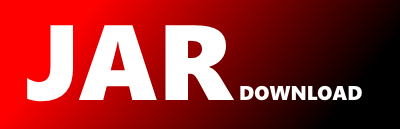
org.wildfly.swarm.config.MessagingActiveMQ Maven / Gradle / Ivy
package org.wildfly.swarm.config;
import org.wildfly.swarm.config.runtime.AttributeDocumentation;
import org.wildfly.swarm.config.runtime.ResourceDocumentation;
import org.wildfly.swarm.config.runtime.SingletonResource;
import org.wildfly.swarm.config.runtime.Address;
import org.wildfly.swarm.config.runtime.ResourceType;
import org.wildfly.swarm.config.runtime.Implicit;
import java.beans.PropertyChangeSupport;
import java.beans.PropertyChangeListener;
import java.util.List;
import org.wildfly.swarm.config.runtime.Subresource;
import org.wildfly.swarm.config.messaging.activemq.ServerConsumer;
import org.wildfly.swarm.config.messaging.activemq.ServerSupplier;
import org.wildfly.swarm.config.messaging.activemq.Server;
import org.wildfly.swarm.config.runtime.SubresourceInfo;
import org.wildfly.swarm.config.messaging.activemq.JMSBridgeConsumer;
import org.wildfly.swarm.config.messaging.activemq.JMSBridgeSupplier;
import org.wildfly.swarm.config.messaging.activemq.JMSBridge;
import org.wildfly.swarm.config.runtime.ModelNodeBinding;
/**
* The messaging-activemq subsystem.
*/
@Address("/subsystem=messaging-activemq")
@ResourceType("subsystem")
@Implicit
public class MessagingActiveMQ>
implements
org.wildfly.swarm.config.runtime.Keyed {
private String key;
private PropertyChangeSupport pcs;
private MessagingActiveMQResources subresources = new MessagingActiveMQResources();
@AttributeDocumentation("Maximum size of the pool of threads used by all ActiveMQ clients running inside this server. If the attribute is undefined (by default), ActiveMQ will configure it to be 8 x the number of available processors.")
private Integer globalClientScheduledThreadPoolMaxSize;
@AttributeDocumentation("Maximum size of the pool of scheduled threads used by all ActiveMQ clients running inside this server.")
private Integer globalClientThreadPoolMaxSize;
public MessagingActiveMQ() {
super();
this.key = "messaging-activemq";
this.pcs = new PropertyChangeSupport(this);
}
public String getKey() {
return this.key;
}
/**
* Adds a property change listener
*/
public void addPropertyChangeListener(PropertyChangeListener listener) {
if (null == this.pcs)
this.pcs = new PropertyChangeSupport(this);
this.pcs.addPropertyChangeListener(listener);
}
/**
* Removes a property change listener
*/
public void removePropertyChangeListener(
java.beans.PropertyChangeListener listener) {
if (this.pcs != null)
this.pcs.removePropertyChangeListener(listener);
}
public MessagingActiveMQResources subresources() {
return this.subresources;
}
/**
* Add all Server objects to this subresource
*
* @return this
* @param value
* List of Server objects.
*/
@SuppressWarnings("unchecked")
public T servers(java.util.List value) {
this.subresources.servers = value;
return (T) this;
}
/**
* Add the Server object to the list of subresources
*
* @param value
* The Server to add
* @return this
*/
@SuppressWarnings("unchecked")
public T server(Server value) {
this.subresources.servers.add(value);
return (T) this;
}
/**
* Create and configure a Server object to the list of subresources
*
* @param key
* The key for the Server resource
* @param config
* The ServerConsumer to use
* @return this
*/
@SuppressWarnings("unchecked")
public T server(java.lang.String childKey, ServerConsumer consumer) {
Server extends Server> child = new Server<>(childKey);
if (consumer != null) {
consumer.accept(child);
}
server(child);
return (T) this;
}
/**
* Create and configure a Server object to the list of subresources
*
* @param key
* The key for the Server resource
* @return this
*/
@SuppressWarnings("unchecked")
public T server(java.lang.String childKey) {
server(childKey, null);
return (T) this;
}
/**
* Install a supplied Server object to the list of subresources
*/
@SuppressWarnings("unchecked")
public T server(ServerSupplier supplier) {
server(supplier.get());
return (T) this;
}
/**
* Add all JMSBridge objects to this subresource
*
* @return this
* @param value
* List of JMSBridge objects.
*/
@SuppressWarnings("unchecked")
public T jmsBridges(java.util.List value) {
this.subresources.jmsBridges = value;
return (T) this;
}
/**
* Add the JMSBridge object to the list of subresources
*
* @param value
* The JMSBridge to add
* @return this
*/
@SuppressWarnings("unchecked")
public T jmsBridge(JMSBridge value) {
this.subresources.jmsBridges.add(value);
return (T) this;
}
/**
* Create and configure a JMSBridge object to the list of subresources
*
* @param key
* The key for the JMSBridge resource
* @param config
* The JMSBridgeConsumer to use
* @return this
*/
@SuppressWarnings("unchecked")
public T jmsBridge(java.lang.String childKey, JMSBridgeConsumer consumer) {
JMSBridge extends JMSBridge> child = new JMSBridge<>(childKey);
if (consumer != null) {
consumer.accept(child);
}
jmsBridge(child);
return (T) this;
}
/**
* Create and configure a JMSBridge object to the list of subresources
*
* @param key
* The key for the JMSBridge resource
* @return this
*/
@SuppressWarnings("unchecked")
public T jmsBridge(java.lang.String childKey) {
jmsBridge(childKey, null);
return (T) this;
}
/**
* Install a supplied JMSBridge object to the list of subresources
*/
@SuppressWarnings("unchecked")
public T jmsBridge(JMSBridgeSupplier supplier) {
jmsBridge(supplier.get());
return (T) this;
}
/**
* Child mutators for MessagingActiveMQ
*/
public static class MessagingActiveMQResources {
/**
* An ActiveMQ server instance.
*/
@ResourceDocumentation("An ActiveMQ server instance.")
@SubresourceInfo("server")
private List servers = new java.util.ArrayList<>();
/**
* A JMS bridge instance.
*/
@ResourceDocumentation("A JMS bridge instance.")
@SubresourceInfo("jmsBridge")
private List jmsBridges = new java.util.ArrayList<>();
/**
* Get the list of Server resources
*
* @return the list of resources
*/
@Subresource
public List servers() {
return this.servers;
}
public Server server(java.lang.String key) {
return this.servers.stream().filter(e -> e.getKey().equals(key))
.findFirst().orElse(null);
}
/**
* Get the list of JMSBridge resources
*
* @return the list of resources
*/
@Subresource
public List jmsBridges() {
return this.jmsBridges;
}
public JMSBridge jmsBridge(java.lang.String key) {
return this.jmsBridges.stream().filter(e -> e.getKey().equals(key))
.findFirst().orElse(null);
}
}
/**
* Maximum size of the pool of threads used by all ActiveMQ clients running
* inside this server. If the attribute is undefined (by default), ActiveMQ
* will configure it to be 8 x the number of available processors.
*/
@ModelNodeBinding(detypedName = "global-client-scheduled-thread-pool-max-size")
public Integer globalClientScheduledThreadPoolMaxSize() {
return this.globalClientScheduledThreadPoolMaxSize;
}
/**
* Maximum size of the pool of threads used by all ActiveMQ clients running
* inside this server. If the attribute is undefined (by default), ActiveMQ
* will configure it to be 8 x the number of available processors.
*/
@SuppressWarnings("unchecked")
public T globalClientScheduledThreadPoolMaxSize(java.lang.Integer value) {
Object oldValue = this.globalClientScheduledThreadPoolMaxSize;
this.globalClientScheduledThreadPoolMaxSize = value;
if (this.pcs != null)
this.pcs.firePropertyChange(
"globalClientScheduledThreadPoolMaxSize", oldValue, value);
return (T) this;
}
/**
* Maximum size of the pool of scheduled threads used by all ActiveMQ
* clients running inside this server.
*/
@ModelNodeBinding(detypedName = "global-client-thread-pool-max-size")
public Integer globalClientThreadPoolMaxSize() {
return this.globalClientThreadPoolMaxSize;
}
/**
* Maximum size of the pool of scheduled threads used by all ActiveMQ
* clients running inside this server.
*/
@SuppressWarnings("unchecked")
public T globalClientThreadPoolMaxSize(java.lang.Integer value) {
Object oldValue = this.globalClientThreadPoolMaxSize;
this.globalClientThreadPoolMaxSize = value;
if (this.pcs != null)
this.pcs.firePropertyChange("globalClientThreadPoolMaxSize",
oldValue, value);
return (T) this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy