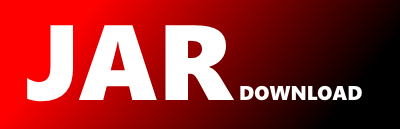
org.wildfly.swarm.config.logging.SocketHandler Maven / Gradle / Ivy
package org.wildfly.swarm.config.logging;
import org.wildfly.swarm.config.runtime.AttributeDocumentation;
import org.wildfly.swarm.config.runtime.ResourceDocumentation;
import org.wildfly.swarm.config.runtime.SingletonResource;
import org.wildfly.swarm.config.runtime.Addresses;
import org.wildfly.swarm.config.runtime.ResourceType;
import java.beans.PropertyChangeSupport;
import java.beans.PropertyChangeListener;
import org.wildfly.swarm.config.runtime.ModelNodeBinding;
import java.util.Arrays;
import org.wildfly.swarm.config.logging.Level;
/**
* Defines a handler which writes to a socket. Note that a socket-handler will
* queue messages during the boot process. These messages will be drained to the
* socket once the resource is fully configured. If the server is in admin-only
* state messages will be discarded.
*/
@Addresses({"/subsystem=logging/socket-handler=*",
"/subsystem=logging/logging-profile=*/socket-handler=*"})
@ResourceType("socket-handler")
public class SocketHandler>
implements
org.wildfly.swarm.config.runtime.Keyed {
private String key;
private PropertyChangeSupport pcs;
@AttributeDocumentation("Automatically flush after each write.")
private Boolean autoflush;
@AttributeDocumentation("If set to true the write methods will block when attempting to reconnect. This is only advisable to be set to true if using an asynchronous handler.")
private Boolean blockOnReconnect;
@AttributeDocumentation("If set to true the handler is enabled and functioning as normal, if set to false the handler is ignored when processing log messages.")
private Boolean enabled;
@AttributeDocumentation("The character encoding used by this Handler.")
private String encoding;
@AttributeDocumentation("A filter expression value to define a filter. Example for a filter that does not match a pattern: not(match(\"JBAS.*\"))")
private String filterSpec;
@AttributeDocumentation("The log level specifying which message levels will be logged by this logger. Message levels lower than this value will be discarded.")
private Level level;
@AttributeDocumentation("The name of the defined formatter to be used on the handler.")
private String namedFormatter;
@AttributeDocumentation("Outbound socket reference for the socket connection.")
private String outboundSocketBindingRef;
@AttributeDocumentation("The protocol the socket should communicate over.")
private Protocol protocol;
@AttributeDocumentation("The reference to the defined SSL context. This is only used if the protocol is set to SSL_TCP.")
private String sslContext;
public SocketHandler(java.lang.String key) {
super();
this.key = key;
}
public String getKey() {
return this.key;
}
/**
* Adds a property change listener
*/
public void addPropertyChangeListener(PropertyChangeListener listener) {
if (null == this.pcs)
this.pcs = new PropertyChangeSupport(this);
this.pcs.addPropertyChangeListener(listener);
}
/**
* Removes a property change listener
*/
public void removePropertyChangeListener(
java.beans.PropertyChangeListener listener) {
if (this.pcs != null)
this.pcs.removePropertyChangeListener(listener);
}
public static enum Protocol {
TCP("TCP"), UDP("UDP"), SSL_TCP("SSL_TCP");
private final String allowedValue;
/**
* Returns the allowed value for the management model.
*
* @return the allowed model value
*/
public String getAllowedValue() {
return allowedValue;
}
Protocol(java.lang.String allowedValue) {
this.allowedValue = allowedValue;
}
@Override
public String toString() {
return allowedValue;
}
}
/**
* Automatically flush after each write.
*/
@ModelNodeBinding(detypedName = "autoflush")
public Boolean autoflush() {
return this.autoflush;
}
/**
* Automatically flush after each write.
*/
@SuppressWarnings("unchecked")
public T autoflush(java.lang.Boolean value) {
Object oldValue = this.autoflush;
this.autoflush = value;
if (this.pcs != null)
this.pcs.firePropertyChange("autoflush", oldValue, value);
return (T) this;
}
/**
* If set to true the write methods will block when attempting to reconnect.
* This is only advisable to be set to true if using an asynchronous
* handler.
*/
@ModelNodeBinding(detypedName = "block-on-reconnect")
public Boolean blockOnReconnect() {
return this.blockOnReconnect;
}
/**
* If set to true the write methods will block when attempting to reconnect.
* This is only advisable to be set to true if using an asynchronous
* handler.
*/
@SuppressWarnings("unchecked")
public T blockOnReconnect(java.lang.Boolean value) {
Object oldValue = this.blockOnReconnect;
this.blockOnReconnect = value;
if (this.pcs != null)
this.pcs.firePropertyChange("blockOnReconnect", oldValue, value);
return (T) this;
}
/**
* If set to true the handler is enabled and functioning as normal, if set
* to false the handler is ignored when processing log messages.
*/
@ModelNodeBinding(detypedName = "enabled")
public Boolean enabled() {
return this.enabled;
}
/**
* If set to true the handler is enabled and functioning as normal, if set
* to false the handler is ignored when processing log messages.
*/
@SuppressWarnings("unchecked")
public T enabled(java.lang.Boolean value) {
Object oldValue = this.enabled;
this.enabled = value;
if (this.pcs != null)
this.pcs.firePropertyChange("enabled", oldValue, value);
return (T) this;
}
/**
* The character encoding used by this Handler.
*/
@ModelNodeBinding(detypedName = "encoding")
public String encoding() {
return this.encoding;
}
/**
* The character encoding used by this Handler.
*/
@SuppressWarnings("unchecked")
public T encoding(java.lang.String value) {
Object oldValue = this.encoding;
this.encoding = value;
if (this.pcs != null)
this.pcs.firePropertyChange("encoding", oldValue, value);
return (T) this;
}
/**
* A filter expression value to define a filter. Example for a filter that
* does not match a pattern: not(match("JBAS.*"))
*/
@ModelNodeBinding(detypedName = "filter-spec")
public String filterSpec() {
return this.filterSpec;
}
/**
* A filter expression value to define a filter. Example for a filter that
* does not match a pattern: not(match("JBAS.*"))
*/
@SuppressWarnings("unchecked")
public T filterSpec(java.lang.String value) {
Object oldValue = this.filterSpec;
this.filterSpec = value;
if (this.pcs != null)
this.pcs.firePropertyChange("filterSpec", oldValue, value);
return (T) this;
}
/**
* The log level specifying which message levels will be logged by this
* logger. Message levels lower than this value will be discarded.
*/
@ModelNodeBinding(detypedName = "level")
public Level level() {
return this.level;
}
/**
* The log level specifying which message levels will be logged by this
* logger. Message levels lower than this value will be discarded.
*/
@SuppressWarnings("unchecked")
public T level(Level value) {
Object oldValue = this.level;
this.level = value;
if (this.pcs != null)
this.pcs.firePropertyChange("level", oldValue, value);
return (T) this;
}
/**
* The name of the defined formatter to be used on the handler.
*/
@ModelNodeBinding(detypedName = "named-formatter")
public String namedFormatter() {
return this.namedFormatter;
}
/**
* The name of the defined formatter to be used on the handler.
*/
@SuppressWarnings("unchecked")
public T namedFormatter(java.lang.String value) {
Object oldValue = this.namedFormatter;
this.namedFormatter = value;
if (this.pcs != null)
this.pcs.firePropertyChange("namedFormatter", oldValue, value);
return (T) this;
}
/**
* Outbound socket reference for the socket connection.
*/
@ModelNodeBinding(detypedName = "outbound-socket-binding-ref")
public String outboundSocketBindingRef() {
return this.outboundSocketBindingRef;
}
/**
* Outbound socket reference for the socket connection.
*/
@SuppressWarnings("unchecked")
public T outboundSocketBindingRef(java.lang.String value) {
Object oldValue = this.outboundSocketBindingRef;
this.outboundSocketBindingRef = value;
if (this.pcs != null)
this.pcs.firePropertyChange("outboundSocketBindingRef", oldValue,
value);
return (T) this;
}
/**
* The protocol the socket should communicate over.
*/
@ModelNodeBinding(detypedName = "protocol")
public Protocol protocol() {
return this.protocol;
}
/**
* The protocol the socket should communicate over.
*/
@SuppressWarnings("unchecked")
public T protocol(Protocol value) {
Object oldValue = this.protocol;
this.protocol = value;
if (this.pcs != null)
this.pcs.firePropertyChange("protocol", oldValue, value);
return (T) this;
}
/**
* The reference to the defined SSL context. This is only used if the
* protocol is set to SSL_TCP.
*/
@ModelNodeBinding(detypedName = "ssl-context")
public String sslContext() {
return this.sslContext;
}
/**
* The reference to the defined SSL context. This is only used if the
* protocol is set to SSL_TCP.
*/
@SuppressWarnings("unchecked")
public T sslContext(java.lang.String value) {
Object oldValue = this.sslContext;
this.sslContext = value;
if (this.pcs != null)
this.pcs.firePropertyChange("sslContext", oldValue, value);
return (T) this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy