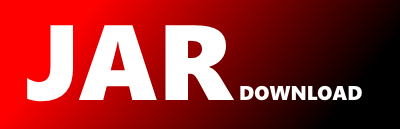
org.wildfly.swarm.config.Remoting Maven / Gradle / Ivy
package org.wildfly.swarm.config;
import org.wildfly.swarm.config.runtime.AttributeDocumentation;
import org.wildfly.swarm.config.runtime.ResourceDocumentation;
import org.wildfly.swarm.config.runtime.SingletonResource;
import org.wildfly.swarm.config.runtime.Address;
import org.wildfly.swarm.config.runtime.ResourceType;
import org.wildfly.swarm.config.runtime.Implicit;
import java.beans.PropertyChangeSupport;
import java.beans.PropertyChangeListener;
import java.util.List;
import org.wildfly.swarm.config.runtime.Subresource;
import org.wildfly.swarm.config.remoting.HTTPConnectorConsumer;
import org.wildfly.swarm.config.remoting.HTTPConnectorSupplier;
import org.wildfly.swarm.config.remoting.HTTPConnector;
import org.wildfly.swarm.config.runtime.SubresourceInfo;
import org.wildfly.swarm.config.remoting.ConnectorConsumer;
import org.wildfly.swarm.config.remoting.ConnectorSupplier;
import org.wildfly.swarm.config.remoting.Connector;
import org.wildfly.swarm.config.remoting.OutboundConnectionConsumer;
import org.wildfly.swarm.config.remoting.OutboundConnectionSupplier;
import org.wildfly.swarm.config.remoting.OutboundConnection;
import org.wildfly.swarm.config.remoting.LocalOutboundConnectionConsumer;
import org.wildfly.swarm.config.remoting.LocalOutboundConnectionSupplier;
import org.wildfly.swarm.config.remoting.LocalOutboundConnection;
import org.wildfly.swarm.config.remoting.RemoteOutboundConnectionConsumer;
import org.wildfly.swarm.config.remoting.RemoteOutboundConnectionSupplier;
import org.wildfly.swarm.config.remoting.RemoteOutboundConnection;
import org.wildfly.swarm.config.remoting.EndpointConfiguration;
import org.wildfly.swarm.config.remoting.EndpointConfigurationConsumer;
import org.wildfly.swarm.config.remoting.EndpointConfigurationSupplier;
import org.wildfly.swarm.config.runtime.ModelNodeBinding;
/**
* The configuration of the Remoting subsystem.
*/
@Address("/subsystem=remoting")
@ResourceType("subsystem")
@Implicit
public class Remoting>
implements
org.wildfly.swarm.config.runtime.Keyed {
private String key;
private PropertyChangeSupport pcs;
private RemotingResources subresources = new RemotingResources();
@AttributeDocumentation("The number of read threads to create for the remoting worker.")
private Integer workerReadThreads;
@AttributeDocumentation("The number of core threads for the remoting worker task thread pool.")
private Integer workerTaskCoreThreads;
@AttributeDocumentation("The number of milliseconds to keep non-core remoting worker task threads alive.")
private Integer workerTaskKeepalive;
@AttributeDocumentation("The maximum number of remoting worker tasks to allow before rejecting.")
private Integer workerTaskLimit;
@AttributeDocumentation("The maximum number of threads for the remoting worker task thread pool.")
private Integer workerTaskMaxThreads;
@AttributeDocumentation("The number of write threads to create for the remoting worker.")
private Integer workerWriteThreads;
public Remoting() {
super();
this.key = "remoting";
this.pcs = new PropertyChangeSupport(this);
}
public String getKey() {
return this.key;
}
/**
* Adds a property change listener
*/
public void addPropertyChangeListener(PropertyChangeListener listener) {
if (null == this.pcs)
this.pcs = new PropertyChangeSupport(this);
this.pcs.addPropertyChangeListener(listener);
}
/**
* Removes a property change listener
*/
public void removePropertyChangeListener(
java.beans.PropertyChangeListener listener) {
if (this.pcs != null)
this.pcs.removePropertyChangeListener(listener);
}
public RemotingResources subresources() {
return this.subresources;
}
/**
* Add all HTTPConnector objects to this subresource
*
* @return this
* @param value
* List of HTTPConnector objects.
*/
@SuppressWarnings("unchecked")
public T httpConnectors(java.util.List value) {
this.subresources.httpConnectors = value;
return (T) this;
}
/**
* Add the HTTPConnector object to the list of subresources
*
* @param value
* The HTTPConnector to add
* @return this
*/
@SuppressWarnings("unchecked")
public T httpConnector(HTTPConnector value) {
this.subresources.httpConnectors.add(value);
return (T) this;
}
/**
* Create and configure a HTTPConnector object to the list of subresources
*
* @param key
* The key for the HTTPConnector resource
* @param config
* The HTTPConnectorConsumer to use
* @return this
*/
@SuppressWarnings("unchecked")
public T httpConnector(java.lang.String childKey,
HTTPConnectorConsumer consumer) {
HTTPConnector extends HTTPConnector> child = new HTTPConnector<>(
childKey);
if (consumer != null) {
consumer.accept(child);
}
httpConnector(child);
return (T) this;
}
/**
* Create and configure a HTTPConnector object to the list of subresources
*
* @param key
* The key for the HTTPConnector resource
* @return this
*/
@SuppressWarnings("unchecked")
public T httpConnector(java.lang.String childKey) {
httpConnector(childKey, null);
return (T) this;
}
/**
* Install a supplied HTTPConnector object to the list of subresources
*/
@SuppressWarnings("unchecked")
public T httpConnector(HTTPConnectorSupplier supplier) {
httpConnector(supplier.get());
return (T) this;
}
/**
* Add all Connector objects to this subresource
*
* @return this
* @param value
* List of Connector objects.
*/
@SuppressWarnings("unchecked")
public T connectors(java.util.List value) {
this.subresources.connectors = value;
return (T) this;
}
/**
* Add the Connector object to the list of subresources
*
* @param value
* The Connector to add
* @return this
*/
@SuppressWarnings("unchecked")
public T connector(Connector value) {
this.subresources.connectors.add(value);
return (T) this;
}
/**
* Create and configure a Connector object to the list of subresources
*
* @param key
* The key for the Connector resource
* @param config
* The ConnectorConsumer to use
* @return this
*/
@SuppressWarnings("unchecked")
public T connector(java.lang.String childKey, ConnectorConsumer consumer) {
Connector extends Connector> child = new Connector<>(childKey);
if (consumer != null) {
consumer.accept(child);
}
connector(child);
return (T) this;
}
/**
* Create and configure a Connector object to the list of subresources
*
* @param key
* The key for the Connector resource
* @return this
*/
@SuppressWarnings("unchecked")
public T connector(java.lang.String childKey) {
connector(childKey, null);
return (T) this;
}
/**
* Install a supplied Connector object to the list of subresources
*/
@SuppressWarnings("unchecked")
public T connector(ConnectorSupplier supplier) {
connector(supplier.get());
return (T) this;
}
/**
* Add all OutboundConnection objects to this subresource
*
* @return this
* @param value
* List of OutboundConnection objects.
*/
@SuppressWarnings("unchecked")
public T outboundConnections(java.util.List value) {
this.subresources.outboundConnections = value;
return (T) this;
}
/**
* Add the OutboundConnection object to the list of subresources
*
* @param value
* The OutboundConnection to add
* @return this
*/
@SuppressWarnings("unchecked")
public T outboundConnection(OutboundConnection value) {
this.subresources.outboundConnections.add(value);
return (T) this;
}
/**
* Create and configure a OutboundConnection object to the list of
* subresources
*
* @param key
* The key for the OutboundConnection resource
* @param config
* The OutboundConnectionConsumer to use
* @return this
*/
@SuppressWarnings("unchecked")
public T outboundConnection(java.lang.String childKey,
OutboundConnectionConsumer consumer) {
OutboundConnection extends OutboundConnection> child = new OutboundConnection<>(
childKey);
if (consumer != null) {
consumer.accept(child);
}
outboundConnection(child);
return (T) this;
}
/**
* Create and configure a OutboundConnection object to the list of
* subresources
*
* @param key
* The key for the OutboundConnection resource
* @return this
*/
@SuppressWarnings("unchecked")
public T outboundConnection(java.lang.String childKey) {
outboundConnection(childKey, null);
return (T) this;
}
/**
* Install a supplied OutboundConnection object to the list of subresources
*/
@SuppressWarnings("unchecked")
public T outboundConnection(OutboundConnectionSupplier supplier) {
outboundConnection(supplier.get());
return (T) this;
}
/**
* Add all LocalOutboundConnection objects to this subresource
*
* @return this
* @param value
* List of LocalOutboundConnection objects.
*/
@SuppressWarnings("unchecked")
public T localOutboundConnections(
java.util.List value) {
this.subresources.localOutboundConnections = value;
return (T) this;
}
/**
* Add the LocalOutboundConnection object to the list of subresources
*
* @param value
* The LocalOutboundConnection to add
* @return this
*/
@SuppressWarnings("unchecked")
public T localOutboundConnection(LocalOutboundConnection value) {
this.subresources.localOutboundConnections.add(value);
return (T) this;
}
/**
* Create and configure a LocalOutboundConnection object to the list of
* subresources
*
* @param key
* The key for the LocalOutboundConnection resource
* @param config
* The LocalOutboundConnectionConsumer to use
* @return this
*/
@SuppressWarnings("unchecked")
public T localOutboundConnection(java.lang.String childKey,
LocalOutboundConnectionConsumer consumer) {
LocalOutboundConnection extends LocalOutboundConnection> child = new LocalOutboundConnection<>(
childKey);
if (consumer != null) {
consumer.accept(child);
}
localOutboundConnection(child);
return (T) this;
}
/**
* Create and configure a LocalOutboundConnection object to the list of
* subresources
*
* @param key
* The key for the LocalOutboundConnection resource
* @return this
*/
@SuppressWarnings("unchecked")
public T localOutboundConnection(java.lang.String childKey) {
localOutboundConnection(childKey, null);
return (T) this;
}
/**
* Install a supplied LocalOutboundConnection object to the list of
* subresources
*/
@SuppressWarnings("unchecked")
public T localOutboundConnection(LocalOutboundConnectionSupplier supplier) {
localOutboundConnection(supplier.get());
return (T) this;
}
/**
* Add all RemoteOutboundConnection objects to this subresource
*
* @return this
* @param value
* List of RemoteOutboundConnection objects.
*/
@SuppressWarnings("unchecked")
public T remoteOutboundConnections(
java.util.List value) {
this.subresources.remoteOutboundConnections = value;
return (T) this;
}
/**
* Add the RemoteOutboundConnection object to the list of subresources
*
* @param value
* The RemoteOutboundConnection to add
* @return this
*/
@SuppressWarnings("unchecked")
public T remoteOutboundConnection(RemoteOutboundConnection value) {
this.subresources.remoteOutboundConnections.add(value);
return (T) this;
}
/**
* Create and configure a RemoteOutboundConnection object to the list of
* subresources
*
* @param key
* The key for the RemoteOutboundConnection resource
* @param config
* The RemoteOutboundConnectionConsumer to use
* @return this
*/
@SuppressWarnings("unchecked")
public T remoteOutboundConnection(java.lang.String childKey,
RemoteOutboundConnectionConsumer consumer) {
RemoteOutboundConnection extends RemoteOutboundConnection> child = new RemoteOutboundConnection<>(
childKey);
if (consumer != null) {
consumer.accept(child);
}
remoteOutboundConnection(child);
return (T) this;
}
/**
* Create and configure a RemoteOutboundConnection object to the list of
* subresources
*
* @param key
* The key for the RemoteOutboundConnection resource
* @return this
*/
@SuppressWarnings("unchecked")
public T remoteOutboundConnection(java.lang.String childKey) {
remoteOutboundConnection(childKey, null);
return (T) this;
}
/**
* Install a supplied RemoteOutboundConnection object to the list of
* subresources
*/
@SuppressWarnings("unchecked")
public T remoteOutboundConnection(RemoteOutboundConnectionSupplier supplier) {
remoteOutboundConnection(supplier.get());
return (T) this;
}
/**
* Endpoint configuration
*/
@SuppressWarnings("unchecked")
public T endpointConfiguration(EndpointConfiguration value) {
this.subresources.endpointConfiguration = value;
return (T) this;
}
/**
* Endpoint configuration
*/
@SuppressWarnings("unchecked")
public T endpointConfiguration(EndpointConfigurationConsumer consumer) {
EndpointConfiguration extends EndpointConfiguration> child = new EndpointConfiguration<>();
if (consumer != null) {
consumer.accept(child);
}
this.subresources.endpointConfiguration = child;
return (T) this;
}
/**
* Endpoint configuration
*/
@SuppressWarnings("unchecked")
public T endpointConfiguration() {
EndpointConfiguration extends EndpointConfiguration> child = new EndpointConfiguration<>();
this.subresources.endpointConfiguration = child;
return (T) this;
}
/**
* Endpoint configuration
*/
@SuppressWarnings("unchecked")
public T endpointConfiguration(EndpointConfigurationSupplier supplier) {
this.subresources.endpointConfiguration = supplier.get();
return (T) this;
}
/**
* Child mutators for Remoting
*/
public static class RemotingResources {
/**
* The configuration of a HTTP Upgrade based Remoting connector.
*/
@ResourceDocumentation("The configuration of a HTTP Upgrade based Remoting connector.")
@SubresourceInfo("httpConnector")
private List httpConnectors = new java.util.ArrayList<>();
/**
* The configuration of a Remoting connector.
*/
@ResourceDocumentation("The configuration of a Remoting connector.")
@SubresourceInfo("connector")
private List connectors = new java.util.ArrayList<>();
/**
* Remoting outbound connection.
*/
@ResourceDocumentation("Remoting outbound connection.")
@SubresourceInfo("outboundConnection")
private List outboundConnections = new java.util.ArrayList<>();
/**
* Remoting outbound connection with an implicit local:// URI scheme.
*/
@ResourceDocumentation("Remoting outbound connection with an implicit local:// URI scheme.")
@SubresourceInfo("localOutboundConnection")
private List localOutboundConnections = new java.util.ArrayList<>();
/**
* Remoting outbound connection.
*/
@ResourceDocumentation("Remoting outbound connection.")
@SubresourceInfo("remoteOutboundConnection")
private List remoteOutboundConnections = new java.util.ArrayList<>();
@SingletonResource
@ResourceDocumentation("Endpoint configuration")
private EndpointConfiguration endpointConfiguration;
/**
* Get the list of HTTPConnector resources
*
* @return the list of resources
*/
@Subresource
public List httpConnectors() {
return this.httpConnectors;
}
public HTTPConnector httpConnector(java.lang.String key) {
return this.httpConnectors.stream()
.filter(e -> e.getKey().equals(key)).findFirst()
.orElse(null);
}
/**
* Get the list of Connector resources
*
* @return the list of resources
*/
@Subresource
public List connectors() {
return this.connectors;
}
public Connector connector(java.lang.String key) {
return this.connectors.stream().filter(e -> e.getKey().equals(key))
.findFirst().orElse(null);
}
/**
* Get the list of OutboundConnection resources
*
* @return the list of resources
*/
@Subresource
public List outboundConnections() {
return this.outboundConnections;
}
public OutboundConnection outboundConnection(java.lang.String key) {
return this.outboundConnections.stream()
.filter(e -> e.getKey().equals(key)).findFirst()
.orElse(null);
}
/**
* Get the list of LocalOutboundConnection resources
*
* @return the list of resources
*/
@Subresource
public List localOutboundConnections() {
return this.localOutboundConnections;
}
public LocalOutboundConnection localOutboundConnection(
java.lang.String key) {
return this.localOutboundConnections.stream()
.filter(e -> e.getKey().equals(key)).findFirst()
.orElse(null);
}
/**
* Get the list of RemoteOutboundConnection resources
*
* @return the list of resources
*/
@Subresource
public List remoteOutboundConnections() {
return this.remoteOutboundConnections;
}
public RemoteOutboundConnection remoteOutboundConnection(
java.lang.String key) {
return this.remoteOutboundConnections.stream()
.filter(e -> e.getKey().equals(key)).findFirst()
.orElse(null);
}
/**
* Endpoint configuration
*/
@Subresource
public EndpointConfiguration endpointConfiguration() {
return this.endpointConfiguration;
}
}
/**
* The number of read threads to create for the remoting worker.
*/
@ModelNodeBinding(detypedName = "worker-read-threads")
public Integer workerReadThreads() {
return this.workerReadThreads;
}
/**
* The number of read threads to create for the remoting worker.
*/
@SuppressWarnings("unchecked")
public T workerReadThreads(java.lang.Integer value) {
Object oldValue = this.workerReadThreads;
this.workerReadThreads = value;
if (this.pcs != null)
this.pcs.firePropertyChange("workerReadThreads", oldValue, value);
return (T) this;
}
/**
* The number of core threads for the remoting worker task thread pool.
*/
@ModelNodeBinding(detypedName = "worker-task-core-threads")
public Integer workerTaskCoreThreads() {
return this.workerTaskCoreThreads;
}
/**
* The number of core threads for the remoting worker task thread pool.
*/
@SuppressWarnings("unchecked")
public T workerTaskCoreThreads(java.lang.Integer value) {
Object oldValue = this.workerTaskCoreThreads;
this.workerTaskCoreThreads = value;
if (this.pcs != null)
this.pcs.firePropertyChange("workerTaskCoreThreads", oldValue,
value);
return (T) this;
}
/**
* The number of milliseconds to keep non-core remoting worker task threads
* alive.
*/
@ModelNodeBinding(detypedName = "worker-task-keepalive")
public Integer workerTaskKeepalive() {
return this.workerTaskKeepalive;
}
/**
* The number of milliseconds to keep non-core remoting worker task threads
* alive.
*/
@SuppressWarnings("unchecked")
public T workerTaskKeepalive(java.lang.Integer value) {
Object oldValue = this.workerTaskKeepalive;
this.workerTaskKeepalive = value;
if (this.pcs != null)
this.pcs.firePropertyChange("workerTaskKeepalive", oldValue, value);
return (T) this;
}
/**
* The maximum number of remoting worker tasks to allow before rejecting.
*/
@ModelNodeBinding(detypedName = "worker-task-limit")
public Integer workerTaskLimit() {
return this.workerTaskLimit;
}
/**
* The maximum number of remoting worker tasks to allow before rejecting.
*/
@SuppressWarnings("unchecked")
public T workerTaskLimit(java.lang.Integer value) {
Object oldValue = this.workerTaskLimit;
this.workerTaskLimit = value;
if (this.pcs != null)
this.pcs.firePropertyChange("workerTaskLimit", oldValue, value);
return (T) this;
}
/**
* The maximum number of threads for the remoting worker task thread pool.
*/
@ModelNodeBinding(detypedName = "worker-task-max-threads")
public Integer workerTaskMaxThreads() {
return this.workerTaskMaxThreads;
}
/**
* The maximum number of threads for the remoting worker task thread pool.
*/
@SuppressWarnings("unchecked")
public T workerTaskMaxThreads(java.lang.Integer value) {
Object oldValue = this.workerTaskMaxThreads;
this.workerTaskMaxThreads = value;
if (this.pcs != null)
this.pcs.firePropertyChange("workerTaskMaxThreads", oldValue, value);
return (T) this;
}
/**
* The number of write threads to create for the remoting worker.
*/
@ModelNodeBinding(detypedName = "worker-write-threads")
public Integer workerWriteThreads() {
return this.workerWriteThreads;
}
/**
* The number of write threads to create for the remoting worker.
*/
@SuppressWarnings("unchecked")
public T workerWriteThreads(java.lang.Integer value) {
Object oldValue = this.workerWriteThreads;
this.workerWriteThreads = value;
if (this.pcs != null)
this.pcs.firePropertyChange("workerWriteThreads", oldValue, value);
return (T) this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy