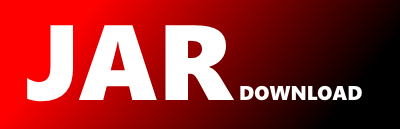
org.wildfly.swarm.config.ejb3.StrictMaxBeanInstancePool Maven / Gradle / Ivy
package org.wildfly.swarm.config.ejb3;
import org.wildfly.swarm.config.runtime.AttributeDocumentation;
import org.wildfly.swarm.config.runtime.ResourceDocumentation;
import org.wildfly.swarm.config.runtime.SingletonResource;
import org.wildfly.swarm.config.runtime.Address;
import org.wildfly.swarm.config.runtime.ResourceType;
import java.beans.PropertyChangeSupport;
import java.beans.PropertyChangeListener;
import org.wildfly.swarm.config.runtime.ModelNodeBinding;
import java.util.Arrays;
/**
* A bean instance pool with a strict upper limit
*/
@Address("/subsystem=ejb3/strict-max-bean-instance-pool=*")
@ResourceType("strict-max-bean-instance-pool")
public class StrictMaxBeanInstancePool>
implements
org.wildfly.swarm.config.runtime.Keyed {
private String key;
private PropertyChangeSupport pcs;
@AttributeDocumentation("Specifies if and what the max pool size should be derived from. An undefined value (or the deprecated value 'none' which is converted to undefined) indicates that the explicit value of max-pool-size should be used. A value of 'from-worker-pools' indicates that the max pool size should be derived from the size of the total threads for all worker pools configured on the system. A value of 'from-cpu-count' indicates that the max pool size should be derived from the total number of processors available on the system. Note that the computation isn't a 1:1 mapping, the values may or may not be augmented by other factors.")
private DeriveSize deriveSize;
@AttributeDocumentation("The maximum number of bean instances that the pool can hold at a given point in time")
private Integer maxPoolSize;
@AttributeDocumentation("The maximum amount of time to wait for a bean instance to be available from the pool")
private Long timeout;
@AttributeDocumentation("The instance acquisition timeout unit")
private TimeoutUnit timeoutUnit;
public StrictMaxBeanInstancePool(java.lang.String key) {
super();
this.key = key;
}
public String getKey() {
return this.key;
}
/**
* Adds a property change listener
*/
public void addPropertyChangeListener(PropertyChangeListener listener) {
if (null == this.pcs)
this.pcs = new PropertyChangeSupport(this);
this.pcs.addPropertyChangeListener(listener);
}
/**
* Removes a property change listener
*/
public void removePropertyChangeListener(
java.beans.PropertyChangeListener listener) {
if (this.pcs != null)
this.pcs.removePropertyChangeListener(listener);
}
public static enum DeriveSize {
FROM_WORKER_POOLS("from-worker-pools"), FROM_CPU_COUNT("from-cpu-count");
private final String allowedValue;
/**
* Returns the allowed value for the management model.
*
* @return the allowed model value
*/
public String getAllowedValue() {
return allowedValue;
}
DeriveSize(java.lang.String allowedValue) {
this.allowedValue = allowedValue;
}
@Override
public String toString() {
return allowedValue;
}
}
public static enum TimeoutUnit {
NANOSECONDS("NANOSECONDS"), MICROSECONDS("MICROSECONDS"), MILLISECONDS(
"MILLISECONDS"), SECONDS("SECONDS"), MINUTES("MINUTES"), HOURS(
"HOURS"), DAYS("DAYS");
private final String allowedValue;
/**
* Returns the allowed value for the management model.
*
* @return the allowed model value
*/
public String getAllowedValue() {
return allowedValue;
}
TimeoutUnit(java.lang.String allowedValue) {
this.allowedValue = allowedValue;
}
@Override
public String toString() {
return allowedValue;
}
}
/**
* Specifies if and what the max pool size should be derived from. An
* undefined value (or the deprecated value 'none' which is converted to
* undefined) indicates that the explicit value of max-pool-size should be
* used. A value of 'from-worker-pools' indicates that the max pool size
* should be derived from the size of the total threads for all worker pools
* configured on the system. A value of 'from-cpu-count' indicates that the
* max pool size should be derived from the total number of processors
* available on the system. Note that the computation isn't a 1:1 mapping,
* the values may or may not be augmented by other factors.
*/
@ModelNodeBinding(detypedName = "derive-size")
public DeriveSize deriveSize() {
return this.deriveSize;
}
/**
* Specifies if and what the max pool size should be derived from. An
* undefined value (or the deprecated value 'none' which is converted to
* undefined) indicates that the explicit value of max-pool-size should be
* used. A value of 'from-worker-pools' indicates that the max pool size
* should be derived from the size of the total threads for all worker pools
* configured on the system. A value of 'from-cpu-count' indicates that the
* max pool size should be derived from the total number of processors
* available on the system. Note that the computation isn't a 1:1 mapping,
* the values may or may not be augmented by other factors.
*/
@SuppressWarnings("unchecked")
public T deriveSize(DeriveSize value) {
Object oldValue = this.deriveSize;
this.deriveSize = value;
if (this.pcs != null)
this.pcs.firePropertyChange("deriveSize", oldValue, value);
return (T) this;
}
/**
* The maximum number of bean instances that the pool can hold at a given
* point in time
*/
@ModelNodeBinding(detypedName = "max-pool-size")
public Integer maxPoolSize() {
return this.maxPoolSize;
}
/**
* The maximum number of bean instances that the pool can hold at a given
* point in time
*/
@SuppressWarnings("unchecked")
public T maxPoolSize(java.lang.Integer value) {
Object oldValue = this.maxPoolSize;
this.maxPoolSize = value;
if (this.pcs != null)
this.pcs.firePropertyChange("maxPoolSize", oldValue, value);
return (T) this;
}
/**
* The maximum amount of time to wait for a bean instance to be available
* from the pool
*/
@ModelNodeBinding(detypedName = "timeout")
public Long timeout() {
return this.timeout;
}
/**
* The maximum amount of time to wait for a bean instance to be available
* from the pool
*/
@SuppressWarnings("unchecked")
public T timeout(java.lang.Long value) {
Object oldValue = this.timeout;
this.timeout = value;
if (this.pcs != null)
this.pcs.firePropertyChange("timeout", oldValue, value);
return (T) this;
}
/**
* The instance acquisition timeout unit
*/
@ModelNodeBinding(detypedName = "timeout-unit")
public TimeoutUnit timeoutUnit() {
return this.timeoutUnit;
}
/**
* The instance acquisition timeout unit
*/
@SuppressWarnings("unchecked")
public T timeoutUnit(TimeoutUnit value) {
Object oldValue = this.timeoutUnit;
this.timeoutUnit = value;
if (this.pcs != null)
this.pcs.firePropertyChange("timeoutUnit", oldValue, value);
return (T) this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy