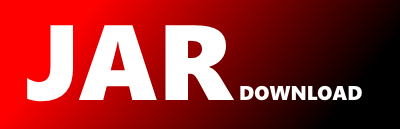
org.wildfly.swarm.config.Datasources Maven / Gradle / Ivy
package org.wildfly.swarm.config;
import org.wildfly.swarm.config.runtime.AttributeDocumentation;
import org.wildfly.swarm.config.runtime.ResourceDocumentation;
import org.wildfly.swarm.config.runtime.SingletonResource;
import org.wildfly.swarm.config.runtime.Address;
import org.wildfly.swarm.config.runtime.ResourceType;
import org.wildfly.swarm.config.runtime.Implicit;
import java.beans.PropertyChangeSupport;
import java.beans.PropertyChangeListener;
import java.util.List;
import org.wildfly.swarm.config.runtime.Subresource;
import org.wildfly.swarm.config.datasources.JDBCDriverConsumer;
import org.wildfly.swarm.config.datasources.JDBCDriverSupplier;
import org.wildfly.swarm.config.datasources.JDBCDriver;
import org.wildfly.swarm.config.runtime.SubresourceInfo;
import org.wildfly.swarm.config.datasources.XADataSourceConsumer;
import org.wildfly.swarm.config.datasources.XADataSourceSupplier;
import org.wildfly.swarm.config.datasources.XADataSource;
import org.wildfly.swarm.config.datasources.DataSourceConsumer;
import org.wildfly.swarm.config.datasources.DataSourceSupplier;
import org.wildfly.swarm.config.datasources.DataSource;
import org.wildfly.swarm.config.runtime.ModelNodeBinding;
import java.util.Map;
import java.util.Arrays;
import java.util.stream.Collectors;
/**
* The data-sources subsystem, used to declare JDBC data-sources
*/
@Address("/subsystem=datasources")
@ResourceType("subsystem")
@Implicit
public class Datasources>
implements
org.wildfly.swarm.config.runtime.Keyed {
private String key;
private PropertyChangeSupport pcs;
private DatasourcesResources subresources = new DatasourcesResources();
@AttributeDocumentation("List of JDBC drivers that have been installed in the runtime")
private List installedDrivers;
public Datasources() {
super();
this.key = "datasources";
this.pcs = new PropertyChangeSupport(this);
}
public String getKey() {
return this.key;
}
/**
* Adds a property change listener
*/
public void addPropertyChangeListener(PropertyChangeListener listener) {
if (null == this.pcs)
this.pcs = new PropertyChangeSupport(this);
this.pcs.addPropertyChangeListener(listener);
}
/**
* Removes a property change listener
*/
public void removePropertyChangeListener(
java.beans.PropertyChangeListener listener) {
if (this.pcs != null)
this.pcs.removePropertyChangeListener(listener);
}
public DatasourcesResources subresources() {
return this.subresources;
}
/**
* Add all JDBCDriver objects to this subresource
*
* @return this
* @param value
* List of JDBCDriver objects.
*/
@SuppressWarnings("unchecked")
public T jdbcDrivers(java.util.List value) {
this.subresources.jdbcDrivers = value;
return (T) this;
}
/**
* Add the JDBCDriver object to the list of subresources
*
* @param value
* The JDBCDriver to add
* @return this
*/
@SuppressWarnings("unchecked")
public T jdbcDriver(JDBCDriver value) {
this.subresources.jdbcDrivers.add(value);
return (T) this;
}
/**
* Create and configure a JDBCDriver object to the list of subresources
*
* @param key
* The key for the JDBCDriver resource
* @param config
* The JDBCDriverConsumer to use
* @return this
*/
@SuppressWarnings("unchecked")
public T jdbcDriver(java.lang.String childKey, JDBCDriverConsumer consumer) {
JDBCDriver extends JDBCDriver> child = new JDBCDriver<>(childKey);
if (consumer != null) {
consumer.accept(child);
}
jdbcDriver(child);
return (T) this;
}
/**
* Create and configure a JDBCDriver object to the list of subresources
*
* @param key
* The key for the JDBCDriver resource
* @return this
*/
@SuppressWarnings("unchecked")
public T jdbcDriver(java.lang.String childKey) {
jdbcDriver(childKey, null);
return (T) this;
}
/**
* Install a supplied JDBCDriver object to the list of subresources
*/
@SuppressWarnings("unchecked")
public T jdbcDriver(JDBCDriverSupplier supplier) {
jdbcDriver(supplier.get());
return (T) this;
}
/**
* Add all XADataSource objects to this subresource
*
* @return this
* @param value
* List of XADataSource objects.
*/
@SuppressWarnings("unchecked")
public T xaDataSources(java.util.List value) {
this.subresources.xaDataSources = value;
return (T) this;
}
/**
* Add the XADataSource object to the list of subresources
*
* @param value
* The XADataSource to add
* @return this
*/
@SuppressWarnings("unchecked")
public T xaDataSource(XADataSource value) {
this.subresources.xaDataSources.add(value);
return (T) this;
}
/**
* Create and configure a XADataSource object to the list of subresources
*
* @param key
* The key for the XADataSource resource
* @param config
* The XADataSourceConsumer to use
* @return this
*/
@SuppressWarnings("unchecked")
public T xaDataSource(java.lang.String childKey,
XADataSourceConsumer consumer) {
XADataSource extends XADataSource> child = new XADataSource<>(
childKey);
if (consumer != null) {
consumer.accept(child);
}
xaDataSource(child);
return (T) this;
}
/**
* Create and configure a XADataSource object to the list of subresources
*
* @param key
* The key for the XADataSource resource
* @return this
*/
@SuppressWarnings("unchecked")
public T xaDataSource(java.lang.String childKey) {
xaDataSource(childKey, null);
return (T) this;
}
/**
* Install a supplied XADataSource object to the list of subresources
*/
@SuppressWarnings("unchecked")
public T xaDataSource(XADataSourceSupplier supplier) {
xaDataSource(supplier.get());
return (T) this;
}
/**
* Add all DataSource objects to this subresource
*
* @return this
* @param value
* List of DataSource objects.
*/
@SuppressWarnings("unchecked")
public T dataSources(java.util.List value) {
this.subresources.dataSources = value;
return (T) this;
}
/**
* Add the DataSource object to the list of subresources
*
* @param value
* The DataSource to add
* @return this
*/
@SuppressWarnings("unchecked")
public T dataSource(DataSource value) {
this.subresources.dataSources.add(value);
return (T) this;
}
/**
* Create and configure a DataSource object to the list of subresources
*
* @param key
* The key for the DataSource resource
* @param config
* The DataSourceConsumer to use
* @return this
*/
@SuppressWarnings("unchecked")
public T dataSource(java.lang.String childKey, DataSourceConsumer consumer) {
DataSource extends DataSource> child = new DataSource<>(childKey);
if (consumer != null) {
consumer.accept(child);
}
dataSource(child);
return (T) this;
}
/**
* Create and configure a DataSource object to the list of subresources
*
* @param key
* The key for the DataSource resource
* @return this
*/
@SuppressWarnings("unchecked")
public T dataSource(java.lang.String childKey) {
dataSource(childKey, null);
return (T) this;
}
/**
* Install a supplied DataSource object to the list of subresources
*/
@SuppressWarnings("unchecked")
public T dataSource(DataSourceSupplier supplier) {
dataSource(supplier.get());
return (T) this;
}
/**
* Child mutators for Datasources
*/
public static class DatasourcesResources {
/**
* Service that make a JDBC driver available for use in the runtime
*/
@ResourceDocumentation("Service that make a JDBC driver available for use in the runtime")
@SubresourceInfo("jdbcDriver")
private List jdbcDrivers = new java.util.ArrayList<>();
/**
* A JDBC XA data-source configuration
*/
@ResourceDocumentation("A JDBC XA data-source configuration")
@SubresourceInfo("xaDataSource")
private List xaDataSources = new java.util.ArrayList<>();
/**
* A JDBC data-source configuration
*/
@ResourceDocumentation("A JDBC data-source configuration")
@SubresourceInfo("dataSource")
private List dataSources = new java.util.ArrayList<>();
/**
* Get the list of JDBCDriver resources
*
* @return the list of resources
*/
@Subresource
public List jdbcDrivers() {
return this.jdbcDrivers;
}
public JDBCDriver jdbcDriver(java.lang.String key) {
return this.jdbcDrivers.stream()
.filter(e -> e.getKey().equals(key)).findFirst()
.orElse(null);
}
/**
* Get the list of XADataSource resources
*
* @return the list of resources
*/
@Subresource
public List xaDataSources() {
return this.xaDataSources;
}
public XADataSource xaDataSource(java.lang.String key) {
return this.xaDataSources.stream()
.filter(e -> e.getKey().equals(key)).findFirst()
.orElse(null);
}
/**
* Get the list of DataSource resources
*
* @return the list of resources
*/
@Subresource
public List dataSources() {
return this.dataSources;
}
public DataSource dataSource(java.lang.String key) {
return this.dataSources.stream()
.filter(e -> e.getKey().equals(key)).findFirst()
.orElse(null);
}
}
/**
* List of JDBC drivers that have been installed in the runtime
*/
@ModelNodeBinding(detypedName = "installed-drivers")
public List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy