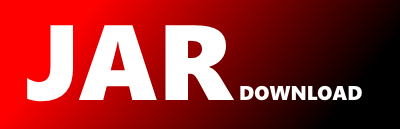
org.wildfly.swarm.config.messaging.activemq.DiscoveryGroup Maven / Gradle / Ivy
package org.wildfly.swarm.config.messaging.activemq;
import org.wildfly.swarm.config.runtime.AttributeDocumentation;
import org.wildfly.swarm.config.runtime.ResourceDocumentation;
import org.wildfly.swarm.config.runtime.SingletonResource;
import org.wildfly.swarm.config.runtime.Addresses;
import org.wildfly.swarm.config.runtime.ResourceType;
import java.beans.PropertyChangeSupport;
import java.beans.PropertyChangeListener;
import org.wildfly.swarm.config.runtime.ModelNodeBinding;
/**
* Multicast group to listen to receive broadcast from other servers announcing
* their connectors.
*/
@Addresses({"/subsystem=messaging-activemq/discovery-group=*",
"/subsystem=messaging-activemq/server=*/discovery-group=*"})
@ResourceType("discovery-group")
public class DiscoveryGroup>
implements
org.wildfly.swarm.config.runtime.Keyed {
private String key;
private PropertyChangeSupport pcs;
@AttributeDocumentation("Period, in ms, to wait for an initial broadcast to give us at least one node in the cluster.")
private Long initialWaitTimeout;
@AttributeDocumentation("References the name of a JGroups channel. If undefined, the default channel will be used.")
private String jgroupsChannel;
@AttributeDocumentation("The logical cluster name.")
private String jgroupsCluster;
@AttributeDocumentation("References the name of a JGroups channel factory.")
private String jgroupsStack;
@AttributeDocumentation("Period the discovery group waits after receiving the last broadcast from a particular server before removing that server's connector pair entry from its list.")
private Long refreshTimeout;
@AttributeDocumentation("The discovery group socket binding.")
private String socketBinding;
public DiscoveryGroup(java.lang.String key) {
super();
this.key = key;
}
public String getKey() {
return this.key;
}
/**
* Adds a property change listener
*/
public void addPropertyChangeListener(PropertyChangeListener listener) {
if (null == this.pcs)
this.pcs = new PropertyChangeSupport(this);
this.pcs.addPropertyChangeListener(listener);
}
/**
* Removes a property change listener
*/
public void removePropertyChangeListener(
java.beans.PropertyChangeListener listener) {
if (this.pcs != null)
this.pcs.removePropertyChangeListener(listener);
}
/**
* Period, in ms, to wait for an initial broadcast to give us at least one
* node in the cluster.
*/
@ModelNodeBinding(detypedName = "initial-wait-timeout")
public Long initialWaitTimeout() {
return this.initialWaitTimeout;
}
/**
* Period, in ms, to wait for an initial broadcast to give us at least one
* node in the cluster.
*/
@SuppressWarnings("unchecked")
public T initialWaitTimeout(java.lang.Long value) {
Object oldValue = this.initialWaitTimeout;
this.initialWaitTimeout = value;
if (this.pcs != null)
this.pcs.firePropertyChange("initialWaitTimeout", oldValue, value);
return (T) this;
}
/**
* References the name of a JGroups channel. If undefined, the default
* channel will be used.
*/
@ModelNodeBinding(detypedName = "jgroups-channel")
public String jgroupsChannel() {
return this.jgroupsChannel;
}
/**
* References the name of a JGroups channel. If undefined, the default
* channel will be used.
*/
@SuppressWarnings("unchecked")
public T jgroupsChannel(java.lang.String value) {
Object oldValue = this.jgroupsChannel;
this.jgroupsChannel = value;
if (this.pcs != null)
this.pcs.firePropertyChange("jgroupsChannel", oldValue, value);
return (T) this;
}
/**
* The logical cluster name.
*/
@ModelNodeBinding(detypedName = "jgroups-cluster")
public String jgroupsCluster() {
return this.jgroupsCluster;
}
/**
* The logical cluster name.
*/
@SuppressWarnings("unchecked")
public T jgroupsCluster(java.lang.String value) {
Object oldValue = this.jgroupsCluster;
this.jgroupsCluster = value;
if (this.pcs != null)
this.pcs.firePropertyChange("jgroupsCluster", oldValue, value);
return (T) this;
}
/**
* References the name of a JGroups channel factory.
*
* @deprecated Deprecated. Use jgroups-channel instead.
*/
@Deprecated
@ModelNodeBinding(detypedName = "jgroups-stack")
public String jgroupsStack() {
return this.jgroupsStack;
}
/**
* References the name of a JGroups channel factory.
*
* @deprecated Deprecated. Use jgroups-channel instead.
*/
@SuppressWarnings("unchecked")
@Deprecated
public T jgroupsStack(java.lang.String value) {
Object oldValue = this.jgroupsStack;
this.jgroupsStack = value;
if (this.pcs != null)
this.pcs.firePropertyChange("jgroupsStack", oldValue, value);
return (T) this;
}
/**
* Period the discovery group waits after receiving the last broadcast from
* a particular server before removing that server's connector pair entry
* from its list.
*/
@ModelNodeBinding(detypedName = "refresh-timeout")
public Long refreshTimeout() {
return this.refreshTimeout;
}
/**
* Period the discovery group waits after receiving the last broadcast from
* a particular server before removing that server's connector pair entry
* from its list.
*/
@SuppressWarnings("unchecked")
public T refreshTimeout(java.lang.Long value) {
Object oldValue = this.refreshTimeout;
this.refreshTimeout = value;
if (this.pcs != null)
this.pcs.firePropertyChange("refreshTimeout", oldValue, value);
return (T) this;
}
/**
* The discovery group socket binding.
*/
@ModelNodeBinding(detypedName = "socket-binding")
public String socketBinding() {
return this.socketBinding;
}
/**
* The discovery group socket binding.
*/
@SuppressWarnings("unchecked")
public T socketBinding(java.lang.String value) {
Object oldValue = this.socketBinding;
this.socketBinding = value;
if (this.pcs != null)
this.pcs.firePropertyChange("socketBinding", oldValue, value);
return (T) this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy