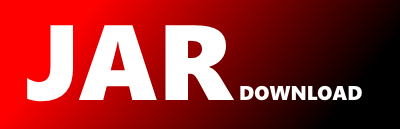
org.wildfly.swarm.config.management.AuditAccess Maven / Gradle / Ivy
package org.wildfly.swarm.config.management;
import org.wildfly.swarm.config.runtime.AttributeDocumentation;
import org.wildfly.swarm.config.runtime.ResourceDocumentation;
import org.wildfly.swarm.config.runtime.SingletonResource;
import org.wildfly.swarm.config.runtime.Address;
import org.wildfly.swarm.config.runtime.ResourceType;
import org.wildfly.swarm.config.runtime.Implicit;
import java.beans.PropertyChangeSupport;
import java.beans.PropertyChangeListener;
import java.util.List;
import org.wildfly.swarm.config.runtime.Subresource;
import org.wildfly.swarm.config.management.access.PeriodicRotatingFileHandlerConsumer;
import org.wildfly.swarm.config.management.access.PeriodicRotatingFileHandlerSupplier;
import org.wildfly.swarm.config.management.access.PeriodicRotatingFileHandler;
import org.wildfly.swarm.config.runtime.SubresourceInfo;
import org.wildfly.swarm.config.management.access.InMemoryHandlerConsumer;
import org.wildfly.swarm.config.management.access.InMemoryHandlerSupplier;
import org.wildfly.swarm.config.management.access.InMemoryHandler;
import org.wildfly.swarm.config.management.access.JsonFormatterConsumer;
import org.wildfly.swarm.config.management.access.JsonFormatterSupplier;
import org.wildfly.swarm.config.management.access.JsonFormatter;
import org.wildfly.swarm.config.management.access.FileHandlerConsumer;
import org.wildfly.swarm.config.management.access.FileHandlerSupplier;
import org.wildfly.swarm.config.management.access.FileHandler;
import org.wildfly.swarm.config.management.access.SizeRotatingFileHandlerConsumer;
import org.wildfly.swarm.config.management.access.SizeRotatingFileHandlerSupplier;
import org.wildfly.swarm.config.management.access.SizeRotatingFileHandler;
import org.wildfly.swarm.config.management.access.SyslogHandlerConsumer;
import org.wildfly.swarm.config.management.access.SyslogHandlerSupplier;
import org.wildfly.swarm.config.management.access.SyslogHandler;
import org.wildfly.swarm.config.management.access.AuditLogLogger;
import org.wildfly.swarm.config.management.access.AuditLogLoggerConsumer;
import org.wildfly.swarm.config.management.access.AuditLogLoggerSupplier;
import org.wildfly.swarm.config.runtime.ModelNodeBinding;
/**
* The management audit logging top-level resource.
*/
@Address("/core-service=management/access=audit")
@ResourceType("access")
@Implicit
public class AuditAccess>
implements
org.wildfly.swarm.config.runtime.Keyed {
private String key;
private PropertyChangeSupport pcs;
private AuditAccessResources subresources = new AuditAccessResources();
public AuditAccess() {
super();
this.key = "audit";
this.pcs = new PropertyChangeSupport(this);
}
public String getKey() {
return this.key;
}
/**
* Adds a property change listener
*/
public void addPropertyChangeListener(PropertyChangeListener listener) {
if (null == this.pcs)
this.pcs = new PropertyChangeSupport(this);
this.pcs.addPropertyChangeListener(listener);
}
/**
* Removes a property change listener
*/
public void removePropertyChangeListener(
java.beans.PropertyChangeListener listener) {
if (this.pcs != null)
this.pcs.removePropertyChangeListener(listener);
}
public AuditAccessResources subresources() {
return this.subresources;
}
/**
* Add all PeriodicRotatingFileHandler objects to this subresource
*
* @return this
* @param value
* List of PeriodicRotatingFileHandler objects.
*/
@SuppressWarnings("unchecked")
public T periodicRotatingFileHandlers(
java.util.List value) {
this.subresources.periodicRotatingFileHandlers = value;
return (T) this;
}
/**
* Add the PeriodicRotatingFileHandler object to the list of subresources
*
* @param value
* The PeriodicRotatingFileHandler to add
* @return this
*/
@SuppressWarnings("unchecked")
public T periodicRotatingFileHandler(PeriodicRotatingFileHandler value) {
this.subresources.periodicRotatingFileHandlers.add(value);
return (T) this;
}
/**
* Create and configure a PeriodicRotatingFileHandler object to the list of
* subresources
*
* @param key
* The key for the PeriodicRotatingFileHandler resource
* @param config
* The PeriodicRotatingFileHandlerConsumer to use
* @return this
*/
@SuppressWarnings("unchecked")
public T periodicRotatingFileHandler(java.lang.String childKey,
PeriodicRotatingFileHandlerConsumer consumer) {
PeriodicRotatingFileHandler extends PeriodicRotatingFileHandler> child = new PeriodicRotatingFileHandler<>(
childKey);
if (consumer != null) {
consumer.accept(child);
}
periodicRotatingFileHandler(child);
return (T) this;
}
/**
* Create and configure a PeriodicRotatingFileHandler object to the list of
* subresources
*
* @param key
* The key for the PeriodicRotatingFileHandler resource
* @return this
*/
@SuppressWarnings("unchecked")
public T periodicRotatingFileHandler(java.lang.String childKey) {
periodicRotatingFileHandler(childKey, null);
return (T) this;
}
/**
* Install a supplied PeriodicRotatingFileHandler object to the list of
* subresources
*/
@SuppressWarnings("unchecked")
public T periodicRotatingFileHandler(
PeriodicRotatingFileHandlerSupplier supplier) {
periodicRotatingFileHandler(supplier.get());
return (T) this;
}
/**
* Add all InMemoryHandler objects to this subresource
*
* @return this
* @param value
* List of InMemoryHandler objects.
*/
@SuppressWarnings("unchecked")
public T inMemoryHandlers(java.util.List value) {
this.subresources.inMemoryHandlers = value;
return (T) this;
}
/**
* Add the InMemoryHandler object to the list of subresources
*
* @param value
* The InMemoryHandler to add
* @return this
*/
@SuppressWarnings("unchecked")
public T inMemoryHandler(InMemoryHandler value) {
this.subresources.inMemoryHandlers.add(value);
return (T) this;
}
/**
* Create and configure a InMemoryHandler object to the list of subresources
*
* @param key
* The key for the InMemoryHandler resource
* @param config
* The InMemoryHandlerConsumer to use
* @return this
*/
@SuppressWarnings("unchecked")
public T inMemoryHandler(java.lang.String childKey,
InMemoryHandlerConsumer consumer) {
InMemoryHandler extends InMemoryHandler> child = new InMemoryHandler<>(
childKey);
if (consumer != null) {
consumer.accept(child);
}
inMemoryHandler(child);
return (T) this;
}
/**
* Create and configure a InMemoryHandler object to the list of subresources
*
* @param key
* The key for the InMemoryHandler resource
* @return this
*/
@SuppressWarnings("unchecked")
public T inMemoryHandler(java.lang.String childKey) {
inMemoryHandler(childKey, null);
return (T) this;
}
/**
* Install a supplied InMemoryHandler object to the list of subresources
*/
@SuppressWarnings("unchecked")
public T inMemoryHandler(InMemoryHandlerSupplier supplier) {
inMemoryHandler(supplier.get());
return (T) this;
}
/**
* Add all JsonFormatter objects to this subresource
*
* @return this
* @param value
* List of JsonFormatter objects.
*/
@SuppressWarnings("unchecked")
public T jsonFormatters(java.util.List value) {
this.subresources.jsonFormatters = value;
return (T) this;
}
/**
* Add the JsonFormatter object to the list of subresources
*
* @param value
* The JsonFormatter to add
* @return this
*/
@SuppressWarnings("unchecked")
public T jsonFormatter(JsonFormatter value) {
this.subresources.jsonFormatters.add(value);
return (T) this;
}
/**
* Create and configure a JsonFormatter object to the list of subresources
*
* @param key
* The key for the JsonFormatter resource
* @param config
* The JsonFormatterConsumer to use
* @return this
*/
@SuppressWarnings("unchecked")
public T jsonFormatter(java.lang.String childKey,
JsonFormatterConsumer consumer) {
JsonFormatter extends JsonFormatter> child = new JsonFormatter<>(
childKey);
if (consumer != null) {
consumer.accept(child);
}
jsonFormatter(child);
return (T) this;
}
/**
* Create and configure a JsonFormatter object to the list of subresources
*
* @param key
* The key for the JsonFormatter resource
* @return this
*/
@SuppressWarnings("unchecked")
public T jsonFormatter(java.lang.String childKey) {
jsonFormatter(childKey, null);
return (T) this;
}
/**
* Install a supplied JsonFormatter object to the list of subresources
*/
@SuppressWarnings("unchecked")
public T jsonFormatter(JsonFormatterSupplier supplier) {
jsonFormatter(supplier.get());
return (T) this;
}
/**
* Add all FileHandler objects to this subresource
*
* @return this
* @param value
* List of FileHandler objects.
*/
@SuppressWarnings("unchecked")
public T fileHandlers(java.util.List value) {
this.subresources.fileHandlers = value;
return (T) this;
}
/**
* Add the FileHandler object to the list of subresources
*
* @param value
* The FileHandler to add
* @return this
*/
@SuppressWarnings("unchecked")
public T fileHandler(FileHandler value) {
this.subresources.fileHandlers.add(value);
return (T) this;
}
/**
* Create and configure a FileHandler object to the list of subresources
*
* @param key
* The key for the FileHandler resource
* @param config
* The FileHandlerConsumer to use
* @return this
*/
@SuppressWarnings("unchecked")
public T fileHandler(java.lang.String childKey, FileHandlerConsumer consumer) {
FileHandler extends FileHandler> child = new FileHandler<>(childKey);
if (consumer != null) {
consumer.accept(child);
}
fileHandler(child);
return (T) this;
}
/**
* Create and configure a FileHandler object to the list of subresources
*
* @param key
* The key for the FileHandler resource
* @return this
*/
@SuppressWarnings("unchecked")
public T fileHandler(java.lang.String childKey) {
fileHandler(childKey, null);
return (T) this;
}
/**
* Install a supplied FileHandler object to the list of subresources
*/
@SuppressWarnings("unchecked")
public T fileHandler(FileHandlerSupplier supplier) {
fileHandler(supplier.get());
return (T) this;
}
/**
* Add all SizeRotatingFileHandler objects to this subresource
*
* @return this
* @param value
* List of SizeRotatingFileHandler objects.
*/
@SuppressWarnings("unchecked")
public T sizeRotatingFileHandlers(
java.util.List value) {
this.subresources.sizeRotatingFileHandlers = value;
return (T) this;
}
/**
* Add the SizeRotatingFileHandler object to the list of subresources
*
* @param value
* The SizeRotatingFileHandler to add
* @return this
*/
@SuppressWarnings("unchecked")
public T sizeRotatingFileHandler(SizeRotatingFileHandler value) {
this.subresources.sizeRotatingFileHandlers.add(value);
return (T) this;
}
/**
* Create and configure a SizeRotatingFileHandler object to the list of
* subresources
*
* @param key
* The key for the SizeRotatingFileHandler resource
* @param config
* The SizeRotatingFileHandlerConsumer to use
* @return this
*/
@SuppressWarnings("unchecked")
public T sizeRotatingFileHandler(java.lang.String childKey,
SizeRotatingFileHandlerConsumer consumer) {
SizeRotatingFileHandler extends SizeRotatingFileHandler> child = new SizeRotatingFileHandler<>(
childKey);
if (consumer != null) {
consumer.accept(child);
}
sizeRotatingFileHandler(child);
return (T) this;
}
/**
* Create and configure a SizeRotatingFileHandler object to the list of
* subresources
*
* @param key
* The key for the SizeRotatingFileHandler resource
* @return this
*/
@SuppressWarnings("unchecked")
public T sizeRotatingFileHandler(java.lang.String childKey) {
sizeRotatingFileHandler(childKey, null);
return (T) this;
}
/**
* Install a supplied SizeRotatingFileHandler object to the list of
* subresources
*/
@SuppressWarnings("unchecked")
public T sizeRotatingFileHandler(SizeRotatingFileHandlerSupplier supplier) {
sizeRotatingFileHandler(supplier.get());
return (T) this;
}
/**
* Add all SyslogHandler objects to this subresource
*
* @return this
* @param value
* List of SyslogHandler objects.
*/
@SuppressWarnings("unchecked")
public T syslogHandlers(java.util.List value) {
this.subresources.syslogHandlers = value;
return (T) this;
}
/**
* Add the SyslogHandler object to the list of subresources
*
* @param value
* The SyslogHandler to add
* @return this
*/
@SuppressWarnings("unchecked")
public T syslogHandler(SyslogHandler value) {
this.subresources.syslogHandlers.add(value);
return (T) this;
}
/**
* Create and configure a SyslogHandler object to the list of subresources
*
* @param key
* The key for the SyslogHandler resource
* @param config
* The SyslogHandlerConsumer to use
* @return this
*/
@SuppressWarnings("unchecked")
public T syslogHandler(java.lang.String childKey,
SyslogHandlerConsumer consumer) {
SyslogHandler extends SyslogHandler> child = new SyslogHandler<>(
childKey);
if (consumer != null) {
consumer.accept(child);
}
syslogHandler(child);
return (T) this;
}
/**
* Create and configure a SyslogHandler object to the list of subresources
*
* @param key
* The key for the SyslogHandler resource
* @return this
*/
@SuppressWarnings("unchecked")
public T syslogHandler(java.lang.String childKey) {
syslogHandler(childKey, null);
return (T) this;
}
/**
* Install a supplied SyslogHandler object to the list of subresources
*/
@SuppressWarnings("unchecked")
public T syslogHandler(SyslogHandlerSupplier supplier) {
syslogHandler(supplier.get());
return (T) this;
}
/**
* The management audit logging top-level resource.
*/
@SuppressWarnings("unchecked")
public T auditLogLogger(AuditLogLogger value) {
this.subresources.auditLogLogger = value;
return (T) this;
}
/**
* The management audit logging top-level resource.
*/
@SuppressWarnings("unchecked")
public T auditLogLogger(AuditLogLoggerConsumer consumer) {
AuditLogLogger extends AuditLogLogger> child = new AuditLogLogger<>();
if (consumer != null) {
consumer.accept(child);
}
this.subresources.auditLogLogger = child;
return (T) this;
}
/**
* The management audit logging top-level resource.
*/
@SuppressWarnings("unchecked")
public T auditLogLogger() {
AuditLogLogger extends AuditLogLogger> child = new AuditLogLogger<>();
this.subresources.auditLogLogger = child;
return (T) this;
}
/**
* The management audit logging top-level resource.
*/
@SuppressWarnings("unchecked")
public T auditLogLogger(AuditLogLoggerSupplier supplier) {
this.subresources.auditLogLogger = supplier.get();
return (T) this;
}
/**
* Child mutators for AuditAccess
*/
public static class AuditAccessResources {
/**
* A management audit log handler which writes to a file, rotating the
* log after a time period derived from the given suffix string, which
* should be in a format understood by java.text.SimpleDateFormat.
*/
@ResourceDocumentation("A management audit log handler which writes to a file, rotating the log after a time period derived from the given suffix string, which should be in a format understood by java.text.SimpleDateFormat.")
@SubresourceInfo("periodicRotatingFileHandler")
private List periodicRotatingFileHandlers = new java.util.ArrayList<>();
/**
* A in-memory handler for use with the management audit logging
* service.
*/
@ResourceDocumentation("A in-memory handler for use with the management audit logging service.")
@SubresourceInfo("inMemoryHandler")
private List inMemoryHandlers = new java.util.ArrayList<>();
/**
* A json formatter for audit log messages.
*/
@ResourceDocumentation("A json formatter for audit log messages.")
@SubresourceInfo("jsonFormatter")
private List jsonFormatters = new java.util.ArrayList<>();
/**
* A file handler for use with the management audit logging service.
*/
@ResourceDocumentation("A file handler for use with the management audit logging service.")
@SubresourceInfo("fileHandler")
private List fileHandlers = new java.util.ArrayList<>();
/**
* A management audit log handler which writes to a file, rotating the
* log after the size of the file grows beyond a certain point and
* keeping a fixed number of backups.
*/
@ResourceDocumentation("A management audit log handler which writes to a file, rotating the log after the size of the file grows beyond a certain point and keeping a fixed number of backups.")
@SubresourceInfo("sizeRotatingFileHandler")
private List sizeRotatingFileHandlers = new java.util.ArrayList<>();
/**
* A syslog handler for use with the management audit logging service.
*/
@ResourceDocumentation("A syslog handler for use with the management audit logging service.")
@SubresourceInfo("syslogHandler")
private List syslogHandlers = new java.util.ArrayList<>();
@SingletonResource
@ResourceDocumentation("The management audit logging top-level resource.")
private AuditLogLogger auditLogLogger;
/**
* Get the list of PeriodicRotatingFileHandler resources
*
* @return the list of resources
*/
@Subresource
public List periodicRotatingFileHandlers() {
return this.periodicRotatingFileHandlers;
}
public PeriodicRotatingFileHandler periodicRotatingFileHandler(
java.lang.String key) {
return this.periodicRotatingFileHandlers.stream()
.filter(e -> e.getKey().equals(key)).findFirst()
.orElse(null);
}
/**
* Get the list of InMemoryHandler resources
*
* @return the list of resources
*/
@Subresource
public List inMemoryHandlers() {
return this.inMemoryHandlers;
}
public InMemoryHandler inMemoryHandler(java.lang.String key) {
return this.inMemoryHandlers.stream()
.filter(e -> e.getKey().equals(key)).findFirst()
.orElse(null);
}
/**
* Get the list of JsonFormatter resources
*
* @return the list of resources
*/
@Subresource
public List jsonFormatters() {
return this.jsonFormatters;
}
public JsonFormatter jsonFormatter(java.lang.String key) {
return this.jsonFormatters.stream()
.filter(e -> e.getKey().equals(key)).findFirst()
.orElse(null);
}
/**
* Get the list of FileHandler resources
*
* @return the list of resources
*/
@Subresource
public List fileHandlers() {
return this.fileHandlers;
}
public FileHandler fileHandler(java.lang.String key) {
return this.fileHandlers.stream()
.filter(e -> e.getKey().equals(key)).findFirst()
.orElse(null);
}
/**
* Get the list of SizeRotatingFileHandler resources
*
* @return the list of resources
*/
@Subresource
public List sizeRotatingFileHandlers() {
return this.sizeRotatingFileHandlers;
}
public SizeRotatingFileHandler sizeRotatingFileHandler(
java.lang.String key) {
return this.sizeRotatingFileHandlers.stream()
.filter(e -> e.getKey().equals(key)).findFirst()
.orElse(null);
}
/**
* Get the list of SyslogHandler resources
*
* @return the list of resources
*/
@Subresource
public List syslogHandlers() {
return this.syslogHandlers;
}
public SyslogHandler syslogHandler(java.lang.String key) {
return this.syslogHandlers.stream()
.filter(e -> e.getKey().equals(key)).findFirst()
.orElse(null);
}
/**
* The management audit logging top-level resource.
*/
@Subresource
public AuditLogLogger auditLogLogger() {
return this.auditLogLogger;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy