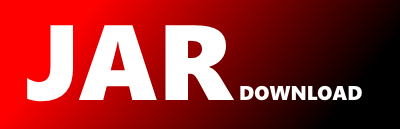
org.wildfly.swarm.config.elytron.KeyStore Maven / Gradle / Ivy
package org.wildfly.swarm.config.elytron;
import org.wildfly.swarm.config.runtime.AttributeDocumentation;
import org.wildfly.swarm.config.runtime.ResourceDocumentation;
import org.wildfly.swarm.config.runtime.SingletonResource;
import org.wildfly.swarm.config.runtime.Address;
import org.wildfly.swarm.config.runtime.ResourceType;
import java.beans.PropertyChangeSupport;
import java.beans.PropertyChangeListener;
import org.wildfly.swarm.config.runtime.ModelNodeBinding;
import java.util.Map;
import java.util.Arrays;
import org.wildfly.swarm.config.elytron.State;
/**
* A KeyStore definition.
*/
@Address("/subsystem=elytron/key-store=*")
@ResourceType("key-store")
public class KeyStore>
implements
org.wildfly.swarm.config.runtime.Keyed {
private String key;
private PropertyChangeSupport pcs;
@AttributeDocumentation("A filter to apply to the aliases returned from the KeyStore, can either be a comma separated list of aliases to return or one of the following formats ALL:-alias1:-alias2, NONE:+alias1:+alias2")
private String aliasFilter;
@AttributeDocumentation("The reference to credential stored in CredentialStore under defined alias or clear text password.")
private Map credentialReference;
@AttributeDocumentation("Information about the provider that was used for this KeyStore.")
private Map loadedProvider;
@AttributeDocumentation("Indicates if the in-memory representation of the KeyStore has been changed since it was last loaded or stored. Note: For some providers updates may be immediate without further load or store calls.")
private Boolean modified;
@AttributeDocumentation("The number of entries in the KeyStore.")
private Integer size;
@AttributeDocumentation("The state of the underlying service that represents this KeyStore at runtime, if it is anything other than UP runtime operations will not be available.")
private State state;
@AttributeDocumentation("The time this KeyStore was last loaded or saved. Note: Some providers may continue to apply updates after the KeyStore was loaded within the application server.")
private String attributeSynchronized;
@AttributeDocumentation("The path to the KeyStore file.")
private String path;
@AttributeDocumentation("The base path this store is relative to.")
private String relativeTo;
@AttributeDocumentation("Is the file required to exist at the time the KeyStore service starts?")
private Boolean required;
@AttributeDocumentation("The name of the provider to use to load the KeyStore, disables searching for the first Provider that can create a KeyStore of the specified type.")
private String providerName;
@AttributeDocumentation("A reference to the providers that should be used to obtain the list of Provider instances to search, if not specified the global list of providers will be used instead.")
private String providers;
@AttributeDocumentation("The type of the KeyStore, used when creating the new KeyStore instance.")
private String type;
public KeyStore(java.lang.String key) {
super();
this.key = key;
}
public String getKey() {
return this.key;
}
/**
* Adds a property change listener
*/
public void addPropertyChangeListener(PropertyChangeListener listener) {
if (null == this.pcs)
this.pcs = new PropertyChangeSupport(this);
this.pcs.addPropertyChangeListener(listener);
}
/**
* Removes a property change listener
*/
public void removePropertyChangeListener(
java.beans.PropertyChangeListener listener) {
if (this.pcs != null)
this.pcs.removePropertyChangeListener(listener);
}
/**
* A filter to apply to the aliases returned from the KeyStore, can either
* be a comma separated list of aliases to return or one of the following
* formats ALL:-alias1:-alias2, NONE:+alias1:+alias2
*/
@ModelNodeBinding(detypedName = "alias-filter")
public String aliasFilter() {
return this.aliasFilter;
}
/**
* A filter to apply to the aliases returned from the KeyStore, can either
* be a comma separated list of aliases to return or one of the following
* formats ALL:-alias1:-alias2, NONE:+alias1:+alias2
*/
@SuppressWarnings("unchecked")
public T aliasFilter(java.lang.String value) {
Object oldValue = this.aliasFilter;
this.aliasFilter = value;
if (this.pcs != null)
this.pcs.firePropertyChange("aliasFilter", oldValue, value);
return (T) this;
}
/**
* The reference to credential stored in CredentialStore under defined alias
* or clear text password.
*/
@ModelNodeBinding(detypedName = "credential-reference")
public Map credentialReference() {
return this.credentialReference;
}
/**
* The reference to credential stored in CredentialStore under defined alias
* or clear text password.
*/
@SuppressWarnings("unchecked")
public T credentialReference(java.util.Map value) {
Object oldValue = this.credentialReference;
this.credentialReference = value;
if (this.pcs != null)
this.pcs.firePropertyChange("credentialReference", oldValue, value);
return (T) this;
}
/**
* The reference to credential stored in CredentialStore under defined alias
* or clear text password.
*/
@SuppressWarnings("unchecked")
public T credentialReference(java.lang.String key, java.lang.Object value) {
if (this.credentialReference == null) {
this.credentialReference = new java.util.HashMap<>();
}
this.credentialReference.put(key, value);
return (T) this;
}
/**
* Information about the provider that was used for this KeyStore.
*/
@ModelNodeBinding(detypedName = "loaded-provider")
public Map loadedProvider() {
return this.loadedProvider;
}
/**
* Information about the provider that was used for this KeyStore.
*/
@SuppressWarnings("unchecked")
public T loadedProvider(java.util.Map value) {
Object oldValue = this.loadedProvider;
this.loadedProvider = value;
if (this.pcs != null)
this.pcs.firePropertyChange("loadedProvider", oldValue, value);
return (T) this;
}
/**
* Information about the provider that was used for this KeyStore.
*/
@SuppressWarnings("unchecked")
public T loadedProvider(java.lang.String key, java.lang.Object value) {
if (this.loadedProvider == null) {
this.loadedProvider = new java.util.HashMap<>();
}
this.loadedProvider.put(key, value);
return (T) this;
}
/**
* Indicates if the in-memory representation of the KeyStore has been
* changed since it was last loaded or stored. Note: For some providers
* updates may be immediate without further load or store calls.
*/
@ModelNodeBinding(detypedName = "modified")
public Boolean modified() {
return this.modified;
}
/**
* Indicates if the in-memory representation of the KeyStore has been
* changed since it was last loaded or stored. Note: For some providers
* updates may be immediate without further load or store calls.
*/
@SuppressWarnings("unchecked")
public T modified(java.lang.Boolean value) {
Object oldValue = this.modified;
this.modified = value;
if (this.pcs != null)
this.pcs.firePropertyChange("modified", oldValue, value);
return (T) this;
}
/**
* The number of entries in the KeyStore.
*/
@ModelNodeBinding(detypedName = "size")
public Integer size() {
return this.size;
}
/**
* The number of entries in the KeyStore.
*/
@SuppressWarnings("unchecked")
public T size(java.lang.Integer value) {
Object oldValue = this.size;
this.size = value;
if (this.pcs != null)
this.pcs.firePropertyChange("size", oldValue, value);
return (T) this;
}
/**
* The state of the underlying service that represents this KeyStore at
* runtime, if it is anything other than UP runtime operations will not be
* available.
*/
@ModelNodeBinding(detypedName = "state")
public State state() {
return this.state;
}
/**
* The state of the underlying service that represents this KeyStore at
* runtime, if it is anything other than UP runtime operations will not be
* available.
*/
@SuppressWarnings("unchecked")
public T state(State value) {
Object oldValue = this.state;
this.state = value;
if (this.pcs != null)
this.pcs.firePropertyChange("state", oldValue, value);
return (T) this;
}
/**
* The time this KeyStore was last loaded or saved. Note: Some providers may
* continue to apply updates after the KeyStore was loaded within the
* application server.
*/
@ModelNodeBinding(detypedName = "synchronized")
public String attributeSynchronized() {
return this.attributeSynchronized;
}
/**
* The time this KeyStore was last loaded or saved. Note: Some providers may
* continue to apply updates after the KeyStore was loaded within the
* application server.
*/
@SuppressWarnings("unchecked")
public T attributeSynchronized(java.lang.String value) {
Object oldValue = this.attributeSynchronized;
this.attributeSynchronized = value;
if (this.pcs != null)
this.pcs.firePropertyChange("attributeSynchronized", oldValue,
value);
return (T) this;
}
/**
* The path to the KeyStore file.
*/
@ModelNodeBinding(detypedName = "path")
public String path() {
return this.path;
}
/**
* The path to the KeyStore file.
*/
@SuppressWarnings("unchecked")
public T path(java.lang.String value) {
Object oldValue = this.path;
this.path = value;
if (this.pcs != null)
this.pcs.firePropertyChange("path", oldValue, value);
return (T) this;
}
/**
* The base path this store is relative to.
*/
@ModelNodeBinding(detypedName = "relative-to")
public String relativeTo() {
return this.relativeTo;
}
/**
* The base path this store is relative to.
*/
@SuppressWarnings("unchecked")
public T relativeTo(java.lang.String value) {
Object oldValue = this.relativeTo;
this.relativeTo = value;
if (this.pcs != null)
this.pcs.firePropertyChange("relativeTo", oldValue, value);
return (T) this;
}
/**
* Is the file required to exist at the time the KeyStore service starts?
*/
@ModelNodeBinding(detypedName = "required")
public Boolean required() {
return this.required;
}
/**
* Is the file required to exist at the time the KeyStore service starts?
*/
@SuppressWarnings("unchecked")
public T required(java.lang.Boolean value) {
Object oldValue = this.required;
this.required = value;
if (this.pcs != null)
this.pcs.firePropertyChange("required", oldValue, value);
return (T) this;
}
/**
* The name of the provider to use to load the KeyStore, disables searching
* for the first Provider that can create a KeyStore of the specified type.
*/
@ModelNodeBinding(detypedName = "provider-name")
public String providerName() {
return this.providerName;
}
/**
* The name of the provider to use to load the KeyStore, disables searching
* for the first Provider that can create a KeyStore of the specified type.
*/
@SuppressWarnings("unchecked")
public T providerName(java.lang.String value) {
Object oldValue = this.providerName;
this.providerName = value;
if (this.pcs != null)
this.pcs.firePropertyChange("providerName", oldValue, value);
return (T) this;
}
/**
* A reference to the providers that should be used to obtain the list of
* Provider instances to search, if not specified the global list of
* providers will be used instead.
*/
@ModelNodeBinding(detypedName = "providers")
public String providers() {
return this.providers;
}
/**
* A reference to the providers that should be used to obtain the list of
* Provider instances to search, if not specified the global list of
* providers will be used instead.
*/
@SuppressWarnings("unchecked")
public T providers(java.lang.String value) {
Object oldValue = this.providers;
this.providers = value;
if (this.pcs != null)
this.pcs.firePropertyChange("providers", oldValue, value);
return (T) this;
}
/**
* The type of the KeyStore, used when creating the new KeyStore instance.
*/
@ModelNodeBinding(detypedName = "type")
public String type() {
return this.type;
}
/**
* The type of the KeyStore, used when creating the new KeyStore instance.
*/
@SuppressWarnings("unchecked")
public T type(java.lang.String value) {
Object oldValue = this.type;
this.type = value;
if (this.pcs != null)
this.pcs.firePropertyChange("type", oldValue, value);
return (T) this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy