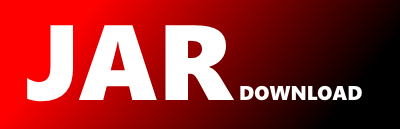
org.wildfly.swarm.config.management.service.ActiveOperation Maven / Gradle / Ivy
package org.wildfly.swarm.config.management.service;
import org.wildfly.swarm.config.runtime.AttributeDocumentation;
import org.wildfly.swarm.config.runtime.ResourceDocumentation;
import org.wildfly.swarm.config.runtime.SingletonResource;
import org.wildfly.swarm.config.runtime.Address;
import org.wildfly.swarm.config.runtime.ResourceType;
import java.beans.PropertyChangeSupport;
import java.beans.PropertyChangeListener;
import org.wildfly.swarm.config.runtime.ModelNodeBinding;
import java.util.Arrays;
import java.util.List;
import org.jboss.dmr.Property;
import java.util.stream.Collectors;
/**
* A currently executing operation.
*/
@Address("/core-service=management/service=management-operations/active-operation=*")
@ResourceType("active-operation")
public class ActiveOperation>
implements
org.wildfly.swarm.config.runtime.Keyed {
private String key;
private PropertyChangeSupport pcs;
@AttributeDocumentation("The mechanism used to submit a request to the server.")
private AccessMechanism accessMechanism;
@AttributeDocumentation("The address of the resource targeted by the operation. The value in the final element of the address will be '' if the caller is not authorized to address the operation's target resource.")
private List address;
@AttributeDocumentation("The name of the thread that is executing the operation.")
private String callerThread;
@AttributeDocumentation("Whether the operation has been cancelled.")
private Boolean cancelled;
@AttributeDocumentation("True if the operation is a subsidiary request on a domain process other than the one directly handling the original operation, executing locally as part of the rollout of the original operation across the domain.")
private Boolean domainRollout;
@AttributeDocumentation("Identifier of an overall multi-process domain operation of which this operation is a part, or undefined if this operation is not associated with such a domain operation.")
private String domainUuid;
@AttributeDocumentation("Amount of time the operation has been executing with the exclusive operation execution lock held, or -1 if the operation does not hold the exclusive execution lock.")
private Long exclusiveRunningTime;
@AttributeDocumentation("The current activity of the operation.")
private ExecutionStatus executionStatus;
@AttributeDocumentation("The name of the operation, or '' if the caller is not authorized to address the operation's target resource.")
private String operation;
@AttributeDocumentation("Amount of time the operation has been executing.")
private Long runningTime;
public ActiveOperation(java.lang.String key) {
super();
this.key = key;
}
public String getKey() {
return this.key;
}
/**
* Adds a property change listener
*/
public void addPropertyChangeListener(PropertyChangeListener listener) {
if (null == this.pcs)
this.pcs = new PropertyChangeSupport(this);
this.pcs.addPropertyChangeListener(listener);
}
/**
* Removes a property change listener
*/
public void removePropertyChangeListener(
java.beans.PropertyChangeListener listener) {
if (this.pcs != null)
this.pcs.removePropertyChangeListener(listener);
}
public static enum AccessMechanism {
NATIVE("NATIVE"), HTTP("HTTP"), JMX("JMX"), IN_VM_USER("IN_VM_USER");
private final String allowedValue;
/**
* Returns the allowed value for the management model.
*
* @return the allowed model value
*/
public String getAllowedValue() {
return allowedValue;
}
AccessMechanism(java.lang.String allowedValue) {
this.allowedValue = allowedValue;
}
@Override
public String toString() {
return allowedValue;
}
}
public static enum ExecutionStatus {
EXECUTING("executing"), AWAITING_OTHER_OPERATION(
"awaiting-other-operation"), AWAITING_STABILITY(
"awaiting-stability"), COMPLETING("completing"), ROLLING_BACK(
"rolling-back");
private final String allowedValue;
/**
* Returns the allowed value for the management model.
*
* @return the allowed model value
*/
public String getAllowedValue() {
return allowedValue;
}
ExecutionStatus(java.lang.String allowedValue) {
this.allowedValue = allowedValue;
}
@Override
public String toString() {
return allowedValue;
}
}
/**
* The mechanism used to submit a request to the server.
*/
@ModelNodeBinding(detypedName = "access-mechanism")
public AccessMechanism accessMechanism() {
return this.accessMechanism;
}
/**
* The mechanism used to submit a request to the server.
*/
@SuppressWarnings("unchecked")
public T accessMechanism(AccessMechanism value) {
Object oldValue = this.accessMechanism;
this.accessMechanism = value;
if (this.pcs != null)
this.pcs.firePropertyChange("accessMechanism", oldValue, value);
return (T) this;
}
/**
* The address of the resource targeted by the operation. The value in the
* final element of the address will be '' if the caller is not
* authorized to address the operation's target resource.
*/
@ModelNodeBinding(detypedName = "address")
public List address() {
return this.address;
}
/**
* The address of the resource targeted by the operation. The value in the
* final element of the address will be '' if the caller is not
* authorized to address the operation's target resource.
*/
@SuppressWarnings("unchecked")
public T address(java.util.List value) {
Object oldValue = this.address;
this.address = value;
if (this.pcs != null)
this.pcs.firePropertyChange("address", oldValue, value);
return (T) this;
}
/**
* The address of the resource targeted by the operation. The value in the
* final element of the address will be '' if the caller is not
* authorized to address the operation's target resource.
*/
@SuppressWarnings("unchecked")
public T address(org.jboss.dmr.Property value) {
if (this.address == null) {
this.address = new java.util.ArrayList<>();
}
this.address.add(value);
return (T) this;
}
/**
* The address of the resource targeted by the operation. The value in the
* final element of the address will be '' if the caller is not
* authorized to address the operation's target resource.
*/
@SuppressWarnings("unchecked")
public T address(org.jboss.dmr.Property... args) {
address(Arrays.stream(args).collect(Collectors.toList()));
return (T) this;
}
/**
* The name of the thread that is executing the operation.
*/
@ModelNodeBinding(detypedName = "caller-thread")
public String callerThread() {
return this.callerThread;
}
/**
* The name of the thread that is executing the operation.
*/
@SuppressWarnings("unchecked")
public T callerThread(java.lang.String value) {
Object oldValue = this.callerThread;
this.callerThread = value;
if (this.pcs != null)
this.pcs.firePropertyChange("callerThread", oldValue, value);
return (T) this;
}
/**
* Whether the operation has been cancelled.
*/
@ModelNodeBinding(detypedName = "cancelled")
public Boolean cancelled() {
return this.cancelled;
}
/**
* Whether the operation has been cancelled.
*/
@SuppressWarnings("unchecked")
public T cancelled(java.lang.Boolean value) {
Object oldValue = this.cancelled;
this.cancelled = value;
if (this.pcs != null)
this.pcs.firePropertyChange("cancelled", oldValue, value);
return (T) this;
}
/**
* True if the operation is a subsidiary request on a domain process other
* than the one directly handling the original operation, executing locally
* as part of the rollout of the original operation across the domain.
*/
@ModelNodeBinding(detypedName = "domain-rollout")
public Boolean domainRollout() {
return this.domainRollout;
}
/**
* True if the operation is a subsidiary request on a domain process other
* than the one directly handling the original operation, executing locally
* as part of the rollout of the original operation across the domain.
*/
@SuppressWarnings("unchecked")
public T domainRollout(java.lang.Boolean value) {
Object oldValue = this.domainRollout;
this.domainRollout = value;
if (this.pcs != null)
this.pcs.firePropertyChange("domainRollout", oldValue, value);
return (T) this;
}
/**
* Identifier of an overall multi-process domain operation of which this
* operation is a part, or undefined if this operation is not associated
* with such a domain operation.
*/
@ModelNodeBinding(detypedName = "domain-uuid")
public String domainUuid() {
return this.domainUuid;
}
/**
* Identifier of an overall multi-process domain operation of which this
* operation is a part, or undefined if this operation is not associated
* with such a domain operation.
*/
@SuppressWarnings("unchecked")
public T domainUuid(java.lang.String value) {
Object oldValue = this.domainUuid;
this.domainUuid = value;
if (this.pcs != null)
this.pcs.firePropertyChange("domainUuid", oldValue, value);
return (T) this;
}
/**
* Amount of time the operation has been executing with the exclusive
* operation execution lock held, or -1 if the operation does not hold the
* exclusive execution lock.
*/
@ModelNodeBinding(detypedName = "exclusive-running-time")
public Long exclusiveRunningTime() {
return this.exclusiveRunningTime;
}
/**
* Amount of time the operation has been executing with the exclusive
* operation execution lock held, or -1 if the operation does not hold the
* exclusive execution lock.
*/
@SuppressWarnings("unchecked")
public T exclusiveRunningTime(java.lang.Long value) {
Object oldValue = this.exclusiveRunningTime;
this.exclusiveRunningTime = value;
if (this.pcs != null)
this.pcs.firePropertyChange("exclusiveRunningTime", oldValue, value);
return (T) this;
}
/**
* The current activity of the operation.
*/
@ModelNodeBinding(detypedName = "execution-status")
public ExecutionStatus executionStatus() {
return this.executionStatus;
}
/**
* The current activity of the operation.
*/
@SuppressWarnings("unchecked")
public T executionStatus(ExecutionStatus value) {
Object oldValue = this.executionStatus;
this.executionStatus = value;
if (this.pcs != null)
this.pcs.firePropertyChange("executionStatus", oldValue, value);
return (T) this;
}
/**
* The name of the operation, or '' if the caller is not authorized
* to address the operation's target resource.
*/
@ModelNodeBinding(detypedName = "operation")
public String operation() {
return this.operation;
}
/**
* The name of the operation, or '' if the caller is not authorized
* to address the operation's target resource.
*/
@SuppressWarnings("unchecked")
public T operation(java.lang.String value) {
Object oldValue = this.operation;
this.operation = value;
if (this.pcs != null)
this.pcs.firePropertyChange("operation", oldValue, value);
return (T) this;
}
/**
* Amount of time the operation has been executing.
*/
@ModelNodeBinding(detypedName = "running-time")
public Long runningTime() {
return this.runningTime;
}
/**
* Amount of time the operation has been executing.
*/
@SuppressWarnings("unchecked")
public T runningTime(java.lang.Long value) {
Object oldValue = this.runningTime;
this.runningTime = value;
if (this.pcs != null)
this.pcs.firePropertyChange("runningTime", oldValue, value);
return (T) this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy