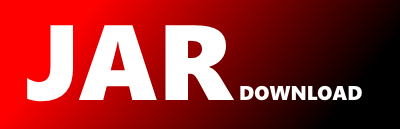
org.wildfly.swarm.config.Webservices Maven / Gradle / Ivy
package org.wildfly.swarm.config;
import org.wildfly.swarm.config.runtime.AttributeDocumentation;
import org.wildfly.swarm.config.runtime.ResourceDocumentation;
import org.wildfly.swarm.config.runtime.SingletonResource;
import org.wildfly.swarm.config.runtime.Address;
import org.wildfly.swarm.config.runtime.ResourceType;
import org.wildfly.swarm.config.runtime.Implicit;
import java.beans.PropertyChangeSupport;
import java.beans.PropertyChangeListener;
import java.util.List;
import org.wildfly.swarm.config.runtime.Subresource;
import org.wildfly.swarm.config.webservices.ClientConfigConsumer;
import org.wildfly.swarm.config.webservices.ClientConfigSupplier;
import org.wildfly.swarm.config.webservices.ClientConfig;
import org.wildfly.swarm.config.runtime.SubresourceInfo;
import org.wildfly.swarm.config.webservices.EndpointConfigConsumer;
import org.wildfly.swarm.config.webservices.EndpointConfigSupplier;
import org.wildfly.swarm.config.webservices.EndpointConfig;
import org.wildfly.swarm.config.runtime.ModelNodeBinding;
import java.util.Arrays;
/**
* The configuration of the web services subsystem.
*/
@Address("/subsystem=webservices")
@ResourceType("subsystem")
@Implicit
public class Webservices>
implements
org.wildfly.swarm.config.runtime.Keyed {
private String key;
private PropertyChangeSupport pcs;
private WebservicesResources subresources = new WebservicesResources();
@AttributeDocumentation("Whether the soap address can be modified.")
private Boolean modifyWsdlAddress;
@AttributeDocumentation("Whether statistics are to be gathered for endpoints, default value is 'false'.")
private Boolean statisticsEnabled;
@AttributeDocumentation("The WSDL, that is a required deployment artifact for an endpoint, has a element which points to the location of the endpoint. JBoss supports rewriting of that SOAP address. If the content of is a valid URL, JBossWS will not rewrite it unless 'modify-wsdl-address' is true. If the content of is not a valid URL, JBossWS will rewrite it using the attribute values given below. If 'wsdl-host' is set to 'jbossws.undefined.host', JBossWS uses requesters host when rewriting the ")
private String wsdlHost;
@AttributeDocumentation("This string defines a SED substitution command (e.g., 's/regexp/replacement/g') that JBossWS executes against the path component of each URL published from the server. When wsdl-path-rewrite-rule is not defined, JBossWS retains the original path component of each URL. When 'modify-wsdl-address' is set to \"false\" this element is ignored.")
private String wsdlPathRewriteRule;
@AttributeDocumentation("The non-secure port that will be used for rewriting the SOAP address. If absent the port will be identified by querying the list of installed connectors.")
private Integer wsdlPort;
@AttributeDocumentation("The secure port that will be used for rewriting the SOAP address. If absent the port will be identified by querying the list of installed connectors.")
private Integer wsdlSecurePort;
@AttributeDocumentation("The URI scheme to use for rewriting . Valid values are 'http' and 'https'. This configuration overrides scheme computed by processing the endpoint (even if a transport guarantee is specified). The provided values for 'wsdl-port' and 'wsdl-secure-port' (or their default values) are used depending on specified scheme.")
private WsdlUriScheme wsdlUriScheme;
public Webservices() {
super();
this.key = "webservices";
this.pcs = new PropertyChangeSupport(this);
}
public String getKey() {
return this.key;
}
/**
* Adds a property change listener
*/
public void addPropertyChangeListener(PropertyChangeListener listener) {
if (null == this.pcs)
this.pcs = new PropertyChangeSupport(this);
this.pcs.addPropertyChangeListener(listener);
}
/**
* Removes a property change listener
*/
public void removePropertyChangeListener(
java.beans.PropertyChangeListener listener) {
if (this.pcs != null)
this.pcs.removePropertyChangeListener(listener);
}
public WebservicesResources subresources() {
return this.subresources;
}
/**
* Add all ClientConfig objects to this subresource
*
* @return this
* @param value
* List of ClientConfig objects.
*/
@SuppressWarnings("unchecked")
public T clientConfigs(java.util.List value) {
this.subresources.clientConfigs = value;
return (T) this;
}
/**
* Add the ClientConfig object to the list of subresources
*
* @param value
* The ClientConfig to add
* @return this
*/
@SuppressWarnings("unchecked")
public T clientConfig(ClientConfig value) {
this.subresources.clientConfigs.add(value);
return (T) this;
}
/**
* Create and configure a ClientConfig object to the list of subresources
*
* @param key
* The key for the ClientConfig resource
* @param config
* The ClientConfigConsumer to use
* @return this
*/
@SuppressWarnings("unchecked")
public T clientConfig(java.lang.String childKey,
ClientConfigConsumer consumer) {
ClientConfig extends ClientConfig> child = new ClientConfig<>(
childKey);
if (consumer != null) {
consumer.accept(child);
}
clientConfig(child);
return (T) this;
}
/**
* Create and configure a ClientConfig object to the list of subresources
*
* @param key
* The key for the ClientConfig resource
* @return this
*/
@SuppressWarnings("unchecked")
public T clientConfig(java.lang.String childKey) {
clientConfig(childKey, null);
return (T) this;
}
/**
* Install a supplied ClientConfig object to the list of subresources
*/
@SuppressWarnings("unchecked")
public T clientConfig(ClientConfigSupplier supplier) {
clientConfig(supplier.get());
return (T) this;
}
/**
* Add all EndpointConfig objects to this subresource
*
* @return this
* @param value
* List of EndpointConfig objects.
*/
@SuppressWarnings("unchecked")
public T endpointConfigs(java.util.List value) {
this.subresources.endpointConfigs = value;
return (T) this;
}
/**
* Add the EndpointConfig object to the list of subresources
*
* @param value
* The EndpointConfig to add
* @return this
*/
@SuppressWarnings("unchecked")
public T endpointConfig(EndpointConfig value) {
this.subresources.endpointConfigs.add(value);
return (T) this;
}
/**
* Create and configure a EndpointConfig object to the list of subresources
*
* @param key
* The key for the EndpointConfig resource
* @param config
* The EndpointConfigConsumer to use
* @return this
*/
@SuppressWarnings("unchecked")
public T endpointConfig(java.lang.String childKey,
EndpointConfigConsumer consumer) {
EndpointConfig extends EndpointConfig> child = new EndpointConfig<>(
childKey);
if (consumer != null) {
consumer.accept(child);
}
endpointConfig(child);
return (T) this;
}
/**
* Create and configure a EndpointConfig object to the list of subresources
*
* @param key
* The key for the EndpointConfig resource
* @return this
*/
@SuppressWarnings("unchecked")
public T endpointConfig(java.lang.String childKey) {
endpointConfig(childKey, null);
return (T) this;
}
/**
* Install a supplied EndpointConfig object to the list of subresources
*/
@SuppressWarnings("unchecked")
public T endpointConfig(EndpointConfigSupplier supplier) {
endpointConfig(supplier.get());
return (T) this;
}
/**
* Child mutators for Webservices
*/
public static class WebservicesResources {
/**
* Webservice client configuration
*/
@ResourceDocumentation("Webservice client configuration")
@SubresourceInfo("clientConfig")
private List clientConfigs = new java.util.ArrayList<>();
/**
* Webservice endpoint configuration
*/
@ResourceDocumentation("Webservice endpoint configuration")
@SubresourceInfo("endpointConfig")
private List endpointConfigs = new java.util.ArrayList<>();
/**
* Get the list of ClientConfig resources
*
* @return the list of resources
*/
@Subresource
public List clientConfigs() {
return this.clientConfigs;
}
public ClientConfig clientConfig(java.lang.String key) {
return this.clientConfigs.stream()
.filter(e -> e.getKey().equals(key)).findFirst()
.orElse(null);
}
/**
* Get the list of EndpointConfig resources
*
* @return the list of resources
*/
@Subresource
public List endpointConfigs() {
return this.endpointConfigs;
}
public EndpointConfig endpointConfig(java.lang.String key) {
return this.endpointConfigs.stream()
.filter(e -> e.getKey().equals(key)).findFirst()
.orElse(null);
}
}
public static enum WsdlUriScheme {
HTTP("http"), HTTPS("https");
private final String allowedValue;
/**
* Returns the allowed value for the management model.
*
* @return the allowed model value
*/
public String getAllowedValue() {
return allowedValue;
}
WsdlUriScheme(java.lang.String allowedValue) {
this.allowedValue = allowedValue;
}
@Override
public String toString() {
return allowedValue;
}
}
/**
* Whether the soap address can be modified.
*/
@ModelNodeBinding(detypedName = "modify-wsdl-address")
public Boolean modifyWsdlAddress() {
return this.modifyWsdlAddress;
}
/**
* Whether the soap address can be modified.
*/
@SuppressWarnings("unchecked")
public T modifyWsdlAddress(java.lang.Boolean value) {
Object oldValue = this.modifyWsdlAddress;
this.modifyWsdlAddress = value;
if (this.pcs != null)
this.pcs.firePropertyChange("modifyWsdlAddress", oldValue, value);
return (T) this;
}
/**
* Whether statistics are to be gathered for endpoints, default value is
* 'false'.
*/
@ModelNodeBinding(detypedName = "statistics-enabled")
public Boolean statisticsEnabled() {
return this.statisticsEnabled;
}
/**
* Whether statistics are to be gathered for endpoints, default value is
* 'false'.
*/
@SuppressWarnings("unchecked")
public T statisticsEnabled(java.lang.Boolean value) {
Object oldValue = this.statisticsEnabled;
this.statisticsEnabled = value;
if (this.pcs != null)
this.pcs.firePropertyChange("statisticsEnabled", oldValue, value);
return (T) this;
}
/**
* The WSDL, that is a required deployment artifact for an endpoint, has a
* element which points to the location of the endpoint.
* JBoss supports rewriting of that SOAP address. If the content of
* is a valid URL, JBossWS will not rewrite it unless
* 'modify-wsdl-address' is true. If the content of is not a
* valid URL, JBossWS will rewrite it using the attribute values given
* below. If 'wsdl-host' is set to 'jbossws.undefined.host', JBossWS uses
* requesters host when rewriting the
*/
@ModelNodeBinding(detypedName = "wsdl-host")
public String wsdlHost() {
return this.wsdlHost;
}
/**
* The WSDL, that is a required deployment artifact for an endpoint, has a
* element which points to the location of the endpoint.
* JBoss supports rewriting of that SOAP address. If the content of
* is a valid URL, JBossWS will not rewrite it unless
* 'modify-wsdl-address' is true. If the content of is not a
* valid URL, JBossWS will rewrite it using the attribute values given
* below. If 'wsdl-host' is set to 'jbossws.undefined.host', JBossWS uses
* requesters host when rewriting the
*/
@SuppressWarnings("unchecked")
public T wsdlHost(java.lang.String value) {
Object oldValue = this.wsdlHost;
this.wsdlHost = value;
if (this.pcs != null)
this.pcs.firePropertyChange("wsdlHost", oldValue, value);
return (T) this;
}
/**
* This string defines a SED substitution command (e.g.,
* 's/regexp/replacement/g') that JBossWS executes against the path
* component of each URL published from the server. When
* wsdl-path-rewrite-rule is not defined, JBossWS retains the original path
* component of each URL. When 'modify-wsdl-address' is set
* to "false" this element is ignored.
*/
@ModelNodeBinding(detypedName = "wsdl-path-rewrite-rule")
public String wsdlPathRewriteRule() {
return this.wsdlPathRewriteRule;
}
/**
* This string defines a SED substitution command (e.g.,
* 's/regexp/replacement/g') that JBossWS executes against the path
* component of each URL published from the server. When
* wsdl-path-rewrite-rule is not defined, JBossWS retains the original path
* component of each URL. When 'modify-wsdl-address' is set
* to "false" this element is ignored.
*/
@SuppressWarnings("unchecked")
public T wsdlPathRewriteRule(java.lang.String value) {
Object oldValue = this.wsdlPathRewriteRule;
this.wsdlPathRewriteRule = value;
if (this.pcs != null)
this.pcs.firePropertyChange("wsdlPathRewriteRule", oldValue, value);
return (T) this;
}
/**
* The non-secure port that will be used for rewriting the SOAP address. If
* absent the port will be identified by querying the list of installed
* connectors.
*/
@ModelNodeBinding(detypedName = "wsdl-port")
public Integer wsdlPort() {
return this.wsdlPort;
}
/**
* The non-secure port that will be used for rewriting the SOAP address. If
* absent the port will be identified by querying the list of installed
* connectors.
*/
@SuppressWarnings("unchecked")
public T wsdlPort(java.lang.Integer value) {
Object oldValue = this.wsdlPort;
this.wsdlPort = value;
if (this.pcs != null)
this.pcs.firePropertyChange("wsdlPort", oldValue, value);
return (T) this;
}
/**
* The secure port that will be used for rewriting the SOAP address. If
* absent the port will be identified by querying the list of installed
* connectors.
*/
@ModelNodeBinding(detypedName = "wsdl-secure-port")
public Integer wsdlSecurePort() {
return this.wsdlSecurePort;
}
/**
* The secure port that will be used for rewriting the SOAP address. If
* absent the port will be identified by querying the list of installed
* connectors.
*/
@SuppressWarnings("unchecked")
public T wsdlSecurePort(java.lang.Integer value) {
Object oldValue = this.wsdlSecurePort;
this.wsdlSecurePort = value;
if (this.pcs != null)
this.pcs.firePropertyChange("wsdlSecurePort", oldValue, value);
return (T) this;
}
/**
* The URI scheme to use for rewriting . Valid values are
* 'http' and 'https'. This configuration overrides scheme computed by
* processing the endpoint (even if a transport guarantee is specified). The
* provided values for 'wsdl-port' and 'wsdl-secure-port' (or their default
* values) are used depending on specified scheme.
*/
@ModelNodeBinding(detypedName = "wsdl-uri-scheme")
public WsdlUriScheme wsdlUriScheme() {
return this.wsdlUriScheme;
}
/**
* The URI scheme to use for rewriting . Valid values are
* 'http' and 'https'. This configuration overrides scheme computed by
* processing the endpoint (even if a transport guarantee is specified). The
* provided values for 'wsdl-port' and 'wsdl-secure-port' (or their default
* values) are used depending on specified scheme.
*/
@SuppressWarnings("unchecked")
public T wsdlUriScheme(WsdlUriScheme value) {
Object oldValue = this.wsdlUriScheme;
this.wsdlUriScheme = value;
if (this.pcs != null)
this.pcs.firePropertyChange("wsdlUriScheme", oldValue, value);
return (T) this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy