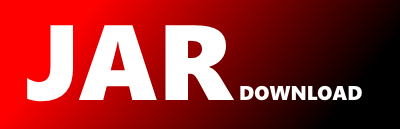
org.wildfly.swarm.config.jgroups.Stack Maven / Gradle / Ivy
package org.wildfly.swarm.config.jgroups;
import org.wildfly.swarm.config.runtime.AttributeDocumentation;
import org.wildfly.swarm.config.runtime.ResourceDocumentation;
import org.wildfly.swarm.config.runtime.SingletonResource;
import org.wildfly.swarm.config.runtime.Address;
import org.wildfly.swarm.config.runtime.ResourceType;
import java.beans.PropertyChangeSupport;
import java.beans.PropertyChangeListener;
import java.util.List;
import org.wildfly.swarm.config.runtime.Subresource;
import org.wildfly.swarm.config.jgroups.ProtocolConsumer;
import org.wildfly.swarm.config.jgroups.ProtocolSupplier;
import org.wildfly.swarm.config.jgroups.Protocol;
import org.wildfly.swarm.config.runtime.SubresourceInfo;
import org.wildfly.swarm.config.jgroups.stack.TransportConsumer;
import org.wildfly.swarm.config.jgroups.stack.TransportSupplier;
import org.wildfly.swarm.config.jgroups.stack.Transport;
import org.wildfly.swarm.config.jgroups.stack.relay.RELAY2;
import org.wildfly.swarm.config.jgroups.stack.relay.RELAY2Consumer;
import org.wildfly.swarm.config.jgroups.stack.relay.RELAY2Supplier;
import org.wildfly.swarm.config.runtime.ModelNodeBinding;
/**
* The configuration of a JGroups protocol stack.
*/
@Address("/subsystem=jgroups/stack=*")
@ResourceType("stack")
public class Stack>
implements
org.wildfly.swarm.config.runtime.Keyed {
private String key;
private PropertyChangeSupport pcs;
private StackResources subresources = new StackResources();
@AttributeDocumentation("Indicates whether or not all protocols in the stack will collect statistics by default.")
private Boolean statisticsEnabled;
public Stack(java.lang.String key) {
super();
this.key = key;
}
public String getKey() {
return this.key;
}
/**
* Adds a property change listener
*/
public void addPropertyChangeListener(PropertyChangeListener listener) {
if (null == this.pcs)
this.pcs = new PropertyChangeSupport(this);
this.pcs.addPropertyChangeListener(listener);
}
/**
* Removes a property change listener
*/
public void removePropertyChangeListener(
java.beans.PropertyChangeListener listener) {
if (this.pcs != null)
this.pcs.removePropertyChangeListener(listener);
}
public StackResources subresources() {
return this.subresources;
}
/**
* Add all Protocol objects to this subresource
*
* @return this
* @param value
* List of Protocol objects.
*/
@SuppressWarnings("unchecked")
public T protocols(java.util.List value) {
this.subresources.protocols = value;
return (T) this;
}
/**
* Add the Protocol object to the list of subresources
*
* @param value
* The Protocol to add
* @return this
*/
@SuppressWarnings("unchecked")
public T protocol(Protocol value) {
this.subresources.protocols.add(value);
return (T) this;
}
/**
* Create and configure a Protocol object to the list of subresources
*
* @param key
* The key for the Protocol resource
* @param config
* The ProtocolConsumer to use
* @return this
*/
@SuppressWarnings("unchecked")
public T protocol(java.lang.String childKey, ProtocolConsumer consumer) {
Protocol extends Protocol> child = new Protocol<>(childKey);
if (consumer != null) {
consumer.accept(child);
}
protocol(child);
return (T) this;
}
/**
* Create and configure a Protocol object to the list of subresources
*
* @param key
* The key for the Protocol resource
* @return this
*/
@SuppressWarnings("unchecked")
public T protocol(java.lang.String childKey) {
protocol(childKey, null);
return (T) this;
}
/**
* Install a supplied Protocol object to the list of subresources
*/
@SuppressWarnings("unchecked")
public T protocol(ProtocolSupplier supplier) {
protocol(supplier.get());
return (T) this;
}
/**
* Add all Transport objects to this subresource
*
* @return this
* @param value
* List of Transport objects.
*/
@SuppressWarnings("unchecked")
public T transports(java.util.List value) {
this.subresources.transports = value;
return (T) this;
}
/**
* Add the Transport object to the list of subresources
*
* @param value
* The Transport to add
* @return this
*/
@SuppressWarnings("unchecked")
public T transport(Transport value) {
this.subresources.transports.add(value);
return (T) this;
}
/**
* Create and configure a Transport object to the list of subresources
*
* @param key
* The key for the Transport resource
* @param config
* The TransportConsumer to use
* @return this
*/
@SuppressWarnings("unchecked")
public T transport(java.lang.String childKey, TransportConsumer consumer) {
Transport extends Transport> child = new Transport<>(childKey);
if (consumer != null) {
consumer.accept(child);
}
transport(child);
return (T) this;
}
/**
* Create and configure a Transport object to the list of subresources
*
* @param key
* The key for the Transport resource
* @return this
*/
@SuppressWarnings("unchecked")
public T transport(java.lang.String childKey) {
transport(childKey, null);
return (T) this;
}
/**
* Install a supplied Transport object to the list of subresources
*/
@SuppressWarnings("unchecked")
public T transport(TransportSupplier supplier) {
transport(supplier.get());
return (T) this;
}
/**
* The configuration of a RELAY protocol
*/
@SuppressWarnings("unchecked")
public T RELAY2(RELAY2 value) {
this.subresources.RELAY2 = value;
return (T) this;
}
/**
* The configuration of a RELAY protocol
*/
@SuppressWarnings("unchecked")
public T RELAY2(RELAY2Consumer consumer) {
RELAY2 extends RELAY2> child = new RELAY2<>();
if (consumer != null) {
consumer.accept(child);
}
this.subresources.RELAY2 = child;
return (T) this;
}
/**
* The configuration of a RELAY protocol
*/
@SuppressWarnings("unchecked")
public T RELAY2() {
RELAY2 extends RELAY2> child = new RELAY2<>();
this.subresources.RELAY2 = child;
return (T) this;
}
/**
* The configuration of a RELAY protocol
*/
@SuppressWarnings("unchecked")
public T RELAY2(RELAY2Supplier supplier) {
this.subresources.RELAY2 = supplier.get();
return (T) this;
}
/**
* Child mutators for Stack
*/
public static class StackResources {
/**
* The configuration of a protocol within a protocol stack.
*/
@ResourceDocumentation("The configuration of a protocol within a protocol stack.")
@SubresourceInfo("protocol")
private List protocols = new java.util.ArrayList<>();
/**
* The configuration of a transport for a protocol stack.
*/
@ResourceDocumentation("The configuration of a transport for a protocol stack.")
@SubresourceInfo("transport")
private List transports = new java.util.ArrayList<>();
@SingletonResource
@ResourceDocumentation("The configuration of a RELAY protocol")
private RELAY2 RELAY2;
/**
* Get the list of Protocol resources
*
* @return the list of resources
*/
@Subresource
public List protocols() {
return this.protocols;
}
public Protocol protocol(java.lang.String key) {
return this.protocols.stream().filter(e -> e.getKey().equals(key))
.findFirst().orElse(null);
}
/**
* Get the list of Transport resources
*
* @return the list of resources
*/
@Subresource
public List transports() {
return this.transports;
}
public Transport transport(java.lang.String key) {
return this.transports.stream().filter(e -> e.getKey().equals(key))
.findFirst().orElse(null);
}
/**
* The configuration of a RELAY protocol
*/
@Subresource
public RELAY2 RELAY2() {
return this.RELAY2;
}
}
/**
* Indicates whether or not all protocols in the stack will collect
* statistics by default.
*/
@ModelNodeBinding(detypedName = "statistics-enabled")
public Boolean statisticsEnabled() {
return this.statisticsEnabled;
}
/**
* Indicates whether or not all protocols in the stack will collect
* statistics by default.
*/
@SuppressWarnings("unchecked")
public T statisticsEnabled(java.lang.Boolean value) {
Object oldValue = this.statisticsEnabled;
this.statisticsEnabled = value;
if (this.pcs != null)
this.pcs.firePropertyChange("statisticsEnabled", oldValue, value);
return (T) this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy