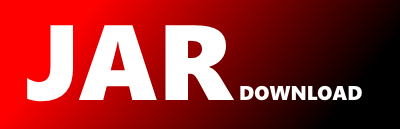
io.thundra.swark.jdbc.spring.JdbcConfiguration Maven / Gradle / Ivy
package io.thundra.swark.jdbc.spring;
import io.thundra.swark.jdbc.spring.monitoring.MonitoredJdbcTemplate;
import io.thundra.swark.jdbc.spring.monitoring.MonitoredNamedParameterJdbcTemplate;
import lombok.Data;
import org.apache.commons.dbcp2.BasicDataSource;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.jdbc.core.JdbcTemplate;
import org.springframework.jdbc.core.namedparam.NamedParameterJdbcTemplate;
import org.springframework.jdbc.datasource.DataSourceTransactionManager;
import org.springframework.transaction.support.ResourceTransactionManager;
import org.springframework.util.StringUtils;
import javax.annotation.PostConstruct;
import javax.sql.DataSource;
/**
* @author serkan
*/
@Data
@Configuration
public class JdbcConfiguration {
@Value("${jdbc.driverClassName:}")
private String driverClassName;
@Value("${jdbc.url:}")
private String url;
@Value("${jdbc.username:}")
private String username;
@Value("${jdbc.password:}")
private String password;
private DataSource defaultDataSource;
@PostConstruct
void init() {
defaultDataSource = createDefaultDataSource();
}
public DataSource createDefaultDataSource() {
if (StringUtils.isEmpty(driverClassName) || StringUtils.isEmpty(url)) {
return null;
}
BasicDataSource dataSource = new BasicDataSource();
dataSource.setDriverClassName(driverClassName);
dataSource.setUrl(url);
dataSource.setUsername(username);
dataSource.setPassword(password);
return dataSource;
}
@Bean
public JdbcTemplate jdbcTemplate(@Autowired(required = false) DataSource dataSource) {
if (dataSource == null) {
dataSource = defaultDataSource;
}
if (dataSource == null) {
return null;
}
return new MonitoredJdbcTemplate(dataSource);
}
@Bean
public NamedParameterJdbcTemplate namedParameterJdbcTemplate(@Autowired(required = false) DataSource dataSource) {
if (dataSource == null) {
dataSource = defaultDataSource;
}
return new MonitoredNamedParameterJdbcTemplate(dataSource);
}
@Bean
public ResourceTransactionManager resourceTransactionManager(@Autowired(required = false) DataSource dataSource) {
if (dataSource == null) {
dataSource = defaultDataSource;
}
return new DataSourceTransactionManager(dataSource);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy