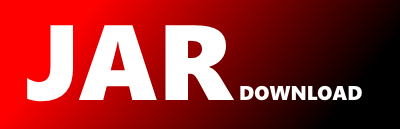
org.wildfly.swarm.config.jgroups.Channel Maven / Gradle / Ivy
package org.wildfly.swarm.config.jgroups;
import org.wildfly.swarm.config.runtime.AttributeDocumentation;
import org.wildfly.swarm.config.runtime.ResourceDocumentation;
import org.wildfly.swarm.config.runtime.SingletonResource;
import org.wildfly.swarm.config.runtime.Address;
import org.wildfly.swarm.config.runtime.ResourceType;
import java.beans.PropertyChangeSupport;
import java.beans.PropertyChangeListener;
import java.util.List;
import org.wildfly.swarm.config.runtime.Subresource;
import org.wildfly.swarm.config.jgroups.channel.ForkConsumer;
import org.wildfly.swarm.config.jgroups.channel.ForkSupplier;
import org.wildfly.swarm.config.jgroups.channel.Fork;
import org.wildfly.swarm.config.runtime.SubresourceInfo;
import org.wildfly.swarm.config.runtime.ModelNodeBinding;
/**
* A JGroups channel.
*/
@Address("/subsystem=jgroups/channel=*")
@ResourceType("channel")
public class Channel>
implements
org.wildfly.swarm.config.runtime.Keyed {
private String key;
private PropertyChangeSupport pcs;
private ChannelResources subresources = new ChannelResources();
@AttributeDocumentation("The IP address of the channel.")
private String address;
@AttributeDocumentation("The address of the channel as a UUID.")
private String addressAsUuid;
@AttributeDocumentation("The cluster name of the JGroups channel. If undefined, the name of the channel will be used.")
private String cluster;
@AttributeDocumentation("If true, do not receive messages sent by this node (ourself).")
private Boolean discardOwnMessages;
@AttributeDocumentation("The module from which to load channel services")
private String module;
@AttributeDocumentation("The current number of timer tasks.")
private Integer numTasksInTimer;
@AttributeDocumentation("The number of timer threads.")
private Integer numTimerThreads;
@AttributeDocumentation("The number of bytes received by this channel.")
private Long receivedBytes;
@AttributeDocumentation("The number of messages received by this channel.")
private Long receivedMessages;
@AttributeDocumentation("The number of bytes sent by this channel.")
private Long sentBytes;
@AttributeDocumentation("The number of messages sent by this channel.")
private Long sentMessages;
@AttributeDocumentation("The protocol stack of the JGroups channel")
private String stack;
@AttributeDocumentation("The state of the channel (OPEN, CONNECTING, CONNECTED, CLOSED).")
private String state;
@AttributeDocumentation("If enabled, collect channel statistics.")
private Boolean statisticsEnabled;
@AttributeDocumentation("If enabled, collect channel statistics.")
private Boolean statsEnabled;
@AttributeDocumentation("The JGroups software version.")
private String version;
@AttributeDocumentation("The channel's view of group membership.")
private String view;
public Channel(java.lang.String key) {
super();
this.key = key;
}
public String getKey() {
return this.key;
}
/**
* Adds a property change listener
*/
public void addPropertyChangeListener(PropertyChangeListener listener) {
if (null == this.pcs)
this.pcs = new PropertyChangeSupport(this);
this.pcs.addPropertyChangeListener(listener);
}
/**
* Removes a property change listener
*/
public void removePropertyChangeListener(
java.beans.PropertyChangeListener listener) {
if (this.pcs != null)
this.pcs.removePropertyChangeListener(listener);
}
public ChannelResources subresources() {
return this.subresources;
}
/**
* Add all Fork objects to this subresource
*
* @return this
* @param value
* List of Fork objects.
*/
@SuppressWarnings("unchecked")
public T forks(java.util.List value) {
this.subresources.forks = value;
return (T) this;
}
/**
* Add the Fork object to the list of subresources
*
* @param value
* The Fork to add
* @return this
*/
@SuppressWarnings("unchecked")
public T fork(Fork value) {
this.subresources.forks.add(value);
return (T) this;
}
/**
* Create and configure a Fork object to the list of subresources
*
* @param key
* The key for the Fork resource
* @param config
* The ForkConsumer to use
* @return this
*/
@SuppressWarnings("unchecked")
public T fork(java.lang.String childKey, ForkConsumer consumer) {
Fork extends Fork> child = new Fork<>(childKey);
if (consumer != null) {
consumer.accept(child);
}
fork(child);
return (T) this;
}
/**
* Create and configure a Fork object to the list of subresources
*
* @param key
* The key for the Fork resource
* @return this
*/
@SuppressWarnings("unchecked")
public T fork(java.lang.String childKey) {
fork(childKey, null);
return (T) this;
}
/**
* Install a supplied Fork object to the list of subresources
*/
@SuppressWarnings("unchecked")
public T fork(ForkSupplier supplier) {
fork(supplier.get());
return (T) this;
}
/**
* Child mutators for Channel
*/
public static class ChannelResources {
/**
* A JGroups channel fork
*/
@ResourceDocumentation("A JGroups channel fork")
@SubresourceInfo("fork")
private List forks = new java.util.ArrayList<>();
/**
* Get the list of Fork resources
*
* @return the list of resources
*/
@Subresource
public List forks() {
return this.forks;
}
public Fork fork(java.lang.String key) {
return this.forks.stream().filter(e -> e.getKey().equals(key))
.findFirst().orElse(null);
}
}
/**
* The IP address of the channel.
*/
@ModelNodeBinding(detypedName = "address")
public String address() {
return this.address;
}
/**
* The IP address of the channel.
*/
@SuppressWarnings("unchecked")
public T address(java.lang.String value) {
Object oldValue = this.address;
this.address = value;
if (this.pcs != null)
this.pcs.firePropertyChange("address", oldValue, value);
return (T) this;
}
/**
* The address of the channel as a UUID.
*/
@ModelNodeBinding(detypedName = "address-as-uuid")
public String addressAsUuid() {
return this.addressAsUuid;
}
/**
* The address of the channel as a UUID.
*/
@SuppressWarnings("unchecked")
public T addressAsUuid(java.lang.String value) {
Object oldValue = this.addressAsUuid;
this.addressAsUuid = value;
if (this.pcs != null)
this.pcs.firePropertyChange("addressAsUuid", oldValue, value);
return (T) this;
}
/**
* The cluster name of the JGroups channel. If undefined, the name of the
* channel will be used.
*/
@ModelNodeBinding(detypedName = "cluster")
public String cluster() {
return this.cluster;
}
/**
* The cluster name of the JGroups channel. If undefined, the name of the
* channel will be used.
*/
@SuppressWarnings("unchecked")
public T cluster(java.lang.String value) {
Object oldValue = this.cluster;
this.cluster = value;
if (this.pcs != null)
this.pcs.firePropertyChange("cluster", oldValue, value);
return (T) this;
}
/**
* If true, do not receive messages sent by this node (ourself).
*/
@ModelNodeBinding(detypedName = "discard-own-messages")
public Boolean discardOwnMessages() {
return this.discardOwnMessages;
}
/**
* If true, do not receive messages sent by this node (ourself).
*/
@SuppressWarnings("unchecked")
public T discardOwnMessages(java.lang.Boolean value) {
Object oldValue = this.discardOwnMessages;
this.discardOwnMessages = value;
if (this.pcs != null)
this.pcs.firePropertyChange("discardOwnMessages", oldValue, value);
return (T) this;
}
/**
* The module from which to load channel services
*/
@ModelNodeBinding(detypedName = "module")
public String module() {
return this.module;
}
/**
* The module from which to load channel services
*/
@SuppressWarnings("unchecked")
public T module(java.lang.String value) {
Object oldValue = this.module;
this.module = value;
if (this.pcs != null)
this.pcs.firePropertyChange("module", oldValue, value);
return (T) this;
}
/**
* The current number of timer tasks.
*
* @deprecated Deprecated.
*/
@Deprecated
@ModelNodeBinding(detypedName = "num-tasks-in-timer")
public Integer numTasksInTimer() {
return this.numTasksInTimer;
}
/**
* The current number of timer tasks.
*
* @deprecated Deprecated.
*/
@SuppressWarnings("unchecked")
@Deprecated
public T numTasksInTimer(java.lang.Integer value) {
Object oldValue = this.numTasksInTimer;
this.numTasksInTimer = value;
if (this.pcs != null)
this.pcs.firePropertyChange("numTasksInTimer", oldValue, value);
return (T) this;
}
/**
* The number of timer threads.
*
* @deprecated Deprecated.
*/
@Deprecated
@ModelNodeBinding(detypedName = "num-timer-threads")
public Integer numTimerThreads() {
return this.numTimerThreads;
}
/**
* The number of timer threads.
*
* @deprecated Deprecated.
*/
@SuppressWarnings("unchecked")
@Deprecated
public T numTimerThreads(java.lang.Integer value) {
Object oldValue = this.numTimerThreads;
this.numTimerThreads = value;
if (this.pcs != null)
this.pcs.firePropertyChange("numTimerThreads", oldValue, value);
return (T) this;
}
/**
* The number of bytes received by this channel.
*/
@ModelNodeBinding(detypedName = "received-bytes")
public Long receivedBytes() {
return this.receivedBytes;
}
/**
* The number of bytes received by this channel.
*/
@SuppressWarnings("unchecked")
public T receivedBytes(java.lang.Long value) {
Object oldValue = this.receivedBytes;
this.receivedBytes = value;
if (this.pcs != null)
this.pcs.firePropertyChange("receivedBytes", oldValue, value);
return (T) this;
}
/**
* The number of messages received by this channel.
*/
@ModelNodeBinding(detypedName = "received-messages")
public Long receivedMessages() {
return this.receivedMessages;
}
/**
* The number of messages received by this channel.
*/
@SuppressWarnings("unchecked")
public T receivedMessages(java.lang.Long value) {
Object oldValue = this.receivedMessages;
this.receivedMessages = value;
if (this.pcs != null)
this.pcs.firePropertyChange("receivedMessages", oldValue, value);
return (T) this;
}
/**
* The number of bytes sent by this channel.
*/
@ModelNodeBinding(detypedName = "sent-bytes")
public Long sentBytes() {
return this.sentBytes;
}
/**
* The number of bytes sent by this channel.
*/
@SuppressWarnings("unchecked")
public T sentBytes(java.lang.Long value) {
Object oldValue = this.sentBytes;
this.sentBytes = value;
if (this.pcs != null)
this.pcs.firePropertyChange("sentBytes", oldValue, value);
return (T) this;
}
/**
* The number of messages sent by this channel.
*/
@ModelNodeBinding(detypedName = "sent-messages")
public Long sentMessages() {
return this.sentMessages;
}
/**
* The number of messages sent by this channel.
*/
@SuppressWarnings("unchecked")
public T sentMessages(java.lang.Long value) {
Object oldValue = this.sentMessages;
this.sentMessages = value;
if (this.pcs != null)
this.pcs.firePropertyChange("sentMessages", oldValue, value);
return (T) this;
}
/**
* The protocol stack of the JGroups channel
*/
@ModelNodeBinding(detypedName = "stack")
public String stack() {
return this.stack;
}
/**
* The protocol stack of the JGroups channel
*/
@SuppressWarnings("unchecked")
public T stack(java.lang.String value) {
Object oldValue = this.stack;
this.stack = value;
if (this.pcs != null)
this.pcs.firePropertyChange("stack", oldValue, value);
return (T) this;
}
/**
* The state of the channel (OPEN, CONNECTING, CONNECTED, CLOSED).
*/
@ModelNodeBinding(detypedName = "state")
public String state() {
return this.state;
}
/**
* The state of the channel (OPEN, CONNECTING, CONNECTED, CLOSED).
*/
@SuppressWarnings("unchecked")
public T state(java.lang.String value) {
Object oldValue = this.state;
this.state = value;
if (this.pcs != null)
this.pcs.firePropertyChange("state", oldValue, value);
return (T) this;
}
/**
* If enabled, collect channel statistics.
*/
@ModelNodeBinding(detypedName = "statistics-enabled")
public Boolean statisticsEnabled() {
return this.statisticsEnabled;
}
/**
* If enabled, collect channel statistics.
*/
@SuppressWarnings("unchecked")
public T statisticsEnabled(java.lang.Boolean value) {
Object oldValue = this.statisticsEnabled;
this.statisticsEnabled = value;
if (this.pcs != null)
this.pcs.firePropertyChange("statisticsEnabled", oldValue, value);
return (T) this;
}
/**
* If enabled, collect channel statistics.
*
* @deprecated Deprecated. Use statistics-enabled instead.
*/
@Deprecated
@ModelNodeBinding(detypedName = "stats-enabled")
public Boolean statsEnabled() {
return this.statsEnabled;
}
/**
* If enabled, collect channel statistics.
*
* @deprecated Deprecated. Use statistics-enabled instead.
*/
@SuppressWarnings("unchecked")
@Deprecated
public T statsEnabled(java.lang.Boolean value) {
Object oldValue = this.statsEnabled;
this.statsEnabled = value;
if (this.pcs != null)
this.pcs.firePropertyChange("statsEnabled", oldValue, value);
return (T) this;
}
/**
* The JGroups software version.
*/
@ModelNodeBinding(detypedName = "version")
public String version() {
return this.version;
}
/**
* The JGroups software version.
*/
@SuppressWarnings("unchecked")
public T version(java.lang.String value) {
Object oldValue = this.version;
this.version = value;
if (this.pcs != null)
this.pcs.firePropertyChange("version", oldValue, value);
return (T) this;
}
/**
* The channel's view of group membership.
*/
@ModelNodeBinding(detypedName = "view")
public String view() {
return this.view;
}
/**
* The channel's view of group membership.
*/
@SuppressWarnings("unchecked")
public T view(java.lang.String value) {
Object oldValue = this.view;
this.view = value;
if (this.pcs != null)
this.pcs.firePropertyChange("view", oldValue, value);
return (T) this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy