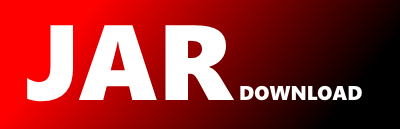
org.wildfly.swarm.config.elytron.ServerSslContext Maven / Gradle / Ivy
package org.wildfly.swarm.config.elytron;
import org.wildfly.swarm.config.runtime.AttributeDocumentation;
import org.wildfly.swarm.config.runtime.ResourceDocumentation;
import org.wildfly.swarm.config.runtime.SingletonResource;
import org.wildfly.swarm.config.runtime.Address;
import org.wildfly.swarm.config.runtime.ResourceType;
import java.beans.PropertyChangeSupport;
import java.beans.PropertyChangeListener;
import java.util.List;
import org.wildfly.swarm.config.runtime.Subresource;
import org.wildfly.swarm.config.elytron.SslSessionConsumer;
import org.wildfly.swarm.config.elytron.SslSessionSupplier;
import org.wildfly.swarm.config.elytron.SslSession;
import org.wildfly.swarm.config.runtime.SubresourceInfo;
import org.wildfly.swarm.config.runtime.ModelNodeBinding;
import java.util.Arrays;
import java.util.stream.Collectors;
/**
* An SSLContext for use on the server side of a connection.
*/
@Address("/subsystem=elytron/server-ssl-context=*")
@ResourceType("server-ssl-context")
public class ServerSslContext>
implements
org.wildfly.swarm.config.runtime.Keyed {
private String key;
private PropertyChangeSupport pcs;
private ServerSslContextResources subresources = new ServerSslContextResources();
@AttributeDocumentation("The count of current active sessions.")
private Integer activeSessionCount;
@AttributeDocumentation("Rejecting of the client certificate by the security domain will not prevent the connection. Allows a fall through to use other authentication mechanisms (like form login) when the client certificate is rejected by security domain. Has an effect only when the security domain is set.")
private Boolean authenticationOptional;
@AttributeDocumentation("The filter to apply to specify the enabled cipher suites.")
private String cipherSuiteFilter;
@AttributeDocumentation("A final principal transformer to apply for this mechanism realm.")
private String finalPrincipalTransformer;
@AttributeDocumentation("Reference to the key manager to use within the SSLContext.")
private String keyManager;
@AttributeDocumentation("The maximum number of SSL sessions in the cache. The default value -1 means use the JVM default value. Value zero means there is no limit.")
private Integer maximumSessionCacheSize;
@AttributeDocumentation("To require a client certificate on SSL handshake. Connection without trusted client certificate (see trust-manager) will be rejected.")
private Boolean needClientAuth;
@AttributeDocumentation("A principal transformer to apply after the realm is selected.")
private String postRealmPrincipalTransformer;
@AttributeDocumentation("A principal transformer to apply before the realm is selected.")
private String preRealmPrincipalTransformer;
@AttributeDocumentation("The enabled protocols.")
private List protocols;
@AttributeDocumentation("The name of the provider to use. If not specified, all providers from providers will be passed to the SSLContext.")
private String providerName;
@AttributeDocumentation("The name of the providers to obtain the Provider[] to use to load the SSLContext.")
private String providers;
@AttributeDocumentation("The realm mapper to be used for SSL authentication.")
private String realmMapper;
@AttributeDocumentation("The security domain to use for authentication during SSL session establishment.")
private String securityDomain;
@AttributeDocumentation("The timeout for SSL sessions, in seconds. The default value -1 means use the JVM default value. Value zero means there is no limit.")
private Integer sessionTimeout;
@AttributeDocumentation("Reference to the trust manager to use within the SSLContext.")
private String trustManager;
@AttributeDocumentation("To honor local cipher suites preference.")
private Boolean useCipherSuitesOrder;
@AttributeDocumentation("To request (but not to require) a client certificate on SSL handshake. If a security domain is referenced and supports X509 evidence, this will be set to true automatically. Ignored when need-client-auth is set.")
private Boolean wantClientAuth;
@AttributeDocumentation("Should the SSLEngine, SSLSocket, and SSLServerSocket instances returned be wrapped to protect against further modification.")
private Boolean wrap;
public ServerSslContext(java.lang.String key) {
super();
this.key = key;
}
public String getKey() {
return this.key;
}
/**
* Adds a property change listener
*/
public void addPropertyChangeListener(PropertyChangeListener listener) {
if (null == this.pcs)
this.pcs = new PropertyChangeSupport(this);
this.pcs.addPropertyChangeListener(listener);
}
/**
* Removes a property change listener
*/
public void removePropertyChangeListener(
java.beans.PropertyChangeListener listener) {
if (this.pcs != null)
this.pcs.removePropertyChangeListener(listener);
}
public ServerSslContextResources subresources() {
return this.subresources;
}
/**
* Add all SslSession objects to this subresource
*
* @return this
* @param value
* List of SslSession objects.
*/
@SuppressWarnings("unchecked")
public T sslSessions(java.util.List value) {
this.subresources.sslSessions = value;
return (T) this;
}
/**
* Add the SslSession object to the list of subresources
*
* @param value
* The SslSession to add
* @return this
*/
@SuppressWarnings("unchecked")
public T sslSession(SslSession value) {
this.subresources.sslSessions.add(value);
return (T) this;
}
/**
* Create and configure a SslSession object to the list of subresources
*
* @param key
* The key for the SslSession resource
* @param config
* The SslSessionConsumer to use
* @return this
*/
@SuppressWarnings("unchecked")
public T sslSession(java.lang.String childKey, SslSessionConsumer consumer) {
SslSession extends SslSession> child = new SslSession<>(childKey);
if (consumer != null) {
consumer.accept(child);
}
sslSession(child);
return (T) this;
}
/**
* Create and configure a SslSession object to the list of subresources
*
* @param key
* The key for the SslSession resource
* @return this
*/
@SuppressWarnings("unchecked")
public T sslSession(java.lang.String childKey) {
sslSession(childKey, null);
return (T) this;
}
/**
* Install a supplied SslSession object to the list of subresources
*/
@SuppressWarnings("unchecked")
public T sslSession(SslSessionSupplier supplier) {
sslSession(supplier.get());
return (T) this;
}
/**
* Child mutators for ServerSslContext
*/
public static class ServerSslContextResources {
/**
* A currently established SSL session.
*/
@ResourceDocumentation("A currently established SSL session.")
@SubresourceInfo("sslSession")
private List sslSessions = new java.util.ArrayList<>();
/**
* Get the list of SslSession resources
*
* @return the list of resources
*/
@Subresource
public List sslSessions() {
return this.sslSessions;
}
public SslSession sslSession(java.lang.String key) {
return this.sslSessions.stream()
.filter(e -> e.getKey().equals(key)).findFirst()
.orElse(null);
}
}
/**
* The count of current active sessions.
*/
@ModelNodeBinding(detypedName = "active-session-count")
public Integer activeSessionCount() {
return this.activeSessionCount;
}
/**
* The count of current active sessions.
*/
@SuppressWarnings("unchecked")
public T activeSessionCount(java.lang.Integer value) {
Object oldValue = this.activeSessionCount;
this.activeSessionCount = value;
if (this.pcs != null)
this.pcs.firePropertyChange("activeSessionCount", oldValue, value);
return (T) this;
}
/**
* Rejecting of the client certificate by the security domain will not
* prevent the connection. Allows a fall through to use other authentication
* mechanisms (like form login) when the client certificate is rejected by
* security domain. Has an effect only when the security domain is set.
*/
@ModelNodeBinding(detypedName = "authentication-optional")
public Boolean authenticationOptional() {
return this.authenticationOptional;
}
/**
* Rejecting of the client certificate by the security domain will not
* prevent the connection. Allows a fall through to use other authentication
* mechanisms (like form login) when the client certificate is rejected by
* security domain. Has an effect only when the security domain is set.
*/
@SuppressWarnings("unchecked")
public T authenticationOptional(java.lang.Boolean value) {
Object oldValue = this.authenticationOptional;
this.authenticationOptional = value;
if (this.pcs != null)
this.pcs.firePropertyChange("authenticationOptional", oldValue,
value);
return (T) this;
}
/**
* The filter to apply to specify the enabled cipher suites.
*/
@ModelNodeBinding(detypedName = "cipher-suite-filter")
public String cipherSuiteFilter() {
return this.cipherSuiteFilter;
}
/**
* The filter to apply to specify the enabled cipher suites.
*/
@SuppressWarnings("unchecked")
public T cipherSuiteFilter(java.lang.String value) {
Object oldValue = this.cipherSuiteFilter;
this.cipherSuiteFilter = value;
if (this.pcs != null)
this.pcs.firePropertyChange("cipherSuiteFilter", oldValue, value);
return (T) this;
}
/**
* A final principal transformer to apply for this mechanism realm.
*/
@ModelNodeBinding(detypedName = "final-principal-transformer")
public String finalPrincipalTransformer() {
return this.finalPrincipalTransformer;
}
/**
* A final principal transformer to apply for this mechanism realm.
*/
@SuppressWarnings("unchecked")
public T finalPrincipalTransformer(java.lang.String value) {
Object oldValue = this.finalPrincipalTransformer;
this.finalPrincipalTransformer = value;
if (this.pcs != null)
this.pcs.firePropertyChange("finalPrincipalTransformer", oldValue,
value);
return (T) this;
}
/**
* Reference to the key manager to use within the SSLContext.
*/
@ModelNodeBinding(detypedName = "key-manager")
public String keyManager() {
return this.keyManager;
}
/**
* Reference to the key manager to use within the SSLContext.
*/
@SuppressWarnings("unchecked")
public T keyManager(java.lang.String value) {
Object oldValue = this.keyManager;
this.keyManager = value;
if (this.pcs != null)
this.pcs.firePropertyChange("keyManager", oldValue, value);
return (T) this;
}
/**
* The maximum number of SSL sessions in the cache. The default value -1
* means use the JVM default value. Value zero means there is no limit.
*/
@ModelNodeBinding(detypedName = "maximum-session-cache-size")
public Integer maximumSessionCacheSize() {
return this.maximumSessionCacheSize;
}
/**
* The maximum number of SSL sessions in the cache. The default value -1
* means use the JVM default value. Value zero means there is no limit.
*/
@SuppressWarnings("unchecked")
public T maximumSessionCacheSize(java.lang.Integer value) {
Object oldValue = this.maximumSessionCacheSize;
this.maximumSessionCacheSize = value;
if (this.pcs != null)
this.pcs.firePropertyChange("maximumSessionCacheSize", oldValue,
value);
return (T) this;
}
/**
* To require a client certificate on SSL handshake. Connection without
* trusted client certificate (see trust-manager) will be rejected.
*/
@ModelNodeBinding(detypedName = "need-client-auth")
public Boolean needClientAuth() {
return this.needClientAuth;
}
/**
* To require a client certificate on SSL handshake. Connection without
* trusted client certificate (see trust-manager) will be rejected.
*/
@SuppressWarnings("unchecked")
public T needClientAuth(java.lang.Boolean value) {
Object oldValue = this.needClientAuth;
this.needClientAuth = value;
if (this.pcs != null)
this.pcs.firePropertyChange("needClientAuth", oldValue, value);
return (T) this;
}
/**
* A principal transformer to apply after the realm is selected.
*/
@ModelNodeBinding(detypedName = "post-realm-principal-transformer")
public String postRealmPrincipalTransformer() {
return this.postRealmPrincipalTransformer;
}
/**
* A principal transformer to apply after the realm is selected.
*/
@SuppressWarnings("unchecked")
public T postRealmPrincipalTransformer(java.lang.String value) {
Object oldValue = this.postRealmPrincipalTransformer;
this.postRealmPrincipalTransformer = value;
if (this.pcs != null)
this.pcs.firePropertyChange("postRealmPrincipalTransformer",
oldValue, value);
return (T) this;
}
/**
* A principal transformer to apply before the realm is selected.
*/
@ModelNodeBinding(detypedName = "pre-realm-principal-transformer")
public String preRealmPrincipalTransformer() {
return this.preRealmPrincipalTransformer;
}
/**
* A principal transformer to apply before the realm is selected.
*/
@SuppressWarnings("unchecked")
public T preRealmPrincipalTransformer(java.lang.String value) {
Object oldValue = this.preRealmPrincipalTransformer;
this.preRealmPrincipalTransformer = value;
if (this.pcs != null)
this.pcs.firePropertyChange("preRealmPrincipalTransformer",
oldValue, value);
return (T) this;
}
/**
* The enabled protocols.
*/
@ModelNodeBinding(detypedName = "protocols")
public List protocols() {
return this.protocols;
}
/**
* The enabled protocols.
*/
@SuppressWarnings("unchecked")
public T protocols(java.util.List value) {
Object oldValue = this.protocols;
this.protocols = value;
if (this.pcs != null)
this.pcs.firePropertyChange("protocols", oldValue, value);
return (T) this;
}
/**
* The enabled protocols.
*/
@SuppressWarnings("unchecked")
public T protocol(String value) {
if (this.protocols == null) {
this.protocols = new java.util.ArrayList<>();
}
this.protocols.add(value);
return (T) this;
}
/**
* The enabled protocols.
*/
@SuppressWarnings("unchecked")
public T protocols(String... args) {
protocols(Arrays.stream(args).collect(Collectors.toList()));
return (T) this;
}
/**
* The name of the provider to use. If not specified, all providers from
* providers will be passed to the SSLContext.
*/
@ModelNodeBinding(detypedName = "provider-name")
public String providerName() {
return this.providerName;
}
/**
* The name of the provider to use. If not specified, all providers from
* providers will be passed to the SSLContext.
*/
@SuppressWarnings("unchecked")
public T providerName(java.lang.String value) {
Object oldValue = this.providerName;
this.providerName = value;
if (this.pcs != null)
this.pcs.firePropertyChange("providerName", oldValue, value);
return (T) this;
}
/**
* The name of the providers to obtain the Provider[] to use to load the
* SSLContext.
*/
@ModelNodeBinding(detypedName = "providers")
public String providers() {
return this.providers;
}
/**
* The name of the providers to obtain the Provider[] to use to load the
* SSLContext.
*/
@SuppressWarnings("unchecked")
public T providers(java.lang.String value) {
Object oldValue = this.providers;
this.providers = value;
if (this.pcs != null)
this.pcs.firePropertyChange("providers", oldValue, value);
return (T) this;
}
/**
* The realm mapper to be used for SSL authentication.
*/
@ModelNodeBinding(detypedName = "realm-mapper")
public String realmMapper() {
return this.realmMapper;
}
/**
* The realm mapper to be used for SSL authentication.
*/
@SuppressWarnings("unchecked")
public T realmMapper(java.lang.String value) {
Object oldValue = this.realmMapper;
this.realmMapper = value;
if (this.pcs != null)
this.pcs.firePropertyChange("realmMapper", oldValue, value);
return (T) this;
}
/**
* The security domain to use for authentication during SSL session
* establishment.
*/
@ModelNodeBinding(detypedName = "security-domain")
public String securityDomain() {
return this.securityDomain;
}
/**
* The security domain to use for authentication during SSL session
* establishment.
*/
@SuppressWarnings("unchecked")
public T securityDomain(java.lang.String value) {
Object oldValue = this.securityDomain;
this.securityDomain = value;
if (this.pcs != null)
this.pcs.firePropertyChange("securityDomain", oldValue, value);
return (T) this;
}
/**
* The timeout for SSL sessions, in seconds. The default value -1 means use
* the JVM default value. Value zero means there is no limit.
*/
@ModelNodeBinding(detypedName = "session-timeout")
public Integer sessionTimeout() {
return this.sessionTimeout;
}
/**
* The timeout for SSL sessions, in seconds. The default value -1 means use
* the JVM default value. Value zero means there is no limit.
*/
@SuppressWarnings("unchecked")
public T sessionTimeout(java.lang.Integer value) {
Object oldValue = this.sessionTimeout;
this.sessionTimeout = value;
if (this.pcs != null)
this.pcs.firePropertyChange("sessionTimeout", oldValue, value);
return (T) this;
}
/**
* Reference to the trust manager to use within the SSLContext.
*/
@ModelNodeBinding(detypedName = "trust-manager")
public String trustManager() {
return this.trustManager;
}
/**
* Reference to the trust manager to use within the SSLContext.
*/
@SuppressWarnings("unchecked")
public T trustManager(java.lang.String value) {
Object oldValue = this.trustManager;
this.trustManager = value;
if (this.pcs != null)
this.pcs.firePropertyChange("trustManager", oldValue, value);
return (T) this;
}
/**
* To honor local cipher suites preference.
*/
@ModelNodeBinding(detypedName = "use-cipher-suites-order")
public Boolean useCipherSuitesOrder() {
return this.useCipherSuitesOrder;
}
/**
* To honor local cipher suites preference.
*/
@SuppressWarnings("unchecked")
public T useCipherSuitesOrder(java.lang.Boolean value) {
Object oldValue = this.useCipherSuitesOrder;
this.useCipherSuitesOrder = value;
if (this.pcs != null)
this.pcs.firePropertyChange("useCipherSuitesOrder", oldValue, value);
return (T) this;
}
/**
* To request (but not to require) a client certificate on SSL handshake. If
* a security domain is referenced and supports X509 evidence, this will be
* set to true automatically. Ignored when need-client-auth is set.
*/
@ModelNodeBinding(detypedName = "want-client-auth")
public Boolean wantClientAuth() {
return this.wantClientAuth;
}
/**
* To request (but not to require) a client certificate on SSL handshake. If
* a security domain is referenced and supports X509 evidence, this will be
* set to true automatically. Ignored when need-client-auth is set.
*/
@SuppressWarnings("unchecked")
public T wantClientAuth(java.lang.Boolean value) {
Object oldValue = this.wantClientAuth;
this.wantClientAuth = value;
if (this.pcs != null)
this.pcs.firePropertyChange("wantClientAuth", oldValue, value);
return (T) this;
}
/**
* Should the SSLEngine, SSLSocket, and SSLServerSocket instances returned
* be wrapped to protect against further modification.
*/
@ModelNodeBinding(detypedName = "wrap")
public Boolean wrap() {
return this.wrap;
}
/**
* Should the SSLEngine, SSLSocket, and SSLServerSocket instances returned
* be wrapped to protect against further modification.
*/
@SuppressWarnings("unchecked")
public T wrap(java.lang.Boolean value) {
Object oldValue = this.wrap;
this.wrap = value;
if (this.pcs != null)
this.pcs.firePropertyChange("wrap", oldValue, value);
return (T) this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy