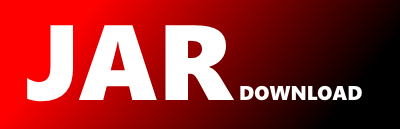
org.wildfly.swarm.config.IO Maven / Gradle / Ivy
package org.wildfly.swarm.config;
import org.wildfly.swarm.config.runtime.AttributeDocumentation;
import org.wildfly.swarm.config.runtime.ResourceDocumentation;
import org.wildfly.swarm.config.runtime.SingletonResource;
import org.wildfly.swarm.config.runtime.Address;
import org.wildfly.swarm.config.runtime.ResourceType;
import org.wildfly.swarm.config.runtime.Implicit;
import java.beans.PropertyChangeSupport;
import java.beans.PropertyChangeListener;
import java.util.List;
import org.wildfly.swarm.config.runtime.Subresource;
import org.wildfly.swarm.config.io.WorkerConsumer;
import org.wildfly.swarm.config.io.WorkerSupplier;
import org.wildfly.swarm.config.io.Worker;
import org.wildfly.swarm.config.runtime.SubresourceInfo;
import org.wildfly.swarm.config.io.BufferPoolConsumer;
import org.wildfly.swarm.config.io.BufferPoolSupplier;
import org.wildfly.swarm.config.io.BufferPool;
import org.wildfly.swarm.config.runtime.ModelNodeBinding;
/**
* IO subsystem
*/
@Address("/subsystem=io")
@ResourceType("subsystem")
@Implicit
public class IO>
implements
org.wildfly.swarm.config.runtime.Keyed {
private String key;
private PropertyChangeSupport pcs;
private IOResources subresources = new IOResources();
public IO() {
super();
this.key = "io";
this.pcs = new PropertyChangeSupport(this);
}
public String getKey() {
return this.key;
}
/**
* Adds a property change listener
*/
public void addPropertyChangeListener(PropertyChangeListener listener) {
if (null == this.pcs)
this.pcs = new PropertyChangeSupport(this);
this.pcs.addPropertyChangeListener(listener);
}
/**
* Removes a property change listener
*/
public void removePropertyChangeListener(
java.beans.PropertyChangeListener listener) {
if (this.pcs != null)
this.pcs.removePropertyChangeListener(listener);
}
public IOResources subresources() {
return this.subresources;
}
/**
* Add all Worker objects to this subresource
*
* @return this
* @param value
* List of Worker objects.
*/
@SuppressWarnings("unchecked")
public T workers(java.util.List value) {
this.subresources.workers = value;
return (T) this;
}
/**
* Add the Worker object to the list of subresources
*
* @param value
* The Worker to add
* @return this
*/
@SuppressWarnings("unchecked")
public T worker(Worker value) {
this.subresources.workers.add(value);
return (T) this;
}
/**
* Create and configure a Worker object to the list of subresources
*
* @param key
* The key for the Worker resource
* @param config
* The WorkerConsumer to use
* @return this
*/
@SuppressWarnings("unchecked")
public T worker(java.lang.String childKey, WorkerConsumer consumer) {
Worker extends Worker> child = new Worker<>(childKey);
if (consumer != null) {
consumer.accept(child);
}
worker(child);
return (T) this;
}
/**
* Create and configure a Worker object to the list of subresources
*
* @param key
* The key for the Worker resource
* @return this
*/
@SuppressWarnings("unchecked")
public T worker(java.lang.String childKey) {
worker(childKey, null);
return (T) this;
}
/**
* Install a supplied Worker object to the list of subresources
*/
@SuppressWarnings("unchecked")
public T worker(WorkerSupplier supplier) {
worker(supplier.get());
return (T) this;
}
/**
* Add all BufferPool objects to this subresource
*
* @return this
* @param value
* List of BufferPool objects.
*/
@SuppressWarnings("unchecked")
public T bufferPools(java.util.List value) {
this.subresources.bufferPools = value;
return (T) this;
}
/**
* Add the BufferPool object to the list of subresources
*
* @param value
* The BufferPool to add
* @return this
*/
@SuppressWarnings("unchecked")
public T bufferPool(BufferPool value) {
this.subresources.bufferPools.add(value);
return (T) this;
}
/**
* Create and configure a BufferPool object to the list of subresources
*
* @param key
* The key for the BufferPool resource
* @param config
* The BufferPoolConsumer to use
* @return this
*/
@SuppressWarnings("unchecked")
public T bufferPool(java.lang.String childKey, BufferPoolConsumer consumer) {
BufferPool extends BufferPool> child = new BufferPool<>(childKey);
if (consumer != null) {
consumer.accept(child);
}
bufferPool(child);
return (T) this;
}
/**
* Create and configure a BufferPool object to the list of subresources
*
* @param key
* The key for the BufferPool resource
* @return this
*/
@SuppressWarnings("unchecked")
public T bufferPool(java.lang.String childKey) {
bufferPool(childKey, null);
return (T) this;
}
/**
* Install a supplied BufferPool object to the list of subresources
*/
@SuppressWarnings("unchecked")
public T bufferPool(BufferPoolSupplier supplier) {
bufferPool(supplier.get());
return (T) this;
}
/**
* Child mutators for IO
*/
public static class IOResources {
/**
* Defines workers
*/
@ResourceDocumentation("Defines workers")
@SubresourceInfo("worker")
private List workers = new java.util.ArrayList<>();
/**
* Defines buffer pool
*/
@ResourceDocumentation("Defines buffer pool")
@SubresourceInfo("bufferPool")
private List bufferPools = new java.util.ArrayList<>();
/**
* Get the list of Worker resources
*
* @return the list of resources
*/
@Subresource
public List workers() {
return this.workers;
}
public Worker worker(java.lang.String key) {
return this.workers.stream().filter(e -> e.getKey().equals(key))
.findFirst().orElse(null);
}
/**
* Get the list of BufferPool resources
*
* @return the list of resources
*/
@Subresource
public List bufferPools() {
return this.bufferPools;
}
public BufferPool bufferPool(java.lang.String key) {
return this.bufferPools.stream()
.filter(e -> e.getKey().equals(key)).findFirst()
.orElse(null);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy