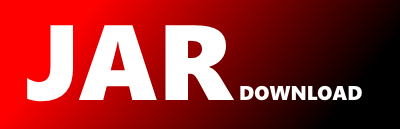
org.wildfly.swarm.config.modcluster.Proxy Maven / Gradle / Ivy
package org.wildfly.swarm.config.modcluster;
import org.wildfly.swarm.config.runtime.AttributeDocumentation;
import org.wildfly.swarm.config.runtime.ResourceDocumentation;
import org.wildfly.swarm.config.runtime.SingletonResource;
import org.wildfly.swarm.config.runtime.Address;
import org.wildfly.swarm.config.runtime.ResourceType;
import java.beans.PropertyChangeSupport;
import java.beans.PropertyChangeListener;
import java.util.List;
import org.wildfly.swarm.config.runtime.Subresource;
import org.wildfly.swarm.config.modcluster.proxy.ConfigurationSsl;
import org.wildfly.swarm.config.modcluster.proxy.ConfigurationSslConsumer;
import org.wildfly.swarm.config.modcluster.proxy.ConfigurationSslSupplier;
import org.wildfly.swarm.config.modcluster.proxy.DynamicLoadProvider;
import org.wildfly.swarm.config.modcluster.proxy.DynamicLoadProviderConsumer;
import org.wildfly.swarm.config.modcluster.proxy.DynamicLoadProviderSupplier;
import org.wildfly.swarm.config.modcluster.proxy.SimpleLoadProvider;
import org.wildfly.swarm.config.modcluster.proxy.SimpleLoadProviderConsumer;
import org.wildfly.swarm.config.modcluster.proxy.SimpleLoadProviderSupplier;
import org.wildfly.swarm.config.runtime.ModelNodeBinding;
import java.util.Arrays;
import java.util.stream.Collectors;
/**
* Proxy resource coupled with single Undertow listener (and server) specifying
* load balancer discovery, its configuration and load balance factor provider.
* Multiple proxy configuration can be specified.
*/
@Address("/subsystem=modcluster/proxy=*")
@ResourceType("proxy")
public class Proxy>
implements
org.wildfly.swarm.config.runtime.Keyed {
private String key;
private PropertyChangeSupport pcs;
private ProxyResources subresources = new ProxyResources();
@AttributeDocumentation("Whether to enable multicast-based advertise mechanism.")
private Boolean advertise;
@AttributeDocumentation("If specified, reverse proxy advertisements checksums will be verified using this value as a salt.")
private String advertiseSecurityKey;
@AttributeDocumentation("Name of socket binding to use for the advertise socket.")
private String advertiseSocket;
@AttributeDocumentation("If false, the contexts are registered with the reverse proxy as disabled, they need to be enabled manually by 'enable-context' operation or via mod_cluster_manager console (if available).")
private Boolean autoEnableContexts;
@AttributeDocumentation("The name of the balancer on the reverse proxy to register with.")
private String balancer;
@AttributeDocumentation("The name of Undertow listener that will be registered with the reverse proxy.")
private String connector;
@AttributeDocumentation("List of contexts to exclude from registration with the reverse proxies.")
private String excludedContexts;
@AttributeDocumentation("Whether to enable packet flushing on the reverse proxy.")
private Boolean flushPackets;
@AttributeDocumentation("Time to wait before flushing packets on the reverse proxy.")
private Integer flushWait;
@AttributeDocumentation("The name of Undertow listener that will be registered with the reverse proxy.")
private String listener;
@AttributeDocumentation("Name of the load balancing group this node belongs to.")
private String loadBalancingGroup;
@AttributeDocumentation("Maximum number of failover attempts by reverse proxy when sending the request to the backend server.")
private Integer maxAttempts;
@AttributeDocumentation("Timeout (in seconds) for proxy connections to a node. That is the time mod_cluster will wait for the back-end response before returning an error.")
private Integer nodeTimeout;
@AttributeDocumentation("Number of seconds for which to wait for a pong answer to a ping.")
private Integer ping;
@AttributeDocumentation("List of reverse proxies for mod_cluster to register with defined by 'outbound-socket-binding' in 'socket-binding-group'.")
private List proxies;
@AttributeDocumentation("List of reverse proxies to register with. Format (hostname:port) separated with commas.")
private String proxyList;
@AttributeDocumentation("Base URL for MCMP requests.")
private String proxyUrl;
@AttributeDocumentation("Session draining strategy used during undeployment of a web application.")
private SessionDrainingStrategy sessionDrainingStrategy;
@AttributeDocumentation("Soft maximum idle connection count for reverse proxy.")
private Integer smax;
@AttributeDocumentation("Timeout to wait for the reverse proxy to answer a MCMP message.")
private Integer socketTimeout;
@AttributeDocumentation("Reference to the SSLContext to be used by mod_cluster.")
private String sslContext;
@AttributeDocumentation("Number of seconds a STATUS message is sent from the application server to the proxy.")
private Integer statusInterval;
@AttributeDocumentation("Indicates whether subsequent requests for a given session should be routed to the same node, if possible.")
private Boolean stickySession;
@AttributeDocumentation("Indicates whether the reverse proxy should run an error in the event that the balancer is unable to route a request to the node to which it is stuck. Ignored if sticky sessions are disabled.")
private Boolean stickySessionForce;
@AttributeDocumentation("Indicates whether the reverse proxy should remove session stickiness in the event that the balancer is unable to route a request to the node to which it is stuck. Ignored if sticky sessions are disabled.")
private Boolean stickySessionRemove;
@AttributeDocumentation("Maximum time to wait for context to process pending requests.")
private Integer stopContextTimeout;
@AttributeDocumentation("Time to live (in seconds) for idle connections above smax.")
private Integer ttl;
@AttributeDocumentation("Number of seconds to wait for a worker to become available to handle a request.")
private Integer workerTimeout;
public Proxy(java.lang.String key) {
super();
this.key = key;
}
public String getKey() {
return this.key;
}
/**
* Adds a property change listener
*/
public void addPropertyChangeListener(PropertyChangeListener listener) {
if (null == this.pcs)
this.pcs = new PropertyChangeSupport(this);
this.pcs.addPropertyChangeListener(listener);
}
/**
* Removes a property change listener
*/
public void removePropertyChangeListener(
java.beans.PropertyChangeListener listener) {
if (this.pcs != null)
this.pcs.removePropertyChangeListener(listener);
}
public ProxyResources subresources() {
return this.subresources;
}
/**
* Configuration and runtime operations for mod_cluster subsystem.
*/
@SuppressWarnings("unchecked")
public T configurationSsl(ConfigurationSsl value) {
this.subresources.configurationSsl = value;
return (T) this;
}
/**
* Configuration and runtime operations for mod_cluster subsystem.
*/
@SuppressWarnings("unchecked")
public T configurationSsl(ConfigurationSslConsumer consumer) {
ConfigurationSsl extends ConfigurationSsl> child = new ConfigurationSsl<>();
if (consumer != null) {
consumer.accept(child);
}
this.subresources.configurationSsl = child;
return (T) this;
}
/**
* Configuration and runtime operations for mod_cluster subsystem.
*/
@SuppressWarnings("unchecked")
public T configurationSsl() {
ConfigurationSsl extends ConfigurationSsl> child = new ConfigurationSsl<>();
this.subresources.configurationSsl = child;
return (T) this;
}
/**
* Configuration and runtime operations for mod_cluster subsystem.
*/
@SuppressWarnings("unchecked")
public T configurationSsl(ConfigurationSslSupplier supplier) {
this.subresources.configurationSsl = supplier.get();
return (T) this;
}
/**
* Configuration and runtime operations for mod_cluster subsystem.
*/
@SuppressWarnings("unchecked")
public T dynamicLoadProvider(DynamicLoadProvider value) {
this.subresources.dynamicLoadProvider = value;
return (T) this;
}
/**
* Configuration and runtime operations for mod_cluster subsystem.
*/
@SuppressWarnings("unchecked")
public T dynamicLoadProvider(DynamicLoadProviderConsumer consumer) {
DynamicLoadProvider extends DynamicLoadProvider> child = new DynamicLoadProvider<>();
if (consumer != null) {
consumer.accept(child);
}
this.subresources.dynamicLoadProvider = child;
return (T) this;
}
/**
* Configuration and runtime operations for mod_cluster subsystem.
*/
@SuppressWarnings("unchecked")
public T dynamicLoadProvider() {
DynamicLoadProvider extends DynamicLoadProvider> child = new DynamicLoadProvider<>();
this.subresources.dynamicLoadProvider = child;
return (T) this;
}
/**
* Configuration and runtime operations for mod_cluster subsystem.
*/
@SuppressWarnings("unchecked")
public T dynamicLoadProvider(DynamicLoadProviderSupplier supplier) {
this.subresources.dynamicLoadProvider = supplier.get();
return (T) this;
}
/**
* Simple load provider returns constant pre-configured load balancing
* factor.
*/
@SuppressWarnings("unchecked")
public T simpleLoadProvider(SimpleLoadProvider value) {
this.subresources.simpleLoadProvider = value;
return (T) this;
}
/**
* Simple load provider returns constant pre-configured load balancing
* factor.
*/
@SuppressWarnings("unchecked")
public T simpleLoadProvider(SimpleLoadProviderConsumer consumer) {
SimpleLoadProvider extends SimpleLoadProvider> child = new SimpleLoadProvider<>();
if (consumer != null) {
consumer.accept(child);
}
this.subresources.simpleLoadProvider = child;
return (T) this;
}
/**
* Simple load provider returns constant pre-configured load balancing
* factor.
*/
@SuppressWarnings("unchecked")
public T simpleLoadProvider() {
SimpleLoadProvider extends SimpleLoadProvider> child = new SimpleLoadProvider<>();
this.subresources.simpleLoadProvider = child;
return (T) this;
}
/**
* Simple load provider returns constant pre-configured load balancing
* factor.
*/
@SuppressWarnings("unchecked")
public T simpleLoadProvider(SimpleLoadProviderSupplier supplier) {
this.subresources.simpleLoadProvider = supplier.get();
return (T) this;
}
/**
* Child mutators for Proxy
*/
public static class ProxyResources {
@SingletonResource
@ResourceDocumentation("Configuration and runtime operations for mod_cluster subsystem.")
private ConfigurationSsl configurationSsl;
@SingletonResource
@ResourceDocumentation("Configuration and runtime operations for mod_cluster subsystem.")
private DynamicLoadProvider dynamicLoadProvider;
@SingletonResource
@ResourceDocumentation("Simple load provider returns constant pre-configured load balancing factor.")
private SimpleLoadProvider simpleLoadProvider;
/**
* Configuration and runtime operations for mod_cluster subsystem.
*/
@Subresource
public ConfigurationSsl configurationSsl() {
return this.configurationSsl;
}
/**
* Configuration and runtime operations for mod_cluster subsystem.
*/
@Subresource
public DynamicLoadProvider dynamicLoadProvider() {
return this.dynamicLoadProvider;
}
/**
* Simple load provider returns constant pre-configured load balancing
* factor.
*/
@Subresource
public SimpleLoadProvider simpleLoadProvider() {
return this.simpleLoadProvider;
}
}
public static enum SessionDrainingStrategy {
DEFAULT("DEFAULT"), ALWAYS("ALWAYS"), NEVER("NEVER");
private final String allowedValue;
/**
* Returns the allowed value for the management model.
*
* @return the allowed model value
*/
public String getAllowedValue() {
return allowedValue;
}
SessionDrainingStrategy(java.lang.String allowedValue) {
this.allowedValue = allowedValue;
}
@Override
public String toString() {
return allowedValue;
}
}
/**
* Whether to enable multicast-based advertise mechanism.
*/
@ModelNodeBinding(detypedName = "advertise")
public Boolean advertise() {
return this.advertise;
}
/**
* Whether to enable multicast-based advertise mechanism.
*/
@SuppressWarnings("unchecked")
public T advertise(java.lang.Boolean value) {
Object oldValue = this.advertise;
this.advertise = value;
if (this.pcs != null)
this.pcs.firePropertyChange("advertise", oldValue, value);
return (T) this;
}
/**
* If specified, reverse proxy advertisements checksums will be verified
* using this value as a salt.
*/
@ModelNodeBinding(detypedName = "advertise-security-key")
public String advertiseSecurityKey() {
return this.advertiseSecurityKey;
}
/**
* If specified, reverse proxy advertisements checksums will be verified
* using this value as a salt.
*/
@SuppressWarnings("unchecked")
public T advertiseSecurityKey(java.lang.String value) {
Object oldValue = this.advertiseSecurityKey;
this.advertiseSecurityKey = value;
if (this.pcs != null)
this.pcs.firePropertyChange("advertiseSecurityKey", oldValue, value);
return (T) this;
}
/**
* Name of socket binding to use for the advertise socket.
*/
@ModelNodeBinding(detypedName = "advertise-socket")
public String advertiseSocket() {
return this.advertiseSocket;
}
/**
* Name of socket binding to use for the advertise socket.
*/
@SuppressWarnings("unchecked")
public T advertiseSocket(java.lang.String value) {
Object oldValue = this.advertiseSocket;
this.advertiseSocket = value;
if (this.pcs != null)
this.pcs.firePropertyChange("advertiseSocket", oldValue, value);
return (T) this;
}
/**
* If false, the contexts are registered with the reverse proxy as disabled,
* they need to be enabled manually by 'enable-context' operation or via
* mod_cluster_manager console (if available).
*/
@ModelNodeBinding(detypedName = "auto-enable-contexts")
public Boolean autoEnableContexts() {
return this.autoEnableContexts;
}
/**
* If false, the contexts are registered with the reverse proxy as disabled,
* they need to be enabled manually by 'enable-context' operation or via
* mod_cluster_manager console (if available).
*/
@SuppressWarnings("unchecked")
public T autoEnableContexts(java.lang.Boolean value) {
Object oldValue = this.autoEnableContexts;
this.autoEnableContexts = value;
if (this.pcs != null)
this.pcs.firePropertyChange("autoEnableContexts", oldValue, value);
return (T) this;
}
/**
* The name of the balancer on the reverse proxy to register with.
*/
@ModelNodeBinding(detypedName = "balancer")
public String balancer() {
return this.balancer;
}
/**
* The name of the balancer on the reverse proxy to register with.
*/
@SuppressWarnings("unchecked")
public T balancer(java.lang.String value) {
Object oldValue = this.balancer;
this.balancer = value;
if (this.pcs != null)
this.pcs.firePropertyChange("balancer", oldValue, value);
return (T) this;
}
/**
* The name of Undertow listener that will be registered with the reverse
* proxy.
*
* @deprecated Deprecated. Functions as alias for the 'listener' attribute.
*/
@Deprecated
@ModelNodeBinding(detypedName = "connector")
public String connector() {
return this.connector;
}
/**
* The name of Undertow listener that will be registered with the reverse
* proxy.
*
* @deprecated Deprecated. Functions as alias for the 'listener' attribute.
*/
@SuppressWarnings("unchecked")
@Deprecated
public T connector(java.lang.String value) {
Object oldValue = this.connector;
this.connector = value;
if (this.pcs != null)
this.pcs.firePropertyChange("connector", oldValue, value);
return (T) this;
}
/**
* List of contexts to exclude from registration with the reverse proxies.
*/
@ModelNodeBinding(detypedName = "excluded-contexts")
public String excludedContexts() {
return this.excludedContexts;
}
/**
* List of contexts to exclude from registration with the reverse proxies.
*/
@SuppressWarnings("unchecked")
public T excludedContexts(java.lang.String value) {
Object oldValue = this.excludedContexts;
this.excludedContexts = value;
if (this.pcs != null)
this.pcs.firePropertyChange("excludedContexts", oldValue, value);
return (T) this;
}
/**
* Whether to enable packet flushing on the reverse proxy.
*/
@ModelNodeBinding(detypedName = "flush-packets")
public Boolean flushPackets() {
return this.flushPackets;
}
/**
* Whether to enable packet flushing on the reverse proxy.
*/
@SuppressWarnings("unchecked")
public T flushPackets(java.lang.Boolean value) {
Object oldValue = this.flushPackets;
this.flushPackets = value;
if (this.pcs != null)
this.pcs.firePropertyChange("flushPackets", oldValue, value);
return (T) this;
}
/**
* Time to wait before flushing packets on the reverse proxy.
*/
@ModelNodeBinding(detypedName = "flush-wait")
public Integer flushWait() {
return this.flushWait;
}
/**
* Time to wait before flushing packets on the reverse proxy.
*/
@SuppressWarnings("unchecked")
public T flushWait(java.lang.Integer value) {
Object oldValue = this.flushWait;
this.flushWait = value;
if (this.pcs != null)
this.pcs.firePropertyChange("flushWait", oldValue, value);
return (T) this;
}
/**
* The name of Undertow listener that will be registered with the reverse
* proxy.
*/
@ModelNodeBinding(detypedName = "listener")
public String listener() {
return this.listener;
}
/**
* The name of Undertow listener that will be registered with the reverse
* proxy.
*/
@SuppressWarnings("unchecked")
public T listener(java.lang.String value) {
Object oldValue = this.listener;
this.listener = value;
if (this.pcs != null)
this.pcs.firePropertyChange("listener", oldValue, value);
return (T) this;
}
/**
* Name of the load balancing group this node belongs to.
*/
@ModelNodeBinding(detypedName = "load-balancing-group")
public String loadBalancingGroup() {
return this.loadBalancingGroup;
}
/**
* Name of the load balancing group this node belongs to.
*/
@SuppressWarnings("unchecked")
public T loadBalancingGroup(java.lang.String value) {
Object oldValue = this.loadBalancingGroup;
this.loadBalancingGroup = value;
if (this.pcs != null)
this.pcs.firePropertyChange("loadBalancingGroup", oldValue, value);
return (T) this;
}
/**
* Maximum number of failover attempts by reverse proxy when sending the
* request to the backend server.
*/
@ModelNodeBinding(detypedName = "max-attempts")
public Integer maxAttempts() {
return this.maxAttempts;
}
/**
* Maximum number of failover attempts by reverse proxy when sending the
* request to the backend server.
*/
@SuppressWarnings("unchecked")
public T maxAttempts(java.lang.Integer value) {
Object oldValue = this.maxAttempts;
this.maxAttempts = value;
if (this.pcs != null)
this.pcs.firePropertyChange("maxAttempts", oldValue, value);
return (T) this;
}
/**
* Timeout (in seconds) for proxy connections to a node. That is the time
* mod_cluster will wait for the back-end response before returning an
* error.
*/
@ModelNodeBinding(detypedName = "node-timeout")
public Integer nodeTimeout() {
return this.nodeTimeout;
}
/**
* Timeout (in seconds) for proxy connections to a node. That is the time
* mod_cluster will wait for the back-end response before returning an
* error.
*/
@SuppressWarnings("unchecked")
public T nodeTimeout(java.lang.Integer value) {
Object oldValue = this.nodeTimeout;
this.nodeTimeout = value;
if (this.pcs != null)
this.pcs.firePropertyChange("nodeTimeout", oldValue, value);
return (T) this;
}
/**
* Number of seconds for which to wait for a pong answer to a ping.
*/
@ModelNodeBinding(detypedName = "ping")
public Integer ping() {
return this.ping;
}
/**
* Number of seconds for which to wait for a pong answer to a ping.
*/
@SuppressWarnings("unchecked")
public T ping(java.lang.Integer value) {
Object oldValue = this.ping;
this.ping = value;
if (this.pcs != null)
this.pcs.firePropertyChange("ping", oldValue, value);
return (T) this;
}
/**
* List of reverse proxies for mod_cluster to register with defined by
* 'outbound-socket-binding' in 'socket-binding-group'.
*/
@ModelNodeBinding(detypedName = "proxies")
public List proxies() {
return this.proxies;
}
/**
* List of reverse proxies for mod_cluster to register with defined by
* 'outbound-socket-binding' in 'socket-binding-group'.
*/
@SuppressWarnings("unchecked")
public T proxies(java.util.List value) {
Object oldValue = this.proxies;
this.proxies = value;
if (this.pcs != null)
this.pcs.firePropertyChange("proxies", oldValue, value);
return (T) this;
}
/**
* List of reverse proxies for mod_cluster to register with defined by
* 'outbound-socket-binding' in 'socket-binding-group'.
*/
@SuppressWarnings("unchecked")
public T proxy(String value) {
if (this.proxies == null) {
this.proxies = new java.util.ArrayList<>();
}
this.proxies.add(value);
return (T) this;
}
/**
* List of reverse proxies for mod_cluster to register with defined by
* 'outbound-socket-binding' in 'socket-binding-group'.
*/
@SuppressWarnings("unchecked")
public T proxies(String... args) {
proxies(Arrays.stream(args).collect(Collectors.toList()));
return (T) this;
}
/**
* List of reverse proxies to register with. Format (hostname:port)
* separated with commas.
*
* @deprecated The 'proxy-list' attribute has been deprecated in favor of
* proxies (list of references to outbound-socket-binding)
*/
@Deprecated
@ModelNodeBinding(detypedName = "proxy-list")
public String proxyList() {
return this.proxyList;
}
/**
* List of reverse proxies to register with. Format (hostname:port)
* separated with commas.
*
* @deprecated The 'proxy-list' attribute has been deprecated in favor of
* proxies (list of references to outbound-socket-binding)
*/
@SuppressWarnings("unchecked")
@Deprecated
public T proxyList(java.lang.String value) {
Object oldValue = this.proxyList;
this.proxyList = value;
if (this.pcs != null)
this.pcs.firePropertyChange("proxyList", oldValue, value);
return (T) this;
}
/**
* Base URL for MCMP requests.
*/
@ModelNodeBinding(detypedName = "proxy-url")
public String proxyUrl() {
return this.proxyUrl;
}
/**
* Base URL for MCMP requests.
*/
@SuppressWarnings("unchecked")
public T proxyUrl(java.lang.String value) {
Object oldValue = this.proxyUrl;
this.proxyUrl = value;
if (this.pcs != null)
this.pcs.firePropertyChange("proxyUrl", oldValue, value);
return (T) this;
}
/**
* Session draining strategy used during undeployment of a web application.
*/
@ModelNodeBinding(detypedName = "session-draining-strategy")
public SessionDrainingStrategy sessionDrainingStrategy() {
return this.sessionDrainingStrategy;
}
/**
* Session draining strategy used during undeployment of a web application.
*/
@SuppressWarnings("unchecked")
public T sessionDrainingStrategy(SessionDrainingStrategy value) {
Object oldValue = this.sessionDrainingStrategy;
this.sessionDrainingStrategy = value;
if (this.pcs != null)
this.pcs.firePropertyChange("sessionDrainingStrategy", oldValue,
value);
return (T) this;
}
/**
* Soft maximum idle connection count for reverse proxy.
*/
@ModelNodeBinding(detypedName = "smax")
public Integer smax() {
return this.smax;
}
/**
* Soft maximum idle connection count for reverse proxy.
*/
@SuppressWarnings("unchecked")
public T smax(java.lang.Integer value) {
Object oldValue = this.smax;
this.smax = value;
if (this.pcs != null)
this.pcs.firePropertyChange("smax", oldValue, value);
return (T) this;
}
/**
* Timeout to wait for the reverse proxy to answer a MCMP message.
*/
@ModelNodeBinding(detypedName = "socket-timeout")
public Integer socketTimeout() {
return this.socketTimeout;
}
/**
* Timeout to wait for the reverse proxy to answer a MCMP message.
*/
@SuppressWarnings("unchecked")
public T socketTimeout(java.lang.Integer value) {
Object oldValue = this.socketTimeout;
this.socketTimeout = value;
if (this.pcs != null)
this.pcs.firePropertyChange("socketTimeout", oldValue, value);
return (T) this;
}
/**
* Reference to the SSLContext to be used by mod_cluster.
*/
@ModelNodeBinding(detypedName = "ssl-context")
public String sslContext() {
return this.sslContext;
}
/**
* Reference to the SSLContext to be used by mod_cluster.
*/
@SuppressWarnings("unchecked")
public T sslContext(java.lang.String value) {
Object oldValue = this.sslContext;
this.sslContext = value;
if (this.pcs != null)
this.pcs.firePropertyChange("sslContext", oldValue, value);
return (T) this;
}
/**
* Number of seconds a STATUS message is sent from the application server to
* the proxy.
*/
@ModelNodeBinding(detypedName = "status-interval")
public Integer statusInterval() {
return this.statusInterval;
}
/**
* Number of seconds a STATUS message is sent from the application server to
* the proxy.
*/
@SuppressWarnings("unchecked")
public T statusInterval(java.lang.Integer value) {
Object oldValue = this.statusInterval;
this.statusInterval = value;
if (this.pcs != null)
this.pcs.firePropertyChange("statusInterval", oldValue, value);
return (T) this;
}
/**
* Indicates whether subsequent requests for a given session should be
* routed to the same node, if possible.
*/
@ModelNodeBinding(detypedName = "sticky-session")
public Boolean stickySession() {
return this.stickySession;
}
/**
* Indicates whether subsequent requests for a given session should be
* routed to the same node, if possible.
*/
@SuppressWarnings("unchecked")
public T stickySession(java.lang.Boolean value) {
Object oldValue = this.stickySession;
this.stickySession = value;
if (this.pcs != null)
this.pcs.firePropertyChange("stickySession", oldValue, value);
return (T) this;
}
/**
* Indicates whether the reverse proxy should run an error in the event that
* the balancer is unable to route a request to the node to which it is
* stuck. Ignored if sticky sessions are disabled.
*/
@ModelNodeBinding(detypedName = "sticky-session-force")
public Boolean stickySessionForce() {
return this.stickySessionForce;
}
/**
* Indicates whether the reverse proxy should run an error in the event that
* the balancer is unable to route a request to the node to which it is
* stuck. Ignored if sticky sessions are disabled.
*/
@SuppressWarnings("unchecked")
public T stickySessionForce(java.lang.Boolean value) {
Object oldValue = this.stickySessionForce;
this.stickySessionForce = value;
if (this.pcs != null)
this.pcs.firePropertyChange("stickySessionForce", oldValue, value);
return (T) this;
}
/**
* Indicates whether the reverse proxy should remove session stickiness in
* the event that the balancer is unable to route a request to the node to
* which it is stuck. Ignored if sticky sessions are disabled.
*/
@ModelNodeBinding(detypedName = "sticky-session-remove")
public Boolean stickySessionRemove() {
return this.stickySessionRemove;
}
/**
* Indicates whether the reverse proxy should remove session stickiness in
* the event that the balancer is unable to route a request to the node to
* which it is stuck. Ignored if sticky sessions are disabled.
*/
@SuppressWarnings("unchecked")
public T stickySessionRemove(java.lang.Boolean value) {
Object oldValue = this.stickySessionRemove;
this.stickySessionRemove = value;
if (this.pcs != null)
this.pcs.firePropertyChange("stickySessionRemove", oldValue, value);
return (T) this;
}
/**
* Maximum time to wait for context to process pending requests.
*/
@ModelNodeBinding(detypedName = "stop-context-timeout")
public Integer stopContextTimeout() {
return this.stopContextTimeout;
}
/**
* Maximum time to wait for context to process pending requests.
*/
@SuppressWarnings("unchecked")
public T stopContextTimeout(java.lang.Integer value) {
Object oldValue = this.stopContextTimeout;
this.stopContextTimeout = value;
if (this.pcs != null)
this.pcs.firePropertyChange("stopContextTimeout", oldValue, value);
return (T) this;
}
/**
* Time to live (in seconds) for idle connections above smax.
*/
@ModelNodeBinding(detypedName = "ttl")
public Integer ttl() {
return this.ttl;
}
/**
* Time to live (in seconds) for idle connections above smax.
*/
@SuppressWarnings("unchecked")
public T ttl(java.lang.Integer value) {
Object oldValue = this.ttl;
this.ttl = value;
if (this.pcs != null)
this.pcs.firePropertyChange("ttl", oldValue, value);
return (T) this;
}
/**
* Number of seconds to wait for a worker to become available to handle a
* request.
*/
@ModelNodeBinding(detypedName = "worker-timeout")
public Integer workerTimeout() {
return this.workerTimeout;
}
/**
* Number of seconds to wait for a worker to become available to handle a
* request.
*/
@SuppressWarnings("unchecked")
public T workerTimeout(java.lang.Integer value) {
Object oldValue = this.workerTimeout;
this.workerTimeout = value;
if (this.pcs != null)
this.pcs.firePropertyChange("workerTimeout", oldValue, value);
return (T) this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy