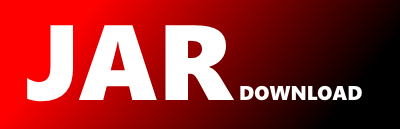
org.wildfly.swarm.config.EE Maven / Gradle / Ivy
package org.wildfly.swarm.config;
import org.wildfly.swarm.config.runtime.AttributeDocumentation;
import org.wildfly.swarm.config.runtime.ResourceDocumentation;
import org.wildfly.swarm.config.runtime.SingletonResource;
import org.wildfly.swarm.config.runtime.Address;
import org.wildfly.swarm.config.runtime.ResourceType;
import org.wildfly.swarm.config.runtime.Implicit;
import java.beans.PropertyChangeSupport;
import java.beans.PropertyChangeListener;
import java.util.List;
import org.wildfly.swarm.config.runtime.Subresource;
import org.wildfly.swarm.config.ee.ManagedThreadFactoryConsumer;
import org.wildfly.swarm.config.ee.ManagedThreadFactorySupplier;
import org.wildfly.swarm.config.ee.ManagedThreadFactory;
import org.wildfly.swarm.config.runtime.SubresourceInfo;
import org.wildfly.swarm.config.ee.ContextServiceConsumer;
import org.wildfly.swarm.config.ee.ContextServiceSupplier;
import org.wildfly.swarm.config.ee.ContextService;
import org.wildfly.swarm.config.ee.ManagedExecutorServiceConsumer;
import org.wildfly.swarm.config.ee.ManagedExecutorServiceSupplier;
import org.wildfly.swarm.config.ee.ManagedExecutorService;
import org.wildfly.swarm.config.ee.ManagedScheduledExecutorServiceConsumer;
import org.wildfly.swarm.config.ee.ManagedScheduledExecutorServiceSupplier;
import org.wildfly.swarm.config.ee.ManagedScheduledExecutorService;
import org.wildfly.swarm.config.ee.DefaultBindingsService;
import org.wildfly.swarm.config.ee.DefaultBindingsServiceConsumer;
import org.wildfly.swarm.config.ee.DefaultBindingsServiceSupplier;
import org.wildfly.swarm.config.runtime.ModelNodeBinding;
import java.util.Map;
import java.util.Arrays;
import java.util.stream.Collectors;
/**
* The configuration of the EE subsystem.
*/
@Address("/subsystem=ee")
@ResourceType("subsystem")
@Implicit
public class EE>
implements
org.wildfly.swarm.config.runtime.Keyed {
private String key;
private PropertyChangeSupport pcs;
private EEResources subresources = new EEResources();
@AttributeDocumentation("Flag indicating whether Jakarta EE annotations will have property replacements applied")
private Boolean annotationPropertyReplacement;
@AttributeDocumentation("Flag indicating whether each of the subdeployments within a .ear can access classes belonging to another subdeployment within the same .ear. A value of false means the subdeployments can see classes belonging to other subdeployments within the .ear.")
private Boolean earSubdeploymentsIsolated;
@AttributeDocumentation("A list of modules that should be made available to all deployments.")
private List globalModules;
@AttributeDocumentation("Flag indicating whether JBoss specific deployment descriptors will have property replacements applied")
private Boolean jbossDescriptorPropertyReplacement;
@AttributeDocumentation("Flag indicating whether descriptors defined by the Jakarta EE specification will have property replacements applied")
private Boolean specDescriptorPropertyReplacement;
public EE() {
super();
this.key = "ee";
this.pcs = new PropertyChangeSupport(this);
}
public String getKey() {
return this.key;
}
/**
* Adds a property change listener
*/
public void addPropertyChangeListener(PropertyChangeListener listener) {
if (null == this.pcs)
this.pcs = new PropertyChangeSupport(this);
this.pcs.addPropertyChangeListener(listener);
}
/**
* Removes a property change listener
*/
public void removePropertyChangeListener(
java.beans.PropertyChangeListener listener) {
if (this.pcs != null)
this.pcs.removePropertyChangeListener(listener);
}
public EEResources subresources() {
return this.subresources;
}
/**
* Add all ManagedThreadFactory objects to this subresource
*
* @return this
* @param value
* List of ManagedThreadFactory objects.
*/
@SuppressWarnings("unchecked")
public T managedThreadFactories(java.util.List value) {
this.subresources.managedThreadFactories = value;
return (T) this;
}
/**
* Add the ManagedThreadFactory object to the list of subresources
*
* @param value
* The ManagedThreadFactory to add
* @return this
*/
@SuppressWarnings("unchecked")
public T managedThreadFactory(ManagedThreadFactory value) {
this.subresources.managedThreadFactories.add(value);
return (T) this;
}
/**
* Create and configure a ManagedThreadFactory object to the list of
* subresources
*
* @param key
* The key for the ManagedThreadFactory resource
* @param config
* The ManagedThreadFactoryConsumer to use
* @return this
*/
@SuppressWarnings("unchecked")
public T managedThreadFactory(java.lang.String childKey,
ManagedThreadFactoryConsumer consumer) {
ManagedThreadFactory extends ManagedThreadFactory> child = new ManagedThreadFactory<>(
childKey);
if (consumer != null) {
consumer.accept(child);
}
managedThreadFactory(child);
return (T) this;
}
/**
* Create and configure a ManagedThreadFactory object to the list of
* subresources
*
* @param key
* The key for the ManagedThreadFactory resource
* @return this
*/
@SuppressWarnings("unchecked")
public T managedThreadFactory(java.lang.String childKey) {
managedThreadFactory(childKey, null);
return (T) this;
}
/**
* Install a supplied ManagedThreadFactory object to the list of
* subresources
*/
@SuppressWarnings("unchecked")
public T managedThreadFactory(ManagedThreadFactorySupplier supplier) {
managedThreadFactory(supplier.get());
return (T) this;
}
/**
* Add all ContextService objects to this subresource
*
* @return this
* @param value
* List of ContextService objects.
*/
@SuppressWarnings("unchecked")
public T contextServices(java.util.List value) {
this.subresources.contextServices = value;
return (T) this;
}
/**
* Add the ContextService object to the list of subresources
*
* @param value
* The ContextService to add
* @return this
*/
@SuppressWarnings("unchecked")
public T contextService(ContextService value) {
this.subresources.contextServices.add(value);
return (T) this;
}
/**
* Create and configure a ContextService object to the list of subresources
*
* @param key
* The key for the ContextService resource
* @param config
* The ContextServiceConsumer to use
* @return this
*/
@SuppressWarnings("unchecked")
public T contextService(java.lang.String childKey,
ContextServiceConsumer consumer) {
ContextService extends ContextService> child = new ContextService<>(
childKey);
if (consumer != null) {
consumer.accept(child);
}
contextService(child);
return (T) this;
}
/**
* Create and configure a ContextService object to the list of subresources
*
* @param key
* The key for the ContextService resource
* @return this
*/
@SuppressWarnings("unchecked")
public T contextService(java.lang.String childKey) {
contextService(childKey, null);
return (T) this;
}
/**
* Install a supplied ContextService object to the list of subresources
*/
@SuppressWarnings("unchecked")
public T contextService(ContextServiceSupplier supplier) {
contextService(supplier.get());
return (T) this;
}
/**
* Add all ManagedExecutorService objects to this subresource
*
* @return this
* @param value
* List of ManagedExecutorService objects.
*/
@SuppressWarnings("unchecked")
public T managedExecutorServices(
java.util.List value) {
this.subresources.managedExecutorServices = value;
return (T) this;
}
/**
* Add the ManagedExecutorService object to the list of subresources
*
* @param value
* The ManagedExecutorService to add
* @return this
*/
@SuppressWarnings("unchecked")
public T managedExecutorService(ManagedExecutorService value) {
this.subresources.managedExecutorServices.add(value);
return (T) this;
}
/**
* Create and configure a ManagedExecutorService object to the list of
* subresources
*
* @param key
* The key for the ManagedExecutorService resource
* @param config
* The ManagedExecutorServiceConsumer to use
* @return this
*/
@SuppressWarnings("unchecked")
public T managedExecutorService(java.lang.String childKey,
ManagedExecutorServiceConsumer consumer) {
ManagedExecutorService extends ManagedExecutorService> child = new ManagedExecutorService<>(
childKey);
if (consumer != null) {
consumer.accept(child);
}
managedExecutorService(child);
return (T) this;
}
/**
* Create and configure a ManagedExecutorService object to the list of
* subresources
*
* @param key
* The key for the ManagedExecutorService resource
* @return this
*/
@SuppressWarnings("unchecked")
public T managedExecutorService(java.lang.String childKey) {
managedExecutorService(childKey, null);
return (T) this;
}
/**
* Install a supplied ManagedExecutorService object to the list of
* subresources
*/
@SuppressWarnings("unchecked")
public T managedExecutorService(ManagedExecutorServiceSupplier supplier) {
managedExecutorService(supplier.get());
return (T) this;
}
/**
* Add all ManagedScheduledExecutorService objects to this subresource
*
* @return this
* @param value
* List of ManagedScheduledExecutorService objects.
*/
@SuppressWarnings("unchecked")
public T managedScheduledExecutorServices(
java.util.List value) {
this.subresources.managedScheduledExecutorServices = value;
return (T) this;
}
/**
* Add the ManagedScheduledExecutorService object to the list of
* subresources
*
* @param value
* The ManagedScheduledExecutorService to add
* @return this
*/
@SuppressWarnings("unchecked")
public T managedScheduledExecutorService(
ManagedScheduledExecutorService value) {
this.subresources.managedScheduledExecutorServices.add(value);
return (T) this;
}
/**
* Create and configure a ManagedScheduledExecutorService object to the list
* of subresources
*
* @param key
* The key for the ManagedScheduledExecutorService resource
* @param config
* The ManagedScheduledExecutorServiceConsumer to use
* @return this
*/
@SuppressWarnings("unchecked")
public T managedScheduledExecutorService(java.lang.String childKey,
ManagedScheduledExecutorServiceConsumer consumer) {
ManagedScheduledExecutorService extends ManagedScheduledExecutorService> child = new ManagedScheduledExecutorService<>(
childKey);
if (consumer != null) {
consumer.accept(child);
}
managedScheduledExecutorService(child);
return (T) this;
}
/**
* Create and configure a ManagedScheduledExecutorService object to the list
* of subresources
*
* @param key
* The key for the ManagedScheduledExecutorService resource
* @return this
*/
@SuppressWarnings("unchecked")
public T managedScheduledExecutorService(java.lang.String childKey) {
managedScheduledExecutorService(childKey, null);
return (T) this;
}
/**
* Install a supplied ManagedScheduledExecutorService object to the list of
* subresources
*/
@SuppressWarnings("unchecked")
public T managedScheduledExecutorService(
ManagedScheduledExecutorServiceSupplier supplier) {
managedScheduledExecutorService(supplier.get());
return (T) this;
}
/**
* The JNDI names for the default EE bindings
*/
@SuppressWarnings("unchecked")
public T defaultBindingsService(DefaultBindingsService value) {
this.subresources.defaultBindingsService = value;
return (T) this;
}
/**
* The JNDI names for the default EE bindings
*/
@SuppressWarnings("unchecked")
public T defaultBindingsService(DefaultBindingsServiceConsumer consumer) {
DefaultBindingsService extends DefaultBindingsService> child = new DefaultBindingsService<>();
if (consumer != null) {
consumer.accept(child);
}
this.subresources.defaultBindingsService = child;
return (T) this;
}
/**
* The JNDI names for the default EE bindings
*/
@SuppressWarnings("unchecked")
public T defaultBindingsService() {
DefaultBindingsService extends DefaultBindingsService> child = new DefaultBindingsService<>();
this.subresources.defaultBindingsService = child;
return (T) this;
}
/**
* The JNDI names for the default EE bindings
*/
@SuppressWarnings("unchecked")
public T defaultBindingsService(DefaultBindingsServiceSupplier supplier) {
this.subresources.defaultBindingsService = supplier.get();
return (T) this;
}
/**
* Child mutators for EE
*/
public static class EEResources {
/**
* A managed thread factory
*/
@ResourceDocumentation("A managed thread factory")
@SubresourceInfo("managedThreadFactory")
private List managedThreadFactories = new java.util.ArrayList<>();
/**
* A context service
*/
@ResourceDocumentation("A context service")
@SubresourceInfo("contextService")
private List contextServices = new java.util.ArrayList<>();
/**
* A managed executor service
*/
@ResourceDocumentation("A managed executor service")
@SubresourceInfo("managedExecutorService")
private List managedExecutorServices = new java.util.ArrayList<>();
/**
* A managed scheduled executor service
*/
@ResourceDocumentation("A managed scheduled executor service")
@SubresourceInfo("managedScheduledExecutorService")
private List managedScheduledExecutorServices = new java.util.ArrayList<>();
@SingletonResource
@ResourceDocumentation("The JNDI names for the default EE bindings")
private DefaultBindingsService defaultBindingsService;
/**
* Get the list of ManagedThreadFactory resources
*
* @return the list of resources
*/
@Subresource
public List managedThreadFactories() {
return this.managedThreadFactories;
}
public ManagedThreadFactory managedThreadFactory(java.lang.String key) {
return this.managedThreadFactories.stream()
.filter(e -> e.getKey().equals(key)).findFirst()
.orElse(null);
}
/**
* Get the list of ContextService resources
*
* @return the list of resources
*/
@Subresource
public List contextServices() {
return this.contextServices;
}
public ContextService contextService(java.lang.String key) {
return this.contextServices.stream()
.filter(e -> e.getKey().equals(key)).findFirst()
.orElse(null);
}
/**
* Get the list of ManagedExecutorService resources
*
* @return the list of resources
*/
@Subresource
public List managedExecutorServices() {
return this.managedExecutorServices;
}
public ManagedExecutorService managedExecutorService(
java.lang.String key) {
return this.managedExecutorServices.stream()
.filter(e -> e.getKey().equals(key)).findFirst()
.orElse(null);
}
/**
* Get the list of ManagedScheduledExecutorService resources
*
* @return the list of resources
*/
@Subresource
public List managedScheduledExecutorServices() {
return this.managedScheduledExecutorServices;
}
public ManagedScheduledExecutorService managedScheduledExecutorService(
java.lang.String key) {
return this.managedScheduledExecutorServices.stream()
.filter(e -> e.getKey().equals(key)).findFirst()
.orElse(null);
}
/**
* The JNDI names for the default EE bindings
*/
@Subresource
public DefaultBindingsService defaultBindingsService() {
return this.defaultBindingsService;
}
}
/**
* Flag indicating whether Jakarta EE annotations will have property
* replacements applied
*/
@ModelNodeBinding(detypedName = "annotation-property-replacement")
public Boolean annotationPropertyReplacement() {
return this.annotationPropertyReplacement;
}
/**
* Flag indicating whether Jakarta EE annotations will have property
* replacements applied
*/
@SuppressWarnings("unchecked")
public T annotationPropertyReplacement(java.lang.Boolean value) {
Object oldValue = this.annotationPropertyReplacement;
this.annotationPropertyReplacement = value;
if (this.pcs != null)
this.pcs.firePropertyChange("annotationPropertyReplacement",
oldValue, value);
return (T) this;
}
/**
* Flag indicating whether each of the subdeployments within a .ear can
* access classes belonging to another subdeployment within the same .ear. A
* value of false means the subdeployments can see classes belonging to
* other subdeployments within the .ear.
*/
@ModelNodeBinding(detypedName = "ear-subdeployments-isolated")
public Boolean earSubdeploymentsIsolated() {
return this.earSubdeploymentsIsolated;
}
/**
* Flag indicating whether each of the subdeployments within a .ear can
* access classes belonging to another subdeployment within the same .ear. A
* value of false means the subdeployments can see classes belonging to
* other subdeployments within the .ear.
*/
@SuppressWarnings("unchecked")
public T earSubdeploymentsIsolated(java.lang.Boolean value) {
Object oldValue = this.earSubdeploymentsIsolated;
this.earSubdeploymentsIsolated = value;
if (this.pcs != null)
this.pcs.firePropertyChange("earSubdeploymentsIsolated", oldValue,
value);
return (T) this;
}
/**
* A list of modules that should be made available to all deployments.
*/
@ModelNodeBinding(detypedName = "global-modules")
public List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy