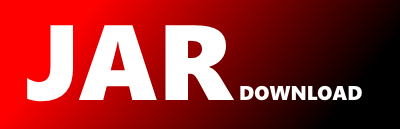
org.wildfly.swarm.cdi.jaxrsapi.deployment.ProxyBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cdi-jaxrsapi Show documentation
Show all versions of cdi-jaxrsapi Show documentation
Generates implementation of external service from interface which can then be injected to call that service.
package org.wildfly.swarm.cdi.jaxrsapi.deployment;
import java.lang.reflect.Method;
import java.lang.reflect.Proxy;
import java.util.Arrays;
import java.util.HashMap;
import java.util.Set;
import javax.ws.rs.Path;
import javax.ws.rs.client.WebTarget;
import javax.ws.rs.core.MediaType;
import org.jboss.resteasy.client.jaxrs.ProxyConfig;
import org.jboss.resteasy.client.jaxrs.ResteasyWebTarget;
import org.jboss.resteasy.client.jaxrs.i18n.Messages;
import org.jboss.resteasy.client.jaxrs.internal.proxy.ClientInvoker;
import org.jboss.resteasy.client.jaxrs.internal.proxy.ClientProxy;
import org.jboss.resteasy.client.jaxrs.internal.proxy.MethodInvoker;
import org.jboss.resteasy.client.jaxrs.internal.proxy.ResteasyClientProxy;
import org.jboss.resteasy.client.jaxrs.internal.proxy.SubResourceInvoker;
import org.jboss.resteasy.util.IsHttpMethod;
/**
* Borrowed from RESTEasy for initial work
*/
public class ProxyBuilder {
private static final Class>[] cClassArgArray = {Class.class};
private final Class iface;
private final ResteasyWebTarget webTarget;
private ClassLoader loader = Thread.currentThread().getContextClassLoader();
private MediaType serverConsumes;
private MediaType serverProduces;
public static ProxyBuilder builder(Class iface, WebTarget webTarget) {
return new ProxyBuilder(iface, (ResteasyWebTarget) webTarget);
}
@SuppressWarnings("unchecked")
public static T proxy(final Class iface, WebTarget base, final ProxyConfig config) {
if (iface.isAnnotationPresent(Path.class)) {
Path path = iface.getAnnotation(Path.class);
if (!path.value().equals("") && !path.value().equals("/")) {
base = base.path(path.value());
}
}
HashMap methodMap = new HashMap();
for (Method method : iface.getMethods()) {
// ignore the as method to allow declaration in client interfaces
if ("as".equals(method.getName()) && Arrays.equals(method.getParameterTypes(), cClassArgArray)) {
continue;
}
// Added by Ken Finnigan
// Ignore default methods
if (method.isDefault()) {
continue;
}
MethodInvoker invoker;
Set httpMethods = IsHttpMethod.getHttpMethods(method);
if ((httpMethods == null || httpMethods.size() == 0) && method.isAnnotationPresent(Path.class) && method.getReturnType().isInterface()) {
invoker = new SubResourceInvoker((ResteasyWebTarget) base, method, config);
} else {
invoker = createClientInvoker(iface, method, (ResteasyWebTarget) base, config);
}
methodMap.put(method, invoker);
}
Class>[] intfs =
{
iface, ResteasyClientProxy.class
};
ClientProxy clientProxy = new ClientProxy(methodMap, base, config);
// this is done so that equals and hashCode work ok. Adding the proxy to a
// Collection will cause equals and hashCode to be invoked. The Spring
// infrastructure had some problems without this.
clientProxy.setClazz(iface);
return (T) Proxy.newProxyInstance(config.getLoader(), intfs, clientProxy);
}
private static ClientInvoker createClientInvoker(Class clazz, Method method, ResteasyWebTarget base, ProxyConfig config) {
Set httpMethods = IsHttpMethod.getHttpMethods(method);
if (httpMethods == null || httpMethods.size() != 1) {
throw new RuntimeException(Messages.MESSAGES.mustUseExactlyOneHttpMethod(method.toString()));
}
ClientInvoker invoker = new ClientInvoker(base, clazz, method, config);
invoker.setHttpMethod(httpMethods.iterator().next());
return invoker;
}
private ProxyBuilder(Class iface, ResteasyWebTarget webTarget) {
this.iface = iface;
this.webTarget = webTarget;
}
public ProxyBuilder classloader(ClassLoader cl) {
this.loader = cl;
return this;
}
public ProxyBuilder defaultProduces(MediaType type) {
this.serverProduces = type;
return this;
}
public ProxyBuilder defaultConsumes(MediaType type) {
this.serverConsumes = type;
return this;
}
public ProxyBuilder defaultProduces(String type) {
this.serverProduces = MediaType.valueOf(type);
return this;
}
public ProxyBuilder defaultConsumes(String type) {
this.serverConsumes = MediaType.valueOf(type);
return this;
}
public T build() {
return proxy(iface, webTarget, new ProxyConfig(loader, serverConsumes, serverProduces));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy