
*/
public String getAlias()
{
return this.alias;
} //-- String getAlias()
/**
* Get specify the version when the parameter was deprecated to
* the API. Similar to Javadoc deprecated.
* This will trigger a warning when a user tries to
* configure a parameter marked as deprecated.
*
* @return String
*/
public String getDeprecated()
{
return this.deprecated;
} //-- String getDeprecated()
/**
* Get the description of this parameter's use inside the Mojo.
*
* @return String
*/
public String getDescription()
{
return this.description;
} //-- String getDescription()
/**
* Get the implementation field.
*
* @return String
*/
public String getImplementation()
{
return this.implementation;
} //-- String getImplementation()
/**
* Get the name of the parameter, to be used while configuring
* this parameter from the Mojo's declared defaults
* or from the POM.
*
* @return String
*/
public String getName()
{
return this.name;
} //-- String getName()
/**
* Get specify the version when the parameter was added to the
* API. Similar to Javadoc since.
*
* @return String
*/
public String getSince()
{
return this.since;
} //-- String getSince()
/**
* Get the Java type for this parameter. This is used to
* validate the result of any expressions used to calculate
* the value which should be injected into the Mojo
* for this parameter.
*
* @return String
*/
public String getType()
{
return this.type;
} //-- String getType()
/**
* Get specifies that this parameter can be configured directly
* by the user (as in the case of POM-specified
* configuration). This is useful when you want to
* force the user to use common POM elements rather than
* plugin configurations, as in the case where you
* want to use the artifact's final name as a parameter. In
* this case, you want the user to modify
* <build><finalName/></build>
* rather
* than specifying a value for finalName directly
* in the plugin configuration section. It is also useful to
* ensure that - for example - a List-typed
* parameter which expects items of type Artifact doesn't get a
* List
* full of Strings.
*
* @return boolean
*/
public boolean isEditable()
{
return this.editable;
} //-- boolean isEditable()
/**
* Get whether this parameter is required for the Mojo to
* function. This is used to validate the configuration
* for a Mojo before it is injected, and before the
* Mojo is executed from some half-state.
*
* @return boolean
*/
public boolean isRequired()
{
return this.required;
} //-- boolean isRequired()
/**
* Set specifies an alias which can be used to configure this
* parameter from the POM.
* This is primarily useful to improve
* user-friendliness, where Mojo field names are not intuitive
* to the
* user or are otherwise not conducive to
* configuration via the POM.
*
* @param alias
*/
public void setAlias( String alias )
{
this.alias = alias;
} //-- void setAlias( String )
/**
* Set specify the version when the parameter was deprecated to
* the API. Similar to Javadoc deprecated.
* This will trigger a warning when a user tries to
* configure a parameter marked as deprecated.
*
* @param deprecated
*/
public void setDeprecated( String deprecated )
{
this.deprecated = deprecated;
} //-- void setDeprecated( String )
/**
* Set the description of this parameter's use inside the Mojo.
*
* @param description
*/
public void setDescription( String description )
{
this.description = description;
} //-- void setDescription( String )
/**
* Set specifies that this parameter can be configured directly
* by the user (as in the case of POM-specified
* configuration). This is useful when you want to
* force the user to use common POM elements rather than
* plugin configurations, as in the case where you
* want to use the artifact's final name as a parameter. In
* this case, you want the user to modify
* <build><finalName/></build>
* rather
* than specifying a value for finalName directly
* in the plugin configuration section. It is also useful to
* ensure that - for example - a List-typed
* parameter which expects items of type Artifact doesn't get a
* List
* full of Strings.
*
* @param editable
*/
public void setEditable( boolean editable )
{
this.editable = editable;
} //-- void setEditable( boolean )
/**
* Set the implementation field.
*
* @param implementation
*/
public void setImplementation( String implementation )
{
this.implementation = implementation;
} //-- void setImplementation( String )
/**
* Set the name of the parameter, to be used while configuring
* this parameter from the Mojo's declared defaults
* or from the POM.
*
* @param name
*/
public void setName( String name )
{
this.name = name;
} //-- void setName( String )
/**
* Set whether this parameter is required for the Mojo to
* function. This is used to validate the configuration
* for a Mojo before it is injected, and before the
* Mojo is executed from some half-state.
*
* @param required
*/
public void setRequired( boolean required )
{
this.required = required;
} //-- void setRequired( boolean )
/**
* Set specify the version when the parameter was added to the
* API. Similar to Javadoc since.
*
* @param since
*/
public void setSince( String since )
{
this.since = since;
} //-- void setSince( String )
/**
* Set the Java type for this parameter. This is used to
* validate the result of any expressions used to calculate
* the value which should be injected into the Mojo
* for this parameter.
*
* @param type
*/
public void setType( String type )
{
this.type = type;
} //-- void setType( String )
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy