- >> pages(List
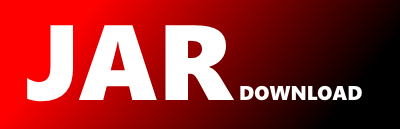
io.thestencil.client.spi.builders.UpdateBuilderImpl Maven / Gradle / Ivy
package io.thestencil.client.spi.builders;
/*-
* #%L
* stencil-persistence
* %%
* Copyright (C) 2021 Copyright 2021 ReSys OÜ
* %%
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* #L%
*/
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.stream.Collectors;
import io.resys.thena.docdb.api.actions.CommitActions.CommitStatus;
import io.resys.thena.docdb.api.actions.ObjectsActions.ObjectsStatus;
import io.smallrye.mutiny.Uni;
import io.thestencil.client.api.StencilClient.Article;
import io.thestencil.client.api.StencilClient.Entity;
import io.thestencil.client.api.StencilClient.EntityType;
import io.thestencil.client.api.StencilClient.Link;
import io.thestencil.client.api.StencilClient.Locale;
import io.thestencil.client.api.StencilClient.Page;
import io.thestencil.client.api.StencilClient.Workflow;
import io.thestencil.client.spi.PersistenceCommands;
import io.thestencil.client.spi.PersistenceConfig;
import io.thestencil.client.spi.PersistenceConfig.EntityState;
import io.thestencil.client.spi.exceptions.QueryException;
import io.thestencil.client.spi.exceptions.SaveException;
import io.thestencil.client.api.ImmutableArticle;
import io.thestencil.client.api.ImmutableEntity;
import io.thestencil.client.api.ImmutableLink;
import io.thestencil.client.api.ImmutableLocale;
import io.thestencil.client.api.ImmutablePage;
import io.thestencil.client.api.ImmutableWorkflow;
import io.thestencil.client.api.UpdateBuilder;
public class UpdateBuilderImpl extends PersistenceCommands implements UpdateBuilder {
public UpdateBuilderImpl(PersistenceConfig config) {
super(config);
}
@Override
public Uni> article(ArticleMutator changes) {
// Get the article
final Uni> query = get(changes.getArticleId(), EntityType.ARTICLE);
// Change the article
return query.onItem().transformToUni(state -> save(changeArticle(state, changes)));
}
private Entity changeArticle(EntityState state, ArticleMutator changes) {
final var start = state.getEntity();
return ImmutableEntity.builder()
.from(start)
.body(ImmutableArticle.builder().from(start.getBody())
.name(changes.getName())
.order(changes.getOrder())
.parentId(changes.getParentId())
.build())
.build();
}
@Override
public Uni> locale(LocaleMutator changes) {
// Get the locale
final Uni> query = get(changes.getLocaleId(), EntityType.LOCALE);
// Change the locale
return query.onItem().transformToUni(state -> save(changeLocale(state, changes)));
}
private Entity changeLocale(EntityState state, LocaleMutator changes) {
final var start = state.getEntity();
return ImmutableEntity.builder()
.from(start)
.body(ImmutableLocale.builder().from(start.getBody())
.value(changes.getValue())
.enabled(changes.getEnabled())
.build())
.build();
}
@Override
public Uni> page(PageMutator changes) {
// Get the page
final Uni> query = get(changes.getPageId(), EntityType.PAGE);
// Change the page
return query.onItem().transformToUni(state -> save(changePage(state, changes)));
}
@Override
public Uni
© 2015 - 2025 Weber Informatics LLC | Privacy Policy