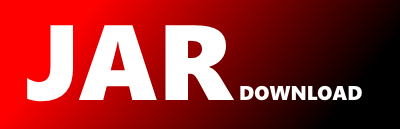
org.wildfly.swarm.config.elytron.AuthenticationConfiguration Maven / Gradle / Ivy
package org.wildfly.swarm.config.elytron;
import org.wildfly.swarm.config.runtime.AttributeDocumentation;
import org.wildfly.swarm.config.runtime.ResourceDocumentation;
import org.wildfly.swarm.config.runtime.SingletonResource;
import org.wildfly.swarm.config.runtime.Address;
import org.wildfly.swarm.config.runtime.ResourceType;
import java.beans.PropertyChangeSupport;
import java.beans.PropertyChangeListener;
import org.wildfly.swarm.config.runtime.ModelNodeBinding;
import java.util.Map;
import java.util.Arrays;
/**
* An individual authentication configuration definition.
*/
@Address("/subsystem=elytron/authentication-configuration=*")
@ResourceType("authentication-configuration")
public class AuthenticationConfiguration>
implements
org.wildfly.swarm.config.runtime.Keyed {
private String key;
private PropertyChangeSupport pcs;
@AttributeDocumentation("Enables anonymous authentication.")
private Boolean anonymous;
@AttributeDocumentation("The authentication name to use.")
private String authenticationName;
@AttributeDocumentation("The authorization name to use.")
private String authorizationName;
@AttributeDocumentation("The reference to credential stored in CredentialStore under defined alias or clear text password.")
private Map credentialReference;
@AttributeDocumentation("A previously defined authentication configuration to extend.")
private String attributeExtends;
@AttributeDocumentation("The type of security identity forwarding to use. A mode of 'authentication' forwarding forwards the principal and credential. A mode of 'authorization' forwards the authorization id, allowing for a different authentication identity.")
private ForwardingMode forwardingMode;
@AttributeDocumentation("The host to use.")
private String host;
@AttributeDocumentation("Reference to a kerberos security factory used to obtain a GSS kerberos credential")
private String kerberosSecurityFactory;
@AttributeDocumentation("Configuration properties for the SASL authentication mechanism.")
private Map mechanismProperties;
@AttributeDocumentation("The port to use.")
private Integer port;
@AttributeDocumentation("The protocol to use.")
private String protocol;
@AttributeDocumentation("The realm to use.")
private String realm;
@AttributeDocumentation("The SASL mechanism selector string.")
private String saslMechanismSelector;
@AttributeDocumentation("Reference to a security domain to obtain a forwarded identity.")
private String securityDomain;
public AuthenticationConfiguration(java.lang.String key) {
super();
this.key = key;
}
public String getKey() {
return this.key;
}
/**
* Adds a property change listener
*/
public void addPropertyChangeListener(PropertyChangeListener listener) {
if (null == this.pcs)
this.pcs = new PropertyChangeSupport(this);
this.pcs.addPropertyChangeListener(listener);
}
/**
* Removes a property change listener
*/
public void removePropertyChangeListener(
java.beans.PropertyChangeListener listener) {
if (this.pcs != null)
this.pcs.removePropertyChangeListener(listener);
}
public static enum ForwardingMode {
AUTHENTICATION("authentication"), AUTHORIZATION("authorization");
private final String allowedValue;
/**
* Returns the allowed value for the management model.
*
* @return the allowed model value
*/
public String getAllowedValue() {
return allowedValue;
}
ForwardingMode(java.lang.String allowedValue) {
this.allowedValue = allowedValue;
}
@Override
public String toString() {
return allowedValue;
}
}
/**
* Enables anonymous authentication.
*/
@ModelNodeBinding(detypedName = "anonymous")
public Boolean anonymous() {
return this.anonymous;
}
/**
* Enables anonymous authentication.
*/
@SuppressWarnings("unchecked")
public T anonymous(java.lang.Boolean value) {
Object oldValue = this.anonymous;
this.anonymous = value;
if (this.pcs != null)
this.pcs.firePropertyChange("anonymous", oldValue, value);
return (T) this;
}
/**
* The authentication name to use.
*/
@ModelNodeBinding(detypedName = "authentication-name")
public String authenticationName() {
return this.authenticationName;
}
/**
* The authentication name to use.
*/
@SuppressWarnings("unchecked")
public T authenticationName(java.lang.String value) {
Object oldValue = this.authenticationName;
this.authenticationName = value;
if (this.pcs != null)
this.pcs.firePropertyChange("authenticationName", oldValue, value);
return (T) this;
}
/**
* The authorization name to use.
*/
@ModelNodeBinding(detypedName = "authorization-name")
public String authorizationName() {
return this.authorizationName;
}
/**
* The authorization name to use.
*/
@SuppressWarnings("unchecked")
public T authorizationName(java.lang.String value) {
Object oldValue = this.authorizationName;
this.authorizationName = value;
if (this.pcs != null)
this.pcs.firePropertyChange("authorizationName", oldValue, value);
return (T) this;
}
/**
* The reference to credential stored in CredentialStore under defined alias
* or clear text password.
*/
@ModelNodeBinding(detypedName = "credential-reference")
public Map credentialReference() {
return this.credentialReference;
}
/**
* The reference to credential stored in CredentialStore under defined alias
* or clear text password.
*/
@SuppressWarnings("unchecked")
public T credentialReference(java.util.Map value) {
Object oldValue = this.credentialReference;
this.credentialReference = value;
if (this.pcs != null)
this.pcs.firePropertyChange("credentialReference", oldValue, value);
return (T) this;
}
/**
* The reference to credential stored in CredentialStore under defined alias
* or clear text password.
*/
@SuppressWarnings("unchecked")
public T credentialReference(java.lang.String key, java.lang.Object value) {
if (this.credentialReference == null) {
this.credentialReference = new java.util.HashMap<>();
}
this.credentialReference.put(key, value);
return (T) this;
}
/**
* A previously defined authentication configuration to extend.
*/
@ModelNodeBinding(detypedName = "extends")
public String attributeExtends() {
return this.attributeExtends;
}
/**
* A previously defined authentication configuration to extend.
*/
@SuppressWarnings("unchecked")
public T attributeExtends(java.lang.String value) {
Object oldValue = this.attributeExtends;
this.attributeExtends = value;
if (this.pcs != null)
this.pcs.firePropertyChange("attributeExtends", oldValue, value);
return (T) this;
}
/**
* The type of security identity forwarding to use. A mode of
* 'authentication' forwarding forwards the principal and credential. A mode
* of 'authorization' forwards the authorization id, allowing for a
* different authentication identity.
*/
@ModelNodeBinding(detypedName = "forwarding-mode")
public ForwardingMode forwardingMode() {
return this.forwardingMode;
}
/**
* The type of security identity forwarding to use. A mode of
* 'authentication' forwarding forwards the principal and credential. A mode
* of 'authorization' forwards the authorization id, allowing for a
* different authentication identity.
*/
@SuppressWarnings("unchecked")
public T forwardingMode(ForwardingMode value) {
Object oldValue = this.forwardingMode;
this.forwardingMode = value;
if (this.pcs != null)
this.pcs.firePropertyChange("forwardingMode", oldValue, value);
return (T) this;
}
/**
* The host to use.
*/
@ModelNodeBinding(detypedName = "host")
public String host() {
return this.host;
}
/**
* The host to use.
*/
@SuppressWarnings("unchecked")
public T host(java.lang.String value) {
Object oldValue = this.host;
this.host = value;
if (this.pcs != null)
this.pcs.firePropertyChange("host", oldValue, value);
return (T) this;
}
/**
* Reference to a kerberos security factory used to obtain a GSS kerberos
* credential
*/
@ModelNodeBinding(detypedName = "kerberos-security-factory")
public String kerberosSecurityFactory() {
return this.kerberosSecurityFactory;
}
/**
* Reference to a kerberos security factory used to obtain a GSS kerberos
* credential
*/
@SuppressWarnings("unchecked")
public T kerberosSecurityFactory(java.lang.String value) {
Object oldValue = this.kerberosSecurityFactory;
this.kerberosSecurityFactory = value;
if (this.pcs != null)
this.pcs.firePropertyChange("kerberosSecurityFactory", oldValue,
value);
return (T) this;
}
/**
* Configuration properties for the SASL authentication mechanism.
*/
@ModelNodeBinding(detypedName = "mechanism-properties")
public Map mechanismProperties() {
return this.mechanismProperties;
}
/**
* Configuration properties for the SASL authentication mechanism.
*/
@SuppressWarnings("unchecked")
public T mechanismProperties(java.util.Map value) {
Object oldValue = this.mechanismProperties;
this.mechanismProperties = value;
if (this.pcs != null)
this.pcs.firePropertyChange("mechanismProperties", oldValue, value);
return (T) this;
}
/**
* Configuration properties for the SASL authentication mechanism.
*/
@SuppressWarnings("unchecked")
public T mechanismProperty(java.lang.String key, java.lang.Object value) {
if (this.mechanismProperties == null) {
this.mechanismProperties = new java.util.HashMap<>();
}
this.mechanismProperties.put(key, value);
return (T) this;
}
/**
* The port to use.
*/
@ModelNodeBinding(detypedName = "port")
public Integer port() {
return this.port;
}
/**
* The port to use.
*/
@SuppressWarnings("unchecked")
public T port(java.lang.Integer value) {
Object oldValue = this.port;
this.port = value;
if (this.pcs != null)
this.pcs.firePropertyChange("port", oldValue, value);
return (T) this;
}
/**
* The protocol to use.
*/
@ModelNodeBinding(detypedName = "protocol")
public String protocol() {
return this.protocol;
}
/**
* The protocol to use.
*/
@SuppressWarnings("unchecked")
public T protocol(java.lang.String value) {
Object oldValue = this.protocol;
this.protocol = value;
if (this.pcs != null)
this.pcs.firePropertyChange("protocol", oldValue, value);
return (T) this;
}
/**
* The realm to use.
*/
@ModelNodeBinding(detypedName = "realm")
public String realm() {
return this.realm;
}
/**
* The realm to use.
*/
@SuppressWarnings("unchecked")
public T realm(java.lang.String value) {
Object oldValue = this.realm;
this.realm = value;
if (this.pcs != null)
this.pcs.firePropertyChange("realm", oldValue, value);
return (T) this;
}
/**
* The SASL mechanism selector string.
*/
@ModelNodeBinding(detypedName = "sasl-mechanism-selector")
public String saslMechanismSelector() {
return this.saslMechanismSelector;
}
/**
* The SASL mechanism selector string.
*/
@SuppressWarnings("unchecked")
public T saslMechanismSelector(java.lang.String value) {
Object oldValue = this.saslMechanismSelector;
this.saslMechanismSelector = value;
if (this.pcs != null)
this.pcs.firePropertyChange("saslMechanismSelector", oldValue,
value);
return (T) this;
}
/**
* Reference to a security domain to obtain a forwarded identity.
*/
@ModelNodeBinding(detypedName = "security-domain")
public String securityDomain() {
return this.securityDomain;
}
/**
* Reference to a security domain to obtain a forwarded identity.
*/
@SuppressWarnings("unchecked")
public T securityDomain(java.lang.String value) {
Object oldValue = this.securityDomain;
this.securityDomain = value;
if (this.pcs != null)
this.pcs.firePropertyChange("securityDomain", oldValue, value);
return (T) this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy