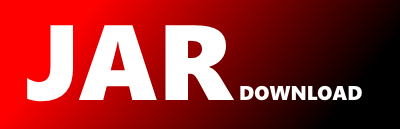
org.wildfly.swarm.config.transactions.CommitMarkableResource Maven / Gradle / Ivy
package org.wildfly.swarm.config.transactions;
import org.wildfly.swarm.config.runtime.AttributeDocumentation;
import org.wildfly.swarm.config.runtime.ResourceDocumentation;
import org.wildfly.swarm.config.runtime.SingletonResource;
import org.wildfly.swarm.config.runtime.Address;
import org.wildfly.swarm.config.runtime.ResourceType;
import java.beans.PropertyChangeSupport;
import java.beans.PropertyChangeListener;
import org.wildfly.swarm.config.runtime.ModelNodeBinding;
/**
* a CMR resource (i.e. a local resource that can reliably participate in an XA
* transaction)
*/
@Address("/subsystem=transactions/commit-markable-resource=*")
@ResourceType("commit-markable-resource")
public class CommitMarkableResource>
implements
org.wildfly.swarm.config.runtime.Keyed {
private String key;
private PropertyChangeSupport pcs;
@AttributeDocumentation("Batch size for this CMR resource")
private Integer batchSize;
@AttributeDocumentation("Immediate cleanup associated to this CMR resource")
private Boolean immediateCleanup;
@AttributeDocumentation("JNDi name of this CMR resource")
private String jndiName;
@AttributeDocumentation("table name for storing XIDs")
private String name;
public CommitMarkableResource(java.lang.String key) {
super();
this.key = key;
}
public String getKey() {
return this.key;
}
/**
* Adds a property change listener
*/
public void addPropertyChangeListener(PropertyChangeListener listener) {
if (null == this.pcs)
this.pcs = new PropertyChangeSupport(this);
this.pcs.addPropertyChangeListener(listener);
}
/**
* Removes a property change listener
*/
public void removePropertyChangeListener(
java.beans.PropertyChangeListener listener) {
if (this.pcs != null)
this.pcs.removePropertyChangeListener(listener);
}
/**
* Batch size for this CMR resource
*/
@ModelNodeBinding(detypedName = "batch-size")
public Integer batchSize() {
return this.batchSize;
}
/**
* Batch size for this CMR resource
*/
@SuppressWarnings("unchecked")
public T batchSize(java.lang.Integer value) {
Object oldValue = this.batchSize;
this.batchSize = value;
if (this.pcs != null)
this.pcs.firePropertyChange("batchSize", oldValue, value);
return (T) this;
}
/**
* Immediate cleanup associated to this CMR resource
*/
@ModelNodeBinding(detypedName = "immediate-cleanup")
public Boolean immediateCleanup() {
return this.immediateCleanup;
}
/**
* Immediate cleanup associated to this CMR resource
*/
@SuppressWarnings("unchecked")
public T immediateCleanup(java.lang.Boolean value) {
Object oldValue = this.immediateCleanup;
this.immediateCleanup = value;
if (this.pcs != null)
this.pcs.firePropertyChange("immediateCleanup", oldValue, value);
return (T) this;
}
/**
* JNDi name of this CMR resource
*/
@ModelNodeBinding(detypedName = "jndi-name")
public String jndiName() {
return this.jndiName;
}
/**
* JNDi name of this CMR resource
*/
@SuppressWarnings("unchecked")
public T jndiName(java.lang.String value) {
Object oldValue = this.jndiName;
this.jndiName = value;
if (this.pcs != null)
this.pcs.firePropertyChange("jndiName", oldValue, value);
return (T) this;
}
/**
* table name for storing XIDs
*/
@ModelNodeBinding(detypedName = "name")
public String name() {
return this.name;
}
/**
* table name for storing XIDs
*/
@SuppressWarnings("unchecked")
public T name(java.lang.String value) {
Object oldValue = this.name;
this.name = value;
if (this.pcs != null)
this.pcs.firePropertyChange("name", oldValue, value);
return (T) this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy