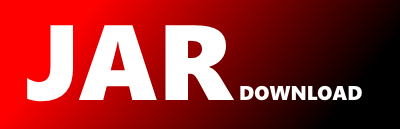
org.wildfly.swarm.config.management.LdapConnection Maven / Gradle / Ivy
package org.wildfly.swarm.config.management;
import org.wildfly.swarm.config.runtime.AttributeDocumentation;
import org.wildfly.swarm.config.runtime.ResourceDocumentation;
import org.wildfly.swarm.config.runtime.SingletonResource;
import org.wildfly.swarm.config.runtime.Address;
import org.wildfly.swarm.config.runtime.ResourceType;
import java.beans.PropertyChangeSupport;
import java.beans.PropertyChangeListener;
import java.util.List;
import org.wildfly.swarm.config.runtime.Subresource;
import org.wildfly.swarm.config.management.PropertyConsumer;
import org.wildfly.swarm.config.management.PropertySupplier;
import org.wildfly.swarm.config.management.Property;
import org.wildfly.swarm.config.runtime.SubresourceInfo;
import org.wildfly.swarm.config.runtime.ModelNodeBinding;
import java.util.Arrays;
import java.util.stream.Collectors;
import java.util.Map;
/**
* A connection factory that can be used by a security realm to access an LDAP
* server as a source of authentication and authorization information.
*/
@Address("/core-service=management/ldap-connection=*")
@ResourceType("ldap-connection")
public class LdapConnection>
implements
org.wildfly.swarm.config.runtime.Keyed {
private String key;
private PropertyChangeSupport pcs;
private LdapConnectionResources subresources = new LdapConnectionResources();
@AttributeDocumentation("If true, the client SSL certificate will be sent to LDAP server with every request; otherwise the client SSL certificate will not be sent when verifying the user credentials")
private Boolean alwaysSendClientCert;
@AttributeDocumentation("List of URLs that this connection handles referrals for.")
private List handlesReferralsFor;
@AttributeDocumentation("The initial context factory to establish the LdapContext.")
private String initialContextFactory;
@AttributeDocumentation("The referral handling mode for this connection.")
private Referrals referrals;
@AttributeDocumentation("The credential to use when connecting to perform a search.")
private String searchCredential;
@AttributeDocumentation("The reference to the search credential stored in CredentialStore under defined alias or clear text password.")
private Map searchCredentialReference;
@AttributeDocumentation("The distinguished name to use when connecting to the LDAP server to perform searches.")
private String searchDn;
@AttributeDocumentation("The security realm to reference to obtain a configured SSLContext to use when establishing the connection.")
private String securityRealm;
@AttributeDocumentation("The URL to use to connect to the LDAP server.")
private String url;
public LdapConnection(java.lang.String key) {
super();
this.key = key;
}
public String getKey() {
return this.key;
}
/**
* Adds a property change listener
*/
public void addPropertyChangeListener(PropertyChangeListener listener) {
if (null == this.pcs)
this.pcs = new PropertyChangeSupport(this);
this.pcs.addPropertyChangeListener(listener);
}
/**
* Removes a property change listener
*/
public void removePropertyChangeListener(
java.beans.PropertyChangeListener listener) {
if (this.pcs != null)
this.pcs.removePropertyChangeListener(listener);
}
public LdapConnectionResources subresources() {
return this.subresources;
}
/**
* Add all Property objects to this subresource
*
* @return this
* @param value
* List of Property objects.
*/
@SuppressWarnings("unchecked")
public T properties(java.util.List value) {
this.subresources.properties = value;
return (T) this;
}
/**
* Add the Property object to the list of subresources
*
* @param value
* The Property to add
* @return this
*/
@SuppressWarnings("unchecked")
public T property(Property value) {
this.subresources.properties.add(value);
return (T) this;
}
/**
* Create and configure a Property object to the list of subresources
*
* @param key
* The key for the Property resource
* @param config
* The PropertyConsumer to use
* @return this
*/
@SuppressWarnings("unchecked")
public T property(java.lang.String childKey, PropertyConsumer consumer) {
Property extends Property> child = new Property<>(childKey);
if (consumer != null) {
consumer.accept(child);
}
property(child);
return (T) this;
}
/**
* Create and configure a Property object to the list of subresources
*
* @param key
* The key for the Property resource
* @return this
*/
@SuppressWarnings("unchecked")
public T property(java.lang.String childKey) {
property(childKey, null);
return (T) this;
}
/**
* Install a supplied Property object to the list of subresources
*/
@SuppressWarnings("unchecked")
public T property(PropertySupplier supplier) {
property(supplier.get());
return (T) this;
}
/**
* Child mutators for LdapConnection
*/
public static class LdapConnectionResources {
/**
* A custom property to use when establishing the LDAP connection.
*/
@ResourceDocumentation("A custom property to use when establishing the LDAP connection.")
@SubresourceInfo("property")
private List properties = new java.util.ArrayList<>();
/**
* Get the list of Property resources
*
* @return the list of resources
*/
@Subresource
public List properties() {
return this.properties;
}
public Property property(java.lang.String key) {
return this.properties.stream().filter(e -> e.getKey().equals(key))
.findFirst().orElse(null);
}
}
public static enum Referrals {
FOLLOW("FOLLOW"), IGNORE("IGNORE"), THROW("THROW");
private final String allowedValue;
/**
* Returns the allowed value for the management model.
*
* @return the allowed model value
*/
public String getAllowedValue() {
return allowedValue;
}
Referrals(java.lang.String allowedValue) {
this.allowedValue = allowedValue;
}
@Override
public String toString() {
return allowedValue;
}
}
/**
* If true, the client SSL certificate will be sent to LDAP server with
* every request; otherwise the client SSL certificate will not be sent when
* verifying the user credentials
*/
@ModelNodeBinding(detypedName = "always-send-client-cert")
public Boolean alwaysSendClientCert() {
return this.alwaysSendClientCert;
}
/**
* If true, the client SSL certificate will be sent to LDAP server with
* every request; otherwise the client SSL certificate will not be sent when
* verifying the user credentials
*/
@SuppressWarnings("unchecked")
public T alwaysSendClientCert(java.lang.Boolean value) {
Object oldValue = this.alwaysSendClientCert;
this.alwaysSendClientCert = value;
if (this.pcs != null)
this.pcs.firePropertyChange("alwaysSendClientCert", oldValue, value);
return (T) this;
}
/**
* List of URLs that this connection handles referrals for.
*/
@ModelNodeBinding(detypedName = "handles-referrals-for")
public List handlesReferralsFor() {
return this.handlesReferralsFor;
}
/**
* List of URLs that this connection handles referrals for.
*/
@SuppressWarnings("unchecked")
public T handlesReferralsFor(java.util.List value) {
Object oldValue = this.handlesReferralsFor;
this.handlesReferralsFor = value;
if (this.pcs != null)
this.pcs.firePropertyChange("handlesReferralsFor", oldValue, value);
return (T) this;
}
/**
* List of URLs that this connection handles referrals for.
*/
@SuppressWarnings("unchecked")
public T handlesReferralsFor(String value) {
if (this.handlesReferralsFor == null) {
this.handlesReferralsFor = new java.util.ArrayList<>();
}
this.handlesReferralsFor.add(value);
return (T) this;
}
/**
* List of URLs that this connection handles referrals for.
*/
@SuppressWarnings("unchecked")
public T handlesReferralsFor(String... args) {
handlesReferralsFor(Arrays.stream(args).collect(Collectors.toList()));
return (T) this;
}
/**
* The initial context factory to establish the LdapContext.
*/
@ModelNodeBinding(detypedName = "initial-context-factory")
public String initialContextFactory() {
return this.initialContextFactory;
}
/**
* The initial context factory to establish the LdapContext.
*/
@SuppressWarnings("unchecked")
public T initialContextFactory(java.lang.String value) {
Object oldValue = this.initialContextFactory;
this.initialContextFactory = value;
if (this.pcs != null)
this.pcs.firePropertyChange("initialContextFactory", oldValue,
value);
return (T) this;
}
/**
* The referral handling mode for this connection.
*/
@ModelNodeBinding(detypedName = "referrals")
public Referrals referrals() {
return this.referrals;
}
/**
* The referral handling mode for this connection.
*/
@SuppressWarnings("unchecked")
public T referrals(Referrals value) {
Object oldValue = this.referrals;
this.referrals = value;
if (this.pcs != null)
this.pcs.firePropertyChange("referrals", oldValue, value);
return (T) this;
}
/**
* The credential to use when connecting to perform a search.
*/
@ModelNodeBinding(detypedName = "search-credential")
public String searchCredential() {
return this.searchCredential;
}
/**
* The credential to use when connecting to perform a search.
*/
@SuppressWarnings("unchecked")
public T searchCredential(java.lang.String value) {
Object oldValue = this.searchCredential;
this.searchCredential = value;
if (this.pcs != null)
this.pcs.firePropertyChange("searchCredential", oldValue, value);
return (T) this;
}
/**
* The reference to the search credential stored in CredentialStore under
* defined alias or clear text password.
*/
@ModelNodeBinding(detypedName = "search-credential-reference")
public Map searchCredentialReference() {
return this.searchCredentialReference;
}
/**
* The reference to the search credential stored in CredentialStore under
* defined alias or clear text password.
*/
@SuppressWarnings("unchecked")
public T searchCredentialReference(java.util.Map value) {
Object oldValue = this.searchCredentialReference;
this.searchCredentialReference = value;
if (this.pcs != null)
this.pcs.firePropertyChange("searchCredentialReference", oldValue,
value);
return (T) this;
}
/**
* The reference to the search credential stored in CredentialStore under
* defined alias or clear text password.
*/
@SuppressWarnings("unchecked")
public T searchCredentialReference(java.lang.String key,
java.lang.Object value) {
if (this.searchCredentialReference == null) {
this.searchCredentialReference = new java.util.HashMap<>();
}
this.searchCredentialReference.put(key, value);
return (T) this;
}
/**
* The distinguished name to use when connecting to the LDAP server to
* perform searches.
*/
@ModelNodeBinding(detypedName = "search-dn")
public String searchDn() {
return this.searchDn;
}
/**
* The distinguished name to use when connecting to the LDAP server to
* perform searches.
*/
@SuppressWarnings("unchecked")
public T searchDn(java.lang.String value) {
Object oldValue = this.searchDn;
this.searchDn = value;
if (this.pcs != null)
this.pcs.firePropertyChange("searchDn", oldValue, value);
return (T) this;
}
/**
* The security realm to reference to obtain a configured SSLContext to use
* when establishing the connection.
*/
@ModelNodeBinding(detypedName = "security-realm")
public String securityRealm() {
return this.securityRealm;
}
/**
* The security realm to reference to obtain a configured SSLContext to use
* when establishing the connection.
*/
@SuppressWarnings("unchecked")
public T securityRealm(java.lang.String value) {
Object oldValue = this.securityRealm;
this.securityRealm = value;
if (this.pcs != null)
this.pcs.firePropertyChange("securityRealm", oldValue, value);
return (T) this;
}
/**
* The URL to use to connect to the LDAP server.
*/
@ModelNodeBinding(detypedName = "url")
public String url() {
return this.url;
}
/**
* The URL to use to connect to the LDAP server.
*/
@SuppressWarnings("unchecked")
public T url(java.lang.String value) {
Object oldValue = this.url;
this.url = value;
if (this.pcs != null)
this.pcs.firePropertyChange("url", oldValue, value);
return (T) this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy