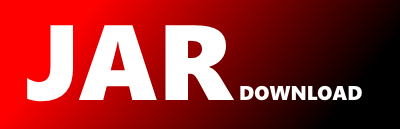
org.wildfly.swarm.config.undertow.ByteBufferPool Maven / Gradle / Ivy
package org.wildfly.swarm.config.undertow;
import org.wildfly.swarm.config.runtime.AttributeDocumentation;
import org.wildfly.swarm.config.runtime.ResourceDocumentation;
import org.wildfly.swarm.config.runtime.SingletonResource;
import org.wildfly.swarm.config.runtime.Address;
import org.wildfly.swarm.config.runtime.ResourceType;
import java.beans.PropertyChangeSupport;
import java.beans.PropertyChangeListener;
import org.wildfly.swarm.config.runtime.ModelNodeBinding;
/**
* A byte buffer pool used for IO operations, this provides the same
* capabilities as the buffer pool from the IO subsystem, so they can be used
* interchangeably and must have a unique name. This buffer pool allows for more
* precise configuration of the total amount of retained memory than the IO
* subsystem.
*/
@Address("/subsystem=undertow/byte-buffer-pool=*")
@ResourceType("byte-buffer-pool")
public class ByteBufferPool>
implements
org.wildfly.swarm.config.runtime.Keyed {
private String key;
private PropertyChangeSupport pcs;
@AttributeDocumentation("The size of the buffer")
private Integer bufferSize;
@AttributeDocumentation("If this is true the buffer pool will use direct buffers, this is recommended for best performance")
private Boolean direct;
@AttributeDocumentation("The percentage of buffers that will be allocated with a leak detector. This should only be larger than zero if you are experiencing issues with buffers leaking.")
private Integer leakDetectionPercent;
@AttributeDocumentation("The maximum amount of buffers to keep in the pool. If more buffers are required at runtime they will be allocated dynamically. Setting this to zero effectively disables pooling.")
private Integer maxPoolSize;
@AttributeDocumentation("The maximum number of buffers to cache on each thread. The actual number may be lower depending on the calculated usage pattern.")
private Integer threadLocalCacheSize;
public ByteBufferPool(java.lang.String key) {
super();
this.key = key;
}
public String getKey() {
return this.key;
}
/**
* Adds a property change listener
*/
public void addPropertyChangeListener(PropertyChangeListener listener) {
if (null == this.pcs)
this.pcs = new PropertyChangeSupport(this);
this.pcs.addPropertyChangeListener(listener);
}
/**
* Removes a property change listener
*/
public void removePropertyChangeListener(
java.beans.PropertyChangeListener listener) {
if (this.pcs != null)
this.pcs.removePropertyChangeListener(listener);
}
/**
* The size of the buffer
*/
@ModelNodeBinding(detypedName = "buffer-size")
public Integer bufferSize() {
return this.bufferSize;
}
/**
* The size of the buffer
*/
@SuppressWarnings("unchecked")
public T bufferSize(java.lang.Integer value) {
Object oldValue = this.bufferSize;
this.bufferSize = value;
if (this.pcs != null)
this.pcs.firePropertyChange("bufferSize", oldValue, value);
return (T) this;
}
/**
* If this is true the buffer pool will use direct buffers, this is
* recommended for best performance
*/
@ModelNodeBinding(detypedName = "direct")
public Boolean direct() {
return this.direct;
}
/**
* If this is true the buffer pool will use direct buffers, this is
* recommended for best performance
*/
@SuppressWarnings("unchecked")
public T direct(java.lang.Boolean value) {
Object oldValue = this.direct;
this.direct = value;
if (this.pcs != null)
this.pcs.firePropertyChange("direct", oldValue, value);
return (T) this;
}
/**
* The percentage of buffers that will be allocated with a leak detector.
* This should only be larger than zero if you are experiencing issues with
* buffers leaking.
*/
@ModelNodeBinding(detypedName = "leak-detection-percent")
public Integer leakDetectionPercent() {
return this.leakDetectionPercent;
}
/**
* The percentage of buffers that will be allocated with a leak detector.
* This should only be larger than zero if you are experiencing issues with
* buffers leaking.
*/
@SuppressWarnings("unchecked")
public T leakDetectionPercent(java.lang.Integer value) {
Object oldValue = this.leakDetectionPercent;
this.leakDetectionPercent = value;
if (this.pcs != null)
this.pcs.firePropertyChange("leakDetectionPercent", oldValue, value);
return (T) this;
}
/**
* The maximum amount of buffers to keep in the pool. If more buffers are
* required at runtime they will be allocated dynamically. Setting this to
* zero effectively disables pooling.
*/
@ModelNodeBinding(detypedName = "max-pool-size")
public Integer maxPoolSize() {
return this.maxPoolSize;
}
/**
* The maximum amount of buffers to keep in the pool. If more buffers are
* required at runtime they will be allocated dynamically. Setting this to
* zero effectively disables pooling.
*/
@SuppressWarnings("unchecked")
public T maxPoolSize(java.lang.Integer value) {
Object oldValue = this.maxPoolSize;
this.maxPoolSize = value;
if (this.pcs != null)
this.pcs.firePropertyChange("maxPoolSize", oldValue, value);
return (T) this;
}
/**
* The maximum number of buffers to cache on each thread. The actual number
* may be lower depending on the calculated usage pattern.
*/
@ModelNodeBinding(detypedName = "thread-local-cache-size")
public Integer threadLocalCacheSize() {
return this.threadLocalCacheSize;
}
/**
* The maximum number of buffers to cache on each thread. The actual number
* may be lower depending on the calculated usage pattern.
*/
@SuppressWarnings("unchecked")
public T threadLocalCacheSize(java.lang.Integer value) {
Object oldValue = this.threadLocalCacheSize;
this.threadLocalCacheSize = value;
if (this.pcs != null)
this.pcs.firePropertyChange("threadLocalCacheSize", oldValue, value);
return (T) this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy