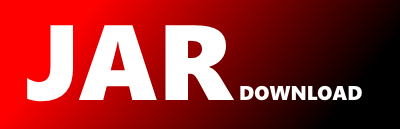
io.tiledb.cloud.rest_api.model.FragmentMetadata Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tiledb-cloud-java Show documentation
Show all versions of tiledb-cloud-java Show documentation
The Java client for the TileDB Cloud Service
The newest version!
/*
* TileDB Storage Platform API
* TileDB Storage Platform REST API
*
* The version of the OpenAPI document: 2.2.19
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package io.tiledb.cloud.rest_api.model;
import java.util.Objects;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import com.google.gson.Gson;
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
import com.google.gson.TypeAdapterFactory;
import com.google.gson.reflect.TypeToken;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Map;
import io.tiledb.cloud.rest_api.JSON;
/**
* Fragment Metadata
*/
@ApiModel(description = "Fragment Metadata")
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2023-10-02T12:25:58.319138+03:00[Europe/Athens]")
public class FragmentMetadata {
public static final String SERIALIZED_NAME_FILE_SIZES = "fileSizes";
@SerializedName(SERIALIZED_NAME_FILE_SIZES)
private List fileSizes = null;
public static final String SERIALIZED_NAME_FILE_VAR_SIZES = "fileVarSizes";
@SerializedName(SERIALIZED_NAME_FILE_VAR_SIZES)
private List fileVarSizes = null;
public static final String SERIALIZED_NAME_FILE_VALIDITY_SIZES = "fileValiditySizes";
@SerializedName(SERIALIZED_NAME_FILE_VALIDITY_SIZES)
private List fileValiditySizes = null;
public static final String SERIALIZED_NAME_FRAGMENT_URI = "fragmentUri";
@SerializedName(SERIALIZED_NAME_FRAGMENT_URI)
private String fragmentUri;
public static final String SERIALIZED_NAME_HAS_TIMESTAMPS = "hasTimestamps";
@SerializedName(SERIALIZED_NAME_HAS_TIMESTAMPS)
private Boolean hasTimestamps;
public static final String SERIALIZED_NAME_HAS_DELETE_META = "hasDeleteMeta";
@SerializedName(SERIALIZED_NAME_HAS_DELETE_META)
private Boolean hasDeleteMeta;
public static final String SERIALIZED_NAME_SPARSE_TILE_NUM = "sparseTileNum";
@SerializedName(SERIALIZED_NAME_SPARSE_TILE_NUM)
private Integer sparseTileNum;
public static final String SERIALIZED_NAME_TILE_INDEX_BASE = "tileIndexBase";
@SerializedName(SERIALIZED_NAME_TILE_INDEX_BASE)
private Integer tileIndexBase;
public static final String SERIALIZED_NAME_TILE_OFFSETS = "tileOffsets";
@SerializedName(SERIALIZED_NAME_TILE_OFFSETS)
private List> tileOffsets = null;
public static final String SERIALIZED_NAME_TILE_VAR_OFFSETS = "tileVarOffsets";
@SerializedName(SERIALIZED_NAME_TILE_VAR_OFFSETS)
private List> tileVarOffsets = null;
public static final String SERIALIZED_NAME_TILE_VAR_SIZES = "tileVarSizes";
@SerializedName(SERIALIZED_NAME_TILE_VAR_SIZES)
private List> tileVarSizes = null;
public static final String SERIALIZED_NAME_TILE_VALIDITY_OFFSETS = "tileValidityOffsets";
@SerializedName(SERIALIZED_NAME_TILE_VALIDITY_OFFSETS)
private List> tileValidityOffsets = null;
public static final String SERIALIZED_NAME_TILE_MIN_BUFFER = "tileMinBuffer";
@SerializedName(SERIALIZED_NAME_TILE_MIN_BUFFER)
private List> tileMinBuffer = null;
public static final String SERIALIZED_NAME_TILE_MIN_VAR_BUFFER = "tileMinVarBuffer";
@SerializedName(SERIALIZED_NAME_TILE_MIN_VAR_BUFFER)
private List> tileMinVarBuffer = null;
public static final String SERIALIZED_NAME_TILE_MAX_BUFFER = "tileMaxBuffer";
@SerializedName(SERIALIZED_NAME_TILE_MAX_BUFFER)
private List> tileMaxBuffer = null;
public static final String SERIALIZED_NAME_TILE_MAX_VAR_BUFFER = "tileMaxVarBuffer";
@SerializedName(SERIALIZED_NAME_TILE_MAX_VAR_BUFFER)
private List> tileMaxVarBuffer = null;
public static final String SERIALIZED_NAME_TILE_SUMS = "tileSums";
@SerializedName(SERIALIZED_NAME_TILE_SUMS)
private List> tileSums = null;
public static final String SERIALIZED_NAME_TILE_NULL_COUNTS = "tileNullCounts";
@SerializedName(SERIALIZED_NAME_TILE_NULL_COUNTS)
private List> tileNullCounts = null;
public static final String SERIALIZED_NAME_FRAGMENT_MINS = "fragmentMins";
@SerializedName(SERIALIZED_NAME_FRAGMENT_MINS)
private List> fragmentMins = null;
public static final String SERIALIZED_NAME_FRAGMENT_MAXS = "fragmentMaxs";
@SerializedName(SERIALIZED_NAME_FRAGMENT_MAXS)
private List> fragmentMaxs = null;
public static final String SERIALIZED_NAME_FRAGMENT_SUMS = "fragmentSums";
@SerializedName(SERIALIZED_NAME_FRAGMENT_SUMS)
private List fragmentSums = null;
public static final String SERIALIZED_NAME_FRAGMENT_NULL_COUNTS = "fragmentNullCounts";
@SerializedName(SERIALIZED_NAME_FRAGMENT_NULL_COUNTS)
private List fragmentNullCounts = null;
public static final String SERIALIZED_NAME_VERSION = "version";
@SerializedName(SERIALIZED_NAME_VERSION)
private Integer version;
public static final String SERIALIZED_NAME_TIMESTAMP_RANGE = "timestampRange";
@SerializedName(SERIALIZED_NAME_TIMESTAMP_RANGE)
private List timestampRange = null;
public static final String SERIALIZED_NAME_LAST_TILE_CELL_NUM = "lastTileCellNum";
@SerializedName(SERIALIZED_NAME_LAST_TILE_CELL_NUM)
private Integer lastTileCellNum;
public FragmentMetadata() {
}
public FragmentMetadata fileSizes(List fileSizes) {
this.fileSizes = fileSizes;
return this;
}
public FragmentMetadata addFileSizesItem(Integer fileSizesItem) {
if (this.fileSizes == null) {
this.fileSizes = new ArrayList<>();
}
this.fileSizes.add(fileSizesItem);
return this;
}
/**
* fixed sizes
* @return fileSizes
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "fixed sizes")
public List getFileSizes() {
return fileSizes;
}
public void setFileSizes(List fileSizes) {
this.fileSizes = fileSizes;
}
public FragmentMetadata fileVarSizes(List fileVarSizes) {
this.fileVarSizes = fileVarSizes;
return this;
}
public FragmentMetadata addFileVarSizesItem(Integer fileVarSizesItem) {
if (this.fileVarSizes == null) {
this.fileVarSizes = new ArrayList<>();
}
this.fileVarSizes.add(fileVarSizesItem);
return this;
}
/**
* var length sizes
* @return fileVarSizes
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "var length sizes")
public List getFileVarSizes() {
return fileVarSizes;
}
public void setFileVarSizes(List fileVarSizes) {
this.fileVarSizes = fileVarSizes;
}
public FragmentMetadata fileValiditySizes(List fileValiditySizes) {
this.fileValiditySizes = fileValiditySizes;
return this;
}
public FragmentMetadata addFileValiditySizesItem(Integer fileValiditySizesItem) {
if (this.fileValiditySizes == null) {
this.fileValiditySizes = new ArrayList<>();
}
this.fileValiditySizes.add(fileValiditySizesItem);
return this;
}
/**
* validity sizes
* @return fileValiditySizes
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "validity sizes")
public List getFileValiditySizes() {
return fileValiditySizes;
}
public void setFileValiditySizes(List fileValiditySizes) {
this.fileValiditySizes = fileValiditySizes;
}
public FragmentMetadata fragmentUri(String fragmentUri) {
this.fragmentUri = fragmentUri;
return this;
}
/**
* Get fragmentUri
* @return fragmentUri
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getFragmentUri() {
return fragmentUri;
}
public void setFragmentUri(String fragmentUri) {
this.fragmentUri = fragmentUri;
}
public FragmentMetadata hasTimestamps(Boolean hasTimestamps) {
this.hasTimestamps = hasTimestamps;
return this;
}
/**
* Get hasTimestamps
* @return hasTimestamps
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public Boolean getHasTimestamps() {
return hasTimestamps;
}
public void setHasTimestamps(Boolean hasTimestamps) {
this.hasTimestamps = hasTimestamps;
}
public FragmentMetadata hasDeleteMeta(Boolean hasDeleteMeta) {
this.hasDeleteMeta = hasDeleteMeta;
return this;
}
/**
* Get hasDeleteMeta
* @return hasDeleteMeta
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public Boolean getHasDeleteMeta() {
return hasDeleteMeta;
}
public void setHasDeleteMeta(Boolean hasDeleteMeta) {
this.hasDeleteMeta = hasDeleteMeta;
}
public FragmentMetadata sparseTileNum(Integer sparseTileNum) {
this.sparseTileNum = sparseTileNum;
return this;
}
/**
* Get sparseTileNum
* @return sparseTileNum
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public Integer getSparseTileNum() {
return sparseTileNum;
}
public void setSparseTileNum(Integer sparseTileNum) {
this.sparseTileNum = sparseTileNum;
}
public FragmentMetadata tileIndexBase(Integer tileIndexBase) {
this.tileIndexBase = tileIndexBase;
return this;
}
/**
* Get tileIndexBase
* @return tileIndexBase
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public Integer getTileIndexBase() {
return tileIndexBase;
}
public void setTileIndexBase(Integer tileIndexBase) {
this.tileIndexBase = tileIndexBase;
}
public FragmentMetadata tileOffsets(List> tileOffsets) {
this.tileOffsets = tileOffsets;
return this;
}
public FragmentMetadata addTileOffsetsItem(List tileOffsetsItem) {
if (this.tileOffsets == null) {
this.tileOffsets = new ArrayList<>();
}
this.tileOffsets.add(tileOffsetsItem);
return this;
}
/**
* Get tileOffsets
* @return tileOffsets
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public List> getTileOffsets() {
return tileOffsets;
}
public void setTileOffsets(List> tileOffsets) {
this.tileOffsets = tileOffsets;
}
public FragmentMetadata tileVarOffsets(List> tileVarOffsets) {
this.tileVarOffsets = tileVarOffsets;
return this;
}
public FragmentMetadata addTileVarOffsetsItem(List tileVarOffsetsItem) {
if (this.tileVarOffsets == null) {
this.tileVarOffsets = new ArrayList<>();
}
this.tileVarOffsets.add(tileVarOffsetsItem);
return this;
}
/**
* Get tileVarOffsets
* @return tileVarOffsets
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public List> getTileVarOffsets() {
return tileVarOffsets;
}
public void setTileVarOffsets(List> tileVarOffsets) {
this.tileVarOffsets = tileVarOffsets;
}
public FragmentMetadata tileVarSizes(List> tileVarSizes) {
this.tileVarSizes = tileVarSizes;
return this;
}
public FragmentMetadata addTileVarSizesItem(List tileVarSizesItem) {
if (this.tileVarSizes == null) {
this.tileVarSizes = new ArrayList<>();
}
this.tileVarSizes.add(tileVarSizesItem);
return this;
}
/**
* Get tileVarSizes
* @return tileVarSizes
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public List> getTileVarSizes() {
return tileVarSizes;
}
public void setTileVarSizes(List> tileVarSizes) {
this.tileVarSizes = tileVarSizes;
}
public FragmentMetadata tileValidityOffsets(List> tileValidityOffsets) {
this.tileValidityOffsets = tileValidityOffsets;
return this;
}
public FragmentMetadata addTileValidityOffsetsItem(List tileValidityOffsetsItem) {
if (this.tileValidityOffsets == null) {
this.tileValidityOffsets = new ArrayList<>();
}
this.tileValidityOffsets.add(tileValidityOffsetsItem);
return this;
}
/**
* Get tileValidityOffsets
* @return tileValidityOffsets
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public List> getTileValidityOffsets() {
return tileValidityOffsets;
}
public void setTileValidityOffsets(List> tileValidityOffsets) {
this.tileValidityOffsets = tileValidityOffsets;
}
public FragmentMetadata tileMinBuffer(List> tileMinBuffer) {
this.tileMinBuffer = tileMinBuffer;
return this;
}
public FragmentMetadata addTileMinBufferItem(List tileMinBufferItem) {
if (this.tileMinBuffer == null) {
this.tileMinBuffer = new ArrayList<>();
}
this.tileMinBuffer.add(tileMinBufferItem);
return this;
}
/**
* Get tileMinBuffer
* @return tileMinBuffer
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public List> getTileMinBuffer() {
return tileMinBuffer;
}
public void setTileMinBuffer(List> tileMinBuffer) {
this.tileMinBuffer = tileMinBuffer;
}
public FragmentMetadata tileMinVarBuffer(List> tileMinVarBuffer) {
this.tileMinVarBuffer = tileMinVarBuffer;
return this;
}
public FragmentMetadata addTileMinVarBufferItem(List tileMinVarBufferItem) {
if (this.tileMinVarBuffer == null) {
this.tileMinVarBuffer = new ArrayList<>();
}
this.tileMinVarBuffer.add(tileMinVarBufferItem);
return this;
}
/**
* Get tileMinVarBuffer
* @return tileMinVarBuffer
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public List> getTileMinVarBuffer() {
return tileMinVarBuffer;
}
public void setTileMinVarBuffer(List> tileMinVarBuffer) {
this.tileMinVarBuffer = tileMinVarBuffer;
}
public FragmentMetadata tileMaxBuffer(List> tileMaxBuffer) {
this.tileMaxBuffer = tileMaxBuffer;
return this;
}
public FragmentMetadata addTileMaxBufferItem(List tileMaxBufferItem) {
if (this.tileMaxBuffer == null) {
this.tileMaxBuffer = new ArrayList<>();
}
this.tileMaxBuffer.add(tileMaxBufferItem);
return this;
}
/**
* Get tileMaxBuffer
* @return tileMaxBuffer
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public List> getTileMaxBuffer() {
return tileMaxBuffer;
}
public void setTileMaxBuffer(List> tileMaxBuffer) {
this.tileMaxBuffer = tileMaxBuffer;
}
public FragmentMetadata tileMaxVarBuffer(List> tileMaxVarBuffer) {
this.tileMaxVarBuffer = tileMaxVarBuffer;
return this;
}
public FragmentMetadata addTileMaxVarBufferItem(List tileMaxVarBufferItem) {
if (this.tileMaxVarBuffer == null) {
this.tileMaxVarBuffer = new ArrayList<>();
}
this.tileMaxVarBuffer.add(tileMaxVarBufferItem);
return this;
}
/**
* Get tileMaxVarBuffer
* @return tileMaxVarBuffer
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public List> getTileMaxVarBuffer() {
return tileMaxVarBuffer;
}
public void setTileMaxVarBuffer(List> tileMaxVarBuffer) {
this.tileMaxVarBuffer = tileMaxVarBuffer;
}
public FragmentMetadata tileSums(List> tileSums) {
this.tileSums = tileSums;
return this;
}
public FragmentMetadata addTileSumsItem(List tileSumsItem) {
if (this.tileSums == null) {
this.tileSums = new ArrayList<>();
}
this.tileSums.add(tileSumsItem);
return this;
}
/**
* Get tileSums
* @return tileSums
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public List> getTileSums() {
return tileSums;
}
public void setTileSums(List> tileSums) {
this.tileSums = tileSums;
}
public FragmentMetadata tileNullCounts(List> tileNullCounts) {
this.tileNullCounts = tileNullCounts;
return this;
}
public FragmentMetadata addTileNullCountsItem(List tileNullCountsItem) {
if (this.tileNullCounts == null) {
this.tileNullCounts = new ArrayList<>();
}
this.tileNullCounts.add(tileNullCountsItem);
return this;
}
/**
* Get tileNullCounts
* @return tileNullCounts
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public List> getTileNullCounts() {
return tileNullCounts;
}
public void setTileNullCounts(List> tileNullCounts) {
this.tileNullCounts = tileNullCounts;
}
public FragmentMetadata fragmentMins(List> fragmentMins) {
this.fragmentMins = fragmentMins;
return this;
}
public FragmentMetadata addFragmentMinsItem(List fragmentMinsItem) {
if (this.fragmentMins == null) {
this.fragmentMins = new ArrayList<>();
}
this.fragmentMins.add(fragmentMinsItem);
return this;
}
/**
* Get fragmentMins
* @return fragmentMins
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public List> getFragmentMins() {
return fragmentMins;
}
public void setFragmentMins(List> fragmentMins) {
this.fragmentMins = fragmentMins;
}
public FragmentMetadata fragmentMaxs(List> fragmentMaxs) {
this.fragmentMaxs = fragmentMaxs;
return this;
}
public FragmentMetadata addFragmentMaxsItem(List fragmentMaxsItem) {
if (this.fragmentMaxs == null) {
this.fragmentMaxs = new ArrayList<>();
}
this.fragmentMaxs.add(fragmentMaxsItem);
return this;
}
/**
* Get fragmentMaxs
* @return fragmentMaxs
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public List> getFragmentMaxs() {
return fragmentMaxs;
}
public void setFragmentMaxs(List> fragmentMaxs) {
this.fragmentMaxs = fragmentMaxs;
}
public FragmentMetadata fragmentSums(List fragmentSums) {
this.fragmentSums = fragmentSums;
return this;
}
public FragmentMetadata addFragmentSumsItem(Integer fragmentSumsItem) {
if (this.fragmentSums == null) {
this.fragmentSums = new ArrayList<>();
}
this.fragmentSums.add(fragmentSumsItem);
return this;
}
/**
* Get fragmentSums
* @return fragmentSums
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public List getFragmentSums() {
return fragmentSums;
}
public void setFragmentSums(List fragmentSums) {
this.fragmentSums = fragmentSums;
}
public FragmentMetadata fragmentNullCounts(List fragmentNullCounts) {
this.fragmentNullCounts = fragmentNullCounts;
return this;
}
public FragmentMetadata addFragmentNullCountsItem(Integer fragmentNullCountsItem) {
if (this.fragmentNullCounts == null) {
this.fragmentNullCounts = new ArrayList<>();
}
this.fragmentNullCounts.add(fragmentNullCountsItem);
return this;
}
/**
* Get fragmentNullCounts
* @return fragmentNullCounts
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public List getFragmentNullCounts() {
return fragmentNullCounts;
}
public void setFragmentNullCounts(List fragmentNullCounts) {
this.fragmentNullCounts = fragmentNullCounts;
}
public FragmentMetadata version(Integer version) {
this.version = version;
return this;
}
/**
* Get version
* @return version
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public Integer getVersion() {
return version;
}
public void setVersion(Integer version) {
this.version = version;
}
public FragmentMetadata timestampRange(List timestampRange) {
this.timestampRange = timestampRange;
return this;
}
public FragmentMetadata addTimestampRangeItem(Integer timestampRangeItem) {
if (this.timestampRange == null) {
this.timestampRange = new ArrayList<>();
}
this.timestampRange.add(timestampRangeItem);
return this;
}
/**
* Get timestampRange
* @return timestampRange
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public List getTimestampRange() {
return timestampRange;
}
public void setTimestampRange(List timestampRange) {
this.timestampRange = timestampRange;
}
public FragmentMetadata lastTileCellNum(Integer lastTileCellNum) {
this.lastTileCellNum = lastTileCellNum;
return this;
}
/**
* Get lastTileCellNum
* @return lastTileCellNum
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public Integer getLastTileCellNum() {
return lastTileCellNum;
}
public void setLastTileCellNum(Integer lastTileCellNum) {
this.lastTileCellNum = lastTileCellNum;
}
/**
* A container for additional, undeclared properties.
* This is a holder for any undeclared properties as specified with
* the 'additionalProperties' keyword in the OAS document.
*/
private Map additionalProperties;
/**
* Set the additional (undeclared) property with the specified name and value.
* If the property does not already exist, create it otherwise replace it.
*/
public FragmentMetadata putAdditionalProperty(String key, Object value) {
if (this.additionalProperties == null) {
this.additionalProperties = new HashMap();
}
this.additionalProperties.put(key, value);
return this;
}
/**
* Return the additional (undeclared) property.
*/
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Return the additional (undeclared) property with the specified name.
*/
public Object getAdditionalProperty(String key) {
if (this.additionalProperties == null) {
return null;
}
return this.additionalProperties.get(key);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
FragmentMetadata fragmentMetadata = (FragmentMetadata) o;
return Objects.equals(this.fileSizes, fragmentMetadata.fileSizes) &&
Objects.equals(this.fileVarSizes, fragmentMetadata.fileVarSizes) &&
Objects.equals(this.fileValiditySizes, fragmentMetadata.fileValiditySizes) &&
Objects.equals(this.fragmentUri, fragmentMetadata.fragmentUri) &&
Objects.equals(this.hasTimestamps, fragmentMetadata.hasTimestamps) &&
Objects.equals(this.hasDeleteMeta, fragmentMetadata.hasDeleteMeta) &&
Objects.equals(this.sparseTileNum, fragmentMetadata.sparseTileNum) &&
Objects.equals(this.tileIndexBase, fragmentMetadata.tileIndexBase) &&
Objects.equals(this.tileOffsets, fragmentMetadata.tileOffsets) &&
Objects.equals(this.tileVarOffsets, fragmentMetadata.tileVarOffsets) &&
Objects.equals(this.tileVarSizes, fragmentMetadata.tileVarSizes) &&
Objects.equals(this.tileValidityOffsets, fragmentMetadata.tileValidityOffsets) &&
Objects.equals(this.tileMinBuffer, fragmentMetadata.tileMinBuffer) &&
Objects.equals(this.tileMinVarBuffer, fragmentMetadata.tileMinVarBuffer) &&
Objects.equals(this.tileMaxBuffer, fragmentMetadata.tileMaxBuffer) &&
Objects.equals(this.tileMaxVarBuffer, fragmentMetadata.tileMaxVarBuffer) &&
Objects.equals(this.tileSums, fragmentMetadata.tileSums) &&
Objects.equals(this.tileNullCounts, fragmentMetadata.tileNullCounts) &&
Objects.equals(this.fragmentMins, fragmentMetadata.fragmentMins) &&
Objects.equals(this.fragmentMaxs, fragmentMetadata.fragmentMaxs) &&
Objects.equals(this.fragmentSums, fragmentMetadata.fragmentSums) &&
Objects.equals(this.fragmentNullCounts, fragmentMetadata.fragmentNullCounts) &&
Objects.equals(this.version, fragmentMetadata.version) &&
Objects.equals(this.timestampRange, fragmentMetadata.timestampRange) &&
Objects.equals(this.lastTileCellNum, fragmentMetadata.lastTileCellNum)&&
Objects.equals(this.additionalProperties, fragmentMetadata.additionalProperties);
}
@Override
public int hashCode() {
return Objects.hash(fileSizes, fileVarSizes, fileValiditySizes, fragmentUri, hasTimestamps, hasDeleteMeta, sparseTileNum, tileIndexBase, tileOffsets, tileVarOffsets, tileVarSizes, tileValidityOffsets, tileMinBuffer, tileMinVarBuffer, tileMaxBuffer, tileMaxVarBuffer, tileSums, tileNullCounts, fragmentMins, fragmentMaxs, fragmentSums, fragmentNullCounts, version, timestampRange, lastTileCellNum, additionalProperties);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class FragmentMetadata {\n");
sb.append(" fileSizes: ").append(toIndentedString(fileSizes)).append("\n");
sb.append(" fileVarSizes: ").append(toIndentedString(fileVarSizes)).append("\n");
sb.append(" fileValiditySizes: ").append(toIndentedString(fileValiditySizes)).append("\n");
sb.append(" fragmentUri: ").append(toIndentedString(fragmentUri)).append("\n");
sb.append(" hasTimestamps: ").append(toIndentedString(hasTimestamps)).append("\n");
sb.append(" hasDeleteMeta: ").append(toIndentedString(hasDeleteMeta)).append("\n");
sb.append(" sparseTileNum: ").append(toIndentedString(sparseTileNum)).append("\n");
sb.append(" tileIndexBase: ").append(toIndentedString(tileIndexBase)).append("\n");
sb.append(" tileOffsets: ").append(toIndentedString(tileOffsets)).append("\n");
sb.append(" tileVarOffsets: ").append(toIndentedString(tileVarOffsets)).append("\n");
sb.append(" tileVarSizes: ").append(toIndentedString(tileVarSizes)).append("\n");
sb.append(" tileValidityOffsets: ").append(toIndentedString(tileValidityOffsets)).append("\n");
sb.append(" tileMinBuffer: ").append(toIndentedString(tileMinBuffer)).append("\n");
sb.append(" tileMinVarBuffer: ").append(toIndentedString(tileMinVarBuffer)).append("\n");
sb.append(" tileMaxBuffer: ").append(toIndentedString(tileMaxBuffer)).append("\n");
sb.append(" tileMaxVarBuffer: ").append(toIndentedString(tileMaxVarBuffer)).append("\n");
sb.append(" tileSums: ").append(toIndentedString(tileSums)).append("\n");
sb.append(" tileNullCounts: ").append(toIndentedString(tileNullCounts)).append("\n");
sb.append(" fragmentMins: ").append(toIndentedString(fragmentMins)).append("\n");
sb.append(" fragmentMaxs: ").append(toIndentedString(fragmentMaxs)).append("\n");
sb.append(" fragmentSums: ").append(toIndentedString(fragmentSums)).append("\n");
sb.append(" fragmentNullCounts: ").append(toIndentedString(fragmentNullCounts)).append("\n");
sb.append(" version: ").append(toIndentedString(version)).append("\n");
sb.append(" timestampRange: ").append(toIndentedString(timestampRange)).append("\n");
sb.append(" lastTileCellNum: ").append(toIndentedString(lastTileCellNum)).append("\n");
sb.append(" additionalProperties: ").append(toIndentedString(additionalProperties)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
public static HashSet openapiFields;
public static HashSet openapiRequiredFields;
static {
// a set of all properties/fields (JSON key names)
openapiFields = new HashSet();
openapiFields.add("fileSizes");
openapiFields.add("fileVarSizes");
openapiFields.add("fileValiditySizes");
openapiFields.add("fragmentUri");
openapiFields.add("hasTimestamps");
openapiFields.add("hasDeleteMeta");
openapiFields.add("sparseTileNum");
openapiFields.add("tileIndexBase");
openapiFields.add("tileOffsets");
openapiFields.add("tileVarOffsets");
openapiFields.add("tileVarSizes");
openapiFields.add("tileValidityOffsets");
openapiFields.add("tileMinBuffer");
openapiFields.add("tileMinVarBuffer");
openapiFields.add("tileMaxBuffer");
openapiFields.add("tileMaxVarBuffer");
openapiFields.add("tileSums");
openapiFields.add("tileNullCounts");
openapiFields.add("fragmentMins");
openapiFields.add("fragmentMaxs");
openapiFields.add("fragmentSums");
openapiFields.add("fragmentNullCounts");
openapiFields.add("version");
openapiFields.add("timestampRange");
openapiFields.add("lastTileCellNum");
// a set of required properties/fields (JSON key names)
openapiRequiredFields = new HashSet();
}
/**
* Validates the JSON Object and throws an exception if issues found
*
* @param jsonObj JSON Object
* @throws IOException if the JSON Object is invalid with respect to FragmentMetadata
*/
public static void validateJsonObject(JsonObject jsonObj) throws IOException {
if (jsonObj == null) {
if (FragmentMetadata.openapiRequiredFields.isEmpty()) {
return;
} else { // has required fields
throw new IllegalArgumentException(String.format("The required field(s) %s in FragmentMetadata is not found in the empty JSON string", FragmentMetadata.openapiRequiredFields.toString()));
}
}
// ensure the json data is an array
if ((jsonObj.get("fileSizes") != null && !jsonObj.get("fileSizes").isJsonNull()) && !jsonObj.get("fileSizes").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `fileSizes` to be an array in the JSON string but got `%s`", jsonObj.get("fileSizes").toString()));
}
// ensure the json data is an array
if ((jsonObj.get("fileVarSizes") != null && !jsonObj.get("fileVarSizes").isJsonNull()) && !jsonObj.get("fileVarSizes").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `fileVarSizes` to be an array in the JSON string but got `%s`", jsonObj.get("fileVarSizes").toString()));
}
// ensure the json data is an array
if ((jsonObj.get("fileValiditySizes") != null && !jsonObj.get("fileValiditySizes").isJsonNull()) && !jsonObj.get("fileValiditySizes").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `fileValiditySizes` to be an array in the JSON string but got `%s`", jsonObj.get("fileValiditySizes").toString()));
}
if ((jsonObj.get("fragmentUri") != null && !jsonObj.get("fragmentUri").isJsonNull()) && !jsonObj.get("fragmentUri").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `fragmentUri` to be a primitive type in the JSON string but got `%s`", jsonObj.get("fragmentUri").toString()));
}
// ensure the json data is an array
if ((jsonObj.get("tileOffsets") != null && !jsonObj.get("tileOffsets").isJsonNull()) && !jsonObj.get("tileOffsets").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `tileOffsets` to be an array in the JSON string but got `%s`", jsonObj.get("tileOffsets").toString()));
}
// ensure the json data is an array
if ((jsonObj.get("tileVarOffsets") != null && !jsonObj.get("tileVarOffsets").isJsonNull()) && !jsonObj.get("tileVarOffsets").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `tileVarOffsets` to be an array in the JSON string but got `%s`", jsonObj.get("tileVarOffsets").toString()));
}
// ensure the json data is an array
if ((jsonObj.get("tileVarSizes") != null && !jsonObj.get("tileVarSizes").isJsonNull()) && !jsonObj.get("tileVarSizes").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `tileVarSizes` to be an array in the JSON string but got `%s`", jsonObj.get("tileVarSizes").toString()));
}
// ensure the json data is an array
if ((jsonObj.get("tileValidityOffsets") != null && !jsonObj.get("tileValidityOffsets").isJsonNull()) && !jsonObj.get("tileValidityOffsets").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `tileValidityOffsets` to be an array in the JSON string but got `%s`", jsonObj.get("tileValidityOffsets").toString()));
}
// ensure the json data is an array
if ((jsonObj.get("tileMinBuffer") != null && !jsonObj.get("tileMinBuffer").isJsonNull()) && !jsonObj.get("tileMinBuffer").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `tileMinBuffer` to be an array in the JSON string but got `%s`", jsonObj.get("tileMinBuffer").toString()));
}
// ensure the json data is an array
if ((jsonObj.get("tileMinVarBuffer") != null && !jsonObj.get("tileMinVarBuffer").isJsonNull()) && !jsonObj.get("tileMinVarBuffer").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `tileMinVarBuffer` to be an array in the JSON string but got `%s`", jsonObj.get("tileMinVarBuffer").toString()));
}
// ensure the json data is an array
if ((jsonObj.get("tileMaxBuffer") != null && !jsonObj.get("tileMaxBuffer").isJsonNull()) && !jsonObj.get("tileMaxBuffer").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `tileMaxBuffer` to be an array in the JSON string but got `%s`", jsonObj.get("tileMaxBuffer").toString()));
}
// ensure the json data is an array
if ((jsonObj.get("tileMaxVarBuffer") != null && !jsonObj.get("tileMaxVarBuffer").isJsonNull()) && !jsonObj.get("tileMaxVarBuffer").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `tileMaxVarBuffer` to be an array in the JSON string but got `%s`", jsonObj.get("tileMaxVarBuffer").toString()));
}
// ensure the json data is an array
if ((jsonObj.get("tileSums") != null && !jsonObj.get("tileSums").isJsonNull()) && !jsonObj.get("tileSums").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `tileSums` to be an array in the JSON string but got `%s`", jsonObj.get("tileSums").toString()));
}
// ensure the json data is an array
if ((jsonObj.get("tileNullCounts") != null && !jsonObj.get("tileNullCounts").isJsonNull()) && !jsonObj.get("tileNullCounts").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `tileNullCounts` to be an array in the JSON string but got `%s`", jsonObj.get("tileNullCounts").toString()));
}
// ensure the json data is an array
if ((jsonObj.get("fragmentMins") != null && !jsonObj.get("fragmentMins").isJsonNull()) && !jsonObj.get("fragmentMins").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `fragmentMins` to be an array in the JSON string but got `%s`", jsonObj.get("fragmentMins").toString()));
}
// ensure the json data is an array
if ((jsonObj.get("fragmentMaxs") != null && !jsonObj.get("fragmentMaxs").isJsonNull()) && !jsonObj.get("fragmentMaxs").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `fragmentMaxs` to be an array in the JSON string but got `%s`", jsonObj.get("fragmentMaxs").toString()));
}
// ensure the json data is an array
if ((jsonObj.get("fragmentSums") != null && !jsonObj.get("fragmentSums").isJsonNull()) && !jsonObj.get("fragmentSums").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `fragmentSums` to be an array in the JSON string but got `%s`", jsonObj.get("fragmentSums").toString()));
}
// ensure the json data is an array
if ((jsonObj.get("fragmentNullCounts") != null && !jsonObj.get("fragmentNullCounts").isJsonNull()) && !jsonObj.get("fragmentNullCounts").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `fragmentNullCounts` to be an array in the JSON string but got `%s`", jsonObj.get("fragmentNullCounts").toString()));
}
// ensure the json data is an array
if ((jsonObj.get("timestampRange") != null && !jsonObj.get("timestampRange").isJsonNull()) && !jsonObj.get("timestampRange").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `timestampRange` to be an array in the JSON string but got `%s`", jsonObj.get("timestampRange").toString()));
}
}
public static class CustomTypeAdapterFactory implements TypeAdapterFactory {
@SuppressWarnings("unchecked")
@Override
public TypeAdapter create(Gson gson, TypeToken type) {
if (!FragmentMetadata.class.isAssignableFrom(type.getRawType())) {
return null; // this class only serializes 'FragmentMetadata' and its subtypes
}
final TypeAdapter elementAdapter = gson.getAdapter(JsonElement.class);
final TypeAdapter thisAdapter
= gson.getDelegateAdapter(this, TypeToken.get(FragmentMetadata.class));
return (TypeAdapter) new TypeAdapter() {
@Override
public void write(JsonWriter out, FragmentMetadata value) throws IOException {
JsonObject obj = thisAdapter.toJsonTree(value).getAsJsonObject();
obj.remove("additionalProperties");
// serialize additonal properties
if (value.getAdditionalProperties() != null) {
for (Map.Entry entry : value.getAdditionalProperties().entrySet()) {
if (entry.getValue() instanceof String)
obj.addProperty(entry.getKey(), (String) entry.getValue());
else if (entry.getValue() instanceof Number)
obj.addProperty(entry.getKey(), (Number) entry.getValue());
else if (entry.getValue() instanceof Boolean)
obj.addProperty(entry.getKey(), (Boolean) entry.getValue());
else if (entry.getValue() instanceof Character)
obj.addProperty(entry.getKey(), (Character) entry.getValue());
else {
obj.add(entry.getKey(), gson.toJsonTree(entry.getValue()).getAsJsonObject());
}
}
}
elementAdapter.write(out, obj);
}
@Override
public FragmentMetadata read(JsonReader in) throws IOException {
JsonObject jsonObj = elementAdapter.read(in).getAsJsonObject();
validateJsonObject(jsonObj);
// store additional fields in the deserialized instance
FragmentMetadata instance = thisAdapter.fromJsonTree(jsonObj);
for (Map.Entry entry : jsonObj.entrySet()) {
if (!openapiFields.contains(entry.getKey())) {
if (entry.getValue().isJsonPrimitive()) { // primitive type
if (entry.getValue().getAsJsonPrimitive().isString())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsString());
else if (entry.getValue().getAsJsonPrimitive().isNumber())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsNumber());
else if (entry.getValue().getAsJsonPrimitive().isBoolean())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsBoolean());
else
throw new IllegalArgumentException(String.format("The field `%s` has unknown primitive type. Value: %s", entry.getKey(), entry.getValue().toString()));
} else { // non-primitive type
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), HashMap.class));
}
}
}
return instance;
}
}.nullSafe();
}
}
/**
* Create an instance of FragmentMetadata given an JSON string
*
* @param jsonString JSON string
* @return An instance of FragmentMetadata
* @throws IOException if the JSON string is invalid with respect to FragmentMetadata
*/
public static FragmentMetadata fromJson(String jsonString) throws IOException {
return JSON.getGson().fromJson(jsonString, FragmentMetadata.class);
}
/**
* Convert an instance of FragmentMetadata to an JSON string
*
* @return JSON string
*/
public String toJson() {
return JSON.getGson().toJson(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy