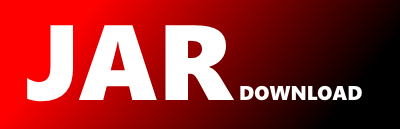
org.wildfly.swarm.config.logging.Logger Maven / Gradle / Ivy
package org.wildfly.swarm.config.logging;
import org.wildfly.swarm.config.runtime.AttributeDocumentation;
import org.wildfly.swarm.config.runtime.ResourceDocumentation;
import org.wildfly.swarm.config.runtime.SingletonResource;
import org.wildfly.swarm.config.runtime.Addresses;
import org.wildfly.swarm.config.runtime.ResourceType;
import java.beans.PropertyChangeSupport;
import java.beans.PropertyChangeListener;
import org.wildfly.swarm.config.runtime.ModelNodeBinding;
import java.util.List;
import java.util.Arrays;
import java.util.stream.Collectors;
import org.wildfly.swarm.config.logging.Level;
/**
* Defines a logger category.
*/
@Addresses({"/subsystem=logging/logger=*",
"/subsystem=logging/logging-profile=*/logger=*"})
@ResourceType("logger")
public class Logger>
implements
org.wildfly.swarm.config.runtime.Keyed {
private String key;
private PropertyChangeSupport pcs;
@AttributeDocumentation("Specifies the category for the logger.")
private String category;
@AttributeDocumentation("A filter expression value to define a filter. Example for a filter that does not match a pattern: not(match(\"JBAS.*\"))")
private String filterSpec;
@AttributeDocumentation("The handlers associated with the logger.")
private List handlers;
@AttributeDocumentation("The log level specifying which message levels will be logged by the logger. Message levels lower than this value will be discarded.")
private Level level;
@AttributeDocumentation("Specifies whether or not this logger should send its output to its parent Logger.")
private Boolean useParentHandlers;
public Logger(java.lang.String key) {
super();
this.key = key;
}
public String getKey() {
return this.key;
}
/**
* Adds a property change listener
*/
public void addPropertyChangeListener(PropertyChangeListener listener) {
if (null == this.pcs)
this.pcs = new PropertyChangeSupport(this);
this.pcs.addPropertyChangeListener(listener);
}
/**
* Removes a property change listener
*/
public void removePropertyChangeListener(
java.beans.PropertyChangeListener listener) {
if (this.pcs != null)
this.pcs.removePropertyChangeListener(listener);
}
/**
* Specifies the category for the logger.
*/
@ModelNodeBinding(detypedName = "category")
public String category() {
return this.category;
}
/**
* Specifies the category for the logger.
*/
@SuppressWarnings("unchecked")
public T category(java.lang.String value) {
Object oldValue = this.category;
this.category = value;
if (this.pcs != null)
this.pcs.firePropertyChange("category", oldValue, value);
return (T) this;
}
/**
* A filter expression value to define a filter. Example for a filter that
* does not match a pattern: not(match("JBAS.*"))
*/
@ModelNodeBinding(detypedName = "filter-spec")
public String filterSpec() {
return this.filterSpec;
}
/**
* A filter expression value to define a filter. Example for a filter that
* does not match a pattern: not(match("JBAS.*"))
*/
@SuppressWarnings("unchecked")
public T filterSpec(java.lang.String value) {
Object oldValue = this.filterSpec;
this.filterSpec = value;
if (this.pcs != null)
this.pcs.firePropertyChange("filterSpec", oldValue, value);
return (T) this;
}
/**
* The handlers associated with the logger.
*/
@ModelNodeBinding(detypedName = "handlers")
public List handlers() {
return this.handlers;
}
/**
* The handlers associated with the logger.
*/
@SuppressWarnings("unchecked")
public T handlers(java.util.List value) {
Object oldValue = this.handlers;
this.handlers = value;
if (this.pcs != null)
this.pcs.firePropertyChange("handlers", oldValue, value);
return (T) this;
}
/**
* The handlers associated with the logger.
*/
@SuppressWarnings("unchecked")
public T handler(String value) {
if (this.handlers == null) {
this.handlers = new java.util.ArrayList<>();
}
this.handlers.add(value);
return (T) this;
}
/**
* The handlers associated with the logger.
*/
@SuppressWarnings("unchecked")
public T handlers(String... args) {
handlers(Arrays.stream(args).collect(Collectors.toList()));
return (T) this;
}
/**
* The log level specifying which message levels will be logged by the
* logger. Message levels lower than this value will be discarded.
*/
@ModelNodeBinding(detypedName = "level")
public Level level() {
return this.level;
}
/**
* The log level specifying which message levels will be logged by the
* logger. Message levels lower than this value will be discarded.
*/
@SuppressWarnings("unchecked")
public T level(Level value) {
Object oldValue = this.level;
this.level = value;
if (this.pcs != null)
this.pcs.firePropertyChange("level", oldValue, value);
return (T) this;
}
/**
* Specifies whether or not this logger should send its output to its parent
* Logger.
*/
@ModelNodeBinding(detypedName = "use-parent-handlers")
public Boolean useParentHandlers() {
return this.useParentHandlers;
}
/**
* Specifies whether or not this logger should send its output to its parent
* Logger.
*/
@SuppressWarnings("unchecked")
public T useParentHandlers(java.lang.Boolean value) {
Object oldValue = this.useParentHandlers;
this.useParentHandlers = value;
if (this.pcs != null)
this.pcs.firePropertyChange("useParentHandlers", oldValue, value);
return (T) this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy