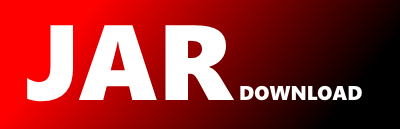
org.wildfly.swarm.config.undertow.Server Maven / Gradle / Ivy
package org.wildfly.swarm.config.undertow;
import org.wildfly.swarm.config.runtime.AttributeDocumentation;
import org.wildfly.swarm.config.runtime.ResourceDocumentation;
import org.wildfly.swarm.config.runtime.SingletonResource;
import org.wildfly.swarm.config.runtime.Address;
import org.wildfly.swarm.config.runtime.ResourceType;
import java.beans.PropertyChangeSupport;
import java.beans.PropertyChangeListener;
import java.util.List;
import org.wildfly.swarm.config.runtime.Subresource;
import org.wildfly.swarm.config.undertow.server.HostConsumer;
import org.wildfly.swarm.config.undertow.server.HostSupplier;
import org.wildfly.swarm.config.undertow.server.Host;
import org.wildfly.swarm.config.runtime.SubresourceInfo;
import org.wildfly.swarm.config.undertow.server.HTTPListenerConsumer;
import org.wildfly.swarm.config.undertow.server.HTTPListenerSupplier;
import org.wildfly.swarm.config.undertow.server.HTTPListener;
import org.wildfly.swarm.config.undertow.server.AJPListenerConsumer;
import org.wildfly.swarm.config.undertow.server.AJPListenerSupplier;
import org.wildfly.swarm.config.undertow.server.AJPListener;
import org.wildfly.swarm.config.undertow.server.HttpsListenerConsumer;
import org.wildfly.swarm.config.undertow.server.HttpsListenerSupplier;
import org.wildfly.swarm.config.undertow.server.HttpsListener;
import org.wildfly.swarm.config.runtime.ModelNodeBinding;
/**
* A server
*/
@Address("/subsystem=undertow/server=*")
@ResourceType("server")
public class Server>
implements
org.wildfly.swarm.config.runtime.Keyed {
private String key;
private PropertyChangeSupport pcs;
private ServerResources subresources = new ServerResources();
@AttributeDocumentation("The servers default virtual host")
private String defaultHost;
@AttributeDocumentation("The servers default servlet container")
private String servletContainer;
public Server(java.lang.String key) {
super();
this.key = key;
}
public String getKey() {
return this.key;
}
/**
* Adds a property change listener
*/
public void addPropertyChangeListener(PropertyChangeListener listener) {
if (null == this.pcs)
this.pcs = new PropertyChangeSupport(this);
this.pcs.addPropertyChangeListener(listener);
}
/**
* Removes a property change listener
*/
public void removePropertyChangeListener(
java.beans.PropertyChangeListener listener) {
if (this.pcs != null)
this.pcs.removePropertyChangeListener(listener);
}
public ServerResources subresources() {
return this.subresources;
}
/**
* Add all Host objects to this subresource
*
* @return this
* @param value
* List of Host objects.
*/
@SuppressWarnings("unchecked")
public T hosts(java.util.List value) {
this.subresources.hosts = value;
return (T) this;
}
/**
* Add the Host object to the list of subresources
*
* @param value
* The Host to add
* @return this
*/
@SuppressWarnings("unchecked")
public T host(Host value) {
this.subresources.hosts.add(value);
return (T) this;
}
/**
* Create and configure a Host object to the list of subresources
*
* @param key
* The key for the Host resource
* @param config
* The HostConsumer to use
* @return this
*/
@SuppressWarnings("unchecked")
public T host(java.lang.String childKey, HostConsumer consumer) {
Host extends Host> child = new Host<>(childKey);
if (consumer != null) {
consumer.accept(child);
}
host(child);
return (T) this;
}
/**
* Create and configure a Host object to the list of subresources
*
* @param key
* The key for the Host resource
* @return this
*/
@SuppressWarnings("unchecked")
public T host(java.lang.String childKey) {
host(childKey, null);
return (T) this;
}
/**
* Install a supplied Host object to the list of subresources
*/
@SuppressWarnings("unchecked")
public T host(HostSupplier supplier) {
host(supplier.get());
return (T) this;
}
/**
* Add all HTTPListener objects to this subresource
*
* @return this
* @param value
* List of HTTPListener objects.
*/
@SuppressWarnings("unchecked")
public T httpListeners(java.util.List value) {
this.subresources.httpListeners = value;
return (T) this;
}
/**
* Add the HTTPListener object to the list of subresources
*
* @param value
* The HTTPListener to add
* @return this
*/
@SuppressWarnings("unchecked")
public T httpListener(HTTPListener value) {
this.subresources.httpListeners.add(value);
return (T) this;
}
/**
* Create and configure a HTTPListener object to the list of subresources
*
* @param key
* The key for the HTTPListener resource
* @param config
* The HTTPListenerConsumer to use
* @return this
*/
@SuppressWarnings("unchecked")
public T httpListener(java.lang.String childKey,
HTTPListenerConsumer consumer) {
HTTPListener extends HTTPListener> child = new HTTPListener<>(
childKey);
if (consumer != null) {
consumer.accept(child);
}
httpListener(child);
return (T) this;
}
/**
* Create and configure a HTTPListener object to the list of subresources
*
* @param key
* The key for the HTTPListener resource
* @return this
*/
@SuppressWarnings("unchecked")
public T httpListener(java.lang.String childKey) {
httpListener(childKey, null);
return (T) this;
}
/**
* Install a supplied HTTPListener object to the list of subresources
*/
@SuppressWarnings("unchecked")
public T httpListener(HTTPListenerSupplier supplier) {
httpListener(supplier.get());
return (T) this;
}
/**
* Add all AJPListener objects to this subresource
*
* @return this
* @param value
* List of AJPListener objects.
*/
@SuppressWarnings("unchecked")
public T ajpListeners(java.util.List value) {
this.subresources.ajpListeners = value;
return (T) this;
}
/**
* Add the AJPListener object to the list of subresources
*
* @param value
* The AJPListener to add
* @return this
*/
@SuppressWarnings("unchecked")
public T ajpListener(AJPListener value) {
this.subresources.ajpListeners.add(value);
return (T) this;
}
/**
* Create and configure a AJPListener object to the list of subresources
*
* @param key
* The key for the AJPListener resource
* @param config
* The AJPListenerConsumer to use
* @return this
*/
@SuppressWarnings("unchecked")
public T ajpListener(java.lang.String childKey, AJPListenerConsumer consumer) {
AJPListener extends AJPListener> child = new AJPListener<>(childKey);
if (consumer != null) {
consumer.accept(child);
}
ajpListener(child);
return (T) this;
}
/**
* Create and configure a AJPListener object to the list of subresources
*
* @param key
* The key for the AJPListener resource
* @return this
*/
@SuppressWarnings("unchecked")
public T ajpListener(java.lang.String childKey) {
ajpListener(childKey, null);
return (T) this;
}
/**
* Install a supplied AJPListener object to the list of subresources
*/
@SuppressWarnings("unchecked")
public T ajpListener(AJPListenerSupplier supplier) {
ajpListener(supplier.get());
return (T) this;
}
/**
* Add all HttpsListener objects to this subresource
*
* @return this
* @param value
* List of HttpsListener objects.
*/
@SuppressWarnings("unchecked")
public T httpsListeners(java.util.List value) {
this.subresources.httpsListeners = value;
return (T) this;
}
/**
* Add the HttpsListener object to the list of subresources
*
* @param value
* The HttpsListener to add
* @return this
*/
@SuppressWarnings("unchecked")
public T httpsListener(HttpsListener value) {
this.subresources.httpsListeners.add(value);
return (T) this;
}
/**
* Create and configure a HttpsListener object to the list of subresources
*
* @param key
* The key for the HttpsListener resource
* @param config
* The HttpsListenerConsumer to use
* @return this
*/
@SuppressWarnings("unchecked")
public T httpsListener(java.lang.String childKey,
HttpsListenerConsumer consumer) {
HttpsListener extends HttpsListener> child = new HttpsListener<>(
childKey);
if (consumer != null) {
consumer.accept(child);
}
httpsListener(child);
return (T) this;
}
/**
* Create and configure a HttpsListener object to the list of subresources
*
* @param key
* The key for the HttpsListener resource
* @return this
*/
@SuppressWarnings("unchecked")
public T httpsListener(java.lang.String childKey) {
httpsListener(childKey, null);
return (T) this;
}
/**
* Install a supplied HttpsListener object to the list of subresources
*/
@SuppressWarnings("unchecked")
public T httpsListener(HttpsListenerSupplier supplier) {
httpsListener(supplier.get());
return (T) this;
}
/**
* Child mutators for Server
*/
public static class ServerResources {
/**
* An Undertow host
*/
@ResourceDocumentation("An Undertow host")
@SubresourceInfo("host")
private List hosts = new java.util.ArrayList<>();
/**
* http listener
*/
@ResourceDocumentation("http listener")
@SubresourceInfo("httpListener")
private List httpListeners = new java.util.ArrayList<>();
/**
* http listener
*/
@ResourceDocumentation("http listener")
@SubresourceInfo("ajpListener")
private List ajpListeners = new java.util.ArrayList<>();
/**
* http listener
*/
@ResourceDocumentation("http listener")
@SubresourceInfo("httpsListener")
private List httpsListeners = new java.util.ArrayList<>();
/**
* Get the list of Host resources
*
* @return the list of resources
*/
@Subresource
public List hosts() {
return this.hosts;
}
public Host host(java.lang.String key) {
return this.hosts.stream().filter(e -> e.getKey().equals(key))
.findFirst().orElse(null);
}
/**
* Get the list of HTTPListener resources
*
* @return the list of resources
*/
@Subresource
public List httpListeners() {
return this.httpListeners;
}
public HTTPListener httpListener(java.lang.String key) {
return this.httpListeners.stream()
.filter(e -> e.getKey().equals(key)).findFirst()
.orElse(null);
}
/**
* Get the list of AJPListener resources
*
* @return the list of resources
*/
@Subresource
public List ajpListeners() {
return this.ajpListeners;
}
public AJPListener ajpListener(java.lang.String key) {
return this.ajpListeners.stream()
.filter(e -> e.getKey().equals(key)).findFirst()
.orElse(null);
}
/**
* Get the list of HttpsListener resources
*
* @return the list of resources
*/
@Subresource
public List httpsListeners() {
return this.httpsListeners;
}
public HttpsListener httpsListener(java.lang.String key) {
return this.httpsListeners.stream()
.filter(e -> e.getKey().equals(key)).findFirst()
.orElse(null);
}
}
/**
* The servers default virtual host
*/
@ModelNodeBinding(detypedName = "default-host")
public String defaultHost() {
return this.defaultHost;
}
/**
* The servers default virtual host
*/
@SuppressWarnings("unchecked")
public T defaultHost(java.lang.String value) {
Object oldValue = this.defaultHost;
this.defaultHost = value;
if (this.pcs != null)
this.pcs.firePropertyChange("defaultHost", oldValue, value);
return (T) this;
}
/**
* The servers default servlet container
*/
@ModelNodeBinding(detypedName = "servlet-container")
public String servletContainer() {
return this.servletContainer;
}
/**
* The servers default servlet container
*/
@SuppressWarnings("unchecked")
public T servletContainer(java.lang.String value) {
Object oldValue = this.servletContainer;
this.servletContainer = value;
if (this.pcs != null)
this.pcs.firePropertyChange("servletContainer", oldValue, value);
return (T) this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy