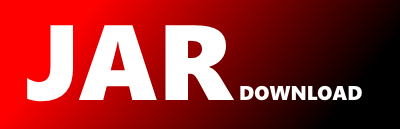
org.wildfly.swarm.config.resource.adapters.ResourceAdapter Maven / Gradle / Ivy
package org.wildfly.swarm.config.resource.adapters;
import org.wildfly.swarm.config.runtime.AttributeDocumentation;
import org.wildfly.swarm.config.runtime.ResourceDocumentation;
import org.wildfly.swarm.config.runtime.SingletonResource;
import org.wildfly.swarm.config.runtime.Address;
import org.wildfly.swarm.config.runtime.ResourceType;
import java.beans.PropertyChangeSupport;
import java.beans.PropertyChangeListener;
import java.util.List;
import org.wildfly.swarm.config.runtime.Subresource;
import org.wildfly.swarm.config.resource.adapters.resource_adapter.ConfigPropertiesConsumer;
import org.wildfly.swarm.config.resource.adapters.resource_adapter.ConfigPropertiesSupplier;
import org.wildfly.swarm.config.resource.adapters.resource_adapter.ConfigProperties;
import org.wildfly.swarm.config.runtime.SubresourceInfo;
import org.wildfly.swarm.config.resource.adapters.resource_adapter.AdminObjectsConsumer;
import org.wildfly.swarm.config.resource.adapters.resource_adapter.AdminObjectsSupplier;
import org.wildfly.swarm.config.resource.adapters.resource_adapter.AdminObjects;
import org.wildfly.swarm.config.resource.adapters.resource_adapter.ConnectionDefinitionsConsumer;
import org.wildfly.swarm.config.resource.adapters.resource_adapter.ConnectionDefinitionsSupplier;
import org.wildfly.swarm.config.resource.adapters.resource_adapter.ConnectionDefinitions;
import org.wildfly.swarm.config.runtime.ModelNodeBinding;
import java.util.Arrays;
import java.util.stream.Collectors;
import java.util.Map;
/**
* The configuration of a resource adapter.
*/
@Address("/subsystem=resource-adapters/resource-adapter=*")
@ResourceType("resource-adapter")
public class ResourceAdapter>
implements
org.wildfly.swarm.config.runtime.Keyed {
private String key;
private PropertyChangeSupport pcs;
private ResourceAdapterResources subresources = new ResourceAdapterResources();
@AttributeDocumentation("Specifies the resource adapter archive.")
private String archive;
@AttributeDocumentation("Specifies the bean validation groups that should be used.")
private List beanvalidationgroups;
@AttributeDocumentation("Specifies the unique name of the bootstrap context that should be used.")
private String bootstrapContext;
@AttributeDocumentation("Specifies the module from which resource adapter will be loaded")
private String module;
@AttributeDocumentation("Define whether runtime statistics are enabled or not.")
private Boolean statisticsEnabled;
@AttributeDocumentation("Specifies the transaction support level of the resource adapter.")
private TransactionSupport transactionSupport;
@AttributeDocumentation("Defines the name of the Elytron security domain that should be used.")
private String wmElytronSecurityDomain;
@AttributeDocumentation("Toggle on/off wm.security for this resource adapter. In case of false all wm-security-* parameters are ignored, even the defaults.")
private Boolean wmSecurity;
@AttributeDocumentation("Defines a default groups list that should be added to the used Subject instance.")
private List wmSecurityDefaultGroups;
@AttributeDocumentation("Defines a default principal name that should be added to the used Subject instance.")
private String wmSecurityDefaultPrincipal;
@AttributeDocumentation("Defines the name of the PicketBox security domain that should be used.")
private String wmSecurityDomain;
@AttributeDocumentation("List of groups mappings.")
private List wmSecurityMappingGroups;
@AttributeDocumentation("Defines if a mapping is required for security credentials.")
private Boolean wmSecurityMappingRequired;
@AttributeDocumentation("List of user mappings.")
private List wmSecurityMappingUsers;
public ResourceAdapter(java.lang.String key) {
super();
this.key = key;
}
public String getKey() {
return this.key;
}
/**
* Adds a property change listener
*/
public void addPropertyChangeListener(PropertyChangeListener listener) {
if (null == this.pcs)
this.pcs = new PropertyChangeSupport(this);
this.pcs.addPropertyChangeListener(listener);
}
/**
* Removes a property change listener
*/
public void removePropertyChangeListener(
java.beans.PropertyChangeListener listener) {
if (this.pcs != null)
this.pcs.removePropertyChangeListener(listener);
}
public ResourceAdapterResources subresources() {
return this.subresources;
}
/**
* Add all ConfigProperties objects to this subresource
*
* @return this
* @param value
* List of ConfigProperties objects.
*/
@SuppressWarnings("unchecked")
public T configProperties(java.util.List value) {
this.subresources.configProperties = value;
return (T) this;
}
/**
* Add the ConfigProperties object to the list of subresources
*
* @param value
* The ConfigProperties to add
* @return this
*/
@SuppressWarnings("unchecked")
public T configProperties(ConfigProperties value) {
this.subresources.configProperties.add(value);
return (T) this;
}
/**
* Create and configure a ConfigProperties object to the list of
* subresources
*
* @param key
* The key for the ConfigProperties resource
* @param config
* The ConfigPropertiesConsumer to use
* @return this
*/
@SuppressWarnings("unchecked")
public T configProperties(java.lang.String childKey,
ConfigPropertiesConsumer consumer) {
ConfigProperties extends ConfigProperties> child = new ConfigProperties<>(
childKey);
if (consumer != null) {
consumer.accept(child);
}
configProperties(child);
return (T) this;
}
/**
* Create and configure a ConfigProperties object to the list of
* subresources
*
* @param key
* The key for the ConfigProperties resource
* @return this
*/
@SuppressWarnings("unchecked")
public T configProperties(java.lang.String childKey) {
configProperties(childKey, null);
return (T) this;
}
/**
* Install a supplied ConfigProperties object to the list of subresources
*/
@SuppressWarnings("unchecked")
public T configProperties(ConfigPropertiesSupplier supplier) {
configProperties(supplier.get());
return (T) this;
}
/**
* Add all AdminObjects objects to this subresource
*
* @return this
* @param value
* List of AdminObjects objects.
*/
@SuppressWarnings("unchecked")
public T adminObjects(java.util.List value) {
this.subresources.adminObjects = value;
return (T) this;
}
/**
* Add the AdminObjects object to the list of subresources
*
* @param value
* The AdminObjects to add
* @return this
*/
@SuppressWarnings("unchecked")
public T adminObjects(AdminObjects value) {
this.subresources.adminObjects.add(value);
return (T) this;
}
/**
* Create and configure a AdminObjects object to the list of subresources
*
* @param key
* The key for the AdminObjects resource
* @param config
* The AdminObjectsConsumer to use
* @return this
*/
@SuppressWarnings("unchecked")
public T adminObjects(java.lang.String childKey,
AdminObjectsConsumer consumer) {
AdminObjects extends AdminObjects> child = new AdminObjects<>(
childKey);
if (consumer != null) {
consumer.accept(child);
}
adminObjects(child);
return (T) this;
}
/**
* Create and configure a AdminObjects object to the list of subresources
*
* @param key
* The key for the AdminObjects resource
* @return this
*/
@SuppressWarnings("unchecked")
public T adminObjects(java.lang.String childKey) {
adminObjects(childKey, null);
return (T) this;
}
/**
* Install a supplied AdminObjects object to the list of subresources
*/
@SuppressWarnings("unchecked")
public T adminObjects(AdminObjectsSupplier supplier) {
adminObjects(supplier.get());
return (T) this;
}
/**
* Add all ConnectionDefinitions objects to this subresource
*
* @return this
* @param value
* List of ConnectionDefinitions objects.
*/
@SuppressWarnings("unchecked")
public T connectionDefinitions(java.util.List value) {
this.subresources.connectionDefinitions = value;
return (T) this;
}
/**
* Add the ConnectionDefinitions object to the list of subresources
*
* @param value
* The ConnectionDefinitions to add
* @return this
*/
@SuppressWarnings("unchecked")
public T connectionDefinitions(ConnectionDefinitions value) {
this.subresources.connectionDefinitions.add(value);
return (T) this;
}
/**
* Create and configure a ConnectionDefinitions object to the list of
* subresources
*
* @param key
* The key for the ConnectionDefinitions resource
* @param config
* The ConnectionDefinitionsConsumer to use
* @return this
*/
@SuppressWarnings("unchecked")
public T connectionDefinitions(java.lang.String childKey,
ConnectionDefinitionsConsumer consumer) {
ConnectionDefinitions extends ConnectionDefinitions> child = new ConnectionDefinitions<>(
childKey);
if (consumer != null) {
consumer.accept(child);
}
connectionDefinitions(child);
return (T) this;
}
/**
* Create and configure a ConnectionDefinitions object to the list of
* subresources
*
* @param key
* The key for the ConnectionDefinitions resource
* @return this
*/
@SuppressWarnings("unchecked")
public T connectionDefinitions(java.lang.String childKey) {
connectionDefinitions(childKey, null);
return (T) this;
}
/**
* Install a supplied ConnectionDefinitions object to the list of
* subresources
*/
@SuppressWarnings("unchecked")
public T connectionDefinitions(ConnectionDefinitionsSupplier supplier) {
connectionDefinitions(supplier.get());
return (T) this;
}
/**
* Child mutators for ResourceAdapter
*/
public static class ResourceAdapterResources {
/**
* A custom defined config property.
*/
@ResourceDocumentation("A custom defined config property.")
@SubresourceInfo("configProperties")
private List configProperties = new java.util.ArrayList<>();
/**
* Specifies an administration object.
*/
@ResourceDocumentation("Specifies an administration object.")
@SubresourceInfo("adminObjects")
private List adminObjects = new java.util.ArrayList<>();
/**
* Specifies a connection definition.
*/
@ResourceDocumentation("Specifies a connection definition.")
@SubresourceInfo("connectionDefinitions")
private List connectionDefinitions = new java.util.ArrayList<>();
/**
* Get the list of ConfigProperties resources
*
* @return the list of resources
*/
@Subresource
public List configProperties() {
return this.configProperties;
}
public ConfigProperties configProperties(java.lang.String key) {
return this.configProperties.stream()
.filter(e -> e.getKey().equals(key)).findFirst()
.orElse(null);
}
/**
* Get the list of AdminObjects resources
*
* @return the list of resources
*/
@Subresource
public List adminObjects() {
return this.adminObjects;
}
public AdminObjects adminObjects(java.lang.String key) {
return this.adminObjects.stream()
.filter(e -> e.getKey().equals(key)).findFirst()
.orElse(null);
}
/**
* Get the list of ConnectionDefinitions resources
*
* @return the list of resources
*/
@Subresource
public List connectionDefinitions() {
return this.connectionDefinitions;
}
public ConnectionDefinitions connectionDefinitions(java.lang.String key) {
return this.connectionDefinitions.stream()
.filter(e -> e.getKey().equals(key)).findFirst()
.orElse(null);
}
}
public static enum TransactionSupport {
NOTRANSACTION("NoTransaction"), LOCALTRANSACTION("LocalTransaction"), XATRANSACTION(
"XATransaction");
private final String allowedValue;
/**
* Returns the allowed value for the management model.
*
* @return the allowed model value
*/
public String getAllowedValue() {
return allowedValue;
}
TransactionSupport(java.lang.String allowedValue) {
this.allowedValue = allowedValue;
}
@Override
public String toString() {
return allowedValue;
}
}
/**
* Specifies the resource adapter archive.
*/
@ModelNodeBinding(detypedName = "archive")
public String archive() {
return this.archive;
}
/**
* Specifies the resource adapter archive.
*/
@SuppressWarnings("unchecked")
public T archive(java.lang.String value) {
Object oldValue = this.archive;
this.archive = value;
if (this.pcs != null)
this.pcs.firePropertyChange("archive", oldValue, value);
return (T) this;
}
/**
* Specifies the bean validation groups that should be used.
*/
@ModelNodeBinding(detypedName = "beanvalidationgroups")
public List beanvalidationgroups() {
return this.beanvalidationgroups;
}
/**
* Specifies the bean validation groups that should be used.
*/
@SuppressWarnings("unchecked")
public T beanvalidationgroups(java.util.List value) {
Object oldValue = this.beanvalidationgroups;
this.beanvalidationgroups = value;
if (this.pcs != null)
this.pcs.firePropertyChange("beanvalidationgroups", oldValue, value);
return (T) this;
}
/**
* Specifies the bean validation groups that should be used.
*/
@SuppressWarnings("unchecked")
public T beanvalidationgroup(String value) {
if (this.beanvalidationgroups == null) {
this.beanvalidationgroups = new java.util.ArrayList<>();
}
this.beanvalidationgroups.add(value);
return (T) this;
}
/**
* Specifies the bean validation groups that should be used.
*/
@SuppressWarnings("unchecked")
public T beanvalidationgroups(String... args) {
beanvalidationgroups(Arrays.stream(args).collect(Collectors.toList()));
return (T) this;
}
/**
* Specifies the unique name of the bootstrap context that should be used.
*/
@ModelNodeBinding(detypedName = "bootstrap-context")
public String bootstrapContext() {
return this.bootstrapContext;
}
/**
* Specifies the unique name of the bootstrap context that should be used.
*/
@SuppressWarnings("unchecked")
public T bootstrapContext(java.lang.String value) {
Object oldValue = this.bootstrapContext;
this.bootstrapContext = value;
if (this.pcs != null)
this.pcs.firePropertyChange("bootstrapContext", oldValue, value);
return (T) this;
}
/**
* Specifies the module from which resource adapter will be loaded
*/
@ModelNodeBinding(detypedName = "module")
public String module() {
return this.module;
}
/**
* Specifies the module from which resource adapter will be loaded
*/
@SuppressWarnings("unchecked")
public T module(java.lang.String value) {
Object oldValue = this.module;
this.module = value;
if (this.pcs != null)
this.pcs.firePropertyChange("module", oldValue, value);
return (T) this;
}
/**
* Define whether runtime statistics are enabled or not.
*/
@ModelNodeBinding(detypedName = "statistics-enabled")
public Boolean statisticsEnabled() {
return this.statisticsEnabled;
}
/**
* Define whether runtime statistics are enabled or not.
*/
@SuppressWarnings("unchecked")
public T statisticsEnabled(java.lang.Boolean value) {
Object oldValue = this.statisticsEnabled;
this.statisticsEnabled = value;
if (this.pcs != null)
this.pcs.firePropertyChange("statisticsEnabled", oldValue, value);
return (T) this;
}
/**
* Specifies the transaction support level of the resource adapter.
*/
@ModelNodeBinding(detypedName = "transaction-support")
public TransactionSupport transactionSupport() {
return this.transactionSupport;
}
/**
* Specifies the transaction support level of the resource adapter.
*/
@SuppressWarnings("unchecked")
public T transactionSupport(TransactionSupport value) {
Object oldValue = this.transactionSupport;
this.transactionSupport = value;
if (this.pcs != null)
this.pcs.firePropertyChange("transactionSupport", oldValue, value);
return (T) this;
}
/**
* Defines the name of the Elytron security domain that should be used.
*/
@ModelNodeBinding(detypedName = "wm-elytron-security-domain")
public String wmElytronSecurityDomain() {
return this.wmElytronSecurityDomain;
}
/**
* Defines the name of the Elytron security domain that should be used.
*/
@SuppressWarnings("unchecked")
public T wmElytronSecurityDomain(java.lang.String value) {
Object oldValue = this.wmElytronSecurityDomain;
this.wmElytronSecurityDomain = value;
if (this.pcs != null)
this.pcs.firePropertyChange("wmElytronSecurityDomain", oldValue,
value);
return (T) this;
}
/**
* Toggle on/off wm.security for this resource adapter. In case of false all
* wm-security-* parameters are ignored, even the defaults.
*/
@ModelNodeBinding(detypedName = "wm-security")
public Boolean wmSecurity() {
return this.wmSecurity;
}
/**
* Toggle on/off wm.security for this resource adapter. In case of false all
* wm-security-* parameters are ignored, even the defaults.
*/
@SuppressWarnings("unchecked")
public T wmSecurity(java.lang.Boolean value) {
Object oldValue = this.wmSecurity;
this.wmSecurity = value;
if (this.pcs != null)
this.pcs.firePropertyChange("wmSecurity", oldValue, value);
return (T) this;
}
/**
* Defines a default groups list that should be added to the used Subject
* instance.
*/
@ModelNodeBinding(detypedName = "wm-security-default-groups")
public List wmSecurityDefaultGroups() {
return this.wmSecurityDefaultGroups;
}
/**
* Defines a default groups list that should be added to the used Subject
* instance.
*/
@SuppressWarnings("unchecked")
public T wmSecurityDefaultGroups(java.util.List value) {
Object oldValue = this.wmSecurityDefaultGroups;
this.wmSecurityDefaultGroups = value;
if (this.pcs != null)
this.pcs.firePropertyChange("wmSecurityDefaultGroups", oldValue,
value);
return (T) this;
}
/**
* Defines a default groups list that should be added to the used Subject
* instance.
*/
@SuppressWarnings("unchecked")
public T wmSecurityDefaultGroup(String value) {
if (this.wmSecurityDefaultGroups == null) {
this.wmSecurityDefaultGroups = new java.util.ArrayList<>();
}
this.wmSecurityDefaultGroups.add(value);
return (T) this;
}
/**
* Defines a default groups list that should be added to the used Subject
* instance.
*/
@SuppressWarnings("unchecked")
public T wmSecurityDefaultGroups(String... args) {
wmSecurityDefaultGroups(Arrays.stream(args)
.collect(Collectors.toList()));
return (T) this;
}
/**
* Defines a default principal name that should be added to the used Subject
* instance.
*/
@ModelNodeBinding(detypedName = "wm-security-default-principal")
public String wmSecurityDefaultPrincipal() {
return this.wmSecurityDefaultPrincipal;
}
/**
* Defines a default principal name that should be added to the used Subject
* instance.
*/
@SuppressWarnings("unchecked")
public T wmSecurityDefaultPrincipal(java.lang.String value) {
Object oldValue = this.wmSecurityDefaultPrincipal;
this.wmSecurityDefaultPrincipal = value;
if (this.pcs != null)
this.pcs.firePropertyChange("wmSecurityDefaultPrincipal", oldValue,
value);
return (T) this;
}
/**
* Defines the name of the PicketBox security domain that should be used.
*/
@ModelNodeBinding(detypedName = "wm-security-domain")
public String wmSecurityDomain() {
return this.wmSecurityDomain;
}
/**
* Defines the name of the PicketBox security domain that should be used.
*/
@SuppressWarnings("unchecked")
public T wmSecurityDomain(java.lang.String value) {
Object oldValue = this.wmSecurityDomain;
this.wmSecurityDomain = value;
if (this.pcs != null)
this.pcs.firePropertyChange("wmSecurityDomain", oldValue, value);
return (T) this;
}
/**
* List of groups mappings.
*/
@ModelNodeBinding(detypedName = "wm-security-mapping-groups")
public List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy