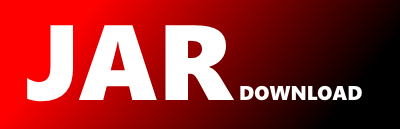
org.wildfly.swarm.config.JGroups Maven / Gradle / Ivy
package org.wildfly.swarm.config;
import org.wildfly.swarm.config.runtime.AttributeDocumentation;
import org.wildfly.swarm.config.runtime.ResourceDocumentation;
import org.wildfly.swarm.config.runtime.SingletonResource;
import org.wildfly.swarm.config.runtime.Address;
import org.wildfly.swarm.config.runtime.ResourceType;
import org.wildfly.swarm.config.runtime.Implicit;
import java.beans.PropertyChangeSupport;
import java.beans.PropertyChangeListener;
import java.util.List;
import org.wildfly.swarm.config.runtime.Subresource;
import org.wildfly.swarm.config.jgroups.StackConsumer;
import org.wildfly.swarm.config.jgroups.StackSupplier;
import org.wildfly.swarm.config.jgroups.Stack;
import org.wildfly.swarm.config.runtime.SubresourceInfo;
import org.wildfly.swarm.config.jgroups.ChannelConsumer;
import org.wildfly.swarm.config.jgroups.ChannelSupplier;
import org.wildfly.swarm.config.jgroups.Channel;
import org.wildfly.swarm.config.runtime.ModelNodeBinding;
/**
* The configuration of the JGroups subsystem.
*/
@Address("/subsystem=jgroups")
@ResourceType("subsystem")
@Implicit
public class JGroups>
implements
org.wildfly.swarm.config.runtime.Keyed {
private String key;
private PropertyChangeSupport pcs;
private JGroupsResources subresources = new JGroupsResources();
@AttributeDocumentation("The default JGroups channel.")
private String defaultChannel;
@AttributeDocumentation("The default JGroups protocol stack.")
private String defaultStack;
public JGroups() {
super();
this.key = "jgroups";
this.pcs = new PropertyChangeSupport(this);
}
public String getKey() {
return this.key;
}
/**
* Adds a property change listener
*/
public void addPropertyChangeListener(PropertyChangeListener listener) {
if (null == this.pcs)
this.pcs = new PropertyChangeSupport(this);
this.pcs.addPropertyChangeListener(listener);
}
/**
* Removes a property change listener
*/
public void removePropertyChangeListener(
java.beans.PropertyChangeListener listener) {
if (this.pcs != null)
this.pcs.removePropertyChangeListener(listener);
}
public JGroupsResources subresources() {
return this.subresources;
}
/**
* Add all Stack objects to this subresource
*
* @return this
* @param value
* List of Stack objects.
*/
@SuppressWarnings("unchecked")
public T stacks(java.util.List value) {
this.subresources.stacks = value;
return (T) this;
}
/**
* Add the Stack object to the list of subresources
*
* @param value
* The Stack to add
* @return this
*/
@SuppressWarnings("unchecked")
public T stack(Stack value) {
this.subresources.stacks.add(value);
return (T) this;
}
/**
* Create and configure a Stack object to the list of subresources
*
* @param key
* The key for the Stack resource
* @param config
* The StackConsumer to use
* @return this
*/
@SuppressWarnings("unchecked")
public T stack(java.lang.String childKey, StackConsumer consumer) {
Stack extends Stack> child = new Stack<>(childKey);
if (consumer != null) {
consumer.accept(child);
}
stack(child);
return (T) this;
}
/**
* Create and configure a Stack object to the list of subresources
*
* @param key
* The key for the Stack resource
* @return this
*/
@SuppressWarnings("unchecked")
public T stack(java.lang.String childKey) {
stack(childKey, null);
return (T) this;
}
/**
* Install a supplied Stack object to the list of subresources
*/
@SuppressWarnings("unchecked")
public T stack(StackSupplier supplier) {
stack(supplier.get());
return (T) this;
}
/**
* Add all Channel objects to this subresource
*
* @return this
* @param value
* List of Channel objects.
*/
@SuppressWarnings("unchecked")
public T channels(java.util.List value) {
this.subresources.channels = value;
return (T) this;
}
/**
* Add the Channel object to the list of subresources
*
* @param value
* The Channel to add
* @return this
*/
@SuppressWarnings("unchecked")
public T channel(Channel value) {
this.subresources.channels.add(value);
return (T) this;
}
/**
* Create and configure a Channel object to the list of subresources
*
* @param key
* The key for the Channel resource
* @param config
* The ChannelConsumer to use
* @return this
*/
@SuppressWarnings("unchecked")
public T channel(java.lang.String childKey, ChannelConsumer consumer) {
Channel extends Channel> child = new Channel<>(childKey);
if (consumer != null) {
consumer.accept(child);
}
channel(child);
return (T) this;
}
/**
* Create and configure a Channel object to the list of subresources
*
* @param key
* The key for the Channel resource
* @return this
*/
@SuppressWarnings("unchecked")
public T channel(java.lang.String childKey) {
channel(childKey, null);
return (T) this;
}
/**
* Install a supplied Channel object to the list of subresources
*/
@SuppressWarnings("unchecked")
public T channel(ChannelSupplier supplier) {
channel(supplier.get());
return (T) this;
}
/**
* Child mutators for JGroups
*/
public static class JGroupsResources {
/**
* The configuration of a JGroups protocol stack.
*/
@ResourceDocumentation("The configuration of a JGroups protocol stack.")
@SubresourceInfo("stack")
private List stacks = new java.util.ArrayList<>();
/**
* A JGroups channel.
*/
@ResourceDocumentation("A JGroups channel.")
@SubresourceInfo("channel")
private List channels = new java.util.ArrayList<>();
/**
* Get the list of Stack resources
*
* @return the list of resources
*/
@Subresource
public List stacks() {
return this.stacks;
}
public Stack stack(java.lang.String key) {
return this.stacks.stream().filter(e -> e.getKey().equals(key))
.findFirst().orElse(null);
}
/**
* Get the list of Channel resources
*
* @return the list of resources
*/
@Subresource
public List channels() {
return this.channels;
}
public Channel channel(java.lang.String key) {
return this.channels.stream().filter(e -> e.getKey().equals(key))
.findFirst().orElse(null);
}
}
/**
* The default JGroups channel.
*/
@ModelNodeBinding(detypedName = "default-channel")
public String defaultChannel() {
return this.defaultChannel;
}
/**
* The default JGroups channel.
*/
@SuppressWarnings("unchecked")
public T defaultChannel(java.lang.String value) {
Object oldValue = this.defaultChannel;
this.defaultChannel = value;
if (this.pcs != null)
this.pcs.firePropertyChange("defaultChannel", oldValue, value);
return (T) this;
}
/**
* The default JGroups protocol stack.
*
* @deprecated Deprecated. This attribute will be removed in a future
* release.
*/
@Deprecated
@ModelNodeBinding(detypedName = "default-stack")
public String defaultStack() {
return this.defaultStack;
}
/**
* The default JGroups protocol stack.
*
* @deprecated Deprecated. This attribute will be removed in a future
* release.
*/
@SuppressWarnings("unchecked")
@Deprecated
public T defaultStack(java.lang.String value) {
Object oldValue = this.defaultStack;
this.defaultStack = value;
if (this.pcs != null)
this.pcs.firePropertyChange("defaultStack", oldValue, value);
return (T) this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy