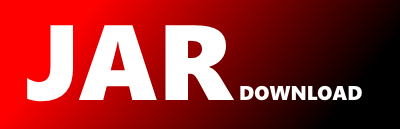
org.wildfly.swarm.config.undertow.configuration.File Maven / Gradle / Ivy
package org.wildfly.swarm.config.undertow.configuration;
import org.wildfly.swarm.config.runtime.AttributeDocumentation;
import org.wildfly.swarm.config.runtime.ResourceDocumentation;
import org.wildfly.swarm.config.runtime.SingletonResource;
import org.wildfly.swarm.config.runtime.Address;
import org.wildfly.swarm.config.runtime.ResourceType;
import java.beans.PropertyChangeSupport;
import java.beans.PropertyChangeListener;
import org.wildfly.swarm.config.runtime.ModelNodeBinding;
import java.util.List;
import java.util.Arrays;
import java.util.stream.Collectors;
/**
* File handler
*/
@Address("/subsystem=undertow/configuration=handler/file=*")
@ResourceType("file")
public class File>
implements
org.wildfly.swarm.config.runtime.Keyed {
private String key;
private PropertyChangeSupport pcs;
@AttributeDocumentation("Size of the buffers, in bytes.")
private Long cacheBufferSize;
@AttributeDocumentation("Number of buffers")
private Long cacheBuffers;
@AttributeDocumentation("Use case sensitive file handling")
private Boolean caseSensitive;
@AttributeDocumentation("Enable directory listing?")
private Boolean directoryListing;
@AttributeDocumentation("Enable following symbolic links")
private Boolean followSymlink;
@AttributeDocumentation("Path on filesystem from where file handler will serve resources")
private String path;
@AttributeDocumentation("Paths that are safe to be targets of symbolic links")
private List safeSymlinkPaths;
public File(java.lang.String key) {
super();
this.key = key;
}
public String getKey() {
return this.key;
}
/**
* Adds a property change listener
*/
public void addPropertyChangeListener(PropertyChangeListener listener) {
if (null == this.pcs)
this.pcs = new PropertyChangeSupport(this);
this.pcs.addPropertyChangeListener(listener);
}
/**
* Removes a property change listener
*/
public void removePropertyChangeListener(
java.beans.PropertyChangeListener listener) {
if (this.pcs != null)
this.pcs.removePropertyChangeListener(listener);
}
/**
* Size of the buffers, in bytes.
*/
@ModelNodeBinding(detypedName = "cache-buffer-size")
public Long cacheBufferSize() {
return this.cacheBufferSize;
}
/**
* Size of the buffers, in bytes.
*/
@SuppressWarnings("unchecked")
public T cacheBufferSize(java.lang.Long value) {
Object oldValue = this.cacheBufferSize;
this.cacheBufferSize = value;
if (this.pcs != null)
this.pcs.firePropertyChange("cacheBufferSize", oldValue, value);
return (T) this;
}
/**
* Number of buffers
*/
@ModelNodeBinding(detypedName = "cache-buffers")
public Long cacheBuffers() {
return this.cacheBuffers;
}
/**
* Number of buffers
*/
@SuppressWarnings("unchecked")
public T cacheBuffers(java.lang.Long value) {
Object oldValue = this.cacheBuffers;
this.cacheBuffers = value;
if (this.pcs != null)
this.pcs.firePropertyChange("cacheBuffers", oldValue, value);
return (T) this;
}
/**
* Use case sensitive file handling
*/
@ModelNodeBinding(detypedName = "case-sensitive")
public Boolean caseSensitive() {
return this.caseSensitive;
}
/**
* Use case sensitive file handling
*/
@SuppressWarnings("unchecked")
public T caseSensitive(java.lang.Boolean value) {
Object oldValue = this.caseSensitive;
this.caseSensitive = value;
if (this.pcs != null)
this.pcs.firePropertyChange("caseSensitive", oldValue, value);
return (T) this;
}
/**
* Enable directory listing?
*/
@ModelNodeBinding(detypedName = "directory-listing")
public Boolean directoryListing() {
return this.directoryListing;
}
/**
* Enable directory listing?
*/
@SuppressWarnings("unchecked")
public T directoryListing(java.lang.Boolean value) {
Object oldValue = this.directoryListing;
this.directoryListing = value;
if (this.pcs != null)
this.pcs.firePropertyChange("directoryListing", oldValue, value);
return (T) this;
}
/**
* Enable following symbolic links
*/
@ModelNodeBinding(detypedName = "follow-symlink")
public Boolean followSymlink() {
return this.followSymlink;
}
/**
* Enable following symbolic links
*/
@SuppressWarnings("unchecked")
public T followSymlink(java.lang.Boolean value) {
Object oldValue = this.followSymlink;
this.followSymlink = value;
if (this.pcs != null)
this.pcs.firePropertyChange("followSymlink", oldValue, value);
return (T) this;
}
/**
* Path on filesystem from where file handler will serve resources
*/
@ModelNodeBinding(detypedName = "path")
public String path() {
return this.path;
}
/**
* Path on filesystem from where file handler will serve resources
*/
@SuppressWarnings("unchecked")
public T path(java.lang.String value) {
Object oldValue = this.path;
this.path = value;
if (this.pcs != null)
this.pcs.firePropertyChange("path", oldValue, value);
return (T) this;
}
/**
* Paths that are safe to be targets of symbolic links
*/
@ModelNodeBinding(detypedName = "safe-symlink-paths")
public List safeSymlinkPaths() {
return this.safeSymlinkPaths;
}
/**
* Paths that are safe to be targets of symbolic links
*/
@SuppressWarnings("unchecked")
public T safeSymlinkPaths(java.util.List value) {
Object oldValue = this.safeSymlinkPaths;
this.safeSymlinkPaths = value;
if (this.pcs != null)
this.pcs.firePropertyChange("safeSymlinkPaths", oldValue, value);
return (T) this;
}
/**
* Paths that are safe to be targets of symbolic links
*/
@SuppressWarnings("unchecked")
public T safeSymlinkPath(String value) {
if (this.safeSymlinkPaths == null) {
this.safeSymlinkPaths = new java.util.ArrayList<>();
}
this.safeSymlinkPaths.add(value);
return (T) this;
}
/**
* Paths that are safe to be targets of symbolic links
*/
@SuppressWarnings("unchecked")
public T safeSymlinkPaths(String... args) {
safeSymlinkPaths(Arrays.stream(args).collect(Collectors.toList()));
return (T) this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy